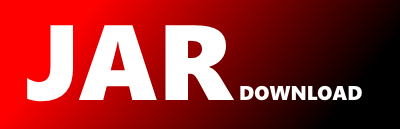
com.xiaomi.infra.galaxy.sds.thrift.GlobalSecondaryIndexSpec Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.2)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.xiaomi.infra.galaxy.sds.thrift;
import libthrift091.scheme.IScheme;
import libthrift091.scheme.SchemeFactory;
import libthrift091.scheme.StandardScheme;
import libthrift091.scheme.TupleScheme;
import libthrift091.protocol.TTupleProtocol;
import libthrift091.protocol.TProtocolException;
import libthrift091.EncodingUtils;
import libthrift091.TException;
import libthrift091.async.AsyncMethodCallback;
import libthrift091.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
/**
* 全局二级索引定义
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.2)", date = "2016-8-10")
public class GlobalSecondaryIndexSpec implements libthrift091.TBase, java.io.Serializable, Cloneable, Comparable {
private static final libthrift091.protocol.TStruct STRUCT_DESC = new libthrift091.protocol.TStruct("GlobalSecondaryIndexSpec");
private static final libthrift091.protocol.TField INDEX_ENTITY_GROUP_FIELD_DESC = new libthrift091.protocol.TField("indexEntityGroup", libthrift091.protocol.TType.STRUCT, (short)1);
private static final libthrift091.protocol.TField INDEX_PRIMARY_KEY_FIELD_DESC = new libthrift091.protocol.TField("indexPrimaryKey", libthrift091.protocol.TType.LIST, (short)2);
private static final libthrift091.protocol.TField PROJECTIONS_FIELD_DESC = new libthrift091.protocol.TField("projections", libthrift091.protocol.TType.LIST, (short)3);
private static final libthrift091.protocol.TField CONSISTENCY_LEVEL_FIELD_DESC = new libthrift091.protocol.TField("consistencyLevel", libthrift091.protocol.TType.I32, (short)4);
private static final libthrift091.protocol.TField UNIQUE_FIELD_DESC = new libthrift091.protocol.TField("unique", libthrift091.protocol.TType.BOOL, (short)5);
private static final libthrift091.protocol.TField THROUGHPUT_FIELD_DESC = new libthrift091.protocol.TField("throughput", libthrift091.protocol.TType.STRUCT, (short)6);
private static final libthrift091.protocol.TField SLAVE_THROUGHPUT_FIELD_DESC = new libthrift091.protocol.TField("slaveThroughput", libthrift091.protocol.TType.STRUCT, (short)7);
private static final libthrift091.protocol.TField EXCEEDED_THROUGHPUT_FIELD_DESC = new libthrift091.protocol.TField("exceededThroughput", libthrift091.protocol.TType.STRUCT, (short)8);
private static final libthrift091.protocol.TField EXCEEDED_SLAVE_THROUGHPUT_FIELD_DESC = new libthrift091.protocol.TField("exceededSlaveThroughput", libthrift091.protocol.TType.STRUCT, (short)9);
private static final libthrift091.protocol.TField PRE_SPLITS_FIELD_DESC = new libthrift091.protocol.TField("preSplits", libthrift091.protocol.TType.I32, (short)10);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new GlobalSecondaryIndexSpecStandardSchemeFactory());
schemes.put(TupleScheme.class, new GlobalSecondaryIndexSpecTupleSchemeFactory());
}
/**
* 索引表的实体组键
* 可用于对索引表进行hash
*/
public EntityGroupSpec indexEntityGroup; // optional
/**
* 索引表的主键
*/
public List indexPrimaryKey; // optional
/**
* 投影的属性,全局二级索引不能读取非投影属性
*/
public List projections; // optional
/**
* 索引数据一致性级别
*
* @see ConsistencyLevel
*/
public ConsistencyLevel consistencyLevel; // optional
/**
* 是否为唯一索引
*/
public boolean unique; // optional
/**
* 索引表的吞吐量配额
*/
public ProvisionThroughput throughput; // optional
/**
* 索引表的备集群的吞吐量配额
*/
public ProvisionThroughput slaveThroughput; // optional
/**
* 索引表的最大超发吞吐量配额
*/
public ProvisionThroughput exceededThroughput; // optional
/**
* 索引表的备集群的最大超发吞吐量配额
*/
public ProvisionThroughput exceededSlaveThroughput; // optional
/**
* 表初始分片数目,仅支持Entity Group开启hash分布的表,且仅在建表时起作用
*/
public int preSplits; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements libthrift091.TFieldIdEnum {
/**
* 索引表的实体组键
* 可用于对索引表进行hash
*/
INDEX_ENTITY_GROUP((short)1, "indexEntityGroup"),
/**
* 索引表的主键
*/
INDEX_PRIMARY_KEY((short)2, "indexPrimaryKey"),
/**
* 投影的属性,全局二级索引不能读取非投影属性
*/
PROJECTIONS((short)3, "projections"),
/**
* 索引数据一致性级别
*
* @see ConsistencyLevel
*/
CONSISTENCY_LEVEL((short)4, "consistencyLevel"),
/**
* 是否为唯一索引
*/
UNIQUE((short)5, "unique"),
/**
* 索引表的吞吐量配额
*/
THROUGHPUT((short)6, "throughput"),
/**
* 索引表的备集群的吞吐量配额
*/
SLAVE_THROUGHPUT((short)7, "slaveThroughput"),
/**
* 索引表的最大超发吞吐量配额
*/
EXCEEDED_THROUGHPUT((short)8, "exceededThroughput"),
/**
* 索引表的备集群的最大超发吞吐量配额
*/
EXCEEDED_SLAVE_THROUGHPUT((short)9, "exceededSlaveThroughput"),
/**
* 表初始分片数目,仅支持Entity Group开启hash分布的表,且仅在建表时起作用
*/
PRE_SPLITS((short)10, "preSplits");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // INDEX_ENTITY_GROUP
return INDEX_ENTITY_GROUP;
case 2: // INDEX_PRIMARY_KEY
return INDEX_PRIMARY_KEY;
case 3: // PROJECTIONS
return PROJECTIONS;
case 4: // CONSISTENCY_LEVEL
return CONSISTENCY_LEVEL;
case 5: // UNIQUE
return UNIQUE;
case 6: // THROUGHPUT
return THROUGHPUT;
case 7: // SLAVE_THROUGHPUT
return SLAVE_THROUGHPUT;
case 8: // EXCEEDED_THROUGHPUT
return EXCEEDED_THROUGHPUT;
case 9: // EXCEEDED_SLAVE_THROUGHPUT
return EXCEEDED_SLAVE_THROUGHPUT;
case 10: // PRE_SPLITS
return PRE_SPLITS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __UNIQUE_ISSET_ID = 0;
private static final int __PRESPLITS_ISSET_ID = 1;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.INDEX_ENTITY_GROUP,_Fields.INDEX_PRIMARY_KEY,_Fields.PROJECTIONS,_Fields.CONSISTENCY_LEVEL,_Fields.UNIQUE,_Fields.THROUGHPUT,_Fields.SLAVE_THROUGHPUT,_Fields.EXCEEDED_THROUGHPUT,_Fields.EXCEEDED_SLAVE_THROUGHPUT,_Fields.PRE_SPLITS};
public static final Map<_Fields, libthrift091.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, libthrift091.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, libthrift091.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.INDEX_ENTITY_GROUP, new libthrift091.meta_data.FieldMetaData("indexEntityGroup", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.StructMetaData(libthrift091.protocol.TType.STRUCT, EntityGroupSpec.class)));
tmpMap.put(_Fields.INDEX_PRIMARY_KEY, new libthrift091.meta_data.FieldMetaData("indexPrimaryKey", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.LIST , "IndexSpec")));
tmpMap.put(_Fields.PROJECTIONS, new libthrift091.meta_data.FieldMetaData("projections", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.LIST , "Attributes")));
tmpMap.put(_Fields.CONSISTENCY_LEVEL, new libthrift091.meta_data.FieldMetaData("consistencyLevel", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.EnumMetaData(libthrift091.protocol.TType.ENUM, ConsistencyLevel.class)));
tmpMap.put(_Fields.UNIQUE, new libthrift091.meta_data.FieldMetaData("unique", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.BOOL)));
tmpMap.put(_Fields.THROUGHPUT, new libthrift091.meta_data.FieldMetaData("throughput", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.StructMetaData(libthrift091.protocol.TType.STRUCT, ProvisionThroughput.class)));
tmpMap.put(_Fields.SLAVE_THROUGHPUT, new libthrift091.meta_data.FieldMetaData("slaveThroughput", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.StructMetaData(libthrift091.protocol.TType.STRUCT, ProvisionThroughput.class)));
tmpMap.put(_Fields.EXCEEDED_THROUGHPUT, new libthrift091.meta_data.FieldMetaData("exceededThroughput", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.StructMetaData(libthrift091.protocol.TType.STRUCT, ProvisionThroughput.class)));
tmpMap.put(_Fields.EXCEEDED_SLAVE_THROUGHPUT, new libthrift091.meta_data.FieldMetaData("exceededSlaveThroughput", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.StructMetaData(libthrift091.protocol.TType.STRUCT, ProvisionThroughput.class)));
tmpMap.put(_Fields.PRE_SPLITS, new libthrift091.meta_data.FieldMetaData("preSplits", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I32)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
libthrift091.meta_data.FieldMetaData.addStructMetaDataMap(GlobalSecondaryIndexSpec.class, metaDataMap);
}
public GlobalSecondaryIndexSpec() {
this.consistencyLevel = com.xiaomi.infra.galaxy.sds.thrift.ConsistencyLevel.STRONG;
this.unique = false;
this.preSplits = 1;
}
/**
* Performs a deep copy on other.
*/
public GlobalSecondaryIndexSpec(GlobalSecondaryIndexSpec other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetIndexEntityGroup()) {
this.indexEntityGroup = new EntityGroupSpec(other.indexEntityGroup);
}
if (other.isSetIndexPrimaryKey()) {
this.indexPrimaryKey = other.indexPrimaryKey;
}
if (other.isSetProjections()) {
this.projections = other.projections;
}
if (other.isSetConsistencyLevel()) {
this.consistencyLevel = other.consistencyLevel;
}
this.unique = other.unique;
if (other.isSetThroughput()) {
this.throughput = new ProvisionThroughput(other.throughput);
}
if (other.isSetSlaveThroughput()) {
this.slaveThroughput = new ProvisionThroughput(other.slaveThroughput);
}
if (other.isSetExceededThroughput()) {
this.exceededThroughput = new ProvisionThroughput(other.exceededThroughput);
}
if (other.isSetExceededSlaveThroughput()) {
this.exceededSlaveThroughput = new ProvisionThroughput(other.exceededSlaveThroughput);
}
this.preSplits = other.preSplits;
}
public GlobalSecondaryIndexSpec deepCopy() {
return new GlobalSecondaryIndexSpec(this);
}
@Override
public void clear() {
this.indexEntityGroup = null;
this.indexPrimaryKey = null;
this.projections = null;
this.consistencyLevel = com.xiaomi.infra.galaxy.sds.thrift.ConsistencyLevel.STRONG;
this.unique = false;
this.throughput = null;
this.slaveThroughput = null;
this.exceededThroughput = null;
this.exceededSlaveThroughput = null;
this.preSplits = 1;
}
/**
* 索引表的实体组键
* 可用于对索引表进行hash
*/
public EntityGroupSpec getIndexEntityGroup() {
return this.indexEntityGroup;
}
/**
* 索引表的实体组键
* 可用于对索引表进行hash
*/
public GlobalSecondaryIndexSpec setIndexEntityGroup(EntityGroupSpec indexEntityGroup) {
this.indexEntityGroup = indexEntityGroup;
return this;
}
public void unsetIndexEntityGroup() {
this.indexEntityGroup = null;
}
/** Returns true if field indexEntityGroup is set (has been assigned a value) and false otherwise */
public boolean isSetIndexEntityGroup() {
return this.indexEntityGroup != null;
}
public void setIndexEntityGroupIsSet(boolean value) {
if (!value) {
this.indexEntityGroup = null;
}
}
public int getIndexPrimaryKeySize() {
return (this.indexPrimaryKey == null) ? 0 : this.indexPrimaryKey.size();
}
public java.util.Iterator getIndexPrimaryKeyIterator() {
return (this.indexPrimaryKey == null) ? null : this.indexPrimaryKey.iterator();
}
public void addToIndexPrimaryKey(KeySpec elem) {
if (this.indexPrimaryKey == null) {
this.indexPrimaryKey = new ArrayList();
}
this.indexPrimaryKey.add(elem);
}
/**
* 索引表的主键
*/
public List getIndexPrimaryKey() {
return this.indexPrimaryKey;
}
/**
* 索引表的主键
*/
public GlobalSecondaryIndexSpec setIndexPrimaryKey(List indexPrimaryKey) {
this.indexPrimaryKey = indexPrimaryKey;
return this;
}
public void unsetIndexPrimaryKey() {
this.indexPrimaryKey = null;
}
/** Returns true if field indexPrimaryKey is set (has been assigned a value) and false otherwise */
public boolean isSetIndexPrimaryKey() {
return this.indexPrimaryKey != null;
}
public void setIndexPrimaryKeyIsSet(boolean value) {
if (!value) {
this.indexPrimaryKey = null;
}
}
public int getProjectionsSize() {
return (this.projections == null) ? 0 : this.projections.size();
}
public java.util.Iterator getProjectionsIterator() {
return (this.projections == null) ? null : this.projections.iterator();
}
public void addToProjections(String elem) {
if (this.projections == null) {
this.projections = new ArrayList();
}
this.projections.add(elem);
}
/**
* 投影的属性,全局二级索引不能读取非投影属性
*/
public List getProjections() {
return this.projections;
}
/**
* 投影的属性,全局二级索引不能读取非投影属性
*/
public GlobalSecondaryIndexSpec setProjections(List projections) {
this.projections = projections;
return this;
}
public void unsetProjections() {
this.projections = null;
}
/** Returns true if field projections is set (has been assigned a value) and false otherwise */
public boolean isSetProjections() {
return this.projections != null;
}
public void setProjectionsIsSet(boolean value) {
if (!value) {
this.projections = null;
}
}
/**
* 索引数据一致性级别
*
* @see ConsistencyLevel
*/
public ConsistencyLevel getConsistencyLevel() {
return this.consistencyLevel;
}
/**
* 索引数据一致性级别
*
* @see ConsistencyLevel
*/
public GlobalSecondaryIndexSpec setConsistencyLevel(ConsistencyLevel consistencyLevel) {
this.consistencyLevel = consistencyLevel;
return this;
}
public void unsetConsistencyLevel() {
this.consistencyLevel = null;
}
/** Returns true if field consistencyLevel is set (has been assigned a value) and false otherwise */
public boolean isSetConsistencyLevel() {
return this.consistencyLevel != null;
}
public void setConsistencyLevelIsSet(boolean value) {
if (!value) {
this.consistencyLevel = null;
}
}
/**
* 是否为唯一索引
*/
public boolean isUnique() {
return this.unique;
}
/**
* 是否为唯一索引
*/
public GlobalSecondaryIndexSpec setUnique(boolean unique) {
this.unique = unique;
setUniqueIsSet(true);
return this;
}
public void unsetUnique() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __UNIQUE_ISSET_ID);
}
/** Returns true if field unique is set (has been assigned a value) and false otherwise */
public boolean isSetUnique() {
return EncodingUtils.testBit(__isset_bitfield, __UNIQUE_ISSET_ID);
}
public void setUniqueIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __UNIQUE_ISSET_ID, value);
}
/**
* 索引表的吞吐量配额
*/
public ProvisionThroughput getThroughput() {
return this.throughput;
}
/**
* 索引表的吞吐量配额
*/
public GlobalSecondaryIndexSpec setThroughput(ProvisionThroughput throughput) {
this.throughput = throughput;
return this;
}
public void unsetThroughput() {
this.throughput = null;
}
/** Returns true if field throughput is set (has been assigned a value) and false otherwise */
public boolean isSetThroughput() {
return this.throughput != null;
}
public void setThroughputIsSet(boolean value) {
if (!value) {
this.throughput = null;
}
}
/**
* 索引表的备集群的吞吐量配额
*/
public ProvisionThroughput getSlaveThroughput() {
return this.slaveThroughput;
}
/**
* 索引表的备集群的吞吐量配额
*/
public GlobalSecondaryIndexSpec setSlaveThroughput(ProvisionThroughput slaveThroughput) {
this.slaveThroughput = slaveThroughput;
return this;
}
public void unsetSlaveThroughput() {
this.slaveThroughput = null;
}
/** Returns true if field slaveThroughput is set (has been assigned a value) and false otherwise */
public boolean isSetSlaveThroughput() {
return this.slaveThroughput != null;
}
public void setSlaveThroughputIsSet(boolean value) {
if (!value) {
this.slaveThroughput = null;
}
}
/**
* 索引表的最大超发吞吐量配额
*/
public ProvisionThroughput getExceededThroughput() {
return this.exceededThroughput;
}
/**
* 索引表的最大超发吞吐量配额
*/
public GlobalSecondaryIndexSpec setExceededThroughput(ProvisionThroughput exceededThroughput) {
this.exceededThroughput = exceededThroughput;
return this;
}
public void unsetExceededThroughput() {
this.exceededThroughput = null;
}
/** Returns true if field exceededThroughput is set (has been assigned a value) and false otherwise */
public boolean isSetExceededThroughput() {
return this.exceededThroughput != null;
}
public void setExceededThroughputIsSet(boolean value) {
if (!value) {
this.exceededThroughput = null;
}
}
/**
* 索引表的备集群的最大超发吞吐量配额
*/
public ProvisionThroughput getExceededSlaveThroughput() {
return this.exceededSlaveThroughput;
}
/**
* 索引表的备集群的最大超发吞吐量配额
*/
public GlobalSecondaryIndexSpec setExceededSlaveThroughput(ProvisionThroughput exceededSlaveThroughput) {
this.exceededSlaveThroughput = exceededSlaveThroughput;
return this;
}
public void unsetExceededSlaveThroughput() {
this.exceededSlaveThroughput = null;
}
/** Returns true if field exceededSlaveThroughput is set (has been assigned a value) and false otherwise */
public boolean isSetExceededSlaveThroughput() {
return this.exceededSlaveThroughput != null;
}
public void setExceededSlaveThroughputIsSet(boolean value) {
if (!value) {
this.exceededSlaveThroughput = null;
}
}
/**
* 表初始分片数目,仅支持Entity Group开启hash分布的表,且仅在建表时起作用
*/
public int getPreSplits() {
return this.preSplits;
}
/**
* 表初始分片数目,仅支持Entity Group开启hash分布的表,且仅在建表时起作用
*/
public GlobalSecondaryIndexSpec setPreSplits(int preSplits) {
this.preSplits = preSplits;
setPreSplitsIsSet(true);
return this;
}
public void unsetPreSplits() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __PRESPLITS_ISSET_ID);
}
/** Returns true if field preSplits is set (has been assigned a value) and false otherwise */
public boolean isSetPreSplits() {
return EncodingUtils.testBit(__isset_bitfield, __PRESPLITS_ISSET_ID);
}
public void setPreSplitsIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __PRESPLITS_ISSET_ID, value);
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case INDEX_ENTITY_GROUP:
if (value == null) {
unsetIndexEntityGroup();
} else {
setIndexEntityGroup((EntityGroupSpec)value);
}
break;
case INDEX_PRIMARY_KEY:
if (value == null) {
unsetIndexPrimaryKey();
} else {
setIndexPrimaryKey((List)value);
}
break;
case PROJECTIONS:
if (value == null) {
unsetProjections();
} else {
setProjections((List)value);
}
break;
case CONSISTENCY_LEVEL:
if (value == null) {
unsetConsistencyLevel();
} else {
setConsistencyLevel((ConsistencyLevel)value);
}
break;
case UNIQUE:
if (value == null) {
unsetUnique();
} else {
setUnique((Boolean)value);
}
break;
case THROUGHPUT:
if (value == null) {
unsetThroughput();
} else {
setThroughput((ProvisionThroughput)value);
}
break;
case SLAVE_THROUGHPUT:
if (value == null) {
unsetSlaveThroughput();
} else {
setSlaveThroughput((ProvisionThroughput)value);
}
break;
case EXCEEDED_THROUGHPUT:
if (value == null) {
unsetExceededThroughput();
} else {
setExceededThroughput((ProvisionThroughput)value);
}
break;
case EXCEEDED_SLAVE_THROUGHPUT:
if (value == null) {
unsetExceededSlaveThroughput();
} else {
setExceededSlaveThroughput((ProvisionThroughput)value);
}
break;
case PRE_SPLITS:
if (value == null) {
unsetPreSplits();
} else {
setPreSplits((Integer)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case INDEX_ENTITY_GROUP:
return getIndexEntityGroup();
case INDEX_PRIMARY_KEY:
return getIndexPrimaryKey();
case PROJECTIONS:
return getProjections();
case CONSISTENCY_LEVEL:
return getConsistencyLevel();
case UNIQUE:
return Boolean.valueOf(isUnique());
case THROUGHPUT:
return getThroughput();
case SLAVE_THROUGHPUT:
return getSlaveThroughput();
case EXCEEDED_THROUGHPUT:
return getExceededThroughput();
case EXCEEDED_SLAVE_THROUGHPUT:
return getExceededSlaveThroughput();
case PRE_SPLITS:
return Integer.valueOf(getPreSplits());
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case INDEX_ENTITY_GROUP:
return isSetIndexEntityGroup();
case INDEX_PRIMARY_KEY:
return isSetIndexPrimaryKey();
case PROJECTIONS:
return isSetProjections();
case CONSISTENCY_LEVEL:
return isSetConsistencyLevel();
case UNIQUE:
return isSetUnique();
case THROUGHPUT:
return isSetThroughput();
case SLAVE_THROUGHPUT:
return isSetSlaveThroughput();
case EXCEEDED_THROUGHPUT:
return isSetExceededThroughput();
case EXCEEDED_SLAVE_THROUGHPUT:
return isSetExceededSlaveThroughput();
case PRE_SPLITS:
return isSetPreSplits();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof GlobalSecondaryIndexSpec)
return this.equals((GlobalSecondaryIndexSpec)that);
return false;
}
public boolean equals(GlobalSecondaryIndexSpec that) {
if (that == null)
return false;
boolean this_present_indexEntityGroup = true && this.isSetIndexEntityGroup();
boolean that_present_indexEntityGroup = true && that.isSetIndexEntityGroup();
if (this_present_indexEntityGroup || that_present_indexEntityGroup) {
if (!(this_present_indexEntityGroup && that_present_indexEntityGroup))
return false;
if (!this.indexEntityGroup.equals(that.indexEntityGroup))
return false;
}
boolean this_present_indexPrimaryKey = true && this.isSetIndexPrimaryKey();
boolean that_present_indexPrimaryKey = true && that.isSetIndexPrimaryKey();
if (this_present_indexPrimaryKey || that_present_indexPrimaryKey) {
if (!(this_present_indexPrimaryKey && that_present_indexPrimaryKey))
return false;
if (!this.indexPrimaryKey.equals(that.indexPrimaryKey))
return false;
}
boolean this_present_projections = true && this.isSetProjections();
boolean that_present_projections = true && that.isSetProjections();
if (this_present_projections || that_present_projections) {
if (!(this_present_projections && that_present_projections))
return false;
if (!this.projections.equals(that.projections))
return false;
}
boolean this_present_consistencyLevel = true && this.isSetConsistencyLevel();
boolean that_present_consistencyLevel = true && that.isSetConsistencyLevel();
if (this_present_consistencyLevel || that_present_consistencyLevel) {
if (!(this_present_consistencyLevel && that_present_consistencyLevel))
return false;
if (!this.consistencyLevel.equals(that.consistencyLevel))
return false;
}
boolean this_present_unique = true && this.isSetUnique();
boolean that_present_unique = true && that.isSetUnique();
if (this_present_unique || that_present_unique) {
if (!(this_present_unique && that_present_unique))
return false;
if (this.unique != that.unique)
return false;
}
boolean this_present_throughput = true && this.isSetThroughput();
boolean that_present_throughput = true && that.isSetThroughput();
if (this_present_throughput || that_present_throughput) {
if (!(this_present_throughput && that_present_throughput))
return false;
if (!this.throughput.equals(that.throughput))
return false;
}
boolean this_present_slaveThroughput = true && this.isSetSlaveThroughput();
boolean that_present_slaveThroughput = true && that.isSetSlaveThroughput();
if (this_present_slaveThroughput || that_present_slaveThroughput) {
if (!(this_present_slaveThroughput && that_present_slaveThroughput))
return false;
if (!this.slaveThroughput.equals(that.slaveThroughput))
return false;
}
boolean this_present_exceededThroughput = true && this.isSetExceededThroughput();
boolean that_present_exceededThroughput = true && that.isSetExceededThroughput();
if (this_present_exceededThroughput || that_present_exceededThroughput) {
if (!(this_present_exceededThroughput && that_present_exceededThroughput))
return false;
if (!this.exceededThroughput.equals(that.exceededThroughput))
return false;
}
boolean this_present_exceededSlaveThroughput = true && this.isSetExceededSlaveThroughput();
boolean that_present_exceededSlaveThroughput = true && that.isSetExceededSlaveThroughput();
if (this_present_exceededSlaveThroughput || that_present_exceededSlaveThroughput) {
if (!(this_present_exceededSlaveThroughput && that_present_exceededSlaveThroughput))
return false;
if (!this.exceededSlaveThroughput.equals(that.exceededSlaveThroughput))
return false;
}
boolean this_present_preSplits = true && this.isSetPreSplits();
boolean that_present_preSplits = true && that.isSetPreSplits();
if (this_present_preSplits || that_present_preSplits) {
if (!(this_present_preSplits && that_present_preSplits))
return false;
if (this.preSplits != that.preSplits)
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy