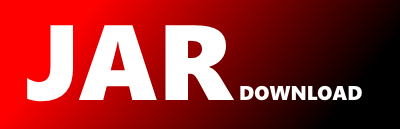
com.xlrit.gears.application.GearsServletProcessApplication Maven / Gradle / Ivy
package com.xlrit.gears.application;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.Map;
import javax.enterprise.inject.Produces;
import javax.enterprise.inject.spi.CDI;
import javax.inject.Inject;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import org.camunda.bpm.application.PostDeploy;
import org.camunda.bpm.application.ProcessApplication;
import org.camunda.bpm.application.impl.ServletProcessApplication;
import org.camunda.bpm.engine.ProcessEngine;
import org.camunda.bpm.engine.impl.javax.el.ELResolver;
import org.camunda.bpm.engine.impl.variable.serializer.DefaultVariableSerializers;
import org.camunda.bpm.engine.impl.variable.serializer.VariableSerializers;
import org.hibernate.boot.Metadata;
import org.hibernate.boot.MetadataSources;
import org.hibernate.boot.registry.StandardServiceRegistry;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
import org.hibernate.cfg.AvailableSettings;
import org.hibernate.tool.hbm2ddl.SchemaExport;
import org.hibernate.tool.schema.TargetType;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.xlrit.gears.base.execution.ExecutionFactory;
import com.xlrit.gears.base.form.ChoicesFactory;
import com.xlrit.gears.engine.GearsProcessApplication;
import com.xlrit.gears.engine.camunda.CdiElResolver;
import com.xlrit.gears.engine.camunda.GearsFormSerializer;
import com.xlrit.gears.engine.camunda.GearsObjectSerializer;
import com.xlrit.gears.engine.config.ConfigProperty;
import com.xlrit.gears.engine.form.CdiCompanionManager;
import com.xlrit.gears.engine.form.ChoicesFactoryImpl;
import com.xlrit.gears.engine.form.GearsFormEngine;
import com.xlrit.gears.engine.form.GearsFormEngineImpl;
import com.xlrit.gears.engine.meta.CdiMetaManager;
import com.xlrit.gears.engine.meta.MetaManager;
@ProcessApplication
public class GearsServletProcessApplication extends ServletProcessApplication implements GearsProcessApplication {
private static final Logger LOG = LoggerFactory.getLogger(GearsServletProcessApplication.class);
private final ELResolver elResolver;
private final MetaManager metaManager;
private final GearsFormEngine formEngine;
private final VariableSerializers variableSerializers;
@PersistenceContext
private EntityManager em;
@Inject @ConfigProperty("gears.persistence.schema.application.action")
private String schemaAction;
@Inject
public GearsServletProcessApplication(ExecutionFactory executionFactory) {
CDI
© 2015 - 2025 Weber Informatics LLC | Privacy Policy