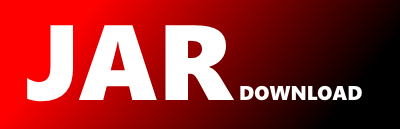
com.xqbase.util.Lazy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xqbase-util-jdk17 Show documentation
Show all versions of xqbase-util-jdk17 Show documentation
Reusable Java components for www.xqbase.com
package com.xqbase.util;
import com.xqbase.util.function.ConsumerEx;
import com.xqbase.util.function.SupplierEx;
/**
* A lazy factory for singletons implemented by double-checked locking.
*
* @param type of the instance
* @param type of exception when initializing
*/
public class Lazy implements AutoCloseable {
private SupplierEx extends T, ? extends E> initializer;
private ConsumerEx super T, ?> finalizer;
private volatile T object = null;
/**
* Create a lazy factory by the given initializer and finalizer.
*
* @param initializer a supplier to create the instance
* @param finalizer a consumer to destroy the instance
*/
public Lazy(SupplierEx extends T, ? extends E> initializer,
ConsumerEx super T, ?> finalizer) {
this.initializer = initializer;
this.finalizer = finalizer;
}
/**
* Create or get the instance.
*
* @return the instance
* @throws E exception thrown by initializer
*/
public T get() throws E {
// use a temporary variable to reduce the number of reads of the volatile field
// see commons-lang3
T result = object;
if (object == null) {
synchronized (this) {
result = object;
if (object == null) {
object = result = initializer.get();
}
}
}
return result;
}
/**
* Close the lazy factory and destroy the instance if created.
*/
@Override
public void close() {
synchronized (this) {
if (object != null) {
T object_ = object;
object = null;
try {
finalizer.accept(object_);
} catch (Exception e) {
// Ignored
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy