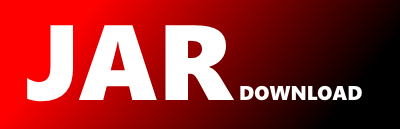
com.xebialabs.overthere.ConnectionOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of overthere Show documentation
Show all versions of overthere Show documentation
Remote file manipulation and process execution framework for Java
/**
* Copyright (c) 2008-2016, XebiaLabs B.V., All rights reserved.
*
*
* Overthere is licensed under the terms of the GPLv2
* , like most XebiaLabs Libraries.
* There are special exceptions to the terms and conditions of the GPLv2 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* This program is free software; you can redistribute it and/or modify it under the terms
* of the GNU General Public License as published by the Free Software Foundation; version 2
* of the License.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with this
* program; if not, write to the Free Software Foundation, Inc., 51 Franklin St, Fifth
* Floor, Boston, MA 02110-1301 USA
*/
package com.xebialabs.overthere;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
/**
* Represents options to use when creating a {@link OverthereConnection connection}.
*/
public class ConnectionOptions {
private static final Set filteredKeys = new HashSet<>();
public static String registerFilteredKey(String key) {
filteredKeys.add(key);
return key;
}
/**
* See the online documentation
*/
public static final String PROTOCOL = "protocol";
/**
* See the online documentation
*/
public static final String OPERATING_SYSTEM = "os";
/**
* See the online documentation
*/
public static final String TEMPORARY_DIRECTORY_PATH = "tmp";
/**
* See the online documentation
*/
public static final String TEMPORARY_DIRECTORY_DELETE_ON_DISCONNECT = "tmpDeleteOnDisconnect";
/**
* See the online documentation
*/
public static final boolean TEMPORARY_DIRECTORY_DELETE_ON_DISCONNECT_DEFAULT = true;
/**
* See the online documentation
*/
public static final String TEMPORARY_FILE_CREATION_RETRIES = "tmpFileCreationRetries";
/**
* See the online documentation
*/
public static final int TEMPORARY_FILE_CREATION_RETRIES_DEFAULT = 100;
/**
* See the online documentation
*/
public static final String CONNECTION_TIMEOUT_MILLIS = "connectionTimeoutMillis";
/**
* See the online documentation
*/
public static final int CONNECTION_TIMEOUT_MILLIS_DEFAULT = 120000;
/**
* See the online documentation
*/
public static final String SOCKET_TIMEOUT_MILLIS = "socketTimeoutMillis";
/**
* See the online documentation
*/
public static final int SOCKET_TIMEOUT_MILLIS_DEFAULT = 0;
/**
* See the online documentation
*/
public static final String ADDRESS = "address";
/**
* See the online documentation
*/
public static final String PORT = "port";
/**
* See the online documentation
*/
public static final String USERNAME = "username";
/**
* See the online documentation
*/
public static final String PASSWORD = registerFilteredKey("password");
/**
* See the online documentation
*/
public static final String JUMPSTATION = "jumpstation";
/**
* See the online documentation
*/
public static final String FILE_COPY_COMMAND_FOR_UNIX = "fileCopyCommandForUnix";
/**
* See the online documentation
*/
public static final String FILE_COPY_COMMAND_FOR_UNIX_DEFAULT = "cp -p {0} {1}";
/**
* See the online documentation
*/
public static final String DIRECTORY_COPY_COMMAND_FOR_UNIX = "directoryCopyCommandForUnix";
/**
* See the online documentation
*/
public static final String DIRECTORY_COPY_COMMAND_FOR_UNIX_DEFAULT = "cd {1} ; tar -cf - -C {0} . | tar xpf -";
/**
* See the online documentation
*/
public static final String FILE_COPY_COMMAND_FOR_WINDOWS = "fileCopyCommandForWindows";
/**
* See the online documentation
*/
public static final String FILE_COPY_COMMAND_FOR_WINDOWS_DEFAULT = "copy {0} {1} /y";
/**
* See the online documentation
*/
public static final String DIRECTORY_COPY_COMMAND_FOR_WINDOWS = "directoryCopyCommandForWindows";
/**
* See the online documentation
*/
public static final String DIRECTORY_COPY_COMMAND_FOR_WINDOWS_DEFAULT = "xcopy {0} {1} /i /y /s /e /h /q";
/**
* See the online documentation
*/
public static final String FILE_COPY_COMMAND_FOR_ZOS = "fileCopyCommandForZos";
/**
* See the online documentation
*/
public static final String FILE_COPY_COMMAND_FOR_ZOS_DEFAULT = "cp -p {0} {1}";
/**
* See the online documentation
*/
public static final String DIRECTORY_COPY_COMMAND_FOR_ZOS = "directoryCopyCommandForZos";
/**
* See the online documentation
*/
public static final String DIRECTORY_COPY_COMMAND_FOR_ZOS_DEFAULT = "tar cC {0} . | tar xmC {1}";
/**
* See the online documentation
*/
public static final String REMOTE_COPY_BUFFER_SIZE = "remoteCopyBufferSize";
/**
* See the online documentation
*/
public static final int REMOTE_COPY_BUFFER_SIZE_DEFAULT = 64 * 1024; // 64 KB
/**
* See the online documentation
*/
public static final String REMOTE_CHARACTER_ENCODING = "remoteCharacterEncoding";
private final Map options;
/**
* Creates an empty options object.
*/
public ConnectionOptions() {
options = new HashMap();
}
/**
* Creates a copy of an existing options object.
*/
public ConnectionOptions(ConnectionOptions options) {
this();
this.options.putAll(options.options);
}
/**
* Sets a connection option.
*
* @param key the key of the connection option.
* @param value the value of the connection option.
*/
public void set(String key, Object value) {
options.put(key, value);
}
/**
* Retrieves the value of a required connection option.
*
* @param the type of the connection option.
* @param key the key of the connection option.
* @return the value of the connection option.
* @throws IllegalArgumentException if no value was supplied for the connection option
*/
@SuppressWarnings("unchecked")
public T get(String key) throws IllegalArgumentException {
T value = (T) options.get(key);
if (value == null) {
throw new IllegalArgumentException("No value specified for required connection option " + key);
}
return value;
}
/**
* Retrieves the value of an optional connection option.
*
* @param the type of the connection option.
* @param key the key of the connection option.
* @return the value of the connection option or null
if that option was not specified.
*/
@SuppressWarnings("unchecked")
public T getOptional(String key) {
return (T) options.get(key);
}
/**
* Retrieves the value of a connection option or a default value if that option has not been set.
*
* @param the type of the connection option.
* @param key the key of the connection option.
* @param defaultValue the default value to use of the connection options has not been set.
* @return the value of the connection option or the default value if that option was not specified.
*/
@SuppressWarnings("unchecked")
public T get(String key, T defaultValue) {
if (options.containsKey(key)) {
return (T) options.get(key);
} else {
return defaultValue;
}
}
public boolean getBoolean(String key) {
Object o = options.get(key);
if (o == null) {
throw new IllegalArgumentException("No value specified for required connection option " + key);
} else if (o instanceof Boolean) {
return (Boolean) o;
} else if (o instanceof String) {
return Boolean.valueOf((String) o);
} else {
throw new IllegalArgumentException("Value specified for required connection option " + key + " is neither a Boolean nor a String");
}
}
public boolean getBoolean(String key, boolean defaultValue) {
Object o = options.get(key);
if (o == null) {
return defaultValue;
} else if (o instanceof Boolean) {
return (Boolean) o;
} else if (o instanceof String) {
return Boolean.valueOf((String) o);
} else {
throw new IllegalArgumentException("Value specified for connection option " + key + " is neither a Boolean nor a String");
}
}
public int getInteger(String key) {
Object o = options.get(key);
if (o == null) {
throw new IllegalArgumentException("No value specified for required connection option " + key);
} else if (o instanceof Integer) {
return (Integer) o;
} else if (o instanceof String) {
return Integer.parseInt((String) o);
} else {
throw new IllegalArgumentException("Value specified for required connection option " + key + " is neither an Integer nor a String");
}
}
public int getInteger(String key, int defaultValue) {
Object o = options.get(key);
if (o == null) {
return defaultValue;
} else if (o instanceof Integer) {
return (Integer) o;
} else if (o instanceof String) {
return Integer.parseInt((String) o);
} else {
throw new IllegalArgumentException("Value specified for connection option " + key + " is neither an Integer nor a String");
}
}
@SuppressWarnings("unchecked")
public > T getEnum(String key, Class enumClazz) {
T o = getEnum(key, enumClazz, null);
if (o == null) {
throw new IllegalArgumentException("No value specified for required connection option " + key);
} else {
return o;
}
}
@SuppressWarnings("unchecked")
public > T getOptionalEnum(String key, Class enumClazz) {
return getEnum(key, enumClazz, null);
}
@SuppressWarnings("unchecked")
public > T getEnum(String key, Class enumClazz, T defaultValue) {
Object o = options.get(key);
if (o == null) {
return defaultValue;
} else if (o.getClass().equals(enumClazz)) {
return (T) o;
} else if (o instanceof String) {
return Enum.valueOf(enumClazz, (String) o);
} else {
throw new IllegalArgumentException("Value specified for connection option " + key + " is neither an instanceof of " + enumClazz.getName()
+ " nor a String");
}
}
/**
* Returns whether a connection option is set.
*
* @param key the key of the connection option.
* @return true iff the connection option is set, false otherwise.
*/
public boolean containsKey(String key) {
return options.containsKey(key);
}
/**
* Returns the keys of all connection options set.
*
* @return a {@link Set} containing the keys.
*/
public Set keys() {
return options.keySet();
}
@Override
public boolean equals(Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
ConnectionOptions that = (ConnectionOptions) o;
return options.equals(that.options);
}
@Override
public int hashCode() {
return options.hashCode();
}
@Override
public String toString() {
return print(this, "");
}
private static String print(ConnectionOptions options, String indent) {
StringBuilder b = new StringBuilder();
b.append("ConnectionOptions[\n");
for (Map.Entry e : options.options.entrySet()) {
b.append(indent).append("\t").append(e.getKey()).append(" --> ");
Object value = e.getValue();
if (value instanceof ConnectionOptions) {
b.append(print((ConnectionOptions) value, indent + "\t"));
} else {
b.append(filteredKeys.contains(e.getKey()) ? "********" : value);
}
b.append("\n");
}
b.append(indent).append("]");
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy