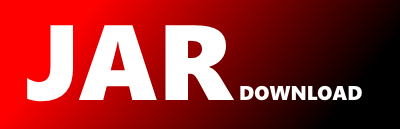
org.quartz.builders.JobBuilder Maven / Gradle / Ivy
/**
* All content copyright Terracotta, Inc., unless otherwise indicated. All rights reserved.
* Copyright 2011-2015 Xeiam, LLC
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*
*/
package org.quartz.builders;
import java.util.UUID;
import org.quartz.jobs.Job;
import org.quartz.jobs.JobDataMap;
import org.quartz.jobs.JobDetail;
import org.quartz.jobs.JobDetailImpl;
import org.quartz.jobs.NoOpJob;
/**
* JobBuilder
is used to instantiate {@link JobDetail}s.
*
* Quartz provides a builder-style API for constructing scheduling-related entities via a Domain-Specific Language (DSL). The DSL can best be utilized
* through the usage of static imports of the methods on the classes TriggerBuilder
, JobBuilder
, DateBuilder
,
* JobKey
, TriggerKey
and the various ScheduleBuilder
implementations.
*
*
* Client code can then use the DSL to write code such as this:
*
*
*
*
*
*
* JobDetail job = newJob(MyJob.class).withIdentity("myJob").build();
*
* Trigger trigger = newTrigger().withIdentity(triggerKey("myTrigger", "myTriggerGroup"))
* .withSchedule(simpleSchedule().withIntervalInHours(1).repeatForever()).startAt(futureDate(10, MINUTES)).build();
*
* scheduler.scheduleJob(job, trigger);
*
*
*
* @author timmolter
*/
public class JobBuilder {
private String key;
private String description;
private Class extends Job> jobClass = NoOpJob.class;
private boolean durability = true;
private boolean isConcurrencyAllowed = false;
private JobDataMap jobDataMap = new JobDataMap();
private JobBuilder() {
}
/**
* Create a JobBuilder with which to define a JobDetail
.
*
* @return a new JobBuilder
*/
public static JobBuilder newJobBuilder() {
return new JobBuilder();
}
/**
* Create a JobBuilder with which to define a JobDetail
, and set the class name of the Job
to be executed.
*
* @return a new JobBuilder
*/
public static JobBuilder newJobBuilder(Class extends Job> jobClass) {
JobBuilder b = new JobBuilder();
b.ofType(jobClass);
return b;
}
/**
* Produce the JobDetail
instance defined by this JobBuilder
.
*
* @return the defined JobDetail.
*/
public JobDetail build() {
JobDetailImpl job = new JobDetailImpl();
job.setJobClass(jobClass);
job.setDescription(description);
if (key == null) {
key = UUID.randomUUID().toString();
}
job.setName(key);
job.setIsConcurrencyAllowed(isConcurrencyAllowed);
if (!jobDataMap.isEmpty()) {
job.setJobDataMap(jobDataMap);
}
return job;
}
/**
* Use a String
to identify the JobDetail.
*
* If none of the 'withIdentity' methods are set on the JobBuilder, then a random, unique JobKey will be generated.
*
*
* @param key the Job's JobKey
* @return the updated JobBuilder
*/
public JobBuilder withIdentity(String key) {
this.key = key;
return this;
}
/**
* Set the given (human-meaningful) description of the Job.
*
* @param description the description for the Job
* @return the updated JobBuilder
* @see JobDetail#getDescription()
*/
public JobBuilder withDescription(String description) {
this.description = description;
return this;
}
/**
* Set the class which will be instantiated and executed when a Trigger fires that is associated with this JobDetail.
*
* @param jobClass a class implementing the Job interface.
* @return the updated JobBuilder
* @see JobDetail#getJobClass()
*/
public JobBuilder ofType(Class extends Job> jobClass) {
this.jobClass = jobClass;
return this;
}
/**
* The default behavior is to veto any job is currently running concurrent. However, concurrent jobs can be created by setting the 'Concurrency' to
* true
*
* @param isConcurrencyAllowed
* @return the updated JobBuilder
*/
public JobBuilder isConcurrencyAllowed(boolean isConcurrencyAllowed) {
this.isConcurrencyAllowed = isConcurrencyAllowed;
return this;
}
/**
* Set the JobDetail's {@link JobDataMap}
*
* @return the updated JobBuilder
* @see JobDetail#getJobDataMap()
*/
public JobBuilder usingJobData(JobDataMap newJobDataMap) {
this.jobDataMap = newJobDataMap; // set new map as the map to use
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy