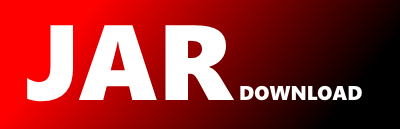
xdev.util.ParseUtils Maven / Gradle / Ivy
/*
* XDEV Application Framework - XDEV Application Framework
* Copyright © 2003 XDEV Software (https://xdev.software)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package xdev.util;
import java.awt.Image;
import java.io.File;
import java.lang.reflect.Array;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.text.DateFormat;
import java.text.DecimalFormat;
import java.text.NumberFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Collection;
import java.util.Date;
import java.util.Hashtable;
import java.util.Locale;
import java.util.Map;
import java.util.regex.Pattern;
import javax.swing.Icon;
import xdev.io.XdevFile;
import xdev.lang.LibraryMember;
import xdev.ui.GraphicUtils;
import xdev.ui.XdevImage;
/**
*
*
* The ParseUtils
class provides parsing methods for various types.
*
*
* @since 2.0
* @author XDEV Software
*
*/
@LibraryMember
public class ParseUtils
{
private ParseUtils()
{
}
/**
* Parse the variable value
to a byte
.
*
*
* ParseUtils.parseByte(7895); returns -44
* ParseUtils.parseByte(-64568); returns -56
*
*
* @param value
* the value which will be parsed to byte
* @return the byte
parsed from the value
, if
* possible.
*
* @throws NumberFormatException
* if value could not be parsed to byte
*
* @see ParseUtils#parseByte(Object, String)
* @see ParseUtils#parseDouble(Object)
*
* @deprecated use {@link ConversionUtils#tobyte(Object)}
*/
@Deprecated
public static byte parseByte(Object value) throws NumberFormatException
{
return (byte)parseDouble(value);
}
/**
* Parse the variable value
to a byte
with the
* help of of the format
. The format of the current language
* setting will be considered.
*
*
* ParseUtils.parseByte(589, "XDEV"); returns 77
* ParseUtils.parseByte(-3695, "XDEV"); returns -111
*
*
* @param value
* the value which will be parsed to byte
* @param pattern
* the format with which the value
should be parsed
* @return the byte
parsed from the value
, if
* possible.
*
* @see ParseUtils#parse(Object, String)
*
* @throws ParseException
* if value could not be parsed to byte
*/
public static byte parseByte(Object value, String pattern) throws ParseException
{
if(value instanceof Number)
{
return ((Number)value).byteValue();
}
try
{
return parse(value,pattern).byteValue();
}
catch(ParseException e)
{
throw new NumberFormatException(e.getMessage());
}
}
/**
* Parse the variable value
to a short
.The format
* of the current language setting will be considered.
*
* Examples
*
*
* ParseUtils.parseShort(2561); returns 2561
* ParseUtils.parseShort(-25615); returns -25615
*
*
*
*
* @param value
* the value which will be parsed to short
* @return the short
parsed from the value
, if
* possible.
*
* @throws NumberFormatException
* if value could not be parsed to short
*
* @see ParseUtils#parseShort(Object, String)
* @see ParseUtils#parseDouble(Object)
*
* @deprecated use {@link ConversionUtils#toshort(Object)}
*/
@Deprecated
public static short parseShort(Object value) throws NumberFormatException
{
return (short)parseDouble(value);
}
/**
* Parse the variable value
to a short
with the
* help of of the format
. The format of the current language
* setting will be considered.
*
* Examples
*
*
* ParseUtils.parseShort("0032767", "##0000"); returns 32767
* ParseUtils.parseShort("235.123", "###"); returns 235
*
*
*
*
*
* @param value
* the value which will be parsed to short
* @param pattern
* the format with which the value
should be parsed
* @return the short
parsed from the value
, if
* possible.
*
* @see ParseUtils#parse(Object, String)
*
* @throws ParseException
* if value could not be parsed to short
*/
public static short parseShort(Object value, String pattern) throws ParseException
{
if(value instanceof Number)
{
return ((Number)value).shortValue();
}
try
{
return parse(value,pattern).shortValue();
}
catch(ParseException e)
{
throw new NumberFormatException(e.getMessage());
}
}
/**
* Parse the variable value
to an int
. The format
* of the current language setting will be considered.
*
* Examples
*
*
* ParseUtils.parseInt(56); returns 56
* ParseUtils.parseInt(-268); returns -268
*
*
*
*
* @param value
* the value which will be parsed to int
* @return the int
parsed from the value
, if
* possible.
*
*
* Warning: The value has to be between
* -2.147.483.648 and 2.147.483.647. Beyond that use
* {@link ParseUtils#parseDouble(Object)}.
*
*
* @see ParseUtils#parseInt(Object, String)
* @see ParseUtils#parseDouble(Object)
* @see ParseUtils#parseDouble(Object, String)
* @see ParseUtils#isInteger(Object)
* @see ParseUtils#parseLong(Object)
* @see ParseUtils#parseLong(Object, String)
*
* @throws NumberFormatException
* if value could not be parsed to int
*
* @deprecated use {@link ConversionUtils#toint(Object)}
*/
@Deprecated
public static int parseInt(Object value) throws NumberFormatException
{
return (int)parseDouble(value);
}
/**
* Parse the variable value
to a int
with the help
* of of the format
. The format of the current language setting
* will be considered.
*
* Examples
*
*
* ParseUtils.parseInt("12.356", "##"); returns 12
* ParseUtils.parseInt("12", "##"); returns 12
*
*
*
*
* @param value
* the value which will be parsed to int
* @param pattern
* the format with which the value
should be parsed
* @return the int
parsed from the value
, if
* possible.
*
*
* Warning: The value has to be between
* -2.147.483.648 and 2.147.483.647. Beyond that use
* {@link ParseUtils#parseDouble(Object, String)}.
*
*
* @see ParseUtils#parse(Object, String)
*
* @throws ParseException
* if value could not be parsed to int
*/
public static int parseInt(Object value, String pattern) throws ParseException
{
if(value instanceof Number)
{
return ((Number)value).intValue();
}
try
{
return parse(value,pattern).intValue();
}
catch(ParseException e)
{
throw new NumberFormatException(e.getMessage());
}
}
/**
* Parse the variable value
to an long
. The format
* of the current language setting will be considered.
*
* Examples
*
*
* ParseUtils.parseLong(223545854); returns 223545854
* ParseUtils.parseLong(-545845854); returns -545845854
*
*
*
*
* @param value
* the value which will be parsed to long
* @return the long
parsed from the value
, if
* possible.
*
* @see ParseUtils#parseInt(Object)
* @see ParseUtils#parseDouble(Object)
* @see ParseUtils#parseDouble(Object)
* @see ParseUtils#isLong(Object)
* @see ParseUtils#parseInt(Object, String)
* @see ParseUtils#parseLong(Object, String)
* @see ParseUtils#parseDouble(Object, String)
*
* @throws NumberFormatException
* if value could not be parsed to long
*
* @deprecated use {@link ConversionUtils#tolong(Object)}
*/
@Deprecated
public static long parseLong(Object value) throws NumberFormatException
{
return (long)parseDouble(value);
}
/**
* Parse the variable value
to a long
with the
* help of of the format
. The format of the current language
* setting will be considered.
*
* Examples
*
*
* ParseUtils.parseLong("546545,64121.385", ",######"); returns 546545
* ParseUtils.parseLong("546872313", "########"); returns 546872313
*
*
*
*
* @param value
* the value which will be parsed to long
* @param pattern
* the format with which the value
should be parsed
* @return the long
parsed from the value
, if
* possible.
*
* @see ParseUtils#parse(Object, String)
*
* @throws ParseException
* if value could not be parsed to long
*/
public static long parseLong(Object value, String pattern) throws ParseException
{
if(value instanceof Number)
{
return ((Number)value).longValue();
}
try
{
return parse(value,pattern).longValue();
}
catch(ParseException e)
{
throw new NumberFormatException(e.getMessage());
}
}
/**
* Parse the variable value
to an float
. The
* format of the current language setting will be considered.
*
* Examples
*
*
* ParseUtils.parseFloat(545687453); returns 545687453
* ParseUtils.parseFloat(-564868745); returns -564868745
*
*
*
*
*
* @param value
* the value which will be parsed to long
* @return the float
parsed from the value
, if
* possible.
*
* @see ParseUtils#parseInt(Object)
* @see ParseUtils#parseDouble(Object)
* @see ParseUtils#parseDouble(Object)
* @see ParseUtils#parseInt(Object, String)
* @see ParseUtils#parseLong(Object, String)
* @see ParseUtils#parseDouble(Object, String)
*
* @throws NumberFormatException
* if value could not be parsed to float
*
* @deprecated use {@link ConversionUtils#tofloat(Object)}
*/
@Deprecated
public static float parseFloat(Object value) throws NumberFormatException
{
return (float)parseDouble(value);
}
/**
* Parse the variable value
to a float
with the
* help of of the format
. The format of the current language
* setting will be considered.
*
* Examples
*
*
* ParseUtils.parseFloat("00003456", "000000"); returns 3456
* ParseUtils.parseFloat("0000014", "000000"); returns 14
*
*
*
*
*
*
* @param value
* the value which will be parsed to float
* @param pattern
* the format with which the value
should be parsed
* @return the float
parsed from the value
, if
* possible.
*
* @see ParseUtils#parse(Object, String)
*
* @throws ParseException
* if value could not be parsed to float
*/
public static float parseFloat(Object value, String pattern) throws ParseException
{
if(value instanceof Number)
{
return ((Number)value).floatValue();
}
try
{
return parse(value,pattern).floatValue();
}
catch(ParseException e)
{
throw new NumberFormatException(e.getMessage());
}
}
/**
* Parse the variable value
to an double
. The
* format of the current language setting will be considered.
*
*
* ParseUtils.parseDouble(5.20); returns 5.20
* ParseUtils.parseDouble(-175.28); returns -175.28
*
*
*
*
* @param value
* the value which will be parsed to double
* @return the double
parsed from the value
, if
* possible.
*
* @see ParseUtils#parseInt(Object, String)
* @see ParseUtils#parseDouble(Object)
* @see ParseUtils#parseDouble(Object, String)
* @see ParseUtils#isDecimal(Object)
* @see ParseUtils#parseLong(Object)
* @see ParseUtils#parseLong(Object, String)
*
* @throws NumberFormatException
* if value could not be parsed to double
*
* @deprecated use {@link ConversionUtils#todouble(Object)}
*/
@Deprecated
public static double parseDouble(Object value) throws NumberFormatException
{
if(value instanceof Number)
{
return ((Number)value).doubleValue();
}
String s = String.valueOf(value);
try
{
return Double.parseDouble(s);
}
catch(Exception e)
{
try
{
return getNumberFormat().parse(s).doubleValue();
}
catch(ParseException pe)
{
throw new NumberFormatException(e.getMessage());
}
}
}
/**
* Parse the variable value
to a double
with the
* help of of the format
. The format of the current language
* setting will be considered.
*
*
* Examples
*
*
* ParseUtils.parseDouble(",35", ".00"); returns 0.35
* ParseUtils.parseDouble("12,340000","#.00"); returns 12.34
*
*
*
*
*
* @param value
* the value which will be parsed to double
* @param pattern
* the format with which the value
should be parsed
* @return the double
parsed from the value
, if
* possible.
*
* @see ParseUtils#parse(Object, String)
*
* @throws ParseException
* if value could not be parsed to double
*/
public static double parseDouble(Object value, String pattern) throws ParseException
{
if(value instanceof Number)
{
return ((Number)value).doubleValue();
}
try
{
return parse(value,pattern).doubleValue();
}
catch(ParseException e)
{
throw new NumberFormatException(e.getMessage());
}
}
/**
* Parse the variable value
to an double
.
* Additional characters behind the value
will be
* considered.
*
*
* Example
*
*
* ParseUtils.parseDouble(2587.6998); returns 2587.6998
* ParseUtils.parseDouble(-5697.6237); returns -5697.6237
*
*
* Hint
*
*
* Can be used as inverse function to {@link ParseUtils#formatAsCurrency(double)}
*
*
* Hint
*
*
* If you don't need the extra function to accepted additional characters
* behind the number you should use {@link ParseUtils#parseDouble(Object)} instead.
* Because fewer mistakes could be slipped in.
* And {@link ParseUtils#parseDouble(Object)} has more performance because fewer cases has to be checked.
*
*
*
*
* @param value
* the value which will be parsed to double
* @return the double
parsed from the value
, if
* possible.
*
* @see ParseUtils#formatAsCurrency(double)
* @see ParseUtils#parseDoubleAsCurrency(Object, String)
* @see ParseUtils#parseDouble(Object)
* @see ParseUtils#parseDouble(Object, String)
*
*/
public static double parseDoubleAsCurrency(Object value)
{
if(value instanceof Number)
{
return ((Number)value).doubleValue();
}
String s = String.valueOf(value);
try
{
return NumberFormat.getCurrencyInstance().parse(s).doubleValue();
}
catch(ParseException pe)
{
throw new NumberFormatException(pe.getMessage());
}
}
/**
* Parse the variable value
to a double
with the
* help of of the format
. The format of the current language
* setting will be considered.
*
* Example
*
*
* ParseUtils.parseDoubleAsCurrency("12,350000", "#.000000"); returns 12.35
* ParseUtils.parseDoubleAsCurrency("12.345,679", ",###"); returns 12345.679
*
*
*
* @param value
* the value which will be parsed to double
* @param pattern
* the format with which the value
should be parsed
* @return the double
parsed from the value
, if
* possible.
*
* @see ParseUtils#parse(Object, String)
* @see ParseUtils#parseDouble(Object, String)
*
* @throws ParseException
* if value could not be parsed to double
*/
public static double parseDoubleAsCurrency(Object value, String pattern) throws ParseException
{
return parseDouble(value,pattern);
}
/**
* Parse the variable value
to an Number
.
*
* Examples
*
*
* ParseUtils.parse(78); return 78
* ParseUtils.parse(-256); return -256
*
*
*
*
* @param value
* the value which will be parsed to Number
* @return the Number
parsed from the value
, if
* possible.
*
*
*
* @throws ParseException
* if value could not be parsed to Number
*
*/
public static Number parse(Object value) throws ParseException
{
if(value instanceof Number)
{
return (Number)value;
}
return getNumberFormat().parse(String.valueOf(value));
}
/**
* Parse the variable value
to an Number
with the
* help of of the format
. The format of the current language
* setting will be considered.
*
*
*
* Examples
*
*
*
* ParseUtils.parse("00000014", "00000000"); returns 14
* ParseUtils.parse("0014000", "0000000"); returns 14000
*
*
* The following Symbols will be supported:
*
*
*
* Symbol
*
* Meaning
*
*
*
* 0
*
* Represent a digit - is the position empty, will be shown
* a zero.
*
*
*
* #
*
* Represent a digit - is the position empty it stays
* empty, with that leading zero and unnecessary zeros after the decimal
* point will be avoided.
*
*
*
* .
*
* Decimal separator. Separates external and internal
* decimal places.
*
*
*
* ,
*
* Groups the digits (a group is as big as the distance
* from "," to ".").
*
*
*
* ;
*
* Delimiter. On the left of it is the pattern for the
* positive, on the right the format for the negative numbers
*
*
*
* -
*
* The standardsymbol for the negativeprefix
*
*
*
*
* %
*
* The number will be multiply with 100 and declared as a
* percent value
*
*
*
* %%
*
* The same as %, only with per mill.
*
*
*
* \u00A4 (¤)
*
*
* National currency sign (&eur; for Germany)
*
*
*
* \u00A4\u00A4 (¤¤)
*
* Internationale currency sign (EUR for Germany)
*
*
*
* X
*
* All other symbols X can be
* used as normal.
*
*
*
*
* '
*
* to mask special symbols in prefix or suffix
*
*
*
*
*
*
*
* Format
*
* Intake Number
*
* Result
*
*
*
*
* 0000
*
* 12
*
* 0012
*
*
*
* 0000
*
* 12,5
*
* 0012
*
*
*
* 0000
*
* 1234567
*
* 1234567
*
*
*
*
* ##
*
* 12
*
* 12
*
*
*
* ##
*
* 12.3456
*
* 12
*
*
*
* ##
*
* 123456
*
* 123456
*
*
*
* .00
*
* 12.3456
*
* 12,35
*
*
*
*
* .00
*
* .3456
*
* ,35
*
*
*
* 0.00
*
* .789
*
* 0,79
*
*
*
* #.000000
*
* 12.34
*
* 12,340000
*
*
*
* ,###
*
* 12345678.901
*
* 12.345.679
*
*
*
* #.#;(#.#)
*
*
* 12345678.901
*
* 12345678,9
*
*
*
* #.#;(#.#)
*
* -12345678.901
*
* (12345678,9)
*
*
*
* ,###.## \u00A4
*
* 12345.6789
*
* 12.345,68 i
*
*
*
* ,#00.00 \u00A4\u00A4
*
* -12345678.9
*
* -12.345.678,90 EUR
*
*
*
* ,#00.00 \u00A4\u00A4
*
* 0.1
*
*
* 00,10 EUR
*
*
*
*
*
* @param value
* the value which will be parsed to Number
* @param pattern
* the format with which the value
should be parsed
* @return the Number
parsed from the value
, if
* possible.
*
* @see ParseUtils#parse(Object)
* @see ParseUtils#parseInt(Object)
* @see ParseUtils#parseInt(Object, String)
* @see ParseUtils#isDecimal(Object)
*
* @throws ParseException
* if value
could not be parsed to
* Number
*/
public static Number parse(Object value, String pattern) throws ParseException
{
// Bug 13349. Numbers was never parsed.
// if(value instanceof Number)
// {
// return (Number)value;
// }
return new DecimalFormat(pattern).parse(String.valueOf(value));
}
/**
* Parse the variable value
to boolean
* .
*
*
* Returns true
for the following cases:
*
- Boolean true
* - Number != 0
* String
"true" (not case sensitive)
* All others cases != null
return false
*
*
* Example
*
* * ParseUtils.parseBoolean(232); return true * ParseUtils.parseBoolean(-232); return true * ParseUtils.parseBoolean("hello"); return false **
* Warning: Instead of {@link ParseUtils#parseInt(Object)}
* and {@link ParseUtils#parseDouble(Object)} this methode will never throw
* a NumberFormatException
*
* @param value
* the value which will be parsed to boolean
.
* @return true
if the value
is a
* boolean
, else false
*
* @see ParseUtils#isBoolean(Object)
* @see ParseUtils#parseInt(Object)
* @see ParseUtils#parseDouble(Object)
*
* @deprecated use {@link ConversionUtils#toboolean(Object)}
*/
@Deprecated
public static boolean parseBoolean(Object value)
{
boolean result = false;
if(value instanceof Boolean)
{
result = ((Boolean)value).booleanValue();
}
else
{
try
{
result = parseDouble(value) != 0;
}
catch(Exception e)
{
result = Boolean.valueOf(String.valueOf(value)).booleanValue();
}
}
return result;
}
/**
* Ckecks whether Object
value
is a
* XdevDate
. If yes it returns a XdevDate
else
* null
.
*
* @param value
* Object
which will be parsed to
* XdevDate
.
* @return XdevDate
if value
is a
* XdevDate
. Else null
.
*
* @see ParseUtils#parseDate(Object, String)
* @see ParseUtils#parseUtilDate(Object)
*
* @deprecated use {@link ConversionUtils#toXdevDate(Object)}
*/
@Deprecated
public static XdevDate parseDate(Object value)
{
if(value == null)
{
return null;
}
XdevDate date = null;
if(value instanceof XdevDate)
{
date = (XdevDate)value;
}
else if(value instanceof Date)
{
date = new XdevDate((Date)value);
}
else if(value instanceof Calendar)
{
date = new XdevDate((Calendar)value);
}
if(date != null)
{
date = date.copy();
}
return date;
}
/**
* Ckecks whether Object
value
is a
* XdevDate
. If yes it returns a XdevDate
else
* null
.
*
*
Examples * ** * @param value ** ParseUtils.parseDate("10.01.2010", "dd.MM.yyyy"); returns 10.01.2010 ** * Hint * ** For valid patterns see {@link SimpleDateFormat} ** *
Object
which will be parsed to
* XdevDate
.
* @param pattern
* the format with which the value
should be parsed.
* @return XdevDate
if value
is a
* XdevDate
. Else null
.
*
* @see ParseUtils#parseDate(Object)
* @see ParseUtils#parseUtilDate(Object)
*/
public static XdevDate parseDate(Object value, String pattern)
{
if(value == null)
{
return null;
}
DateFormat dateFormat = null;
XdevDate date = parseDate(value);
if(date == null)
{
String dateStr = String.valueOf(value);
try
{
dateFormat = new SimpleDateFormat(pattern);
Date d = dateFormat.parse(dateStr);
date = new XdevDate(d);
}
catch(ParseException e)
{
throw new DateFormatException(e.getMessage());
}
}
date.setFormat(dateFormat);
return date;
}
/**
* Ckecks whether Object
value
is a
* Date
. If yes it returns a Date
else
* null
.
*
* @param value
* Object
which will be parsed to Date
.
* @return XdevDate
if value
is a
* Date
. Else null
.
*
* @see ParseUtils#parseDate(Object, String)
* @see ParseUtils#parseDate(Object)
*
* @deprecated use {@link ConversionUtils#toDate(Object)}
*/
@Deprecated
public static Date parseUtilDate(Object value)
{
if(value == null)
{
return null;//
}
Date date = null;
if(value instanceof Date)
{
date = (Date)value;
}
else if(value instanceof Calendar)
{
date = ((Calendar)value).getTime();
}
return date;
}
/**
* Ckecks whether Object
value
is a
* XdevList
. If yes it returns a XdevList
else
* null
.
*
* @param value
* Object
which will be parsed to
* XdevList
.
* @return XdevDate
if value
is a
* XdevList
. Else null
.
*
* @deprecated use {@link ConversionUtils#toXdevList(Object)}
*/
@Deprecated
public static XdevList parseList(Object value)
{
if(value == null)
{
return null;
}
XdevList list = null;
if(value instanceof XdevList)
{
list = (XdevList)value;
}
else if(value instanceof Collection)
{
list = new XdevList((Collection)value);
}
else if(value.getClass().isArray())
{
int c = Array.getLength(value);
list = new XdevList(c);
for(int i = 0; i < c; i++)
{
list.add(Array.get(value,i));
}
}
return list;
}
/**
* Ckecks whether Object
value
is a
* XdevHashtable
. If yes it returns a
* XdevHashtable
else null
.
*
* @param value
* Object
which will be parsed to
* XdevHashtable
.
* @return XdevHashtable
if value
is a
* XdevHashtable
. Else null
.
*
* @deprecated use {@link ConversionUtils#toXdevHashtable(Object)}
*/
@Deprecated
public static XdevHashtable parseHashtable(Object value)
{
if(value == null)
{
return null;
}
XdevHashtable hashtable = null;
if(value instanceof XdevHashtable)
{
hashtable = (XdevHashtable)value;
}
else if(value instanceof Map)
{
hashtable = new XdevHashtable((Map)value);
}
else if(value.getClass().isArray() && Array.getLength(value) % 2 == 0)
{
hashtable = new XdevHashtable((Object[])value);
}
return hashtable;
}
/**
* Ckecks whether Object
value
is a
* XdevFile
. If yes it returns a XdevFile
else
* null
.
*
* @param value
* Object
which will be parsed to
* XdevFile
.
* @return XdevFile
if value
is a
* XdevFile
. Else null
.
*
* @deprecated use {@link ConversionUtils#toXdevFile(Object)}
*/
@Deprecated
public static XdevFile parseFile(Object value)
{
if(value == null)
{
return null;
}
XdevFile file = null;
if(value instanceof XdevFile)
{
file = (XdevFile)value;
}
else if(value instanceof File)
{
file = new XdevFile((File)value);
}
else if(value instanceof URI)
{
file = new XdevFile((URI)value);
}
else if(value instanceof URL)
{
try
{
file = new XdevFile(((URL)value).toURI());
}
catch(URISyntaxException e)
{
throw new RuntimeException(e);
}
}
return file;
}
/**
* Ckecks whether Object
value
is a
* XdevImage
. If yes it returns a XdevImage
else
* null
.
*
* @param value
* Object
which will be parsed to
* XdevImage
.
* @return XdevImage
if value
is a
* XdevImage
. Else null
.
*
* @deprecated use {@link ConversionUtils#toXdevImage(Object)}
*/
@Deprecated
public static XdevImage parseImage(Object value)
{
if(value == null)
{
return null;
}
XdevImage image = null;
if(value instanceof XdevImage)
{
image = (XdevImage)value;
}
else if(value instanceof Image)
{
image = new XdevImage((Image)value);
}
else if(value instanceof Icon)
{
image = new XdevImage(GraphicUtils.createImageFromIcon((Icon)value));
}
return image;
}
/**
* Parse the variable i
to HexString
. * Examples * ** * @param i ** ParseUtils.toHexString(25); returns 19 * ParseUtils.toHexString(-62); returns ffffffc2 ** *
int
which will be parsed to
* HexString
* @return String HexSring parsed from i
*
* @see Integer#toHexString(int)
*/
public static String toHexString(int i)
{
return Integer.toHexString(i);
}
/**
* Parse the variable l
to HexString
.
*
* Examples * ** * @param l ** ParseUtils.toHexString(-25897); returns ffff9ad7 * ParseUtils.toHexString(2589); returns a1d * ** *
long
which will be parsed to
* HexString
* @return String HexSring parsed from l
*
* @see Long#toHexString(long)
*/
public static String toHexString(long l)
{
return Long.toHexString(l);
}
/**
* Parse the variable i
to OctalString
.
*
* Examples * ** * @param i ** ParseUtils.toOctalString(597632); returns 2217200 * ParseUtils.toOctalString(-1025897); returns 37774054227 * ** *
int
which will be parsed to
* OctalString
* @return String OctalString parsed from i
*
* @see Integer#toOctalString(int)
*/
public static String toOctalString(int i)
{
return Integer.toOctalString(i);
}
/**
* Parse the variable l
to OctalString
.
*
* Examples * ** * @param l ** ParseUtils.toOctalString(1025856889); returns 7511252571 * ParseUtils.toOctalString(-589789567); returns 33466103201 * ** *
long
which will be parsed to
* OctalString
* @return String OctalString parsed from l
*
* @see Long#toOctalString(long)
*/
public static String toOctalString(long l)
{
return Long.toOctalString(l);
}
/**
* Parse the variable i
to BinaryString
.
*
*
* Examples * ** * @param i ** ParseUtils.toBinaryString(-589789567); returns 11011100110110001000011010000001 * ParseUtils.toBinaryString(897898967); returns 110101100001001101100111010111 * ** *
int
which will be parsed to
* BinaryString
* @return String BinaryString parsed from i
*
* @see Integer#toBinaryString(int)
*/
public static String toBinaryString(int i)
{
return Integer.toBinaryString(i);
}
/**
* Parse the variable l
to BinaryString
.
*
*
* Examples * ** * @param l ** ParseUtils.toBinaryString(897295967); returns 110101011110111010011001011111 * ParseUtils.toBinaryString(-831878067); returns 11001110011010101000110001001101 * ** *
long
which will be parsed to
* BinaryString
* @return String BinaryString parsed from l
*
* @see Long#toBinaryString(long)
*/
public static String toBinaryString(long l)
{
return Long.toBinaryString(l);
}
/**
* Checks if the variable value
is a value between
* min
and max
. With the condition:
* min
<= value
<= max
.
*
* Examples * ** * @param value * the value which will be checked * @param min * the(inclusive)lower border for* ParseUtils.isNumeric(489999995, 12000, 698777); returns false * ParseUtils.isNumeric(5000, 5000, 5000); returns true * ** *
value
* @param max
* the(inclusive)upper border for value
* @return true if min
<= value
<=
* max
*
* @see ParseUtils#isAlphaNumeric(String, int, int)
* @see ParseUtils#isNumeric(Object, double, double)
*/
public static boolean isNumeric(Object value, long min, long max)
{
try
{
long l = parseLong(value);
return l >= min && l <= max;
}
catch(Exception e)
{
return false;
}
}
/**
* * Checks if the variable value
is a value between
* min
and max
. With the condition:
* min
<= value
<= max
.
*
* Examples * ** * @param value * the value which will be checked * @param min * the(inclusive)lower border for* ParseUtils.isNumeric(5600.80, 500.20, 4000.90); returns false * ParseUtils.isNumeric(-5000.90, -489.25, -800.65); returns false * ParseUtils.isNumeric(-5000.90, -5000.90, -5000.90); returns true ** *
value
* @param max
* the(inclusive)upper border for value
* @return true if min
<= value
<=
* max
*
* @see ParseUtils#isAlphaNumeric(String, int, int)
* @see ParseUtils#isNumeric(Object, long, long)
*/
public static boolean isNumeric(Object value, double min, double max)
{
try
{
double d = parseDouble(value);
return d >= min && d <= max;
}
catch(Exception e)
{
return false;
}
}
/**
* Checks if the variable String
is not a number and is it has
* a length between minLen
and maxLen
.
*
* If this applies it returns true
, else false
.
*
* Example * ** * @param value * the* ParseUtils.isAlphaNumeric("Hello10", 2, 30); returns true * ParseUtils.isAlphaNumeric("Hello-15", -40, 30); returns true * ParseUtils.isAlphaNumeric("Hello XDEV3", -40, -15); returns false ** *
String
which length will be checked
* @param min
* the(inclusive)minimum length for value
* @param max
* the(inclusive)maximum length for value
* @return true if minLen
<= value.length
<=
* maxLen
*
* @see ParseUtils#isNumeric(Object, double, double)
* @see ParseUtils#isNumeric(Object, long, long)
*/
public static boolean isAlphaNumeric(String value, int min, int max)
{
try
{
Double.valueOf(value);
}
catch(NumberFormatException e)
{
int anl = value.length();
if(anl >= min && anl <= max)
{
return true;
}
}
return false;
}
private final static String EMAIL_REGEX = "^[a-zA-Z0-9._%-]+@[a-zA-ZZÄÖÜäöü0-9._%-]+\\.[a-zA-Z]{2,4}$";
/**
* Checks if the variable String
is a valid Email address.
*
* Example * *Hint * ** ParseUtils.isEmail("[email protected]"); returns true * ParseUtils.isEmail(" "); returns false ** *
* It can only be checked whether the String has the right format for an email address, not whether this email address truely exists. ** * * @param value * the
String
which format will be checked
* @return true if the String has a valid email address format.
*
* @see ParseUtils#isNumeric(Object, double, double)
* @see ParseUtils#isNumeric(Object, long, long)
*/
public static boolean isEmail(String value)
{
return Pattern.compile(EMAIL_REGEX).matcher(value).matches();
}
/**
* Formats the long
l
with the actual language
* setting and returns it as String
.
*
* Example * ** * * ** ParseUtils.format(894302256); returns 894.302.256 * ParseUtils.format(-789602314); returns -789.602.314 ** *
* The german standard setting for decimals is:
*
- Thousand delimiter "."
- decimal point ","
- round to 3 decimal places
*
*
long
which will be formated.
* @return long
in format of the actual language setting.
*
* @see ParseUtils#format(double)
* @see ParseUtils#format(long, String)
*/
public static String format(long l)
{
return getNumberFormat().format(l);
}
/**
* Formates the number l
to a String
with the help
* of the format
.
*
* Example * ** * Hint * ** ParseUtils.format(789302125, "Lange Zahl "); returns Lange Zahl 789302125 * ParseUtils.format(-569780212, "Negative Zahl "); returns -Negative Zahl 569780212 ** *
* for valid patterns see {@link ParseUtils#parse(Object, String)} ** * * @param l * the
long
which will be formated.
* @param pattern
* the String
with which l
will be
* formated.
* @return l
formated with pattern pattern
.
*
* @see ParseUtils#format(long)
* @see ParseUtils#format(double, String)
*/
public static String format(long l, String pattern)
{
pattern = pattern.replace('\\','\u2030');
pattern = pattern.replace('_','\u00A4');
return new DecimalFormat(pattern).format(l);
}
/**
* Formats the double
d
with the actual language
* setting and returns it as String
.
*
* Example * ** * * ** * ParseUtils.format(2000.3505); returns 2.000,35 * ParseUtils.format(-23205.3589705); returns -23.205,359 * ** *
* The german standard setting for decimals is:
*
- Thousand delimiter "."
- decimal point ","
- round to 3 decimal places
*
*
double
which will be formated.
* @return double
in format of the actual language setting.
*
* @see ParseUtils#format(long)
* @see ParseUtils#format(double, String)
*/
public static String format(double d)
{
return getNumberFormat().format(d);
}
/**
* Formates the number l
to a String
with the help
* of the format
.
*
* Example
*
* * ParseUtils.format(-7892.0365478, "Euro"); returns -Euro7892 * ParseUtils.format(25897.6321, "hello"); returns hello25898 * ** * * @param d * the
long
which will be formated.
* @param pattern
* the String
with which l
will be
* formated.
* @return l
formated with pattern pattern
.
*
* @see ParseUtils#format(long, String)
* @see ParseUtils#format(double)
*/
public static String format(double d, String pattern)
{
pattern = pattern.replace('\\','\u2030');
pattern = pattern.replace('_','\u00A4');
return new DecimalFormat(pattern).format(d);
}
/**
* Formates the long
l
as currency with the actual
* language setting and returns it as String
.
*
* Example * *Hint * ** * ParseUtils.formatAsCurrency(897565258); returns 897.565.258,00 € * ParseUtils.formatAsCurrency(-45752378); returns -45.752.378,00 € * ** *
* The german default setting for currency is:
*
- Thousand delimiter "."
- decimal point ","
- round to 2 decimal places
- closing "€"-sign with preceding space
*
*
*
long
which will be formated as currency
* @return the long
formated as currency with the actual
* language setting.
*
* @see ParseUtils#format(double)
* @see ParseUtils#format(long, String)
* @see ParseUtils#parseDouble(Object)
* @see ParseUtils#parseDouble(Object, String)
*/
public static String formatAsCurrency(long l)
{
return NumberFormat.getCurrencyInstance().format(l);
}
/**
* Formates the double
d
as currency with the
* actual language setting and returns it as String
.
*
* Example * *Hint * ** ParseUtils.formatAsCurrency(78963.256); returns 78.963,26 € * ParseUtils.formatAsCurrency(-458.256); returns -458,26 € * ** *
* The german default setting for currency is:
*
- Thousand delimiter "."
- decimal point ","
- round to 2 decimal places
- closing "€"-sign with preceding space
*
*
*
double
which will be formated as currency
* @return the double
formated as currency with the actual
* language setting.
*
* @see ParseUtils#format(double)
* @see ParseUtils#format(long, String)
* @see ParseUtils#parseDouble(Object)
* @see ParseUtils#parseDouble(Object, String)
*/
public static String formatAsCurrency(double d)
{
return NumberFormat.getCurrencyInstance().format(d);
}
/**
* Checks whether Object
value
is a type of
* Class
type
. If yes it returns
* value
else ClassCastException
.
*
* @param value
* Object
which type will be checked
* @param type
* Class
of which Object
will be
* checked.
* @return value
if value
is type of
* type
. Else ClassCastException
.
*
* @see ParseUtils#isType(Object, String)
* @see ParseUtils#isType(Object, Class)
*
*/
public static Object castTo(Object value, Class type)
{
if(isType(value,type))
{
return value;
}
throw new ClassCastException(value + " is not a " + type);
}
/**
* Checks whether Object
value
is a type of
* Class
type
. If yes it returns true
* else false
.
*
* @param value
* Object
which type will be checked
* @param type
* Class
of which Object
will be
* checked.
* @return true if Object
is type of type
. Else
* false
.
*
* @see ParseUtils#isType(Object, String)
*/
public static boolean isType(Object value, Class type)
{
return type.isAssignableFrom(value.getClass());
}
/**
* Checks whether Object
value
is a type of
* Class
className
. If yes it returns
* true
else false
.
*
* Example* *
* ParseUtils.isType(65, "double"); returns false * ** * @param value *
Object
which type will be checked
* @param className
* className
of which Object
will be
* checked.
* @return true if Object
is type of className
.
* Else false
.
*
* @see ParseUtils#isType(Object, Class)
*/
public static boolean isType(Object value, String className)
{
try
{
return Class.forName(className).isAssignableFrom(value.getClass());
}
catch(Exception e)
{
return false;
}
}
/**
* Checks if the Object
value
is an integer. That
* means whether value
can be parsed to an integer without
* losing information.
*
* Example * ** ** * ParseUtils.isInteger(20); returns true * ParseUtils.isInteger(null); returns false * ** *
* The number range of Integer
is between -2.147.483.648 and 2.147.483.647 .
*
*
* @param value
* the Object
value
which will be
* checked
* @return true if value
is an integer or can be parsed to
* without losing information
*
* @see ParseUtils#isInteger(Object)
* @see ParseUtils#isDecimal(Object)
* @see ParseUtils#isBoolean(Object)
* @see ParseUtils#parseLong(Object)
* @see ParseUtils#parseLong(Object, String)
*/
public static boolean isInteger(Object value)
{
try
{
double d = parseDouble(value);
int i = (int)d;
return i == d && i >= Integer.MIN_VALUE && i <= Integer.MAX_VALUE;
}
catch(Exception e)
{
return false;
}
}
/**
* Checks if the Object
value
is an integer. That
* means whether value
can be parsed to an integer without
* losing information.
*
* Example * * ParseUtils.isLong(20875884855699l); returns true ParseUtils.isLong(null); * returns false * ** *
* The number range of long
is between -9.223.372.036.854.775.808 and 9.223.372.036.854.775.807 .
*
*
* @param value
* the Object
value
which will be
* checked
* @return true if value
is an integer or can be parsed to
* without losing information
*
* @see ParseUtils#isLong(Object)
* @see ParseUtils#isDecimal(Object)
* @see ParseUtils#isBoolean(Object)
* @see ParseUtils#parseLong(Object)
* @see ParseUtils#parseLong(Object, String)
*/
public static boolean isLong(Object value)
{
try
{
double d = parseDouble(value);
long l = (long)d;
return l == d;
}
catch(Exception e)
{
return false;
}
}
/**
* Checks if the Object
value
is a decimal or can
* parsed to.
*
* 1. General * *Hint * ** isDecimal("3.14") * * returns* * 2. Integer * *true
** isDecimal("5") * * returns* * 3. Zero * *true
. ** isDecimal("0") * * returns* * 4. Exponent spelling 1 * *true
. ** isDecimal("1E3") * * * returns* * 5. Exponent spelling 2 * *true
. * (1E3 = 1 * 103 = 1000) ** isDecimal(1E-3) * * returns* * 6. Not a number * *true
. * (1E3 = 1 * 10-3 = 0,001) ** isDecimal("NaN") * * returns* * 7. Minus infinity * *true
. ** isDecimal("Infinity") * * returns* * 8. Minus infinity * *true
. ** isDecimal("-Infinity") * * returns* *true
. *
* The check wil be made with the regular expression: * ^[a-zA-Z0-9._%-]+@[a-zA-ZÄÖÜäöü0-9._%-]+\\.[a-zA-Z]{2,4}$ ** * Hint * *
* "NaN", "Infinity" and "-Infinity" are case sensitive. * That means "NAN", "nan", "infinity", "INFINITY", etc can't be recognized. * Also "Negative Infinity" or something like that can't be recognized. * * For the exponent notation can instead of "E" also "e" be used. * Likewise, "5E09", "0E4" or "6.5E12" can be used too. Is not possible to use e.g. "E3" or "5E9.1" * ** * Hint * *
* This methode has approximately the same performance as {@link ParseUtils#parseDouble(Object)}. * That means if* * * @param value * theObject
value
will be used as decimal, after this methode, it is convenient * to write: *
*
*
* ParseUtils.isDecimal(200.05); returns true * ParseUtils.isDecimal(null); returns false *
*
* * With this the check will be made only once. * *
Object
value
which will be
* checked
* @return true if value
is a decimal or can be parsed to.
*
* @see ParseUtils#isInteger(Object)
* @see ParseUtils#isNumeric(Object, double, double)
* @see ParseUtils#parseDouble(Object)
*/
public static boolean isDecimal(Object value)
{
try
{
parseDouble(value);
return true;
}
catch(Exception e)
{
return false;
}
}
/**
* Checks if the variable value
is type of boolean
* .* ** * * ** simple * ParseUtils.isBoolean(* *true
) * ParseUtils.isBoolean(false
) * * Returnstrue
. * * other Value * isBoolean("true") * isBoolean(5) * isBoolean("alles andere") * * Returnsfalse
. * (also "true" isnt a Boolean, because the type is a {@link String}) *
* Warning: While {@link ParseUtils#parseBoolean(Object)}
* trys to parse a given value to boolean
, checks this methode
* not if a given value can be parsed to boolean
, but if a
* given value is type of boolean
.
*
* To check if a value (like the String
"true") can be
* interprete as boolean
can be used the following Code:
*
* ParseUtils.isBoolean(null); returns false
* ParseUtils.isBoolean(150); returns false
*
boolean
.
* @return true
if the value
is a
* boolean
, else false
*
* @see ParseUtils#parseBoolean(Object)
* @see ParseUtils#isInteger(Object)
* @see ParseUtils#isDecimal(Object)
* @see ParseUtils#isLong(Object)
*
*/
public static boolean isBoolean(Object value)
{
return value != null && value instanceof Boolean;
}
private static Hashtable numberFormatCache = new Hashtable();
private static NumberFormat getNumberFormat()
{
Locale locale = Locale.getDefault();
if(numberFormatCache.containsKey(locale))
{
return (NumberFormat)numberFormatCache.get(locale);
}
NumberFormat format = NumberFormat.getNumberInstance(locale);
numberFormatCache.put(locale,format);
return format;
}
}