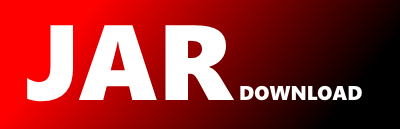
com.xiongyingqi.util.comparator.StringComparator Maven / Gradle / Ivy
package com.xiongyingqi.util.comparator;
import java.util.Comparator;
/**
* Created by 瑛琪xiongyingqi.com on 2014/5/4 0004.
*/
public class StringComparator implements Comparator {
/**
* Compares its two arguments for order. Returns a negative integer,
* zero, or a positive integer as the first argument is less than, equal
* to, or greater than the second.
*
* In the foregoing description, the notation
* sgn(expression) designates the mathematical
* signum function, which is defined to return one of -1,
* 0, or 1 according to whether the value of
* expression is negative, zero or positive.
*
* The implementor must ensure that sgn(compare(x, y)) ==
* -sgn(compare(y, x)) for all x and y. (This
* implies that compare(x, y) must throw an exception if and only
* if compare(y, x) throws an exception.)
*
* The implementor must also ensure that the relation is transitive:
* ((compare(x, y)>0) && (compare(y, z)>0)) implies
* compare(x, z)>0.
*
* Finally, the implementor must ensure that compare(x, y)==0
* implies that sgn(compare(x, z))==sgn(compare(y, z)) for all
* z.
*
* It is generally the case, but not strictly required that
* (compare(x, y)==0) == (x.equals(y)). Generally speaking,
* any comparator that violates this condition should clearly indicate
* this fact. The recommended language is "Note: this comparator
* imposes orderings that are inconsistent with equals."
*
* @param o1 the first object to be compared.
* @param o2 the second object to be compared.
* @return a negative integer, zero, or a positive integer as the
* first argument is less than, equal to, or greater than the
* second.
* @throws NullPointerException if an argument is null and this
* comparator does not permit null arguments
* @throws ClassCastException if the arguments' types prevent them from
* being compared by this comparator.
*/
@Override
public int compare(String o1, String o2) {
if (o1 == null || o2 == null) {
throw new NullPointerException();
}
if (o1.getClass() != o2.getClass()) {
throw new ClassCastException();
}
if (o1.length() == o2.length()) {
if (o1.hashCode() < o2.hashCode()) {
return -1;
} else if (o1.hashCode() > o2.hashCode()) {
return 1;
}
if (o1.hashCode() == o2.hashCode()) {
return 0;
}
}
char[] chars1 = o1.toCharArray();
char[] chars2 = o2.toCharArray();
int c = 0;
int minLength = (chars1.length < chars2.length) ? chars1.length : chars2.length;
for (int i = 0; i < minLength; i++) {
if (chars1[i] != chars2[i]) {
if (chars1[i] < chars2[i]) {
c = -1;
} else {
c = 1;
}
break;
}
}
if (c == 0) {
c = (chars1.length < chars2.length) ? -1 : 1;
}
return c;
}
/**
* Indicates whether some other object is "equal to" this
* comparator. This method must obey the general contract of
* {@link Object#equals(Object)}. Additionally, this method can return
* true only if the specified object is also a comparator
* and it imposes the same ordering as this comparator. Thus,
* comp1.equals(comp2)
implies that sgn(comp1.compare(o1,
* o2))==sgn(comp2.compare(o1, o2)) for every object reference
* o1 and o2.
*
* Note that it is always safe not to override
* Object.equals(Object). However, overriding this method may,
* in some cases, improve performance by allowing programs to determine
* that two distinct comparators impose the same order.
*
* @param obj the reference object with which to compare.
* @return true
only if the specified object is also
* a comparator and it imposes the same ordering as this
* comparator.
* @see Object#equals(Object)
* @see Object#hashCode()
*/
@Override
public boolean equals(Object obj) {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy