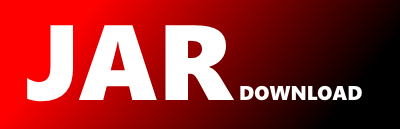
com.xnx3.doc.JavaDoc Maven / Gradle / Ivy
package com.xnx3.doc;
import java.io.File;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Parameter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.xnx3.FileUtil;
import com.xnx3.ScanClassUtil;
import com.xnx3.SystemUtil;
import com.xnx3.UrlUtil;
import com.xnx3.doc.bean.ClassBean;
import com.xnx3.doc.bean.MethodBean;
import com.xnx3.doc.bean.ParamBean;
import com.xnx3.doc.javadoc.JavaDocBean;
import com.xnx3.doc.javadoc.JavaDocMethodBean;
import com.xnx3.doc.javadoc.JavaDocUtil;
import com.xnx3.net.HttpResponse;
import com.xnx3.net.HttpUtil;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
/**
* JavaDoc 生成接口文档
* @author 管雷鸣
*
*/
public class JavaDoc {
private String packageName; //要搜索的包名,如 com.xnx3.wangmarket ,会自动搜索这个包下所有符合的自动生成文档
public String templatePath = "http://res.zvo.cn.obs.cn-north-4.myhuaweicloud.com/javadoc/v1.8/"; //模板所在路径,如 http://res.zvo.cn/javadoc/template/ 这个目录下有 template.html、style.css、javadoc.js
/******* 生成的文档中的一些默认值 *******/
public String name = "API文档"; //文档名字,如 云商城用户端API文档,不设置默认为 API文档
public String version = "1.0"; //当前文档对应你系统的版本,默认是1.0,这里是你当前系统的版本
public String domain = "http://localhost:8080"; //API接口请求域名,不设置默认是 http://localhost:8080,这里是第一次打开文档,没有设置请求域名时,默认的域名
public String token = ""; //第一次打开文档时,默认的token值,不设置默认为 “” 空字符串
public String welcome = ""; //会在index.html中显示,作为入口欢迎页的说明显示。可以将一些文档通用性说明放到这里。可设置为html格式(CSS直接写到里面)以使之更美观。
/**
* java源文件所在的文件夹路径,里面的路径如:
* H:\git\wm\
*/
public static List javaSourceFolderList = new ArrayList();
/**
* 创建 JavaDoc 接口文档对象
* @param packageName 要搜索的包名,如 com.xnx3.wangmarket ,会自动搜索这个包下所有符合的自动生成文档
*/
public JavaDoc(String packageName) {
this.packageName = packageName;
String[] subProjectName = {"wm","xnx3_util","xnx3_weixin","wangmarket_shop","wangmarket"};
File file = new File(SystemUtil.getCurrentDir());
for (int i = 0; i < subProjectName.length; i++) {
String subJarProjectPath = file.getParentFile().getAbsolutePath()+File.separator+subProjectName[i]+File.separator;
JavaDoc.javaSourceFolderList.add(subJarProjectPath);
}
}
public static void main(String[] args) {
JavaDoc doc = new JavaDoc("com.xnx3.demo");
doc.javaSourceFolderList.add("elseProject");
doc.generateHtmlDoc();
}
/**
* 生成 HTML DOC 文档
*/
public void generateHtmlDoc() {
List list = searchController();
//过滤一些如request、model 这种的无用的参数
for (int i = 0; i < list.size(); i++) {
ClassBean classBean = list.get(i);
for(int m = 0; m < classBean.getMethodList().size(); m++) {
MethodBean mb = classBean.getMethodList().get(m);
List removeKey = new ArrayList();
for (Map.Entry entry : mb.getParams().entrySet()) {
if("HttpServletRequest".equalsIgnoreCase(entry.getValue().getType())) {
removeKey.add(entry.getKey());
}else if("Model".equalsIgnoreCase(entry.getValue().getType())) {
removeKey.add(entry.getKey());
}
}
for (int rk = 0; rk < removeKey.size(); rk++) {
mb.getParams().remove(removeKey.get(rk));
}
}
}
/**** 拉下最新的模板、css、js相关 ****/
TemplateUtil template = new TemplateUtil(this);
String apiTemplate = template.getTemplate();
//创建存放 html的目录
String path = SystemUtil.getCurrentDir();
String htmldocPath = path+"/htmldoc/";
File file = new File(htmldocPath);
file.mkdir();
Log.log("自动创建doc文档存放目录: "+htmldocPath);
/**** 生成具体api的文档 ****/
for (int i = 0; i < list.size(); i++) {
ClassBean classBean = list.get(i);
List methodList = classBean.getMethodList();
for(int m = 0; m "+methodBean.getApiUrl()+".html");
}
}
//生成 style.css
// FileUtil.write(htmldocPath+"style.css", cssHr.getContent());
//生成 htmldoc.js
// FileUtil.write(htmldocPath+"htmldoc.js", jsHr.getContent());
/**** 生成文档目录 ****/
String indexTemplate = template.getIndex();
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy