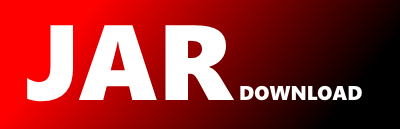
ai.digital.integration.server.tasks.CheckUILibVersionsTask.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of integratoin-server-gradle-plugin Show documentation
Show all versions of integratoin-server-gradle-plugin Show documentation
The easy way to get custom setup for Deploy up and running
The newest version!
package ai.digital.integration.server.tasks
import ai.digital.integration.server.util.LocationUtil
import de.vandermeer.asciitable.AsciiTable
import de.vandermeer.skb.interfaces.transformers.textformat.TextAlignment
import groovy.json.JsonSlurper
import org.apache.commons.io.IOUtils
import org.gradle.api.DefaultTask
import org.gradle.api.GradleException
import org.gradle.api.Project
import org.gradle.api.tasks.TaskAction
import java.nio.charset.Charset
import java.nio.file.Paths
import java.util.zip.ZipEntry
import java.util.zip.ZipFile
import java.util.zip.ZipInputStream
import static ai.digital.integration.server.constant.PluginConstant.PLUGIN_GROUP
class CheckUILibVersionsTask extends DefaultTask {
static NAME = "checkUILibVersions"
CheckUILibVersionsTask() {
this.configure {
group = PLUGIN_GROUP
}
}
private static def parseVersions(ZipInputStream stream) {
def jsonSlurper = new JsonSlurper()
jsonSlurper.parse(IOUtils.toByteArray(stream))
}
private static def parsePluginName(ZipInputStream stream) {
def prefix = "plugin="
IOUtils
.toString(stream, Charset.defaultCharset())
.split("\n")
.find { it.startsWith(prefix) }
.substring(prefix.length())
}
private static def extractPluginMetadata(ZipFile xldpZip, ZipEntry internalJarEntry) {
def stream = new ZipInputStream(xldpZip.getInputStream(internalJarEntry))
def entry = stream.nextEntry
def pluginName
def versions
while (entry != null && (pluginName == null || versions == null)) {
if (entry.name.endsWith("-metadata.json")) {
versions = parseVersions(stream)
}
if (entry.name.equals("plugin-version.properties")) {
pluginName = parsePluginName(stream)
}
entry = stream.nextEntry
}
try {
pluginName != null && versions != null ? [[plugin: pluginName, versions: versions]] : []
} finally {
stream.close()
}
}
private static def checkForMismatch(String libName, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy