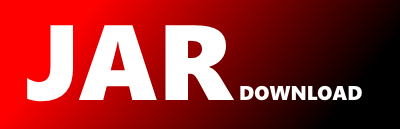
com.xiongyingqi.util.CollectionHelper Maven / Gradle / Ivy
/**
* YIXUN_2.0
*/
package com.xiongyingqi.util;
import java.util.*;
/**
* @author 瑛琪 xiongyingqi.com
* @version 2013-8-22 下午3:50:35
*/
public class CollectionHelper {
public static boolean notNullAndHasSize(Collection> collection) {
return collection != null && collection.size() > 0;
}
public static Collection checkOrInitHashSet(Collection collection) {
if (collection == null) {
collection = new HashSet();
}
return collection;
}
public static Collection checkOrInitLinkedHashSet(Collection collection) {
if (collection == null) {
collection = new LinkedHashSet();
}
return collection;
}
public static Map checkOrInitHashMap(Map map) {
if (map == null) {
map = new HashMap();
}
return map;
}
public static Map checkOrInitLinkedHashMap(Map map) {
if (map == null) {
map = new LinkedHashMap();
}
return map;
}
public static Collection checkOrInitArrayList(Collection collection) {
if (collection == null) {
collection = new ArrayList();
}
return collection;
}
public static Collection checkOrInitLinkedList(Collection collection) {
if (collection == null) {
collection = new LinkedList();
}
return collection;
}
public static void main(String[] args) {
Object a = null;
a = checkOrInitHashSet((Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy