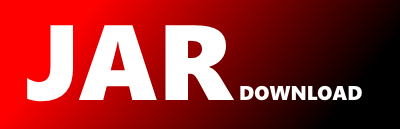
com.xfvape.swagger.spring.boot.autoconfigure.SwaggerAutoConfigration Maven / Gradle / Ivy
The newest version!
package com.xfvape.swagger.spring.boot.autoconfigure;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.autoconfigure.condition.ConditionalOnBean;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.boot.autoconfigure.condition.ConditionalOnWebApplication;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import io.swagger.annotations.SwaggerDefinition;
import io.swagger.models.Swagger;
import springfox.documentation.builders.ApiInfoBuilder;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.service.Contact;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.DocumentationCache;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
import springfox.documentation.swagger2.mappers.ServiceModelToSwagger2Mapper;
import springfox.documentation.swagger2.web.Swagger2Controller;
@Configuration
@EnableSwagger2 // Unable to infer base url. This is common when using dynamic servlet
// registration or when the API is behind an API Gateway. The base url is the
// root of where all the swagger resources are served. For e.g. if the api is
// available at http://example.org/api/v2/api-docs then the base url is
// http://example.org/api/. Please enter the location manually:
@ConditionalOnClass({ DocumentationType.class, Swagger.class, Swagger2Controller.class, SwaggerDefinition.class })
@EnableConfigurationProperties(SwaggerProperties.class)
@ConditionalOnWebApplication
public class SwaggerAutoConfigration {
private Logger logger = LoggerFactory.getLogger(getClass());
@Autowired
private SwaggerProperties swaggerProperties;
@Bean
@ConditionalOnMissingBean(Contact.class)
public Contact contact() {
com.xfvape.swagger.spring.boot.autoconfigure.SwaggerProperties.Contact contact = swaggerProperties.getContact();
if (contact == null) {
return new Contact("wenhaoran", "", "");
}
return new Contact(contact.getName(), contact.getUrl(), contact.getEmail());
}
@Bean
@ConditionalOnMissingBean(ApiInfo.class)
public ApiInfo apiInfo(Contact contact) {
return new ApiInfoBuilder().title(swaggerProperties.getTitle()).description(swaggerProperties.getDescription())
.termsOfServiceUrl(swaggerProperties.getTermsOfServiceUrl()).contact(contact)
.version(swaggerProperties.getVersion()).build();
}
@Bean
@ConditionalOnMissingBean(Docket.class)
@ConditionalOnProperty(value = "spring.swagger.scan-package")
public Docket createRestApi(Contact contact) {
logger.info("Swagger scan Package: " + swaggerProperties.getScanPackage());
return new Docket(DocumentationType.SWAGGER_2).useDefaultResponseMessages(false).apiInfo(apiInfo(contact))
.select().apis(RequestHandlerSelectors.basePackage(swaggerProperties.getScanPackage()))
.paths(PathSelectors.any()).build();
}
@Bean
@ConditionalOnBean({ DocumentationCache.class, ServiceModelToSwagger2Mapper.class })
public Swagger2Facade swagger2Facade(DocumentationCache documentationCache, ServiceModelToSwagger2Mapper mapper) {
return new Swagger2Facade(documentationCache, mapper);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy