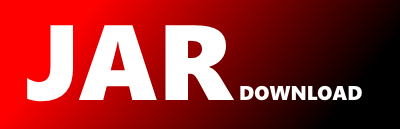
xdev.util.ArrayUtils Maven / Gradle / Ivy
/*
* XDEV Application Framework - XDEV Application Framework
* Copyright © 2003 XDEV Software (https://xdev.software)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package xdev.util;
import java.lang.reflect.Array;
import java.util.Arrays;
import java.util.Comparator;
import java.util.Iterator;
import java.util.NoSuchElementException;
import xdev.lang.LibraryMember;
import xdev.lang.Nullable;
/**
*
* The ArrayUtils
class provides utility methods for array
* handling.
*
*
* @since 2.0
*
* @author XDEV Software
*/
@LibraryMember
public final class ArrayUtils
{
/**
*
* ArrayUtils
instances can not be instantiated. The class
* should be used as utility class:
* ArrayUtils.contains(myArray, "bar");
.
*
*/
private ArrayUtils()
{
}
/**
* Checks if the two arrays are equal.
* The arrays are sorted before comparison, if they are not
* null
.
*
* @throws NullPointerException
* if an element of an array is null
*
* @param array1
* an array or null
* @param array2
* an array or null
* @param
* type of the array to compare
* @return true
if and only if the arrays have the same length
* and the array's elements are equal, false
otherwise
*/
public static > boolean equals(T[] array1, T[] array2)
throws NullPointerException
{
if(array1 == array2)
{
return true;
}
if(array1 == null || array2 == null)
{
return false;
}
if(array1.length != array2.length)
{
return false;
}
Arrays.sort(array1);
Arrays.sort(array2);
for(int i = 0; i < array1.length; i++)
{
if(!ObjectUtils.equals(array1[i],array2[i]))
{
return false;
}
}
return true;
}
/**
* Applies to: Object array
*
*
* Returns true if the specified array
contains the
* specified value
. More formally, returns true if and
* only if the specified array
contains at least one element
* value.
*
* @param array
* to search in
*
* @param value
* element whose presence in the specified array
is
* to be tested
* @return true if this array
contains the specified
* element
*/
public static boolean contains(Object[] array, Object value)
{
return indexOf(array,value) >= 0;
}
/**
* Applies to: Object array
*
*
* Returns the index of the first occurrence of the specified element
* value
in the given array, or -1 if the given
* array
does not contain the element value
.
*
*
*
* @param array
* to search in
*
* @param value
* element to search for
* @return the index of the first occurrence of the specified element in the
* given array
, or -1 if the given array
* does not contain the element
*/
public static int indexOf(Object[] array, @Nullable Object value)
{
if(value == null)
{
for(int i = 0; i < array.length; i++)
{
if(array[i] == null)
{
return i;
}
}
}
else
{
for(int i = 0; i < array.length; i++)
{
if(ObjectUtils.equals(array[i],value))
{
return i;
}
}
}
return -1;
}
/**
* Applies to: boolean array
*
*
* Returns true if the specified array
contains the
* specified value
. More formally, returns true if and
* only if the specified array
contains at least one element
* value.
*
* @param array
* to search in
*
* @param value
* element whose presence in the specified array
is
* to be tested
* @return true if this array
contains the specified
* element
*/
public static boolean contains(boolean[] array, boolean value)
{
return indexOf(array,value) >= 0;
}
/**
* Applies to: boolean array
*
*
* Returns the index of the first occurrence of the specified element
* value
in the given array, or -1 if the given
* array
does not contain the element value
.
*
*
*
* @param array
* to search in
*
* @param value
* element to search for
* @return the index of the first occurrence of the specified element in the
* given array
, or -1 if the given array
* does not contain the element
*/
public static int indexOf(boolean[] array, boolean value)
{
for(int i = 0; i < array.length; i++)
{
if(array[i] == value)
{
return i;
}
}
return -1;
}
/**
* Applies to: char array
*
*
* Returns true if the specified array
contains the
* specified value
. More formally, returns true if and
* only if the specified array
contains at least one element
* value.
*
* @param array
* to search in
*
* @param value
* element whose presence in the specified array
is
* to be tested
* @return true if this array
contains the specified
* element
*/
public static boolean contains(char[] array, char value)
{
return indexOf(array,value) >= 0;
}
/**
* Applies to: char array
*
*
* Returns the index of the first occurrence of the specified element
* value
in the given array, or -1 if the given
* array
does not contain the element value
.
*
*
*
* @param array
* to search in
*
* @param value
* element to search for
* @return the index of the first occurrence of the specified element in the
* given array
, or -1 if the given array
* does not contain the element
*/
public static int indexOf(char[] array, char value)
{
for(int i = 0; i < array.length; i++)
{
if(array[i] == value)
{
return i;
}
}
return -1;
}
/**
* Applies to: byte array
*
*
* Returns true if the specified array
contains the
* specified value
. More formally, returns true if and
* only if the specified array
contains at least one element
* value.
*
* @param array
* to search in
*
* @param value
* element whose presence in the specified array
is
* to be tested
* @return true if this array
contains the specified
* element
*/
public static boolean contains(byte[] array, byte value)
{
return indexOf(array,value) >= 0;
}
/**
* Applies to: byte array
*
*
* Returns the index of the first occurrence of the specified element
* value
in the given array, or -1 if the given
* array
does not contain the element value
.
*
*
*
* @param array
* to search in
*
* @param value
* element to search for
* @return the index of the first occurrence of the specified element in the
* given array
, or -1 if the given array
* does not contain the element
*/
public static int indexOf(byte[] array, byte value)
{
for(int i = 0; i < array.length; i++)
{
if(array[i] == value)
{
return i;
}
}
return -1;
}
/**
* Applies to: short array
*
*
* Returns true if the specified array
contains the
* specified value
. More formally, returns true if and
* only if the specified array
contains at least one element
* value.
*
* @param array
* to search in
*
* @param value
* element whose presence in the specified array
is
* to be tested
* @return true if this array
contains the specified
* element
*/
public static boolean contains(short[] array, short value)
{
return indexOf(array,value) >= 0;
}
/**
* Applies to: short array
*
*
* Returns the index of the first occurrence of the specified element
* value
in the given array, or -1 if the given
* array
does not contain the element value
.
*
*
*
* @param array
* to search in
*
* @param value
* element to search for
* @return the index of the first occurrence of the specified element in the
* given array
, or -1 if the given array
* does not contain the element
*/
public static int indexOf(short[] array, short value)
{
for(int i = 0; i < array.length; i++)
{
if(array[i] == value)
{
return i;
}
}
return -1;
}
/**
* Applies to: int array
*
*
* Returns true if the specified array
contains the
* specified value
. More formally, returns true if and
* only if the specified array
contains at least one element
* value.
*
* @param array
* to search in
*
* @param value
* element whose presence in the specified array
is
* to be tested
* @return true if this array
contains the specified
* element
*/
public static boolean contains(int[] array, int value)
{
return indexOf(array,value) >= 0;
}
/**
* Applies to: int array
*
*
* Returns the index of the first occurrence of the specified element
* value
in the given array, or -1 if the given
* array
does not contain the element value
.
*
*
*
* @param array
* to search in
*
* @param value
* element to search for
* @return the index of the first occurrence of the specified element in the
* given array
, or -1 if the given array
* does not contain the element
*/
public static int indexOf(int[] array, int value)
{
for(int i = 0; i < array.length; i++)
{
if(array[i] == value)
{
return i;
}
}
return -1;
}
/**
* Applies to: long array
*
*
* Returns true if the specified array
contains the
* specified value
. More formally, returns true if and
* only if the specified array
contains at least one element
* value.
*
* @param array
* to search in
*
* @param value
* element whose presence in the specified array
is
* to be tested
* @return true if this array
contains the specified
* element
*/
public static boolean contains(long[] array, long value)
{
return indexOf(array,value) >= 0;
}
/**
* Applies to: long array
*
*
* Returns the index of the first occurrence of the specified element
* value
in the given array, or -1 if the given
* array
does not contain the element value
.
*
*
*
* @param array
* to search in
*
* @param value
* element to search for
* @return the index of the first occurrence of the specified element in the
* given array
, or -1 if the given array
* does not contain the element
*/
public static int indexOf(long[] array, long value)
{
for(int i = 0; i < array.length; i++)
{
if(array[i] == value)
{
return i;
}
}
return -1;
}
/**
* Applies to: float array
*
*
* Returns true if the specified array
contains the
* specified value
. More formally, returns true if and
* only if the specified array
contains at least one element
* value.
*
* @param array
* to search in
*
* @param value
* element whose presence in the specified array
is
* to be tested
* @return true if this array
contains the specified
* element
*/
public static boolean contains(float[] array, float value)
{
return indexOf(array,value) >= 0;
}
/**
* Applies to: float array
*
*
* Returns the index of the first occurrence of the specified element
* value
in the given array, or -1 if the given
* array
does not contain the element value
.
*
*
*
* @param array
* to search in
*
* @param value
* element to search for
* @return the index of the first occurrence of the specified element in the
* given array
, or -1 if the given array
* does not contain the element
*/
public static int indexOf(float[] array, float value)
{
for(int i = 0; i < array.length; i++)
{
if(array[i] == value)
{
return i;
}
}
return -1;
}
/**
* Applies to: double array
*
*
* Returns true if the specified array
contains the
* specified value
. More formally, returns true if and
* only if the specified array
contains at least one element
* value.
*
* @param array
* to search in
*
* @param value
* element whose presence in the specified array
is
* to be tested
* @return true if this array
contains the specified
* element
*/
public static boolean contains(double[] array, double value)
{
return indexOf(array,value) >= 0;
}
/**
* Applies to: double array
*
*
* Returns the index of the first occurrence of the specified element
* value
in the given array, or -1 if the given
* array
does not contain the element value
.
*
*
*
* @param array
* to search in
*
* @param value
* element to search for
* @return the index of the first occurrence of the specified element in the
* given array
, or -1 if the given array
* does not contain the element
*/
public static int indexOf(double[] array, double value)
{
for(int i = 0; i < array.length; i++)
{
if(array[i] == value)
{
return i;
}
}
return -1;
}
/**
* Applies to: T array
*
*
* Returns true if the specified array
contains the
* specified value
. More formally, returns true if and
* only if the specified array
contains at least one element
* value.
*
* @param
* type of the array to search in
* @param array
* to search in
*
* @param value
* element whose presence in the specified array
is
* to be tested
*
* @param c
* {@link Comparer} to use
* @return true if this array
contains the specified
* element
*
* @see Comparer
*/
public static boolean contains(T[] array, T value, Comparer c)
{
return indexOf(array,value,c) >= 0;
}
/**
* Applies to: T array
*
*
* Returns the index of the first occurrence of the specified element
* value
in the given array, or -1 if the given
* array
does not contain the element value
.
*
*
* @param
* type of the array to search in
*
* @param array
* to search in
*
* @param value
* element to search for
*
* @param c
* {@link Comparer} to use
* @return the index of the first occurrence of the specified element in the
* given array
, or -1 if the given array
* does not contain the element
* @see Comparer
*/
public static int indexOf(T[] array, T value, Comparer c)
{
if(array == null)
{
return -1;
}
for(int i = 0; i < array.length; i++)
{
if(c.equals(array[i],value))
{
return i;
}
}
return -1;
}
/**
* Applies to: T array
*
*
* Creates a (defensive) copy of the given array
.
*
*
* @param
* type of the array to copy
*
* @param data
* array
to copy
*
* @return a (defensive) copy of the given array
.
*/
public static T[] copyOf(T[] data)
{
return Arrays.copyOf(data,data.length);
}
/**
* Applies to: boolean array
*
*
* Creates a (defensive) copy of the given array
.
*
*
*
* @param data
* array
to copy
*
* @return a (defensive) copy of the given array
.
*/
public static boolean[] copyOf(boolean[] data)
{
return Arrays.copyOf(data,data.length);
}
/**
* Applies to: char array
*
*
* Creates a (defensive) copy of the given array
.
*
*
*
* @param data
* array
to copy
*
* @return a (defensive) copy of the given array
.
*/
public static char[] copyOf(char[] data)
{
return Arrays.copyOf(data,data.length);
}
/**
* Applies to: byte array
*
*
* Creates a (defensive) copy of the given array
.
*
*
*
* @param data
* array
to copy
*
* @return a (defensive) copy of the given array
.
*/
public static byte[] copy(byte[] data)
{
return Arrays.copyOf(data,data.length);
}
/**
* Applies to: short array
*
*
* Creates a (defensive) copy of the given array
.
*
*
*
* @param data
* array
to copy
*
* @return a (defensive) copy of the given array
.
*/
public static short[] copyOf(short[] data)
{
return Arrays.copyOf(data,data.length);
}
/**
* Applies to: int array
*
*
* Creates a (defensive) copy of the given array
.
*
*
*
* @param data
* array
to copy
*
* @return a (defensive) copy of the given array
.
*/
public static int[] copyOf(int[] data)
{
return Arrays.copyOf(data,data.length);
}
/**
* Applies to: long array
*
*
* Creates a (defensive) copy of the given array
.
*
*
*
* @param data
* array
to copy
*
* @return a (defensive) copy of the given array
.
*/
public static long[] copyOf(long[] data)
{
return Arrays.copyOf(data,data.length);
}
/**
* Applies to: float array
*
*
* Creates a (defensive) copy of the given array
.
*
*
*
* @param data
* array
to copy
*
* @return a (defensive) copy of the given array
.
*/
public static float[] copyOf(float[] data)
{
return Arrays.copyOf(data,data.length);
}
/**
* Applies to: double array
*
*
* Creates a (defensive) copy of the given array
.
*
*
*
* @param data
* array
to copy
*
* @return a (defensive) copy of the given array
.
*/
public static double[] copyOf(double[] data)
{
return Arrays.copyOf(data,data.length);
}
/**
* Applies to: T array and T element
*
*
* Concatenates the given array
with the given element. A new
* array is returned that contains all elements of the given
* array
and as last element the given element
* elem
. The length of the returned array will be
* givenArray.length + 1
.
*
*
* @param
* type of the array's elements
* @param type
* class of the array's elements
* @param array
* array to concatenate
* @param elem
* element to concatenate
* @return new array that contains all elements of the given
* array
and as last element the given element
* elem
.
*/
public static T[] concat(Class type, T[] array, T elem)
{
@SuppressWarnings("unchecked")
// OK, because the new instance will always be T (or a subtype of T)
T[] result = (T[])Array.newInstance(type,array.length + 1);
System.arraycopy(array,0,result,0,array.length);
result[result.length - 1] = elem;
return result;
}
/**
* Applies to: boolean array and boolean element
*
*
* Concatenates the given array
with the given element. A new
* array is returned that contains all elements of the given
* array
and as last element the given element
* elem
. The length of the returned array will be
* givenArray.length + 1
.
*
*
* @param array
* array to concatenate
* @param elem
* element to concatenate
* @return new array that contains all elements of the given
* array
and as last element the given element
* elem
.
*/
public static boolean[] concat(boolean[] array, boolean elem)
{
boolean[] result = new boolean[array.length + 1];
System.arraycopy(array,0,result,0,array.length);
result[result.length - 1] = elem;
return result;
}
/**
* Applies to: char array and char element
*
*
* Concatenates the given array
with the given element. A new
* array is returned that contains all elements of the given
* array
and as last element the given element
* elem
. The length of the returned array will be
* givenArray.length + 1
.
*
*
* @param array
* array to concatenate
* @param elem
* element to concatenate
* @return new array that contains all elements of the given
* array
and as last element the given element
* elem
.
*/
public static char[] concat(char[] array, char elem)
{
char[] result = new char[array.length + 1];
System.arraycopy(array,0,result,0,array.length);
result[result.length - 1] = elem;
return result;
}
/**
* Applies to: byte array and byte element
*
*
* Concatenates the given array
with the given element. A new
* array is returned that contains all elements of the given
* array
and as last element the given element
* elem
. The length of the returned array will be
* givenArray.length + 1
.
*
*
* @param array
* array to concatenate
* @param elem
* element to concatenate
* @return new array that contains all elements of the given
* array
and as last element the given element
* elem
.
*/
public static byte[] concat(byte[] array, byte elem)
{
byte[] result = new byte[array.length + 1];
System.arraycopy(array,0,result,0,array.length);
result[result.length - 1] = elem;
return result;
}
/**
* Applies to: short array and short element
*
*
* Concatenates the given array
with the given element. A new
* array is returned that contains all elements of the given
* array
and as last element the given element
* elem
. The length of the returned array will be
* givenArray.length + 1
.
*
*
* @param array
* array to concatenate
* @param elem
* element to concatenate
* @return new array that contains all elements of the given
* array
and as last element the given element
* elem
.
*/
public static short[] concat(short[] array, short elem)
{
short[] result = new short[array.length + 1];
System.arraycopy(array,0,result,0,array.length);
result[result.length - 1] = elem;
return result;
}
/**
* Applies to: int array and int element
*
*
* Concatenates the given array
with the given element. A new
* array is returned that contains all elements of the given
* array
and as last element the given element
* elem
. The length of the returned array will be
* givenArray.length + 1
.
*
*
* @param array
* array to concatenate
* @param elem
* element to concatenate
* @return new array that contains all elements of the given
* array
and as last element the given element
* elem
.
*/
public static int[] concat(int[] array, int elem)
{
int[] result = new int[array.length + 1];
System.arraycopy(array,0,result,0,array.length);
result[result.length - 1] = elem;
return result;
}
/**
* Applies to: long array and long element
*
*
* Concatenates the given array
with the given element. A new
* array is returned that contains all elements of the given
* array
and as last element the given element
* elem
. The length of the returned array will be
* givenArray.length + 1
.
*
*
* @param array
* array to concatenate
* @param elem
* element to concatenate
* @return new array that contains all elements of the given
* array
and as last element the given element
* elem
.
*/
public static long[] concat(long[] array, long elem)
{
long[] result = new long[array.length + 1];
System.arraycopy(array,0,result,0,array.length);
result[result.length - 1] = elem;
return result;
}
/**
* Applies to: float array and float element
*
*
* Concatenates the given array
with the given element. A new
* array is returned that contains all elements of the given
* array
and as last element the given element
* elem
. The length of the returned array will be
* givenArray.length + 1
.
*
*
* @param array
* array to concatenate
* @param elem
* element to concatenate
* @return new array that contains all elements of the given
* array
and as last element the given element
* elem
.
*/
public static float[] concat(float[] array, float elem)
{
float[] result = new float[array.length + 1];
System.arraycopy(array,0,result,0,array.length);
result[result.length - 1] = elem;
return result;
}
/**
* Applies to: double array and double element
*
*
* Concatenates the given array
with the given element. A new
* array is returned that contains all elements of the given
* array
and as last element the given element
* elem
. The length of the returned array will be
* givenArray.length + 1
.
*
*
* @param array
* array to concatenate
* @param elem
* element to concatenate
* @return new array that contains all elements of the given
* array
and as last element the given element
* elem
.
*/
public static double[] concat(double[] array, double elem)
{
double[] result = new double[array.length + 1];
System.arraycopy(array,0,result,0,array.length);
result[result.length - 1] = elem;
return result;
}
/**
* Applies to: T arrays
*
*
* Concatenates the given arrays
. A new array is returned that
* contains all elements of the given arrays
in the order the
* arrays
were passed to the method.
*
*
*
* @param
* type of the array elements
* @param type
* class of the array elements
*
* @param arrays
* arrays to concatenate
*
* @return new array is returned that contains all elements of the given
* arrays
in the order the arrays
were
* passed to the method.
*/
public static T[] concat(Class type, T[]... arrays)
{
int len = 0;
for(int i = 0; i < arrays.length; i++)
{
len += arrays[i].length;
}
@SuppressWarnings("unchecked")
// OK, because the new instance will always be T (or a subtype of T)
T[] result = (T[])Array.newInstance(type,len);
for(int i = 0, offset = 0; i < arrays.length; i++)
{
System.arraycopy(arrays[i],0,result,offset,arrays[i].length);
offset += arrays[i].length;
}
return result;
}
/**
* Applies to: boolean arrays
*
*
* Concatenates the given arrays
. A new array is returned that
* contains all elements of the given arrays
in the order the
* arrays
were passed to the method.
*
*
* @param arrays
* arrays to concatenate
*
* @return new array is returned that contains all elements of the given
* arrays
in the order the arrays
were
* passed to the method.
*/
public static boolean[] concat(boolean[]... arrays)
{
int len = 0;
for(int i = 0; i < arrays.length; i++)
{
len += arrays[i].length;
}
boolean[] result = new boolean[len];
for(int i = 0, offset = 0; i < arrays.length; i++)
{
System.arraycopy(arrays[i],0,result,offset,arrays[i].length);
offset += arrays[i].length;
}
return result;
}
/**
* Applies to: char arrays
*
*
* Concatenates the given arrays
. A new array is returned that
* contains all elements of the given arrays
in the order the
* arrays
were passed to the method.
*
*
* @param arrays
* arrays to concatenate
*
* @return new array is returned that contains all elements of the given
* arrays
in the order the arrays
were
* passed to the method.
*/
public static char[] concat(char[]... arrays)
{
int len = 0;
for(int i = 0; i < arrays.length; i++)
{
len += arrays[i].length;
}
char[] result = new char[len];
for(int i = 0, offset = 0; i < arrays.length; i++)
{
System.arraycopy(arrays[i],0,result,offset,arrays[i].length);
offset += arrays[i].length;
}
return result;
}
/**
* Applies to: byte arrays
*
*
* Concatenates the given arrays
. A new array is returned that
* contains all elements of the given arrays
in the order the
* arrays
were passed to the method.
*
*
* @param arrays
* arrays to concatenate
*
* @return new array is returned that contains all elements of the given
* arrays
in the order the arrays
were
* passed to the method.
*/
public static byte[] concat(byte[]... arrays)
{
int len = 0;
for(int i = 0; i < arrays.length; i++)
{
len += arrays[i].length;
}
byte[] result = new byte[len];
for(int i = 0, offset = 0; i < arrays.length; i++)
{
System.arraycopy(arrays[i],0,result,offset,arrays[i].length);
offset += arrays[i].length;
}
return result;
}
/**
* Applies to: short arrays
*
*
* Concatenates the given arrays
. A new array is returned that
* contains all elements of the given arrays
in the order the
* arrays
were passed to the method.
*
*
* @param arrays
* arrays to concatenate
*
* @return new array is returned that contains all elements of the given
* arrays
in the order the arrays
were
* passed to the method.
*/
public static short[] concat(short[]... arrays)
{
int len = 0;
for(int i = 0; i < arrays.length; i++)
{
len += arrays[i].length;
}
short[] result = new short[len];
for(int i = 0, offset = 0; i < arrays.length; i++)
{
System.arraycopy(arrays[i],0,result,offset,arrays[i].length);
offset += arrays[i].length;
}
return result;
}
/**
* Applies to: int arrays
*
*
* Concatenates the given arrays
. A new array is returned that
* contains all elements of the given arrays
in the order the
* arrays
were passed to the method.
*
*
* @param arrays
* arrays to concatenate
*
* @return new array is returned that contains all elements of the given
* arrays
in the order the arrays
were
* passed to the method.
*/
public static int[] concat(int[]... arrays)
{
int len = 0;
for(int i = 0; i < arrays.length; i++)
{
len += arrays[i].length;
}
int[] result = new int[len];
for(int i = 0, offset = 0; i < arrays.length; i++)
{
System.arraycopy(arrays[i],0,result,offset,arrays[i].length);
offset += arrays[i].length;
}
return result;
}
/**
* Applies to: long arrays
*
*
* Concatenates the given arrays
. A new array is returned that
* contains all elements of the given arrays
in the order the
* arrays
were passed to the method.
*
*
* @param arrays
* arrays to concatenate
*
* @return new array is returned that contains all elements of the given
* arrays
in the order the arrays
were
* passed to the method.
*/
public static long[] concat(long[]... arrays)
{
int len = 0;
for(int i = 0; i < arrays.length; i++)
{
len += arrays[i].length;
}
long[] result = new long[len];
for(int i = 0, offset = 0; i < arrays.length; i++)
{
System.arraycopy(arrays[i],0,result,offset,arrays[i].length);
offset += arrays[i].length;
}
return result;
}
/**
* Applies to: float arrays
*
*
* Concatenates the given arrays
. A new array is returned that
* contains all elements of the given arrays
in the order the
* arrays
were passed to the method.
*
*
* @param arrays
* arrays to concatenate
*
* @return new array is returned that contains all elements of the given
* arrays
in the order the arrays
were
* passed to the method.
*/
public static float[] concat(float[]... arrays)
{
int len = 0;
for(int i = 0; i < arrays.length; i++)
{
len += arrays[i].length;
}
float[] result = new float[len];
for(int i = 0, offset = 0; i < arrays.length; i++)
{
System.arraycopy(arrays[i],0,result,offset,arrays[i].length);
offset += arrays[i].length;
}
return result;
}
/**
* Applies to: double arrays
*
*
* Concatenates the given arrays
. A new array is returned that
* contains all elements of the given arrays
in the order the
* arrays
were passed to the method.
*
*
* @param arrays
* arrays to concatenate
*
* @return new array is returned that contains all elements of the given
* arrays
in the order the arrays
were
* passed to the method.
*/
public static double[] concat(double[]... arrays)
{
int len = 0;
for(int i = 0; i < arrays.length; i++)
{
len += arrays[i].length;
}
double[] result = new double[len];
for(int i = 0, offset = 0; i < arrays.length; i++)
{
System.arraycopy(arrays[i],0,result,offset,arrays[i].length);
offset += arrays[i].length;
}
return result;
}
/**
* Applies to: T arrays
*
*
* Removes the element at the specified position from the given
* array
. Shifts any subsequent elements to the left (subtracts
* one from their indices). Returns the element that was removed from the
* given array
.
*
*
* @param
* type of the array elements
* @param type
* class of the array elements
*
* @param array
* to remove element from
* @param index
* the index of the element to be removed
* @return the element previously at the specified position
*/
public static T[] remove(Class type, T[] array, int index)
{
@SuppressWarnings("unchecked")
// OK, because the new instance will always be T (or a subtype of T)
T[] result = (T[])Array.newInstance(type,array.length - 1);
if(index == 0)
{
System.arraycopy(array,1,result,0,array.length - 1);
}
else if(index == array.length - 1)
{
System.arraycopy(array,0,result,0,array.length - 1);
}
else
{
System.arraycopy(array,0,result,0,index);
System.arraycopy(array,index + 1,result,index,array.length - index - 1);
}
return result;
}
/**
* Applies to: boolean arrays
*
*
* Removes the element at the specified position from the given
* array
. Shifts any subsequent elements to the left (subtracts
* one from their indices). Returns the element that was removed from the
* given array
.
*
*
*
* @param array
* to remove element from
* @param index
* the index of the element to be removed
* @return the element previously at the specified position
*/
public static boolean[] remove(boolean[] array, int index)
{
boolean[] result = new boolean[array.length - 1];
if(index == 0)
{
System.arraycopy(array,1,result,0,array.length - 1);
}
else if(index == array.length - 1)
{
System.arraycopy(array,0,result,0,array.length - 1);
}
else
{
System.arraycopy(array,0,result,0,index);
System.arraycopy(array,index + 1,result,index,array.length - index - 1);
}
return result;
}
/**
* Applies to: char arrays
*
*
* Removes the element at the specified position from the given
* array
. Shifts any subsequent elements to the left (subtracts
* one from their indices). Returns the element that was removed from the
* given array
.
*
*
*
* @param array
* to remove element from
* @param index
* the index of the element to be removed
* @return the element previously at the specified position
*/
public static char[] remove(char[] array, int index)
{
char[] result = new char[array.length - 1];
if(index == 0)
{
System.arraycopy(array,1,result,0,array.length - 1);
}
else if(index == array.length - 1)
{
System.arraycopy(array,0,result,0,array.length - 1);
}
else
{
System.arraycopy(array,0,result,0,index);
System.arraycopy(array,index + 1,result,index,array.length - index - 1);
}
return result;
}
/**
* Applies to: byte arrays
*
*
* Removes the element at the specified position from the given
* array
. Shifts any subsequent elements to the left (subtracts
* one from their indices). Returns the element that was removed from the
* given array
.
*
*
*
* @param array
* to remove element from
* @param index
* the index of the element to be removed
* @return the element previously at the specified position
*/
public static byte[] remove(byte[] array, int index)
{
byte[] result = new byte[array.length - 1];
if(index == 0)
{
System.arraycopy(array,1,result,0,array.length - 1);
}
else if(index == array.length - 1)
{
System.arraycopy(array,0,result,0,array.length - 1);
}
else
{
System.arraycopy(array,0,result,0,index);
System.arraycopy(array,index + 1,result,index,array.length - index - 1);
}
return result;
}
/**
* Applies to: short arrays
*
*
* Removes the element at the specified position from the given
* array
. Shifts any subsequent elements to the left (subtracts
* one from their indices). Returns the element that was removed from the
* given array
.
*
*
*
* @param array
* to remove element from
* @param index
* the index of the element to be removed
* @return the element previously at the specified position
*/
public static short[] remove(short[] array, int index)
{
short[] result = new short[array.length - 1];
if(index == 0)
{
System.arraycopy(array,1,result,0,array.length - 1);
}
else if(index == array.length - 1)
{
System.arraycopy(array,0,result,0,array.length - 1);
}
else
{
System.arraycopy(array,0,result,0,index);
System.arraycopy(array,index + 1,result,index,array.length - index - 1);
}
return result;
}
/**
* Applies to: int arrays
*
*
* Removes the element at the specified position from the given
* array
. Shifts any subsequent elements to the left (subtracts
* one from their indices). Returns the element that was removed from the
* given array
.
*
*
*
* @param array
* to remove element from
* @param index
* the index of the element to be removed
* @return the element previously at the specified position
*/
public static int[] remove(int[] array, int index)
{
int[] result = new int[array.length - 1];
if(index == 0)
{
System.arraycopy(array,1,result,0,array.length - 1);
}
else if(index == array.length - 1)
{
System.arraycopy(array,0,result,0,array.length - 1);
}
else
{
System.arraycopy(array,0,result,0,index);
System.arraycopy(array,index + 1,result,index,array.length - index - 1);
}
return result;
}
/**
* Applies to: long arrays
*
*
* Removes the element at the specified position from the given
* array
. Shifts any subsequent elements to the left (subtracts
* one from their indices). Returns the element that was removed from the
* given array
.
*
*
*
* @param array
* to remove element from
* @param index
* the index of the element to be removed
* @return the element previously at the specified position
*/
public static long[] remove(long[] array, int index)
{
long[] result = new long[array.length - 1];
if(index == 0)
{
System.arraycopy(array,1,result,0,array.length - 1);
}
else if(index == array.length - 1)
{
System.arraycopy(array,0,result,0,array.length - 1);
}
else
{
System.arraycopy(array,0,result,0,index);
System.arraycopy(array,index + 1,result,index,array.length - index - 1);
}
return result;
}
/**
* Applies to: float arrays
*
*
* Removes the element at the specified position from the given
* array
. Shifts any subsequent elements to the left (subtracts
* one from their indices). Returns the element that was removed from the
* given array
.
*
*
*
* @param array
* to remove element from
* @param index
* the index of the element to be removed
* @return the element previously at the specified position
*/
public static float[] remove(float[] array, int index)
{
float[] result = new float[array.length - 1];
if(index == 0)
{
System.arraycopy(array,1,result,0,array.length - 1);
}
else if(index == array.length - 1)
{
System.arraycopy(array,0,result,0,array.length - 1);
}
else
{
System.arraycopy(array,0,result,0,index);
System.arraycopy(array,index + 1,result,index,array.length - index - 1);
}
return result;
}
/**
* Applies to: double arrays
*
*
* Removes the element at the specified position from the given
* array
. Shifts any subsequent elements to the left (subtracts
* one from their indices). Returns the element that was removed from the
* given array
.
*
*
*
* @param array
* to remove element from
* @param index
* the index of the element to be removed
* @return the element previously at the specified position
*/
public static double[] remove(double[] array, int index)
{
double[] result = new double[array.length - 1];
if(index == 0)
{
System.arraycopy(array,1,result,0,array.length - 1);
}
else if(index == array.length - 1)
{
System.arraycopy(array,0,result,0,array.length - 1);
}
else
{
System.arraycopy(array,0,result,0,index);
System.arraycopy(array,index + 1,result,index,array.length - index - 1);
}
return result;
}
/**
* Returns a {@link Iterable} for the given array
.
*
* @param
* type of the array elements
*
*
* @param array
* to get the {@link Iterable} for
* @return a {@link Iterable} for the given array
.
*/
public static Iterable getIterable(final T[] array)
{
return getIterable(array,0,array.length);
}
/**
* Returns a {@link Iterable} for the given array
.
*
* @param
* type of the array elements
* @param offset
* the start offset of the iterator
* @param length
* the length of the iterator
*
* @param array
* to get the {@link Iterable} for
* @return a {@link Iterable} for the given array
.
*/
public static Iterable getIterable(final T[] array, final int offset, final int length)
{
return new Iterable()
{
public Iterator iterator()
{
return getIterator(array,offset,length);
}
};
}
/**
* Returns a {@link Iterator} for the given array
.
*
* {@link Iterator#remove()} is not supported.
*
* @param
* type of the array elements
*
*
* @param array
* to get the {@link Iterator} for
* @return a {@link Iterator} for the given array
.
*/
public static Iterator getIterator(final T[] array)
{
return getIterator(array,0,array.length);
}
/**
* Returns a {@link Iterator} for the given array
.
*
* {@link Iterator#remove()} is not supported.
*
* @param
* type of the array elements
* @param array
* to get the {@link Iterator} for
* @param offset
* the start offset of the iterator
* @param length
* the length of the iterator
* @return a {@link Iterator} for the given array
.
*/
public static Iterator getIterator(final T[] array, final int offset, final int length)
{
return new Iterator()
{
int index = offset;
int maxIndex = offset + length - 1;
@Override
public boolean hasNext()
{
return index <= maxIndex;
}
@Override
public T next()
{
if(index > maxIndex)
{
throw new NoSuchElementException();
}
return array[index++];
}
/**
* Always throws an {@link UnsupportedOperationException}
*/
@Override
public void remove()
{
throw new UnsupportedOperationException();
}
};
}
/**
*
* Sorts the given array
of {@link Enum}s by name.
*
* @param
* type of the array elements
* @param array
* to sort
* @see Enum
* @see Enum#name()
* @see String#compareTo(String)
*/
public static > void sortByName(E[] array)
{
Arrays.sort(array,new Comparator()
{
public int compare(E e1, E e2)
{
return e1.name().compareTo(e2.name());
}
});
}
/**
* Converts a array of wrapped {@link Byte}s to a primitive byte array.
*
* @param wrapper
* the wrapped array of {@link Byte}s
* @return a unwrapped array of bytes
* @throws NullPointerException
* if wrapper itself or one element of wrapper is
* null
*/
public static byte[] unwrap(Byte[] wrapper) throws NullPointerException
{
int c = wrapper.length;
byte[] primitive = new byte[c];
for(int i = 0; i < c; i++)
{
primitive[i] = wrapper[i];
}
return primitive;
}
/**
* Converts a array of wrapped {@link Short}s to a primitive short array.
*
* @param wrapper
* the wrapped array of {@link Short}s
* @return a unwrapped array of shorts
* @throws NullPointerException
* if wrapper itself or one element of wrapper is
* null
*/
public static short[] unwrap(Short[] wrapper) throws NullPointerException
{
int c = wrapper.length;
short[] primitive = new short[c];
for(int i = 0; i < c; i++)
{
primitive[i] = wrapper[i];
}
return primitive;
}
/**
* Converts a array of wrapped {@link Integer}s to a primitive int array.
*
* @param wrapper
* the wrapped array of {@link Integer}s
* @return a unwrapped array of ints
* @throws NullPointerException
* if wrapper itself or one element of wrapper is
* null
*/
public static int[] unwrap(Integer[] wrapper) throws NullPointerException
{
int c = wrapper.length;
int[] primitive = new int[c];
for(int i = 0; i < c; i++)
{
primitive[i] = wrapper[i];
}
return primitive;
}
/**
* Converts a array of wrapped {@link Long}s to a primitive long array.
*
* @param wrapper
* the wrapped array of {@link Long}s
* @return a unwrapped array of longs
* @throws NullPointerException
* if wrapper itself or one element of wrapper is
* null
*/
public static long[] unwrap(Long[] wrapper) throws NullPointerException
{
int c = wrapper.length;
long[] primitive = new long[c];
for(int i = 0; i < c; i++)
{
primitive[i] = wrapper[i];
}
return primitive;
}
/**
* Converts a array of wrapped {@link Boolean}s to a primitive boolean
* array.
*
* @param wrapper
* the wrapped array of {@link Boolean}s
* @return a unwrapped array of booleans
* @throws NullPointerException
* if wrapper itself or one element of wrapper is
* null
*/
public static boolean[] unwrap(Boolean[] wrapper) throws NullPointerException
{
int c = wrapper.length;
boolean[] primitive = new boolean[c];
for(int i = 0; i < c; i++)
{
primitive[i] = wrapper[i];
}
return primitive;
}
/**
* Converts a array of wrapped {@link Character}s to a primitive char array.
*
* @param wrapper
* the wrapped array of {@link Character}s
* @return a unwrapped array of chars
* @throws NullPointerException
* if wrapper itself or one element of wrapper is
* null
*/
public static char[] unwrap(Character[] wrapper) throws NullPointerException
{
int c = wrapper.length;
char[] primitive = new char[c];
for(int i = 0; i < c; i++)
{
primitive[i] = wrapper[i];
}
return primitive;
}
}