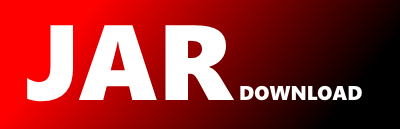
com.xeiam.xchange.examples.bitstamp.account.BitstampAccountDemo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xchange-examples Show documentation
Show all versions of xchange-examples Show documentation
Provides a set of examples that demonstrate how to use XChange in client applications
The newest version!
package com.xeiam.xchange.examples.bitstamp.account;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.List;
import com.xeiam.xchange.Exchange;
import com.xeiam.xchange.bitstamp.dto.account.BitstampBalance;
import com.xeiam.xchange.bitstamp.dto.account.BitstampDepositAddress;
import com.xeiam.xchange.bitstamp.dto.account.BitstampWithdrawal;
import com.xeiam.xchange.bitstamp.dto.account.DepositTransaction;
import com.xeiam.xchange.bitstamp.dto.account.WithdrawalRequest;
import com.xeiam.xchange.bitstamp.service.polling.BitstampAccountServiceRaw;
import com.xeiam.xchange.currency.Currencies;
import com.xeiam.xchange.dto.account.AccountInfo;
import com.xeiam.xchange.examples.bitstamp.BitstampDemoUtils;
import com.xeiam.xchange.service.polling.account.PollingAccountService;
/**
*
* Example showing the following:
*
*
* - Connect to Bitstamp exchange with authentication
* - View account balance
* - Get the bitcoin deposit address
* - List unconfirmed deposits (raw interface only)
* - List recent withdrawals (raw interface only)
* - Withdraw a small amount of BTC
*
*/
public class BitstampAccountDemo {
public static void main(String[] args) throws IOException {
Exchange bitstamp = BitstampDemoUtils.createExchange();
PollingAccountService accountService = bitstamp.getPollingAccountService();
generic(accountService);
raw((BitstampAccountServiceRaw) accountService);
}
private static void generic(PollingAccountService accountService) throws IOException {
// Get the account information
AccountInfo accountInfo = accountService.getAccountInfo();
System.out.println("AccountInfo as String: " + accountInfo.toString());
String depositAddress = accountService.requestDepositAddress(Currencies.BTC);
System.out.println("Deposit address: " + depositAddress);
String withdrawResult = accountService.withdrawFunds("BTC", new BigDecimal(1).movePointLeft(4), "1PxYUsgKdw75sdLmM7HYP2p74LEq3mxM6L");
System.out.println("withdrawResult = " + withdrawResult);
}
private static void raw(BitstampAccountServiceRaw accountService) throws IOException {
// Get the account information
BitstampBalance bitstampBalance = accountService.getBitstampBalance();
System.out.println("BitstampBalance: " + bitstampBalance);
BitstampDepositAddress depositAddress = accountService.getBitstampBitcoinDepositAddress();
System.out.println("BitstampDepositAddress address: " + depositAddress);
final List unconfirmedDeposits = accountService.getUnconfirmedDeposits();
System.out.println("Unconfirmed deposits:");
for (DepositTransaction unconfirmedDeposit : unconfirmedDeposits) {
System.out.println(unconfirmedDeposit);
}
final List withdrawalRequests = accountService.getWithdrawalRequests();
System.out.println("Withdrawal requests:");
for (WithdrawalRequest unconfirmedDeposit : withdrawalRequests) {
System.out.println(unconfirmedDeposit);
}
BitstampWithdrawal withdrawResult = accountService.withdrawBitstampFunds(new BigDecimal(1).movePointLeft(4),
"1PxYUsgKdw75sdLmM7HYP2p74LEq3mxM6L");
System.out.println("BitstampBooleanResponse = " + withdrawResult);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy