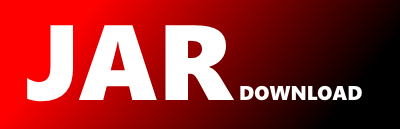
cn.xishan.oftenporter.bridge.http.websocket.SessionImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Porter-Bridge-Http Show documentation
Show all versions of Porter-Bridge-Http Show documentation
转接远程的http接口,服务器响应正确的数据格式必须是JResponse定义的格式。
客户端websocket使用"org.java-websocket:Java-WebSocket:1.5.2",项目地址https://github.com/TooTallNate/Java-WebSocket;
对Java-WebSocket做了适当修改。
The newest version!
package cn.xishan.oftenporter.bridge.http.websocket;
import org.java_websocket.client.WebSocketClient;
import org.java_websocket.framing.PingFrame;
import org.java_websocket.framing.PongFrame;
import java.nio.ByteBuffer;
import java.util.Hashtable;
import java.util.Map;
/**
* @author Created by https://github.com/CLovinr on 2017/11/20.
*/
class SessionImpl implements Session
{
WebSocketClient webSocketClient;
private OnClose onClose;
private String id;
private Map attrMap;
interface OnClose
{
void onClosed();
}
public SessionImpl(WebSocketClient webSocketClient, OnClose onClose, String id)
{
this.webSocketClient = webSocketClient;
this.onClose = onClose;
this.id = id;
this.attrMap = new Hashtable<>();
}
@Override
public String getId()
{
return id;
}
@Override
public Map getUserProperties()
{
return attrMap;
}
@Override
public void close()
{
webSocketClient.close();
onClose.onClosed();
}
@Override
public boolean isClosed()
{
return webSocketClient.isClosed();
}
@Override
public void close(int code, String reason)
{
webSocketClient.close(code, reason);
onClose.onClosed();
}
@Override
public void sendPing(ByteBuffer applicationData)
{
PingFrame pingFrame = new PingFrame();
if (applicationData != null)
{
pingFrame.setPayload(applicationData);
}
webSocketClient.sendFrame(pingFrame);
}
@Override
public void sendPong(ByteBuffer applicationData)
{
PongFrame pongFrame = new PongFrame();
if (applicationData != null)
{
pongFrame.setPayload(applicationData);
}
webSocketClient.sendFrame(pongFrame);
}
@Override
public void send(String text)
{
webSocketClient.send(text);
}
@Override
public void send(ByteBuffer byteBuffer)
{
webSocketClient.send(byteBuffer);
}
@Override
public void send(byte[] bs)
{
webSocketClient.send(bs);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy