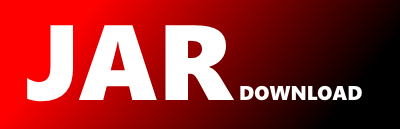
xdev.ui.XdevTabbedPane Maven / Gradle / Ivy
/*
* XDEV Application Framework - XDEV Application Framework
* Copyright © 2003 XDEV Software (https://xdev.software)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package xdev.ui;
import java.awt.Component;
import javax.swing.Icon;
import javax.swing.JTabbedPane;
import xdev.lang.NotNull;
import xdev.ui.persistence.Persistable;
/**
* The standard tabbed pane in XDEV. Based on {@link JTabbedPane}.
*
* @see JTabbedPane
*
* @author XDEV Software
*
* @since 2.0
*/
@BeanSettings(acceptChildren = true, useXdevCustomizer = true)
@XdevTabbedPaneSettings(tabType = XdevTab.class)
public class XdevTabbedPane extends JTabbedPane implements Persistable
{
/**
* serialVersionUID.
*/
private static final long serialVersionUID = 1601949782765071454L;
/**
* Should the gui state be persisted? Defaults to {@code true}.
*/
private boolean persistenceEnabled = true;
/**
* Creates an empty TabbedPane
with a default tab placement of
* JTabbedPane.TOP
.
*/
public XdevTabbedPane()
{
super();
}
/**
* Creates an empty TabbedPane
with the specified tab placement
* of either: JTabbedPane.TOP
, JTabbedPane.BOTTOM
,
* JTabbedPane.LEFT
, or JTabbedPane.RIGHT
.
*
* @param tabPlacement
* the placement for the tabs relative to the content
*
*/
public XdevTabbedPane(int tabPlacement)
{
super(tabPlacement);
}
/**
* Creates an empty TabbedPane
with the specified tab placement
* and tab layout policy. Tab placement may be either:
* JTabbedPane.TOP
, JTabbedPane.BOTTOM
,
* JTabbedPane.LEFT
, or JTabbedPane.RIGHT
. Tab
* layout policy may be either: JTabbedPane.WRAP_TAB_LAYOUT
or
* JTabbedPane.SCROLL_TAB_LAYOUT
.
*
* @param tabPlacement
* the placement for the tabs relative to the content
* @param tabLayoutPolicy
* the policy for laying out tabs when all tabs will not fit on
* one run
* @throws IllegalArgumentException
* if tab placement or tab layout policy are not one of the
* above supported values
*
*
*/
public XdevTabbedPane(int tabPlacement, int tabLayoutPolicy) throws IllegalArgumentException
{
super(tabPlacement,tabLayoutPolicy);
}
/**
* Adds a {@link XdevTab} to the this {@link XdevTabbedPane}.
*
* @param tab
* the {@link XdevTab} to add
*
* @throws NullPointerException
* if tab
is null
*
*/
public void addTab(@NotNull XdevTab tab) throws NullPointerException
{
addTab(tab.getTitle(),tab.getIcon(),tab,tab.getToolTipText());
}
/**
* {@inheritDoc}
*/
@Override
public void insertTab(String title, Icon icon, Component component, String tip, int index)
{
super.insertTab(title,icon,component,tip,index);
setEnabledAt(index,component.isEnabled());
if(component instanceof XdevTab)
{
((XdevTab)component).setIndex(index);
}
}
/**
* Returns the component at index
.
*
* @param index
* the index of the item being queried
* @return the Component
at index
* @throws IndexOutOfBoundsException
* if index is out of range (index < 0 || index >= tab count)
*
*/
public Component getTabAt(int index) throws IndexOutOfBoundsException
{
return getComponentAt(index);
}
/**
* {@inheritDoc}
*/
@Override
public String toString()
{
String toString = UIUtils.toString(this);
if(toString != null)
{
return toString;
}
return super.toString();
}
/**
*
* Use {@link #addTab(XdevTab)} to add a {@link XdevTab} to the
* {@link XdevTabbedPane}. If use one of the "add" methods the
* {@link XdevTab} will not be displayed as tab.
*
*
* This method is only overridden to place a hint related to the use of the
* {@link #addTab(XdevTab)} method.
*
*
* {@inheritDoc}
*/
@Override
public Component add(Component comp)
{
return super.add(comp);
}
/**
*
* Use {@link #addTab(XdevTab)} to add a {@link XdevTab} to the
* {@link XdevTabbedPane}. If use one of the "add" methods the
* {@link XdevTab} will not be displayed as tab.
*
*
* This method is only overridden to place a hint related to the use of the
* {@link #addTab(XdevTab)} method.
*
*
* {@inheritDoc}
*/
@Override
public Component add(Component comp, int index)
{
return super.add(comp,index);
}
/**
*
* Use {@link #addTab(XdevTab)} to add a {@link XdevTab} to the
* {@link XdevTabbedPane}. If use one of the "add" methods the
* {@link XdevTab} will not be displayed as tab.
*
*
* This method is only overridden to place a hint related to the use of the
* {@link #addTab(XdevTab)} method.
*
*
* {@inheritDoc}
*/
@Override
public void add(Component comp, Object constraints)
{
super.add(comp,constraints);
}
/**
*
* Use {@link #addTab(XdevTab)} to add a {@link XdevTab} to the
* {@link XdevTabbedPane}. If use one of the "add" methods the
* {@link XdevTab} will not be displayed as tab.
*
*
* This method is only overridden to place a hint related to the use of the
* {@link #addTab(XdevTab)} method.
*
*
* {@inheritDoc}
*/
@Override
public void add(Component comp, Object constraints, int index)
{
super.add(comp,constraints,index);
}
/**
*
* Use {@link #addTab(XdevTab)} to add a {@link XdevTab} to the
* {@link XdevTabbedPane}. If use one of the "add" methods the
* {@link XdevTab} will not be displayed as tab.
*
*
* This method is only overridden to place a hint related to the use of the
* {@link #addTab(XdevTab)} method.
*
*
* {@inheritDoc}
*/
@Override
public Component add(String name, Component comp)
{
return super.add(name,comp);
}
/**
* {@inheritDoc}
*
* @see #savePersistentState()
*/
@Override
public void loadPersistentState(String persistentState)
{
this.setSelectedIndex(Integer.parseInt(persistentState));
}
/**
* {@inheritDoc}
*
* Persisted properties:
*
* - selected index
*
*
*/
@Override
public String savePersistentState()
{
return Integer.toString(this.getSelectedIndex());
}
/**
* Uses the name of the component as a persistent id.
*
* If no name is specified the name of the class will be used. This will
* work only for one persistent instance of the class!
*
{@inheritDoc}
*/
@Override
public String getPersistentId()
{
return (this.getName() != null) ? this.getName() : this.getClass().getSimpleName();
}
/**
* {@inheritDoc}
*/
@Override
public boolean isPersistenceEnabled()
{
return persistenceEnabled;
}
/**
* Sets the persistenceEnabled flag.
*
* @param persistenceEnabled
* the state for this instance
*/
public void setPersistenceEnabled(boolean persistenceEnabled)
{
this.persistenceEnabled = persistenceEnabled;
}
}