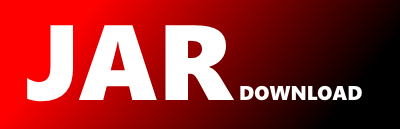
com.xiaomi.infra.galaxy.emr.thrift.InstanceDetail Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.2)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.xiaomi.infra.galaxy.emr.thrift;
import libthrift091.scheme.IScheme;
import libthrift091.scheme.SchemeFactory;
import libthrift091.scheme.StandardScheme;
import libthrift091.scheme.TupleScheme;
import libthrift091.protocol.TTupleProtocol;
import libthrift091.protocol.TProtocolException;
import libthrift091.EncodingUtils;
import libthrift091.TException;
import libthrift091.async.AsyncMethodCallback;
import libthrift091.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.2)", date = "2016-8-2")
public class InstanceDetail implements libthrift091.TBase, java.io.Serializable, Cloneable, Comparable {
private static final libthrift091.protocol.TStruct STRUCT_DESC = new libthrift091.protocol.TStruct("InstanceDetail");
private static final libthrift091.protocol.TField INSTANCE_ID_FIELD_DESC = new libthrift091.protocol.TField("instanceId", libthrift091.protocol.TType.STRING, (short)1);
private static final libthrift091.protocol.TField OS_INSTANCE_ID_FIELD_DESC = new libthrift091.protocol.TField("osInstanceId", libthrift091.protocol.TType.STRING, (short)2);
private static final libthrift091.protocol.TField NAME_FIELD_DESC = new libthrift091.protocol.TField("name", libthrift091.protocol.TType.STRING, (short)3);
private static final libthrift091.protocol.TField INSTANCE_TYPE_FIELD_DESC = new libthrift091.protocol.TField("instanceType", libthrift091.protocol.TType.STRING, (short)4);
private static final libthrift091.protocol.TField PRIVATE_IP_ADDRESS_FIELD_DESC = new libthrift091.protocol.TField("privateIpAddress", libthrift091.protocol.TType.STRING, (short)5);
private static final libthrift091.protocol.TField PUBLIC_IP_ADDRESS_FIELD_DESC = new libthrift091.protocol.TField("publicIpAddress", libthrift091.protocol.TType.STRING, (short)6);
private static final libthrift091.protocol.TField PRIVATE_DNS_NAME_FIELD_DESC = new libthrift091.protocol.TField("privateDnsName", libthrift091.protocol.TType.STRING, (short)7);
private static final libthrift091.protocol.TField PUBLIC_DNS_NAME_FIELD_DESC = new libthrift091.protocol.TField("publicDnsName", libthrift091.protocol.TType.STRING, (short)8);
private static final libthrift091.protocol.TField INSTANCE_STATUS_FIELD_DESC = new libthrift091.protocol.TField("instanceStatus", libthrift091.protocol.TType.STRUCT, (short)9);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new InstanceDetailStandardSchemeFactory());
schemes.put(TupleScheme.class, new InstanceDetailTupleSchemeFactory());
}
public String instanceId; // required
public String osInstanceId; // required
public String name; // optional
public String instanceType; // optional
public String privateIpAddress; // optional
public String publicIpAddress; // optional
public String privateDnsName; // optional
public String publicDnsName; // optional
public Status instanceStatus; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements libthrift091.TFieldIdEnum {
INSTANCE_ID((short)1, "instanceId"),
OS_INSTANCE_ID((short)2, "osInstanceId"),
NAME((short)3, "name"),
INSTANCE_TYPE((short)4, "instanceType"),
PRIVATE_IP_ADDRESS((short)5, "privateIpAddress"),
PUBLIC_IP_ADDRESS((short)6, "publicIpAddress"),
PRIVATE_DNS_NAME((short)7, "privateDnsName"),
PUBLIC_DNS_NAME((short)8, "publicDnsName"),
INSTANCE_STATUS((short)9, "instanceStatus");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // INSTANCE_ID
return INSTANCE_ID;
case 2: // OS_INSTANCE_ID
return OS_INSTANCE_ID;
case 3: // NAME
return NAME;
case 4: // INSTANCE_TYPE
return INSTANCE_TYPE;
case 5: // PRIVATE_IP_ADDRESS
return PRIVATE_IP_ADDRESS;
case 6: // PUBLIC_IP_ADDRESS
return PUBLIC_IP_ADDRESS;
case 7: // PRIVATE_DNS_NAME
return PRIVATE_DNS_NAME;
case 8: // PUBLIC_DNS_NAME
return PUBLIC_DNS_NAME;
case 9: // INSTANCE_STATUS
return INSTANCE_STATUS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final _Fields optionals[] = {_Fields.NAME,_Fields.INSTANCE_TYPE,_Fields.PRIVATE_IP_ADDRESS,_Fields.PUBLIC_IP_ADDRESS,_Fields.PRIVATE_DNS_NAME,_Fields.PUBLIC_DNS_NAME,_Fields.INSTANCE_STATUS};
public static final Map<_Fields, libthrift091.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, libthrift091.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, libthrift091.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.INSTANCE_ID, new libthrift091.meta_data.FieldMetaData("instanceId", libthrift091.TFieldRequirementType.REQUIRED,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.STRING)));
tmpMap.put(_Fields.OS_INSTANCE_ID, new libthrift091.meta_data.FieldMetaData("osInstanceId", libthrift091.TFieldRequirementType.REQUIRED,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.STRING)));
tmpMap.put(_Fields.NAME, new libthrift091.meta_data.FieldMetaData("name", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.STRING)));
tmpMap.put(_Fields.INSTANCE_TYPE, new libthrift091.meta_data.FieldMetaData("instanceType", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.STRING)));
tmpMap.put(_Fields.PRIVATE_IP_ADDRESS, new libthrift091.meta_data.FieldMetaData("privateIpAddress", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.STRING)));
tmpMap.put(_Fields.PUBLIC_IP_ADDRESS, new libthrift091.meta_data.FieldMetaData("publicIpAddress", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.STRING)));
tmpMap.put(_Fields.PRIVATE_DNS_NAME, new libthrift091.meta_data.FieldMetaData("privateDnsName", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.STRING)));
tmpMap.put(_Fields.PUBLIC_DNS_NAME, new libthrift091.meta_data.FieldMetaData("publicDnsName", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.STRING)));
tmpMap.put(_Fields.INSTANCE_STATUS, new libthrift091.meta_data.FieldMetaData("instanceStatus", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.StructMetaData(libthrift091.protocol.TType.STRUCT, Status.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
libthrift091.meta_data.FieldMetaData.addStructMetaDataMap(InstanceDetail.class, metaDataMap);
}
public InstanceDetail() {
}
public InstanceDetail(
String instanceId,
String osInstanceId)
{
this();
this.instanceId = instanceId;
this.osInstanceId = osInstanceId;
}
/**
* Performs a deep copy on other.
*/
public InstanceDetail(InstanceDetail other) {
if (other.isSetInstanceId()) {
this.instanceId = other.instanceId;
}
if (other.isSetOsInstanceId()) {
this.osInstanceId = other.osInstanceId;
}
if (other.isSetName()) {
this.name = other.name;
}
if (other.isSetInstanceType()) {
this.instanceType = other.instanceType;
}
if (other.isSetPrivateIpAddress()) {
this.privateIpAddress = other.privateIpAddress;
}
if (other.isSetPublicIpAddress()) {
this.publicIpAddress = other.publicIpAddress;
}
if (other.isSetPrivateDnsName()) {
this.privateDnsName = other.privateDnsName;
}
if (other.isSetPublicDnsName()) {
this.publicDnsName = other.publicDnsName;
}
if (other.isSetInstanceStatus()) {
this.instanceStatus = new Status(other.instanceStatus);
}
}
public InstanceDetail deepCopy() {
return new InstanceDetail(this);
}
@Override
public void clear() {
this.instanceId = null;
this.osInstanceId = null;
this.name = null;
this.instanceType = null;
this.privateIpAddress = null;
this.publicIpAddress = null;
this.privateDnsName = null;
this.publicDnsName = null;
this.instanceStatus = null;
}
public String getInstanceId() {
return this.instanceId;
}
public InstanceDetail setInstanceId(String instanceId) {
this.instanceId = instanceId;
return this;
}
public void unsetInstanceId() {
this.instanceId = null;
}
/** Returns true if field instanceId is set (has been assigned a value) and false otherwise */
public boolean isSetInstanceId() {
return this.instanceId != null;
}
public void setInstanceIdIsSet(boolean value) {
if (!value) {
this.instanceId = null;
}
}
public String getOsInstanceId() {
return this.osInstanceId;
}
public InstanceDetail setOsInstanceId(String osInstanceId) {
this.osInstanceId = osInstanceId;
return this;
}
public void unsetOsInstanceId() {
this.osInstanceId = null;
}
/** Returns true if field osInstanceId is set (has been assigned a value) and false otherwise */
public boolean isSetOsInstanceId() {
return this.osInstanceId != null;
}
public void setOsInstanceIdIsSet(boolean value) {
if (!value) {
this.osInstanceId = null;
}
}
public String getName() {
return this.name;
}
public InstanceDetail setName(String name) {
this.name = name;
return this;
}
public void unsetName() {
this.name = null;
}
/** Returns true if field name is set (has been assigned a value) and false otherwise */
public boolean isSetName() {
return this.name != null;
}
public void setNameIsSet(boolean value) {
if (!value) {
this.name = null;
}
}
public String getInstanceType() {
return this.instanceType;
}
public InstanceDetail setInstanceType(String instanceType) {
this.instanceType = instanceType;
return this;
}
public void unsetInstanceType() {
this.instanceType = null;
}
/** Returns true if field instanceType is set (has been assigned a value) and false otherwise */
public boolean isSetInstanceType() {
return this.instanceType != null;
}
public void setInstanceTypeIsSet(boolean value) {
if (!value) {
this.instanceType = null;
}
}
public String getPrivateIpAddress() {
return this.privateIpAddress;
}
public InstanceDetail setPrivateIpAddress(String privateIpAddress) {
this.privateIpAddress = privateIpAddress;
return this;
}
public void unsetPrivateIpAddress() {
this.privateIpAddress = null;
}
/** Returns true if field privateIpAddress is set (has been assigned a value) and false otherwise */
public boolean isSetPrivateIpAddress() {
return this.privateIpAddress != null;
}
public void setPrivateIpAddressIsSet(boolean value) {
if (!value) {
this.privateIpAddress = null;
}
}
public String getPublicIpAddress() {
return this.publicIpAddress;
}
public InstanceDetail setPublicIpAddress(String publicIpAddress) {
this.publicIpAddress = publicIpAddress;
return this;
}
public void unsetPublicIpAddress() {
this.publicIpAddress = null;
}
/** Returns true if field publicIpAddress is set (has been assigned a value) and false otherwise */
public boolean isSetPublicIpAddress() {
return this.publicIpAddress != null;
}
public void setPublicIpAddressIsSet(boolean value) {
if (!value) {
this.publicIpAddress = null;
}
}
public String getPrivateDnsName() {
return this.privateDnsName;
}
public InstanceDetail setPrivateDnsName(String privateDnsName) {
this.privateDnsName = privateDnsName;
return this;
}
public void unsetPrivateDnsName() {
this.privateDnsName = null;
}
/** Returns true if field privateDnsName is set (has been assigned a value) and false otherwise */
public boolean isSetPrivateDnsName() {
return this.privateDnsName != null;
}
public void setPrivateDnsNameIsSet(boolean value) {
if (!value) {
this.privateDnsName = null;
}
}
public String getPublicDnsName() {
return this.publicDnsName;
}
public InstanceDetail setPublicDnsName(String publicDnsName) {
this.publicDnsName = publicDnsName;
return this;
}
public void unsetPublicDnsName() {
this.publicDnsName = null;
}
/** Returns true if field publicDnsName is set (has been assigned a value) and false otherwise */
public boolean isSetPublicDnsName() {
return this.publicDnsName != null;
}
public void setPublicDnsNameIsSet(boolean value) {
if (!value) {
this.publicDnsName = null;
}
}
public Status getInstanceStatus() {
return this.instanceStatus;
}
public InstanceDetail setInstanceStatus(Status instanceStatus) {
this.instanceStatus = instanceStatus;
return this;
}
public void unsetInstanceStatus() {
this.instanceStatus = null;
}
/** Returns true if field instanceStatus is set (has been assigned a value) and false otherwise */
public boolean isSetInstanceStatus() {
return this.instanceStatus != null;
}
public void setInstanceStatusIsSet(boolean value) {
if (!value) {
this.instanceStatus = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case INSTANCE_ID:
if (value == null) {
unsetInstanceId();
} else {
setInstanceId((String)value);
}
break;
case OS_INSTANCE_ID:
if (value == null) {
unsetOsInstanceId();
} else {
setOsInstanceId((String)value);
}
break;
case NAME:
if (value == null) {
unsetName();
} else {
setName((String)value);
}
break;
case INSTANCE_TYPE:
if (value == null) {
unsetInstanceType();
} else {
setInstanceType((String)value);
}
break;
case PRIVATE_IP_ADDRESS:
if (value == null) {
unsetPrivateIpAddress();
} else {
setPrivateIpAddress((String)value);
}
break;
case PUBLIC_IP_ADDRESS:
if (value == null) {
unsetPublicIpAddress();
} else {
setPublicIpAddress((String)value);
}
break;
case PRIVATE_DNS_NAME:
if (value == null) {
unsetPrivateDnsName();
} else {
setPrivateDnsName((String)value);
}
break;
case PUBLIC_DNS_NAME:
if (value == null) {
unsetPublicDnsName();
} else {
setPublicDnsName((String)value);
}
break;
case INSTANCE_STATUS:
if (value == null) {
unsetInstanceStatus();
} else {
setInstanceStatus((Status)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case INSTANCE_ID:
return getInstanceId();
case OS_INSTANCE_ID:
return getOsInstanceId();
case NAME:
return getName();
case INSTANCE_TYPE:
return getInstanceType();
case PRIVATE_IP_ADDRESS:
return getPrivateIpAddress();
case PUBLIC_IP_ADDRESS:
return getPublicIpAddress();
case PRIVATE_DNS_NAME:
return getPrivateDnsName();
case PUBLIC_DNS_NAME:
return getPublicDnsName();
case INSTANCE_STATUS:
return getInstanceStatus();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case INSTANCE_ID:
return isSetInstanceId();
case OS_INSTANCE_ID:
return isSetOsInstanceId();
case NAME:
return isSetName();
case INSTANCE_TYPE:
return isSetInstanceType();
case PRIVATE_IP_ADDRESS:
return isSetPrivateIpAddress();
case PUBLIC_IP_ADDRESS:
return isSetPublicIpAddress();
case PRIVATE_DNS_NAME:
return isSetPrivateDnsName();
case PUBLIC_DNS_NAME:
return isSetPublicDnsName();
case INSTANCE_STATUS:
return isSetInstanceStatus();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof InstanceDetail)
return this.equals((InstanceDetail)that);
return false;
}
public boolean equals(InstanceDetail that) {
if (that == null)
return false;
boolean this_present_instanceId = true && this.isSetInstanceId();
boolean that_present_instanceId = true && that.isSetInstanceId();
if (this_present_instanceId || that_present_instanceId) {
if (!(this_present_instanceId && that_present_instanceId))
return false;
if (!this.instanceId.equals(that.instanceId))
return false;
}
boolean this_present_osInstanceId = true && this.isSetOsInstanceId();
boolean that_present_osInstanceId = true && that.isSetOsInstanceId();
if (this_present_osInstanceId || that_present_osInstanceId) {
if (!(this_present_osInstanceId && that_present_osInstanceId))
return false;
if (!this.osInstanceId.equals(that.osInstanceId))
return false;
}
boolean this_present_name = true && this.isSetName();
boolean that_present_name = true && that.isSetName();
if (this_present_name || that_present_name) {
if (!(this_present_name && that_present_name))
return false;
if (!this.name.equals(that.name))
return false;
}
boolean this_present_instanceType = true && this.isSetInstanceType();
boolean that_present_instanceType = true && that.isSetInstanceType();
if (this_present_instanceType || that_present_instanceType) {
if (!(this_present_instanceType && that_present_instanceType))
return false;
if (!this.instanceType.equals(that.instanceType))
return false;
}
boolean this_present_privateIpAddress = true && this.isSetPrivateIpAddress();
boolean that_present_privateIpAddress = true && that.isSetPrivateIpAddress();
if (this_present_privateIpAddress || that_present_privateIpAddress) {
if (!(this_present_privateIpAddress && that_present_privateIpAddress))
return false;
if (!this.privateIpAddress.equals(that.privateIpAddress))
return false;
}
boolean this_present_publicIpAddress = true && this.isSetPublicIpAddress();
boolean that_present_publicIpAddress = true && that.isSetPublicIpAddress();
if (this_present_publicIpAddress || that_present_publicIpAddress) {
if (!(this_present_publicIpAddress && that_present_publicIpAddress))
return false;
if (!this.publicIpAddress.equals(that.publicIpAddress))
return false;
}
boolean this_present_privateDnsName = true && this.isSetPrivateDnsName();
boolean that_present_privateDnsName = true && that.isSetPrivateDnsName();
if (this_present_privateDnsName || that_present_privateDnsName) {
if (!(this_present_privateDnsName && that_present_privateDnsName))
return false;
if (!this.privateDnsName.equals(that.privateDnsName))
return false;
}
boolean this_present_publicDnsName = true && this.isSetPublicDnsName();
boolean that_present_publicDnsName = true && that.isSetPublicDnsName();
if (this_present_publicDnsName || that_present_publicDnsName) {
if (!(this_present_publicDnsName && that_present_publicDnsName))
return false;
if (!this.publicDnsName.equals(that.publicDnsName))
return false;
}
boolean this_present_instanceStatus = true && this.isSetInstanceStatus();
boolean that_present_instanceStatus = true && that.isSetInstanceStatus();
if (this_present_instanceStatus || that_present_instanceStatus) {
if (!(this_present_instanceStatus && that_present_instanceStatus))
return false;
if (!this.instanceStatus.equals(that.instanceStatus))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy