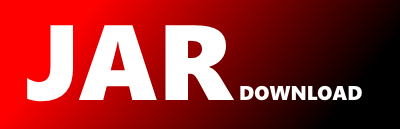
com.xlvchao.clickhouse.model.ClickHouseSinkRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickhouse-highlevel-sinker Show documentation
Show all versions of clickhouse-highlevel-sinker Show documentation
High Performance SDK for Batch Write ClickHouse Database!
The newest version!
package com.xlvchao.clickhouse.model;
import java.util.List;
public class ClickHouseSinkRequest {
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy