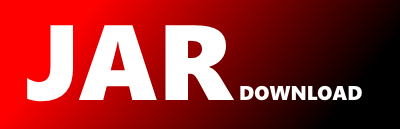
org.quartz.triggers.Trigger Maven / Gradle / Ivy
/**
* Copyright 2001-2009 Terracotta, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*
*/
package org.quartz.triggers;
import java.io.Serializable;
import java.util.Comparator;
import java.util.Date;
import org.quartz.builders.TriggerBuilder;
import org.quartz.core.Calendar;
import org.quartz.core.Scheduler;
import org.quartz.jobs.JobDataMap;
/**
* The base interface with properties common to all Trigger
s - use {@link TriggerBuilder} to instantiate an actual Trigger.
*
* Triggers
s have a {@link TriggerKey} associated with them, which should uniquely identify them within a single
* {@link Scheduler}
.
*
*
* Trigger
s are the 'mechanism' by which Job
s are scheduled. Many Trigger
s can point to the same
* Job
, but a single Trigger
can only point to one Job
.
*
*
* Triggers can 'send' parameters/data to Job
s by placing contents into the JobDataMap
on the Trigger
.
*
*
* @author James House
*/
public interface Trigger extends Serializable, Cloneable, Comparable {
public static final long serialVersionUID = -3904243490805975570L;
/**
*
* NOOP
Instructs the {@link Scheduler}
that the {@link Trigger}
has no further instructions.
*
*
* RE_EXECUTE_JOB
Instructs the {@link Scheduler}
that the {@link Trigger}
wants the
* {@link org.quartz.jobs.JobDetail}
to re-execute immediately. If not in a 'RECOVERING' or 'FAILED_OVER' situation, the execution
* context will be re-used (giving the Job
the ability to 'see' anything placed in the context by its last execution).
*
*
* SET_TRIGGER_COMPLETE
Instructs the {@link Scheduler}
that the {@link Trigger}
should be put in the
* COMPLETE
state.
*
*
* DELETE_TRIGGER
Instructs the {@link Scheduler}
that the {@link Trigger}
wants itself deleted.
*
*
* SET_ALL_JOB_TRIGGERS_COMPLETE
Instructs the {@link Scheduler}
that all Trigger
s referencing the same
* {@link org.quartz.jobs.JobDetail}
as this one should be put in the COMPLETE
state.
*
*
* SET_TRIGGER_ERROR
Instructs the {@link Scheduler}
that all Trigger
s referencing the same
* {@link org.quartz.jobs.JobDetail}
as this one should be put in the ERROR
state.
*
*
* SET_ALL_JOB_TRIGGERS_ERROR
Instructs the {@link Scheduler}
that the Trigger
should be put in the
* ERROR
state.
*
*/
public enum CompletedExecutionInstruction {
NOOP, RE_EXECUTE_JOB, SET_TRIGGER_COMPLETE, DELETE_TRIGGER, SET_ALL_JOB_TRIGGERS_COMPLETE, SET_TRIGGER_ERROR, SET_ALL_JOB_TRIGGERS_ERROR
};
/**
* Instructs the {@link Scheduler}
that upon a mis-fire situation, the updateAfterMisfire()
method will be called on the
* Trigger
to determine the mis-fire instruction, which logic will be trigger-implementation-dependent.
*
* In order to see if this instruction fits your needs, you should look at the documentation for the getSmartMisfirePolicy()
method on
* the particular Trigger
implementation you are using.
*
*/
public static final int MISFIRE_INSTRUCTION_SMART_POLICY = 0;
/**
* Instructs the {@link Scheduler}
that the Trigger
will never be evaluated for a misfire situation, and that the
* scheduler will simply try to fire it as soon as it can, and then update the Trigger as if it had fired at the proper time.
*
* NOTE: if a trigger uses this instruction, and it has missed several of its scheduled firings, then
*/
public static final int MISFIRE_INSTRUCTION_IGNORE_MISFIRE_POLICY = -1;
/**
* The default value for priority.
*/
public static final int DEFAULT_PRIORITY = 5;
/**
*
* Get the name of this Trigger
.
*
*/
public String getName();
/**
*
* Get the name of the associated {@link org.quartz.jobs.JobDetail}
.
*
*/
public String getJobName();
/**
* Return the description given to the Trigger
instance by its creator (if any).
*
* @return null if no description was set.
*/
public String getDescription();
/**
* Get the name of the {@link Calendar}
associated with this Trigger.
*
* @return null
if there is no associated Calendar.
*/
public String getCalendarName();
/**
* Get the JobDataMap
that is associated with the Trigger
.
*
* Changes made to this map during job execution are not re-persisted, and in fact typically result in an IllegalStateException
.
*
*/
public JobDataMap getJobDataMap();
/**
* The priority of a Trigger
acts as a tiebreaker such that if two Trigger
s have the same scheduled fire time, then the
* one with the higher priority will get first access to a worker thread.
*
* If not explicitly set, the default value is 5
.
*
*
* @see #DEFAULT_PRIORITY
*/
public int getPriority();
/**
* Used by the {@link Scheduler}
to determine whether or not it is possible for this Trigger
to fire again.
*
* If the returned value is false
then the Scheduler
may remove the Trigger
from the
* {@link org.quartz.core.JobStore}
.
*
*/
public boolean mayFireAgain();
/**
* Get the time at which the Trigger
should occur.
*/
public Date getStartTime();
/**
* Get the time at which the Trigger
should quit repeating - regardless of any remaining repeats (based on the trigger's particular
* repeat settings).
*
* @see #getFinalFireTime()
*/
public Date getEndTime();
/**
* Returns the next time at which the Trigger
is scheduled to fire. If the trigger will not fire again, null
will be
* returned. Note that the time returned can possibly be in the past, if the time that was computed for the trigger to next fire has already
* arrived, but the scheduler has not yet been able to fire the trigger (which would likely be due to lack of resources e.g. threads).
*
* The value returned is not guaranteed to be valid until after the Trigger
has been added to the scheduler.
*
*
* @see TriggerUtils#computeFireTimesBetween(Trigger, Calendar, Date, Date)
*/
public Date getNextFireTime();
/**
* Returns the previous time at which the Trigger
fired. If the trigger has not yet fired, null
will be returned.
*/
public Date getPreviousFireTime();
/**
* Returns the next time at which the Trigger
will fire, after the given time. If the trigger will not fire after the given time,
* null
will be returned.
*/
public Date getFireTimeAfter(Date afterTime);
/**
* Returns the last time at which the Trigger
will fire, if the Trigger will repeat indefinitely, null will be returned.
*
* Note that the return time *may* be in the past.
*
*/
public Date getFinalFireTime();
/**
* Get the instruction the Scheduler
should be given for handling misfire situations for this Trigger
- the concrete
* Trigger
type that you are using will have defined a set of additional MISFIRE_INSTRUCTION_XXX
constants that may be set
* as this property's value.
*
* If not explicitly set, the default value is MISFIRE_INSTRUCTION_SMART_POLICY
.
*
*
* @see #MISFIRE_INSTRUCTION_SMART_POLICY
* @see #updateAfterMisfire(Calendar)
* @see SimpleTrigger
* @see CronTrigger
*/
public int getMisfireInstruction();
/**
* Trigger equality is based upon the equality of the TriggerKey.
*
* @return true if the key of this Trigger equals that of the given Trigger.
*/
@Override
public boolean equals(Object other);
/**
* A Comparator that compares trigger's next fire times, or in other words, sorts them according to earliest next fire time. If the fire times are
* the same, then the triggers are sorted according to priority (highest value first), if the priorities are the same, then they are sorted by key.
*/
class TriggerTimeComparator implements Comparator, Serializable {
@Override
public int compare(Trigger trig1, Trigger trig2) {
Date t1 = trig1.getNextFireTime();
Date t2 = trig2.getNextFireTime();
if (t1 != null || t2 != null) {
if (t1 == null) {
return 1;
}
if (t2 == null) {
return -1;
}
if (t1.before(t2)) {
return -1;
}
if (t1.after(t2)) {
return 1;
}
}
int comp = trig2.getPriority() - trig1.getPriority();
if (comp != 0) {
return comp;
}
return trig1.getName().compareTo(trig2.getName());
}
}
}