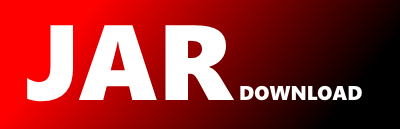
com.xiaoleilu.hutool.cron.Scheduler Maven / Gradle / Ivy
package com.xiaoleilu.hutool.cron;
import java.util.Map.Entry;
import java.util.TimeZone;
import java.util.UUID;
import com.xiaoleilu.hutool.collection.CollectionUtil;
import com.xiaoleilu.hutool.convert.Convert;
import com.xiaoleilu.hutool.cron.listener.TaskListener;
import com.xiaoleilu.hutool.cron.listener.TaskListenerManager;
import com.xiaoleilu.hutool.cron.pattern.CronPattern;
import com.xiaoleilu.hutool.cron.task.InvokeTask;
import com.xiaoleilu.hutool.cron.task.RunnableTask;
import com.xiaoleilu.hutool.cron.task.Task;
import com.xiaoleilu.hutool.setting.Setting;
import com.xiaoleilu.hutool.thread.ThreadUtil;
/**
* 任务调度器
*
* 调度器启动流程:
*
*
* 启动Timer =》 启动TaskLauncher =》 启动TaskExecutor
*
*
* 调度器关闭流程:
*
*
* 关闭Timer =》 关闭所有运行中的TaskLauncher =》 关闭所有运行中的TaskExecutor
*
*
* 其中:
*
*
* TaskLauncher:定时器每分钟调用一次(如果{@link Scheduler#isMatchSecond()}为true
每秒调用一次),
* 负责检查TaskTable是否有匹配到此时间运行的Task
*
*
*
* TaskExecutor:TaskLauncher匹配成功后,触发TaskExecutor执行具体的作业,执行完毕销毁
*
*
* @author Looly
*
*/
public class Scheduler {
private Object lock = new Object();
/** 时区 */
private TimeZone timezone;
/** 是否已经启动 */
private boolean started = false;
/** 是否支持秒匹配 */
protected boolean matchSecond = false;
/** 是否支持年匹配 */
protected boolean matchYear = false;
/** 是否为守护线程 */
protected boolean daemon;
/** 定时器 */
private CronTimer timer;
/** 定时任务表 */
protected TaskTable taskTable = new TaskTable(this);
/** 启动器管理器 */
protected TaskLauncherManager taskLauncherManager;
/** 执行器管理器 */
protected TaskExecutorManager taskExecutorManager;
/** 监听管理器列表 */
protected TaskListenerManager listenerManager = new TaskListenerManager();
// --------------------------------------------------------- Getters and Setters start
/**
* 设置时区
*
* @param timezone 时区
* @return this
*/
public Scheduler setTimeZone(TimeZone timezone) {
this.timezone = timezone;
return this;
}
/**
* 获得时区,默认为 {@link TimeZone#getDefault()}
*
* @return 时区
*/
public TimeZone getTimeZone() {
return timezone != null ? timezone : TimeZone.getDefault();
}
/**
* 设置是否为守护线程
* 默认非守护线程
*
* @param on true
为守护线程,否则非守护线程
* @return this
* @throws CronException 定时任务已经启动抛出此异常
*/
public Scheduler setDaemon(boolean on) throws CronException {
synchronized (lock) {
if (started) {
throw new CronException("Scheduler already started!");
}
this.daemon = on;
}
return this;
}
/**
* 是否为守护线程
*
* @return 是否为守护线程
*/
public boolean isDeamon() {
return this.daemon;
}
/**
* 是否支持秒匹配
*
* @return true
使用,false
不使用
*/
public boolean isMatchSecond() {
return this.matchSecond;
}
/**
* 设置是否支持秒匹配,默认不使用
*
* @param isMatchSecond true
支持,false
不支持
* @return this
*/
public Scheduler setMatchSecond(boolean isMatchSecond) {
this.matchSecond = isMatchSecond;
return this;
}
/**
* 是否支持年匹配
*
* @return true
使用,false
不使用
*/
public boolean isMatchYear() {
return this.matchYear;
}
/**
* 设置是否支持年匹配,默认不使用
*
* @param isMatchYear true
支持,false
不支持
* @return this
*/
public Scheduler setMatchYear(boolean isMatchYear) {
this.matchYear = isMatchYear;
return this;
}
/**
* 增加监听器
*
* @param listener {@link TaskListener}
* @return this
*/
public Scheduler addListener(TaskListener listener) {
this.listenerManager.addListener(listener);
return this;
}
/**
* 移除监听器
*
* @param listener {@link TaskListener}
* @return this
*/
public Scheduler removeListener(TaskListener listener) {
this.listenerManager.removeListener(listener);
return this;
}
// --------------------------------------------------------- Getters and Setters end
// -------------------------------------------------------------------- shcedule start
/**
* 批量加入配置文件中的定时任务
* 配置文件格式为: xxx.xxx.xxx.Class.method = * * * * *
*
* @param cronSetting 定时任务设置文件
* @return this
*/
public Scheduler schedule(Setting cronSetting) {
if (CollectionUtil.isNotEmpty(cronSetting)) {
for (Entry
© 2015 - 2024 Weber Informatics LLC | Privacy Policy