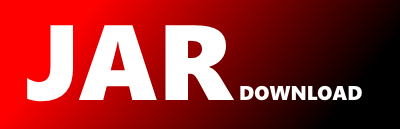
org.quartz.core.Scheduler Maven / Gradle / Ivy
/**
* Copyright 2001-2009 Terracotta, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*
*/
package org.quartz.core;
import java.util.Date;
import java.util.List;
import java.util.Set;
import org.quartz.classloading.CascadingClassLoadHelper;
import org.quartz.exceptions.SchedulerException;
import org.quartz.jobs.JobDataMap;
import org.quartz.jobs.JobDetail;
import org.quartz.listeners.JobListener;
import org.quartz.listeners.ListenerManager;
import org.quartz.listeners.SchedulerListener;
import org.quartz.listeners.TriggerListener;
import org.quartz.triggers.OperableTrigger;
import org.quartz.triggers.Trigger;
/**
* This is the main interface of a Quartz Scheduler.
*
* A Scheduler
maintains a registry of {@link org.quartz.jobs.JobDetail}
s and {@link Trigger}
s. Once
* registered, the Scheduler
is responsible for executing Job
s when their associated Trigger
s fire (when
* their scheduled time arrives).
*
*
* Scheduler
instances are produced by a {@link SchedulerFactory}
. A scheduler that has already been created/initialized can
* be found and used through the same factory that produced it. After a Scheduler
has been created, it is in "stand-by" mode, and must
* have its start()
method called before it will fire any Job
s.
*
*
* Job
s are to be created by the 'client program', by defining a class that implements the {@link org.quartz.jobs.Job}
* interface. {@link JobDetail}
objects are then created (also by the client) to define a individual instances of the Job
.
* JobDetail
instances can then be registered with the Scheduler
via the scheduleJob(JobDetail, Trigger)
or
* addJob(JobDetail, boolean)
method.
*
*
* Trigger
s can then be defined to fire individual Job
instances based on given schedules. SimpleTrigger
s are
* most useful for one-time firings, or firing at an exact moment in time, with N repeats with a given delay between them. CronTrigger
s
* allow scheduling based on time of day, day of week, day of month, and month of year.
*
*
* Job
s and Trigger
s have a name and group associated with them, which should uniquely identify them within a single
* {@link Scheduler}
. The 'group' feature may be useful for creating logical groupings or categorizations of Jobs
s and
* Triggers
s. If you don't have need for assigning a group to a given Jobs
of Triggers
, then you can use the
* DEFAULT_GROUP
constant defined on this interface.
*
*
* Stored Job
s can also be 'manually' triggered through the use of the triggerJob(String jobName, String jobGroup)
* function.
*
*
* Client programs may also be interested in the 'listener' interfaces that are available from Quartz. The {@link JobListener}
interface
* provides notifications of Job
executions. The {@link TriggerListener}
interface provides notifications of
* Trigger
firings. The {@link SchedulerListener}
interface provides notifications of Scheduler
events and
* errors. Listeners can be associated with local schedulers through the {@link ListenerManager} interface.
*
*
* The setup/configuration of a Scheduler
instance is very customizable. Please consult the documentation distributed with Quartz.
*
*
* @author James House
* @author Sharada Jambula
*/
public interface Scheduler {
/*
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Interface.
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*/
// /////////////////////////////////////////////////////////////////////////
// /
// / Scheduler State Management Methods
// /
// /////////////////////////////////////////////////////////////////////////
/**
* Starts the Scheduler
's threads that fire {@link Trigger}s
. When a scheduler is first created it is in "stand-by" mode,
* and will not fire triggers. The scheduler can also be put into stand-by mode by calling the standby()
method.
*
* The misfire/recovery process will be started, if it is the initial call to this method on this scheduler instance.
*
*
* @throws SchedulerException if shutdown()
has been called, or there is an error within the Scheduler
.
*/
void start() throws SchedulerException;
/**
* Calls {#start()} after the indicated number of seconds. (This call does not block). This can be useful within applications that have initializers
* that create the scheduler immediately, before the resources needed by the executing jobs have been fully initialized.
*
* @throws SchedulerException if shutdown()
has been called, or there is an error within the Scheduler
.
*/
void startDelayed(int seconds) throws SchedulerException;
/**
* Whether the scheduler has been started.
*
* Note: This only reflects whether {@link #start()}
has ever been called on this Scheduler, so it will return true
even
* if the Scheduler
is currently in standby mode or has been since shutdown.
*
*/
public boolean isStarted() throws SchedulerException;
/**
* Temporarily halts the Scheduler
's firing of {@link Trigger}s
.
*
* When start()
is called (to bring the scheduler out of stand-by mode), trigger misfire instructions will NOT be applied during the
* execution of the start()
method - any misfires will be detected immediately afterward (by the JobStore
's normal
* process).
*
*
* The scheduler is not destroyed, and can be re-started at any time.
*
*/
void standby() throws SchedulerException;
/**
* Reports whether the Scheduler
is in stand-by mode.
*/
boolean isInStandbyMode() throws SchedulerException;
/**
* Halts the Scheduler
's firing of {@link Trigger}s
, and cleans up all resources associated with the Scheduler.
*
* The scheduler cannot be re-started.
*
*
* @param waitForJobsToComplete if true
the scheduler will not allow this method to return until all currently executing jobs have
* completed.
*/
void shutdown(boolean waitForJobsToComplete) throws SchedulerException;
/**
* Reports whether the Scheduler
has been shutdown.
*/
boolean isShutdown() throws SchedulerException;
/**
* Return a list of JobExecutionContext
objects that represent all currently executing Jobs in this Scheduler instance.
*
* This method is not cluster aware. That is, it will only return Jobs currently executing in this Scheduler instance, not across the entire
* cluster.
*
*
* Note that the list returned is an 'instantaneous' snap-shot, and that as soon as it's returned, the true list of executing jobs may be different.
* Also please read the doc associated with JobExecutionContext
- especially if you're using RMI.
*
*/
List getCurrentlyExecutingJobs() throws SchedulerException;
/**
* Get the keys of all the {@link org.quartz.jobs.JobDetail}s
in the matching groups.
*
* @param matcher Matcher to evaluate against known groups
* @return Set of all keys matching
* @throws SchedulerException On error
*/
Set getJobKeys() throws SchedulerException;
/**
* Get a reference to the scheduler's ListenerManager
, through which listeners may be registered.
*
* @return the scheduler's ListenerManager
* @throws SchedulerException if the scheduler is not local
*/
ListenerManager getListenerManager() throws SchedulerException;
// /////////////////////////////////////////////////////////////////////////
// /
// / Scheduling-related Methods
// /
// /////////////////////////////////////////////////////////////////////////
/**
* Add the given {@link org.quartz.jobs.JobDetail}
to the Scheduler, and associate the given {@link OperableTrigger}
with
* it.
*
* If the given Trigger does not reference any Job
, then it will be set to reference the Job passed with it into this method.
*
*
* @throws SchedulerException if the Job or Trigger cannot be added to the Scheduler, or there is an internal Scheduler error.
*/
Date scheduleJob(JobDetail jobDetail, OperableTrigger trigger) throws SchedulerException;
/**
* Schedule the given {@link org.quartz.triggers.OperableTrigger}
with the Job
identified by the Trigger
's
* settings.
*
* @throws SchedulerException if the indicated Job does not exist, or the Trigger cannot be added to the Scheduler, or there is an internal
* Scheduler error.
*/
Date scheduleJob(OperableTrigger trigger) throws SchedulerException;
/**
* Remove (delete) the {@link org.quartz.triggers.OperableTrigger}
with the given key, and store the new given one - which must be
* associated with the same job (the new trigger must have the job name specified) - however, the new trigger need not have the same name as the old
* trigger.
*
* @param triggerName identity of the trigger to replace
* @param newTrigger The new Trigger
to be stored.
* @return null
if a Trigger
with the given name & group was not found and removed from the store, otherwise the first
* fire time of the newly scheduled trigger.
*/
Date rescheduleJob(String triggerName, OperableTrigger newTrigger) throws SchedulerException;
/**
* Add the given Job
to the Scheduler - with no associated Trigger
. The Job
will be 'dormant' until it is
* scheduled with a Trigger
, or Scheduler.triggerJob()
is called for it.
*
* The Job
must by definition be 'durable', if it is not, SchedulerException will be thrown.
*
*
* @throws SchedulerException if there is an internal Scheduler error, or if the Job is not durable, or a Job with the same name already exists, and
* replace
is false
.
*/
void addJob(JobDetail jobDetail) throws SchedulerException;
/**
* Trigger the identified {@link org.quartz.jobs.JobDetail}
(execute it now).
*
* @param data the (possibly null
) JobDataMap to be associated with the trigger that fires the job immediately.
*/
void triggerJob(String jobKey, JobDataMap data) throws SchedulerException;
/**
* Get all {@link Trigger}
s that are associated with the identified {@link org.quartz.jobs.JobDetail}
.
*
* The returned Trigger objects will be snap-shots of the actual stored triggers. If you wish to modify a trigger, you must re-store the trigger
* afterward (e.g. see {@link #rescheduleJob(TriggerKey, Trigger)}).
*
*/
List getTriggersOfJob(String jobKey) throws SchedulerException;
/**
* Get the {@link JobDetail}
for the Job
instance with the given key.
*
* The returned JobDetail object will be a snap-shot of the actual stored JobDetail. If you wish to modify the JobDetail, you must re-store the
* JobDetail afterward (e.g. see {@link #addJob(JobDetail, boolean)}).
*
*/
JobDetail getJobDetail(String jobKey) throws SchedulerException;
/**
* Get the {@link Trigger}
instance with the given key.
*
* The returned Trigger object will be a snap-shot of the actual stored trigger. If you wish to modify the trigger, you must re-store the trigger
* afterward (e.g. see {@link #rescheduleJob(TriggerKey, Trigger)}).
*
*/
Trigger getTrigger(String triggerKey) throws SchedulerException;
/**
* Delete the identified Job
from the Scheduler - and any associated Trigger
s.
*
* @return true if the Job was found and deleted.
* @throws SchedulerException if there is an internal Scheduler error.
*/
void deleteJob(String jobKey) throws SchedulerException;
/**
* Remove the indicated {@link Trigger}
from the scheduler.
*
* If the related job does not have any other triggers, and the job is not durable, then the job will also be deleted.
*
*/
void unscheduleJob(String triggerKey) throws SchedulerException;
CascadingClassLoadHelper getCascadingClassLoadHelper();
}