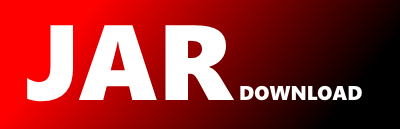
com.xiongyingqi.common.http.HttpParameterHandler Maven / Gradle / Ivy
package com.xiongyingqi.common.http;
import com.xiongyingqi.common.utils.reflect.ReflectionUtils;
import com.xiongyingqi.common.http.annotation.HttpParameter;
import com.xiongyingqi.common.http.annotation.ParameterIgnore;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.HashMap;
import java.util.Map;
/**
* @author xiongyingqi
* @since 16-12-17 下午5:01
*/
public class HttpParameterHandler {
private static final Logger logger = LoggerFactory.getLogger(HttpParameterHandler.class);
public Class type;
private Field[] declaredFields;
private Map fieldParameterNameMap = new HashMap();
public HttpParameterHandler(Class type) {
this.type = type;
initFieldParameterNameMap();
}
private void initFieldParameterNameMap() {
synchronized (this) {
fieldParameterNameMap.clear();
Map map = parseClass(type);
fieldParameterNameMap.putAll(map);
logger.info("Initialized field parameters: {} by class: {}", fieldParameterNameMap, type);
declaredFields = ReflectionUtils.findFields(type, true);
}
}
private boolean inited() {
if (fieldParameterNameMap == null) {
synchronized (this) {
if (fieldParameterNameMap == null) {
return false;
}
}
}
return true;
}
private void check() {
if (!inited()) {
initFieldParameterNameMap();
}
}
public Map getParameterMap(T instance) {
if (instance == null) {
return null;
}
check();
return parseParameters(instance);
}
@SuppressWarnings("unchecked")
public Map getParameterMapWithObject(Object instance) {
if (instance == null) {
return null;
}
if (!instance.getClass().equals(type)) {
return null;
}
T i = (T) instance;
return getParameterMap(i);
}
private Map parseParameters(T instance) {
Map map = new HashMap();
for (Field declaredField : declaredFields) {
if (Modifier.isStatic(declaredField.getModifiers()) || Modifier.isNative(declaredField.getModifiers())) {
continue;
}
String name;
if ((name = fieldParameterNameMap.get(declaredField)) == null) {
if (logger.isDebugEnabled()) {
logger.debug("Could'nt found parameter name on field: {}", declaredField);
}
continue;
}
Object fieldValue = null;
try {
fieldValue = ReflectionUtils.getFieldValue(declaredField, instance);
} catch (IllegalAccessException e) {
logger.error("", e);
}
if (fieldValue == null) {
if (logger.isDebugEnabled()) {
logger.debug("Null value of field: {}", declaredField);
}
continue;
}
String value = fieldValue.toString();
map.put(name, value);
}
return map;
}
private static Map parseClass(Class> clazz) {
Map map = new HashMap();
Field[] fields = ReflectionUtils.findFields(clazz, true);
for (Field field : fields) {
if (Modifier.isStatic(field.getModifiers()) || Modifier.isNative(field.getModifiers())) {
continue;
}
String parameterName = getFieldParameterName(field);
if (parameterName == null || "".equals(parameterName)) {
continue;
}
map.put(field, parameterName);
}
return map;
}
public static String getFieldParameterName(Field field) {
if (field.getAnnotation(ParameterIgnore.class) != null) {
return null;
}
String methodName = null;
if (!field.isAnnotationPresent(HttpParameter.class)) {
methodName = parseMethod(field);
}
if (methodName == null || "".equals(methodName)) {
methodName = parseField(field);
}
return methodName;
}
public static String parseField(Field field) {
HttpParameter annotation = field.getAnnotation(HttpParameter.class);
String value;
if (annotation == null || "".equals((value = annotation.value()))) {
return field.getName();
}
return value;
}
public static String parseMethod(Field field) {
try {
Method getMethod = ReflectionUtils.findGetMethod(field);
if (!getMethod.isAnnotationPresent(HttpParameter.class)) {
return null;
}
HttpParameter annotation = getMethod.getAnnotation(HttpParameter.class);
if (annotation == null) {
return null;
}
return annotation.value();
} catch (NoSuchMethodException ignored) {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy