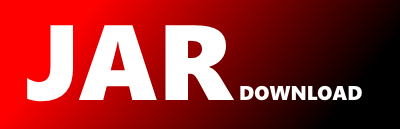
org.quartz.core.JobStore Maven / Gradle / Ivy
/*
* Copyright 2001-2009 Terracotta, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*
*/
package org.quartz.core;
import java.util.List;
import java.util.Set;
import org.quartz.exceptions.JobPersistenceException;
import org.quartz.exceptions.ObjectAlreadyExistsException;
import org.quartz.exceptions.SchedulerConfigException;
import org.quartz.exceptions.SchedulerException;
import org.quartz.jobs.JobDetail;
import org.quartz.triggers.OperableTrigger;
import org.quartz.triggers.Trigger;
import org.quartz.triggers.Trigger.CompletedExecutionInstruction;
/**
*
* The interface to be implemented by classes that want to provide a {@link org.quartz.jobs.Job}
and
* {@link org.quartz.triggers.Trigger}
storage mechanism for the {@link org.quartz.QuartzScheduler}
's use.
*
*
* Storage of Job
s and Trigger
s should be keyed on the combination of their name and group for uniqueness.
*
*
* @author James House
* @author Eric Mueller
*/
public interface JobStore {
/*
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Interface.
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*/
/**
* Called by the QuartzScheduler before the JobStore
is used, in order to give the it a chance to initialize.
*/
void initialize(SchedulerSignaler signaler) throws SchedulerConfigException;
/**
* Called by the QuartzScheduler to inform the JobStore
that the scheduler has started.
*/
void schedulerStarted() throws SchedulerException;
// ///////////////////////////////////////////////////////////////////////////
//
// Job & Trigger Storage methods
//
// ///////////////////////////////////////////////////////////////////////////
/**
* Store the given {@link org.quartz.jobs.JobDetail}
and {@link org.quartz.triggers.Trigger}
.
*
* @param newJob The JobDetail
to be stored.
* @param newTrigger The Trigger
to be stored.
* @throws ObjectAlreadyExistsException if a Job
with the same name/group already exists.
*/
void storeJobAndTrigger(JobDetail newJob, OperableTrigger newTrigger) throws ObjectAlreadyExistsException, JobPersistenceException;
/**
* Store the given {@link org.quartz.jobs.JobDetail}
.
*
* @param newJob The JobDetail
to be stored.
* @param replaceExisting If true
, any Job
existing in the JobStore
with the same name should be
* over-written.
* @throws ObjectAlreadyExistsException if a Job
with the same name/group already exists, and replaceExisting is set to false.
*/
void storeJob(JobDetail newJob, boolean replaceExisting) throws ObjectAlreadyExistsException, JobPersistenceException;
/**
* Remove (delete) the {@link org.quartz.jobs.Job}
with the given key, and any {@link org.quartz.triggers.Trigger}
s that
* reference it.
*
* If removal of the Job
results in an empty group, the group should be removed from the JobStore
's list of known group
* names.
*
*
* @return true
if a Job
with the given name & group was found and removed from the store.
*/
boolean removeJob(String jobKey) throws JobPersistenceException;
/**
* Retrieve the {@link org.quartz.jobs.JobDetail}
for the given {@link org.quartz.jobs.Job}
.
*
* @return The desired Job
, or null if there is no match.
*/
JobDetail retrieveJob(String jobKey) throws JobPersistenceException;
/**
* Store the given {@link org.quartz.triggers.Trigger}
.
*
* @param newTrigger The Trigger
to be stored.
* @param replaceExisting If true
, any Trigger
existing in the JobStore
with the same name & group should be
* over-written.
* @throws ObjectAlreadyExistsException if a Trigger
with the same name/group already exists, and replaceExisting is set to false.
* @see #pauseTriggers(org.quartz.impl.matchers.GroupMatcher)
*/
void storeTrigger(OperableTrigger newTrigger, boolean replaceExisting) throws ObjectAlreadyExistsException, JobPersistenceException;
/**
* Remove (delete) the {@link org.quartz.triggers.Trigger}
with the given key.
*
* If removal of the Trigger
results in an empty group, the group should be removed from the JobStore
's list of known
* group names.
*
*
* If removal of the Trigger
results in an 'orphaned' Job
that is not 'durable', then the Job
should be
* deleted also.
*
*
* @return true
if a Trigger
with the given name was found and removed from the store.
*/
boolean removeTrigger(String triggerKey) throws JobPersistenceException;
/**
* Remove (delete) the {@link org.quartz.triggers.Trigger}
with the given key, and store the new given one - which must be associated
* with the same job.
*
* @param newTrigger The new Trigger
to be stored.
* @return true
if a Trigger
with the given name was found and removed from the store.
*/
boolean replaceTrigger(String triggerKey, OperableTrigger newTrigger) throws JobPersistenceException;
/**
* Retrieve the given {@link org.quartz.triggers.Trigger}
.
*
* @return The desired Trigger
, or null if there is no match.
*/
OperableTrigger retrieveTrigger(String triggerKey) throws JobPersistenceException;
/**
* Retrieve the given {@link org.quartz.triggers.Trigger}
.
*
* @param calName The name of the Calendar
to be retrieved.
* @return The desired Calendar
, or null if there is no match.
*/
Calendar retrieveCalendar(String calName) throws JobPersistenceException;
// ///////////////////////////////////////////////////////////////////////////
//
// Informational methods
//
// ///////////////////////////////////////////////////////////////////////////
/**
* Get all of the Triggers that are associated to the given Job.
*
* If there are no matches, a zero-length array should be returned.
*
*/
List getTriggersForJob(String jobKey) throws JobPersistenceException;
/**
* Get the keys of all of the {@link org.quartz.jobs.Job}
s
*
* If there are no jobs in the given group name, the result should be an empty collection (not null
).
*
*/
Set getJobKeys() throws JobPersistenceException;
// ///////////////////////////////////////////////////////////////////////////
//
// Trigger State manipulation methods
//
// ///////////////////////////////////////////////////////////////////////////
// ///////////////////////////////////////////////////////////////////////////
//
// Trigger-Firing methods
//
// ///////////////////////////////////////////////////////////////////////////
/**
* Get a handle to the next trigger to be fired, and mark it as 'reserved' by the calling scheduler.
*
* @param noLaterThan If > 0, the JobStore should only return a Trigger that will fire no later than the time represented in this value as
* milliseconds.
* @see #releaseAcquiredTrigger(Trigger)
*/
List acquireNextTriggers(long noLaterThan, int maxCount, long timeWindow) throws JobPersistenceException;
/**
* Inform the JobStore
that the scheduler no longer plans to fire the given Trigger
, that it had previously acquired
* (reserved).
*/
void releaseAcquiredTrigger(OperableTrigger trigger) throws JobPersistenceException;
/**
* Inform the JobStore
that the scheduler is now firing the given Trigger
(executing its associated Job
),
* that it had previously acquired (reserved).
*
* @return may return null if all the triggers or their calendars no longer exist, or if the trigger was not successfully put into the 'executing'
* state. Preference is to return an empty list if none of the triggers could be fired.
*/
List triggersFired(List triggers) throws JobPersistenceException;
/**
* Inform the JobStore
that the scheduler has completed the firing of the given Trigger
(and the execution of its
* associated Job
completed, threw an exception, or was vetoed), and that the {@link org.quartz.jobs.JobDataMap}
in the
* given JobDetail
should be updated if the Job
is stateful.
*/
void triggeredJobComplete(OperableTrigger trigger, JobDetail jobDetail, CompletedExecutionInstruction triggerInstCode)
throws JobPersistenceException;
/**
* Tells the JobStore the pool size used to execute jobs
*
* @param poolSize amount of threads allocated for job execution
* @since 2.0
*/
void setThreadPoolSize(int poolSize);
}