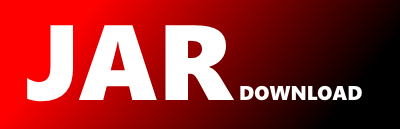
com.xiaomi.infra.galaxy.sds.thrift.QuotaInfo Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.2)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.xiaomi.infra.galaxy.sds.thrift;
import libthrift091.scheme.IScheme;
import libthrift091.scheme.SchemeFactory;
import libthrift091.scheme.StandardScheme;
import libthrift091.scheme.TupleScheme;
import libthrift091.protocol.TTupleProtocol;
import libthrift091.protocol.TProtocolException;
import libthrift091.EncodingUtils;
import libthrift091.TException;
import libthrift091.async.AsyncMethodCallback;
import libthrift091.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.2)", date = "2016-8-10")
public class QuotaInfo implements libthrift091.TBase, java.io.Serializable, Cloneable, Comparable {
private static final libthrift091.protocol.TStruct STRUCT_DESC = new libthrift091.protocol.TStruct("QuotaInfo");
private static final libthrift091.protocol.TField ACCOUNT_ID_FIELD_DESC = new libthrift091.protocol.TField("accountId", libthrift091.protocol.TType.STRING, (short)1);
private static final libthrift091.protocol.TField TABLE_NUM_FIELD_DESC = new libthrift091.protocol.TField("tableNum", libthrift091.protocol.TType.I32, (short)2);
private static final libthrift091.protocol.TField TABLE_NUM_USED_FIELD_DESC = new libthrift091.protocol.TField("tableNumUsed", libthrift091.protocol.TType.I32, (short)3);
private static final libthrift091.protocol.TField SPACE_FIELD_DESC = new libthrift091.protocol.TField("space", libthrift091.protocol.TType.I64, (short)4);
private static final libthrift091.protocol.TField SPACE_USED_FIELD_DESC = new libthrift091.protocol.TField("spaceUsed", libthrift091.protocol.TType.I64, (short)5);
private static final libthrift091.protocol.TField READ_CAPACITY_FIELD_DESC = new libthrift091.protocol.TField("readCapacity", libthrift091.protocol.TType.I64, (short)6);
private static final libthrift091.protocol.TField READ_CAPACITY_USED_FIELD_DESC = new libthrift091.protocol.TField("readCapacityUsed", libthrift091.protocol.TType.I64, (short)7);
private static final libthrift091.protocol.TField WRITE_CAPACITY_FIELD_DESC = new libthrift091.protocol.TField("writeCapacity", libthrift091.protocol.TType.I64, (short)8);
private static final libthrift091.protocol.TField WRITE_CAPACITY_USED_FIELD_DESC = new libthrift091.protocol.TField("writeCapacityUsed", libthrift091.protocol.TType.I64, (short)9);
private static final libthrift091.protocol.TField SLAVE_READ_CAPACITY_FIELD_DESC = new libthrift091.protocol.TField("slaveReadCapacity", libthrift091.protocol.TType.I64, (short)10);
private static final libthrift091.protocol.TField SLAVE_READ_CAPACITY_USED_FIELD_DESC = new libthrift091.protocol.TField("slaveReadCapacityUsed", libthrift091.protocol.TType.I64, (short)11);
private static final libthrift091.protocol.TField SLAVE_WRITE_CAPACITY_FIELD_DESC = new libthrift091.protocol.TField("slaveWriteCapacity", libthrift091.protocol.TType.I64, (short)12);
private static final libthrift091.protocol.TField SLAVE_WRITE_CAPACITY_USED_FIELD_DESC = new libthrift091.protocol.TField("slaveWriteCapacityUsed", libthrift091.protocol.TType.I64, (short)13);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new QuotaInfoStandardSchemeFactory());
schemes.put(TupleScheme.class, new QuotaInfoTupleSchemeFactory());
}
/**
* 用户AccountId
*/
public String accountId; // optional
/**
* 表数量限制
*/
public int tableNum; // optional
/**
* 已存在表数量
*/
public int tableNumUsed; // optional
/**
* 用户总的空间quota限制
*/
public long space; // optional
/**
* 已使用空间quota
*/
public long spaceUsed; // optional
/**
* 用户总的读quota限制
*/
public long readCapacity; // optional
/**
* 已使用读quota
*/
public long readCapacityUsed; // optional
/**
* 用户总的写quota限制
*/
public long writeCapacity; // optional
/**
* 已使用写quota
*/
public long writeCapacityUsed; // optional
/**
* 用户总的备集群读quota限制
*/
public long slaveReadCapacity; // optional
/**
* 已使用备集群读quota
*/
public long slaveReadCapacityUsed; // optional
/**
* 用户总的备集群写quota限制
*/
public long slaveWriteCapacity; // optional
/**
* 已使用备集群写quota
*/
public long slaveWriteCapacityUsed; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements libthrift091.TFieldIdEnum {
/**
* 用户AccountId
*/
ACCOUNT_ID((short)1, "accountId"),
/**
* 表数量限制
*/
TABLE_NUM((short)2, "tableNum"),
/**
* 已存在表数量
*/
TABLE_NUM_USED((short)3, "tableNumUsed"),
/**
* 用户总的空间quota限制
*/
SPACE((short)4, "space"),
/**
* 已使用空间quota
*/
SPACE_USED((short)5, "spaceUsed"),
/**
* 用户总的读quota限制
*/
READ_CAPACITY((short)6, "readCapacity"),
/**
* 已使用读quota
*/
READ_CAPACITY_USED((short)7, "readCapacityUsed"),
/**
* 用户总的写quota限制
*/
WRITE_CAPACITY((short)8, "writeCapacity"),
/**
* 已使用写quota
*/
WRITE_CAPACITY_USED((short)9, "writeCapacityUsed"),
/**
* 用户总的备集群读quota限制
*/
SLAVE_READ_CAPACITY((short)10, "slaveReadCapacity"),
/**
* 已使用备集群读quota
*/
SLAVE_READ_CAPACITY_USED((short)11, "slaveReadCapacityUsed"),
/**
* 用户总的备集群写quota限制
*/
SLAVE_WRITE_CAPACITY((short)12, "slaveWriteCapacity"),
/**
* 已使用备集群写quota
*/
SLAVE_WRITE_CAPACITY_USED((short)13, "slaveWriteCapacityUsed");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // ACCOUNT_ID
return ACCOUNT_ID;
case 2: // TABLE_NUM
return TABLE_NUM;
case 3: // TABLE_NUM_USED
return TABLE_NUM_USED;
case 4: // SPACE
return SPACE;
case 5: // SPACE_USED
return SPACE_USED;
case 6: // READ_CAPACITY
return READ_CAPACITY;
case 7: // READ_CAPACITY_USED
return READ_CAPACITY_USED;
case 8: // WRITE_CAPACITY
return WRITE_CAPACITY;
case 9: // WRITE_CAPACITY_USED
return WRITE_CAPACITY_USED;
case 10: // SLAVE_READ_CAPACITY
return SLAVE_READ_CAPACITY;
case 11: // SLAVE_READ_CAPACITY_USED
return SLAVE_READ_CAPACITY_USED;
case 12: // SLAVE_WRITE_CAPACITY
return SLAVE_WRITE_CAPACITY;
case 13: // SLAVE_WRITE_CAPACITY_USED
return SLAVE_WRITE_CAPACITY_USED;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __TABLENUM_ISSET_ID = 0;
private static final int __TABLENUMUSED_ISSET_ID = 1;
private static final int __SPACE_ISSET_ID = 2;
private static final int __SPACEUSED_ISSET_ID = 3;
private static final int __READCAPACITY_ISSET_ID = 4;
private static final int __READCAPACITYUSED_ISSET_ID = 5;
private static final int __WRITECAPACITY_ISSET_ID = 6;
private static final int __WRITECAPACITYUSED_ISSET_ID = 7;
private static final int __SLAVEREADCAPACITY_ISSET_ID = 8;
private static final int __SLAVEREADCAPACITYUSED_ISSET_ID = 9;
private static final int __SLAVEWRITECAPACITY_ISSET_ID = 10;
private static final int __SLAVEWRITECAPACITYUSED_ISSET_ID = 11;
private short __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.ACCOUNT_ID,_Fields.TABLE_NUM,_Fields.TABLE_NUM_USED,_Fields.SPACE,_Fields.SPACE_USED,_Fields.READ_CAPACITY,_Fields.READ_CAPACITY_USED,_Fields.WRITE_CAPACITY,_Fields.WRITE_CAPACITY_USED,_Fields.SLAVE_READ_CAPACITY,_Fields.SLAVE_READ_CAPACITY_USED,_Fields.SLAVE_WRITE_CAPACITY,_Fields.SLAVE_WRITE_CAPACITY_USED};
public static final Map<_Fields, libthrift091.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, libthrift091.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, libthrift091.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.ACCOUNT_ID, new libthrift091.meta_data.FieldMetaData("accountId", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.STRING)));
tmpMap.put(_Fields.TABLE_NUM, new libthrift091.meta_data.FieldMetaData("tableNum", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I32)));
tmpMap.put(_Fields.TABLE_NUM_USED, new libthrift091.meta_data.FieldMetaData("tableNumUsed", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I32)));
tmpMap.put(_Fields.SPACE, new libthrift091.meta_data.FieldMetaData("space", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I64)));
tmpMap.put(_Fields.SPACE_USED, new libthrift091.meta_data.FieldMetaData("spaceUsed", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I64)));
tmpMap.put(_Fields.READ_CAPACITY, new libthrift091.meta_data.FieldMetaData("readCapacity", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I64)));
tmpMap.put(_Fields.READ_CAPACITY_USED, new libthrift091.meta_data.FieldMetaData("readCapacityUsed", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I64)));
tmpMap.put(_Fields.WRITE_CAPACITY, new libthrift091.meta_data.FieldMetaData("writeCapacity", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I64)));
tmpMap.put(_Fields.WRITE_CAPACITY_USED, new libthrift091.meta_data.FieldMetaData("writeCapacityUsed", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I64)));
tmpMap.put(_Fields.SLAVE_READ_CAPACITY, new libthrift091.meta_data.FieldMetaData("slaveReadCapacity", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I64)));
tmpMap.put(_Fields.SLAVE_READ_CAPACITY_USED, new libthrift091.meta_data.FieldMetaData("slaveReadCapacityUsed", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I64)));
tmpMap.put(_Fields.SLAVE_WRITE_CAPACITY, new libthrift091.meta_data.FieldMetaData("slaveWriteCapacity", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I64)));
tmpMap.put(_Fields.SLAVE_WRITE_CAPACITY_USED, new libthrift091.meta_data.FieldMetaData("slaveWriteCapacityUsed", libthrift091.TFieldRequirementType.OPTIONAL,
new libthrift091.meta_data.FieldValueMetaData(libthrift091.protocol.TType.I64)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
libthrift091.meta_data.FieldMetaData.addStructMetaDataMap(QuotaInfo.class, metaDataMap);
}
public QuotaInfo() {
}
/**
* Performs a deep copy on other.
*/
public QuotaInfo(QuotaInfo other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetAccountId()) {
this.accountId = other.accountId;
}
this.tableNum = other.tableNum;
this.tableNumUsed = other.tableNumUsed;
this.space = other.space;
this.spaceUsed = other.spaceUsed;
this.readCapacity = other.readCapacity;
this.readCapacityUsed = other.readCapacityUsed;
this.writeCapacity = other.writeCapacity;
this.writeCapacityUsed = other.writeCapacityUsed;
this.slaveReadCapacity = other.slaveReadCapacity;
this.slaveReadCapacityUsed = other.slaveReadCapacityUsed;
this.slaveWriteCapacity = other.slaveWriteCapacity;
this.slaveWriteCapacityUsed = other.slaveWriteCapacityUsed;
}
public QuotaInfo deepCopy() {
return new QuotaInfo(this);
}
@Override
public void clear() {
this.accountId = null;
setTableNumIsSet(false);
this.tableNum = 0;
setTableNumUsedIsSet(false);
this.tableNumUsed = 0;
setSpaceIsSet(false);
this.space = 0;
setSpaceUsedIsSet(false);
this.spaceUsed = 0;
setReadCapacityIsSet(false);
this.readCapacity = 0;
setReadCapacityUsedIsSet(false);
this.readCapacityUsed = 0;
setWriteCapacityIsSet(false);
this.writeCapacity = 0;
setWriteCapacityUsedIsSet(false);
this.writeCapacityUsed = 0;
setSlaveReadCapacityIsSet(false);
this.slaveReadCapacity = 0;
setSlaveReadCapacityUsedIsSet(false);
this.slaveReadCapacityUsed = 0;
setSlaveWriteCapacityIsSet(false);
this.slaveWriteCapacity = 0;
setSlaveWriteCapacityUsedIsSet(false);
this.slaveWriteCapacityUsed = 0;
}
/**
* 用户AccountId
*/
public String getAccountId() {
return this.accountId;
}
/**
* 用户AccountId
*/
public QuotaInfo setAccountId(String accountId) {
this.accountId = accountId;
return this;
}
public void unsetAccountId() {
this.accountId = null;
}
/** Returns true if field accountId is set (has been assigned a value) and false otherwise */
public boolean isSetAccountId() {
return this.accountId != null;
}
public void setAccountIdIsSet(boolean value) {
if (!value) {
this.accountId = null;
}
}
/**
* 表数量限制
*/
public int getTableNum() {
return this.tableNum;
}
/**
* 表数量限制
*/
public QuotaInfo setTableNum(int tableNum) {
this.tableNum = tableNum;
setTableNumIsSet(true);
return this;
}
public void unsetTableNum() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __TABLENUM_ISSET_ID);
}
/** Returns true if field tableNum is set (has been assigned a value) and false otherwise */
public boolean isSetTableNum() {
return EncodingUtils.testBit(__isset_bitfield, __TABLENUM_ISSET_ID);
}
public void setTableNumIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __TABLENUM_ISSET_ID, value);
}
/**
* 已存在表数量
*/
public int getTableNumUsed() {
return this.tableNumUsed;
}
/**
* 已存在表数量
*/
public QuotaInfo setTableNumUsed(int tableNumUsed) {
this.tableNumUsed = tableNumUsed;
setTableNumUsedIsSet(true);
return this;
}
public void unsetTableNumUsed() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __TABLENUMUSED_ISSET_ID);
}
/** Returns true if field tableNumUsed is set (has been assigned a value) and false otherwise */
public boolean isSetTableNumUsed() {
return EncodingUtils.testBit(__isset_bitfield, __TABLENUMUSED_ISSET_ID);
}
public void setTableNumUsedIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __TABLENUMUSED_ISSET_ID, value);
}
/**
* 用户总的空间quota限制
*/
public long getSpace() {
return this.space;
}
/**
* 用户总的空间quota限制
*/
public QuotaInfo setSpace(long space) {
this.space = space;
setSpaceIsSet(true);
return this;
}
public void unsetSpace() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __SPACE_ISSET_ID);
}
/** Returns true if field space is set (has been assigned a value) and false otherwise */
public boolean isSetSpace() {
return EncodingUtils.testBit(__isset_bitfield, __SPACE_ISSET_ID);
}
public void setSpaceIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __SPACE_ISSET_ID, value);
}
/**
* 已使用空间quota
*/
public long getSpaceUsed() {
return this.spaceUsed;
}
/**
* 已使用空间quota
*/
public QuotaInfo setSpaceUsed(long spaceUsed) {
this.spaceUsed = spaceUsed;
setSpaceUsedIsSet(true);
return this;
}
public void unsetSpaceUsed() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __SPACEUSED_ISSET_ID);
}
/** Returns true if field spaceUsed is set (has been assigned a value) and false otherwise */
public boolean isSetSpaceUsed() {
return EncodingUtils.testBit(__isset_bitfield, __SPACEUSED_ISSET_ID);
}
public void setSpaceUsedIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __SPACEUSED_ISSET_ID, value);
}
/**
* 用户总的读quota限制
*/
public long getReadCapacity() {
return this.readCapacity;
}
/**
* 用户总的读quota限制
*/
public QuotaInfo setReadCapacity(long readCapacity) {
this.readCapacity = readCapacity;
setReadCapacityIsSet(true);
return this;
}
public void unsetReadCapacity() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __READCAPACITY_ISSET_ID);
}
/** Returns true if field readCapacity is set (has been assigned a value) and false otherwise */
public boolean isSetReadCapacity() {
return EncodingUtils.testBit(__isset_bitfield, __READCAPACITY_ISSET_ID);
}
public void setReadCapacityIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __READCAPACITY_ISSET_ID, value);
}
/**
* 已使用读quota
*/
public long getReadCapacityUsed() {
return this.readCapacityUsed;
}
/**
* 已使用读quota
*/
public QuotaInfo setReadCapacityUsed(long readCapacityUsed) {
this.readCapacityUsed = readCapacityUsed;
setReadCapacityUsedIsSet(true);
return this;
}
public void unsetReadCapacityUsed() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __READCAPACITYUSED_ISSET_ID);
}
/** Returns true if field readCapacityUsed is set (has been assigned a value) and false otherwise */
public boolean isSetReadCapacityUsed() {
return EncodingUtils.testBit(__isset_bitfield, __READCAPACITYUSED_ISSET_ID);
}
public void setReadCapacityUsedIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __READCAPACITYUSED_ISSET_ID, value);
}
/**
* 用户总的写quota限制
*/
public long getWriteCapacity() {
return this.writeCapacity;
}
/**
* 用户总的写quota限制
*/
public QuotaInfo setWriteCapacity(long writeCapacity) {
this.writeCapacity = writeCapacity;
setWriteCapacityIsSet(true);
return this;
}
public void unsetWriteCapacity() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __WRITECAPACITY_ISSET_ID);
}
/** Returns true if field writeCapacity is set (has been assigned a value) and false otherwise */
public boolean isSetWriteCapacity() {
return EncodingUtils.testBit(__isset_bitfield, __WRITECAPACITY_ISSET_ID);
}
public void setWriteCapacityIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __WRITECAPACITY_ISSET_ID, value);
}
/**
* 已使用写quota
*/
public long getWriteCapacityUsed() {
return this.writeCapacityUsed;
}
/**
* 已使用写quota
*/
public QuotaInfo setWriteCapacityUsed(long writeCapacityUsed) {
this.writeCapacityUsed = writeCapacityUsed;
setWriteCapacityUsedIsSet(true);
return this;
}
public void unsetWriteCapacityUsed() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __WRITECAPACITYUSED_ISSET_ID);
}
/** Returns true if field writeCapacityUsed is set (has been assigned a value) and false otherwise */
public boolean isSetWriteCapacityUsed() {
return EncodingUtils.testBit(__isset_bitfield, __WRITECAPACITYUSED_ISSET_ID);
}
public void setWriteCapacityUsedIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __WRITECAPACITYUSED_ISSET_ID, value);
}
/**
* 用户总的备集群读quota限制
*/
public long getSlaveReadCapacity() {
return this.slaveReadCapacity;
}
/**
* 用户总的备集群读quota限制
*/
public QuotaInfo setSlaveReadCapacity(long slaveReadCapacity) {
this.slaveReadCapacity = slaveReadCapacity;
setSlaveReadCapacityIsSet(true);
return this;
}
public void unsetSlaveReadCapacity() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __SLAVEREADCAPACITY_ISSET_ID);
}
/** Returns true if field slaveReadCapacity is set (has been assigned a value) and false otherwise */
public boolean isSetSlaveReadCapacity() {
return EncodingUtils.testBit(__isset_bitfield, __SLAVEREADCAPACITY_ISSET_ID);
}
public void setSlaveReadCapacityIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __SLAVEREADCAPACITY_ISSET_ID, value);
}
/**
* 已使用备集群读quota
*/
public long getSlaveReadCapacityUsed() {
return this.slaveReadCapacityUsed;
}
/**
* 已使用备集群读quota
*/
public QuotaInfo setSlaveReadCapacityUsed(long slaveReadCapacityUsed) {
this.slaveReadCapacityUsed = slaveReadCapacityUsed;
setSlaveReadCapacityUsedIsSet(true);
return this;
}
public void unsetSlaveReadCapacityUsed() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __SLAVEREADCAPACITYUSED_ISSET_ID);
}
/** Returns true if field slaveReadCapacityUsed is set (has been assigned a value) and false otherwise */
public boolean isSetSlaveReadCapacityUsed() {
return EncodingUtils.testBit(__isset_bitfield, __SLAVEREADCAPACITYUSED_ISSET_ID);
}
public void setSlaveReadCapacityUsedIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __SLAVEREADCAPACITYUSED_ISSET_ID, value);
}
/**
* 用户总的备集群写quota限制
*/
public long getSlaveWriteCapacity() {
return this.slaveWriteCapacity;
}
/**
* 用户总的备集群写quota限制
*/
public QuotaInfo setSlaveWriteCapacity(long slaveWriteCapacity) {
this.slaveWriteCapacity = slaveWriteCapacity;
setSlaveWriteCapacityIsSet(true);
return this;
}
public void unsetSlaveWriteCapacity() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __SLAVEWRITECAPACITY_ISSET_ID);
}
/** Returns true if field slaveWriteCapacity is set (has been assigned a value) and false otherwise */
public boolean isSetSlaveWriteCapacity() {
return EncodingUtils.testBit(__isset_bitfield, __SLAVEWRITECAPACITY_ISSET_ID);
}
public void setSlaveWriteCapacityIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __SLAVEWRITECAPACITY_ISSET_ID, value);
}
/**
* 已使用备集群写quota
*/
public long getSlaveWriteCapacityUsed() {
return this.slaveWriteCapacityUsed;
}
/**
* 已使用备集群写quota
*/
public QuotaInfo setSlaveWriteCapacityUsed(long slaveWriteCapacityUsed) {
this.slaveWriteCapacityUsed = slaveWriteCapacityUsed;
setSlaveWriteCapacityUsedIsSet(true);
return this;
}
public void unsetSlaveWriteCapacityUsed() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __SLAVEWRITECAPACITYUSED_ISSET_ID);
}
/** Returns true if field slaveWriteCapacityUsed is set (has been assigned a value) and false otherwise */
public boolean isSetSlaveWriteCapacityUsed() {
return EncodingUtils.testBit(__isset_bitfield, __SLAVEWRITECAPACITYUSED_ISSET_ID);
}
public void setSlaveWriteCapacityUsedIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __SLAVEWRITECAPACITYUSED_ISSET_ID, value);
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case ACCOUNT_ID:
if (value == null) {
unsetAccountId();
} else {
setAccountId((String)value);
}
break;
case TABLE_NUM:
if (value == null) {
unsetTableNum();
} else {
setTableNum((Integer)value);
}
break;
case TABLE_NUM_USED:
if (value == null) {
unsetTableNumUsed();
} else {
setTableNumUsed((Integer)value);
}
break;
case SPACE:
if (value == null) {
unsetSpace();
} else {
setSpace((Long)value);
}
break;
case SPACE_USED:
if (value == null) {
unsetSpaceUsed();
} else {
setSpaceUsed((Long)value);
}
break;
case READ_CAPACITY:
if (value == null) {
unsetReadCapacity();
} else {
setReadCapacity((Long)value);
}
break;
case READ_CAPACITY_USED:
if (value == null) {
unsetReadCapacityUsed();
} else {
setReadCapacityUsed((Long)value);
}
break;
case WRITE_CAPACITY:
if (value == null) {
unsetWriteCapacity();
} else {
setWriteCapacity((Long)value);
}
break;
case WRITE_CAPACITY_USED:
if (value == null) {
unsetWriteCapacityUsed();
} else {
setWriteCapacityUsed((Long)value);
}
break;
case SLAVE_READ_CAPACITY:
if (value == null) {
unsetSlaveReadCapacity();
} else {
setSlaveReadCapacity((Long)value);
}
break;
case SLAVE_READ_CAPACITY_USED:
if (value == null) {
unsetSlaveReadCapacityUsed();
} else {
setSlaveReadCapacityUsed((Long)value);
}
break;
case SLAVE_WRITE_CAPACITY:
if (value == null) {
unsetSlaveWriteCapacity();
} else {
setSlaveWriteCapacity((Long)value);
}
break;
case SLAVE_WRITE_CAPACITY_USED:
if (value == null) {
unsetSlaveWriteCapacityUsed();
} else {
setSlaveWriteCapacityUsed((Long)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case ACCOUNT_ID:
return getAccountId();
case TABLE_NUM:
return Integer.valueOf(getTableNum());
case TABLE_NUM_USED:
return Integer.valueOf(getTableNumUsed());
case SPACE:
return Long.valueOf(getSpace());
case SPACE_USED:
return Long.valueOf(getSpaceUsed());
case READ_CAPACITY:
return Long.valueOf(getReadCapacity());
case READ_CAPACITY_USED:
return Long.valueOf(getReadCapacityUsed());
case WRITE_CAPACITY:
return Long.valueOf(getWriteCapacity());
case WRITE_CAPACITY_USED:
return Long.valueOf(getWriteCapacityUsed());
case SLAVE_READ_CAPACITY:
return Long.valueOf(getSlaveReadCapacity());
case SLAVE_READ_CAPACITY_USED:
return Long.valueOf(getSlaveReadCapacityUsed());
case SLAVE_WRITE_CAPACITY:
return Long.valueOf(getSlaveWriteCapacity());
case SLAVE_WRITE_CAPACITY_USED:
return Long.valueOf(getSlaveWriteCapacityUsed());
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case ACCOUNT_ID:
return isSetAccountId();
case TABLE_NUM:
return isSetTableNum();
case TABLE_NUM_USED:
return isSetTableNumUsed();
case SPACE:
return isSetSpace();
case SPACE_USED:
return isSetSpaceUsed();
case READ_CAPACITY:
return isSetReadCapacity();
case READ_CAPACITY_USED:
return isSetReadCapacityUsed();
case WRITE_CAPACITY:
return isSetWriteCapacity();
case WRITE_CAPACITY_USED:
return isSetWriteCapacityUsed();
case SLAVE_READ_CAPACITY:
return isSetSlaveReadCapacity();
case SLAVE_READ_CAPACITY_USED:
return isSetSlaveReadCapacityUsed();
case SLAVE_WRITE_CAPACITY:
return isSetSlaveWriteCapacity();
case SLAVE_WRITE_CAPACITY_USED:
return isSetSlaveWriteCapacityUsed();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof QuotaInfo)
return this.equals((QuotaInfo)that);
return false;
}
public boolean equals(QuotaInfo that) {
if (that == null)
return false;
boolean this_present_accountId = true && this.isSetAccountId();
boolean that_present_accountId = true && that.isSetAccountId();
if (this_present_accountId || that_present_accountId) {
if (!(this_present_accountId && that_present_accountId))
return false;
if (!this.accountId.equals(that.accountId))
return false;
}
boolean this_present_tableNum = true && this.isSetTableNum();
boolean that_present_tableNum = true && that.isSetTableNum();
if (this_present_tableNum || that_present_tableNum) {
if (!(this_present_tableNum && that_present_tableNum))
return false;
if (this.tableNum != that.tableNum)
return false;
}
boolean this_present_tableNumUsed = true && this.isSetTableNumUsed();
boolean that_present_tableNumUsed = true && that.isSetTableNumUsed();
if (this_present_tableNumUsed || that_present_tableNumUsed) {
if (!(this_present_tableNumUsed && that_present_tableNumUsed))
return false;
if (this.tableNumUsed != that.tableNumUsed)
return false;
}
boolean this_present_space = true && this.isSetSpace();
boolean that_present_space = true && that.isSetSpace();
if (this_present_space || that_present_space) {
if (!(this_present_space && that_present_space))
return false;
if (this.space != that.space)
return false;
}
boolean this_present_spaceUsed = true && this.isSetSpaceUsed();
boolean that_present_spaceUsed = true && that.isSetSpaceUsed();
if (this_present_spaceUsed || that_present_spaceUsed) {
if (!(this_present_spaceUsed && that_present_spaceUsed))
return false;
if (this.spaceUsed != that.spaceUsed)
return false;
}
boolean this_present_readCapacity = true && this.isSetReadCapacity();
boolean that_present_readCapacity = true && that.isSetReadCapacity();
if (this_present_readCapacity || that_present_readCapacity) {
if (!(this_present_readCapacity && that_present_readCapacity))
return false;
if (this.readCapacity != that.readCapacity)
return false;
}
boolean this_present_readCapacityUsed = true && this.isSetReadCapacityUsed();
boolean that_present_readCapacityUsed = true && that.isSetReadCapacityUsed();
if (this_present_readCapacityUsed || that_present_readCapacityUsed) {
if (!(this_present_readCapacityUsed && that_present_readCapacityUsed))
return false;
if (this.readCapacityUsed != that.readCapacityUsed)
return false;
}
boolean this_present_writeCapacity = true && this.isSetWriteCapacity();
boolean that_present_writeCapacity = true && that.isSetWriteCapacity();
if (this_present_writeCapacity || that_present_writeCapacity) {
if (!(this_present_writeCapacity && that_present_writeCapacity))
return false;
if (this.writeCapacity != that.writeCapacity)
return false;
}
boolean this_present_writeCapacityUsed = true && this.isSetWriteCapacityUsed();
boolean that_present_writeCapacityUsed = true && that.isSetWriteCapacityUsed();
if (this_present_writeCapacityUsed || that_present_writeCapacityUsed) {
if (!(this_present_writeCapacityUsed && that_present_writeCapacityUsed))
return false;
if (this.writeCapacityUsed != that.writeCapacityUsed)
return false;
}
boolean this_present_slaveReadCapacity = true && this.isSetSlaveReadCapacity();
boolean that_present_slaveReadCapacity = true && that.isSetSlaveReadCapacity();
if (this_present_slaveReadCapacity || that_present_slaveReadCapacity) {
if (!(this_present_slaveReadCapacity && that_present_slaveReadCapacity))
return false;
if (this.slaveReadCapacity != that.slaveReadCapacity)
return false;
}
boolean this_present_slaveReadCapacityUsed = true && this.isSetSlaveReadCapacityUsed();
boolean that_present_slaveReadCapacityUsed = true && that.isSetSlaveReadCapacityUsed();
if (this_present_slaveReadCapacityUsed || that_present_slaveReadCapacityUsed) {
if (!(this_present_slaveReadCapacityUsed && that_present_slaveReadCapacityUsed))
return false;
if (this.slaveReadCapacityUsed != that.slaveReadCapacityUsed)
return false;
}
boolean this_present_slaveWriteCapacity = true && this.isSetSlaveWriteCapacity();
boolean that_present_slaveWriteCapacity = true && that.isSetSlaveWriteCapacity();
if (this_present_slaveWriteCapacity || that_present_slaveWriteCapacity) {
if (!(this_present_slaveWriteCapacity && that_present_slaveWriteCapacity))
return false;
if (this.slaveWriteCapacity != that.slaveWriteCapacity)
return false;
}
boolean this_present_slaveWriteCapacityUsed = true && this.isSetSlaveWriteCapacityUsed();
boolean that_present_slaveWriteCapacityUsed = true && that.isSetSlaveWriteCapacityUsed();
if (this_present_slaveWriteCapacityUsed || that_present_slaveWriteCapacityUsed) {
if (!(this_present_slaveWriteCapacityUsed && that_present_slaveWriteCapacityUsed))
return false;
if (this.slaveWriteCapacityUsed != that.slaveWriteCapacityUsed)
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy