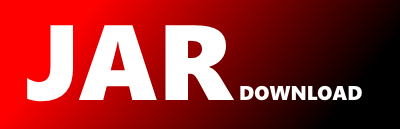
com.xeiam.xchange.kraken.service.KrakenDigest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xchange-kraken Show documentation
Show all versions of xchange-kraken Show documentation
XChange implementation for the Kraken Exchange
package com.xeiam.xchange.kraken.service;
import java.io.IOException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import javax.crypto.Mac;
import javax.ws.rs.FormParam;
import si.mazi.rescu.RestInvocation;
import si.mazi.rescu.utils.Base64;
import com.xeiam.xchange.service.BaseParamsDigest;
/**
* @author Benedikt Bünz
*/
public class KrakenDigest extends BaseParamsDigest {
/**
* Constructor
*
* @param secretKeyBase64
* @throws IllegalArgumentException if key is invalid (cannot be base-64-decoded or the decoded key is invalid).
*/
private KrakenDigest(byte[] secretKeyBase64) {
super(secretKeyBase64, HMAC_SHA_512);
}
public static KrakenDigest createInstance(String secretKeyBase64) {
try {
if (secretKeyBase64 != null)
return new KrakenDigest(Base64.decode(secretKeyBase64.getBytes()));
} catch (IOException e) {
throw new IllegalArgumentException("Could not decode Base 64 string", e);
}
return null;
}
@Override
public String digestParams(RestInvocation restInvocation) {
MessageDigest sha256;
try {
sha256 = MessageDigest.getInstance("SHA-256");
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException("Illegal algorithm for post body digest. Check the implementation.");
}
sha256.update(restInvocation.getParamValue(FormParam.class, "nonce").toString().getBytes());
sha256.update(restInvocation.getRequestBody().getBytes());
Mac mac512 = getMac();
mac512.update(("/" + restInvocation.getPath()).getBytes());
mac512.update(sha256.digest());
return Base64.encodeBytes(mac512.doFinal()).trim();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy