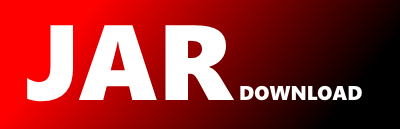
info.monitorenter.util.StringUtil Maven / Gradle / Ivy
/*
* StringUtil.java, helpers for strings.
* Copyright (C) 2001 Achim Westermann
*
* ***** BEGIN LICENSE BLOCK *****
* Version: MPL 1.1/GPL 2.0/LGPL 2.1
*
* The contents of this collection are subject to the Mozilla Public License Version
* 1.1 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License
* for the specific language governing rights and limitations under the
* License.
*
* The Original Code is the cpDetector code in [sub] packages info.monitorenter and
* cpdetector.
*
* The Initial Developer of the Original Code is
* Achim Westermann .
*
* Portions created by the Initial Developer are Copyright (c) 2007
* the Initial Developer. All Rights Reserved.
*
* Contributor(s):
*
* Alternatively, the contents of this file may be used under the terms of
* either the GNU General Public License Version 2 or later (the "GPL"), or
* the GNU Lesser General Public License Version 2.1 or later (the "LGPL"),
* in which case the provisions of the GPL or the LGPL are applicable instead
* of those above. If you wish to allow use of your version of this file only
* under the terms of either the GPL or the LGPL, and not to allow others to
* use your version of this file under the terms of the MPL, indicate your
* decision by deleting the provisions above and replace them with the notice
* and other provisions required by the GPL or the LGPL. If you do not delete
* the provisions above, a recipient may use your version of this file under
* the terms of any one of the MPL, the GPL or the LGPL.
*
* ***** END LICENSE BLOCK ***** *
*
* If you modify or optimize the code in a useful way please let me know.
* [email protected] *
*/package info.monitorenter.util;
import java.lang.reflect.Array;
import java.util.List;
import java.util.Map;
/**
* Nice static helpers for working with Strings.
*
* Maybe not always the fastest solution to call in here, but working. Also
* usable for seeing examples and cutting code for manual inlining.
*
*
* @author [email protected]
*
* @version $Revision: 1.3 $
*/
public final class StringUtil {
/** Singleton instance. */
private static StringUtil instance = null;
/**
* Appends the given amount of spaces to the String.
*
*
* Not intended for big append -operations because in a loop alway just one
* space is added.
*
*
* @param s
* the base String to append spaces to.
*
* @param count
* the amount of spaces to append.
*
* @return a String consisting of s and count trailing whitespaces.
*/
public static final String appendSpaces(final String s, final int count) {
StringBuffer tmp = new StringBuffer(s);
for (int i = 0; i < count; i++) {
tmp.append(" ");
}
return tmp.toString();
}
/**
* If the given Object is no Array, it's toString - method is invoked.
* Primitive type - Arrays and Object - Arrays are introspected using
* java.lang.reflect.Array. Convention for creation fo String -
* representation:
*
* @see info.monitorenter.util.StringUtil#arrayToString(Object, String)
*
* @param isArr
* The Array to represent as String.
*
* @return a String-representation of the Object.
*
*
*/
public static final String arrayToString(final Object isArr) {
String result = StringUtil.arrayToString(isArr, ",");
return result;
}
/**
* If the given Object is no Array, it's toString - method is invoked.
* Primitive type - Arrays and Object - Arrays are introspected using
* java.lang.reflect.Array. Convention for creation for String -
* representation:
*
*
* // Primitive arrays:
* "["+isArr[0]+"<separator>"+isArr[1]+.. ..+isArr[isArr.length-1]+"]"
*
*
* //Object arrays :
* "["+isArr[0].toString()+"<separator>"+.. ..+isArr[isArr.length-1].toString+"]"
* // Two or three - dimensional Arrays are not supported
* //(should be reflected in a special output method, e.g.as a field)
*
* // other Objects:
* toString()
*
*
* @param separator put in-between each array element in the resulting string.
*
* @param isArr
* The Array to represent as String.
*
* @return a String-representation of the Object.
*
*
*/
public static final String arrayToString(final Object isArr, final String separator) {
String result;
if (isArr == null) {
result = "null";
} else {
Object element;
StringBuffer tmp = new StringBuffer();
try {
int length = Array.getLength(isArr);
tmp.append("[");
for (int i = 0; i < length; i++) {
element = Array.get(isArr, i);
if (element == null) {
tmp.append("null");
} else {
tmp.append(element.toString());
}
if (i < length - 1) {
tmp.append(separator);
}
}
tmp.append("]");
result = tmp.toString();
} catch (ArrayIndexOutOfBoundsException bound) {
// programming mistake or bad Array.getLength(obj).
tmp.append("]");
result = tmp.toString();
} catch (IllegalArgumentException noarr) {
result = isArr.toString();
}
}
return result;
}
/**
* Returns the system - dependent line separator.
*
*
* Only call this method once (not in a loop) and keep the result.
*
*
* @return the system - dependent line separator.
*/
public static String getNewLine() {
return System.getProperty("line.separator");
}
/**
* Returns the singleton instance of this class.
*
*
* This method is useless for now as all methods are static. It may be used in
* future if VM-global configuration will be put to the state of the instance.
*
* #
*
* @return the singleton instance of this class.
*/
public static StringUtil instance() {
if (StringUtil.instance == null) {
StringUtil.instance = new StringUtil();
}
return StringUtil.instance;
}
/**
* Returns true if the argument is null or consists of whitespaces only.
*
*
* @param test
* the String
to test.
*
* @return true if the argument is null or consists of whitespaces only.
*/
public static boolean isEmpty(final String test) {
boolean result;
if (test == null) {
result = true;
} else {
result = test.trim().length() == 0;
}
return result;
}
/**
* Little String output - helper that modifies the given LinkedList by getting
* it's Objects and replace them by their toString() - representation.
*
*
* What is special?
* If an Object in the given List is an Array (of Objects or primitive data
* types) reflection will be used to create a String - representation of them.
* The changes are reflected in the Objects that are in the given List. So
* keep a reference to it. If you are sure, that your List does not contain
* Arrays do not use this method to avoid overhead.
*
*
* Avoid structural modifications (remove) of the list while using this
* method. This method or better: the given List is only thread - safe if the
* list is synchronized.
*
*
* A clever VM (hotspot) will be able to inline this function because of void
* return.
*
*
* @param objects
* the List of objects that will be changed to a list of the String
* representation of the Objects with respect to special array
* treatment.
*
*/
public static final void listOfArraysToString(final List