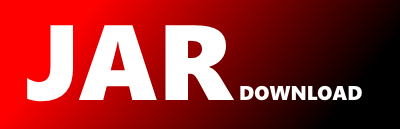
xtdb.antlr.SqlVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xtdb-core Show documentation
Show all versions of xtdb-core Show documentation
An open source document database with bitemporal graph queries
The newest version!
// Generated from xtdb/antlr/Sql.g4 by ANTLR 4.13.1
package xtdb.antlr;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link Sql}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface SqlVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link Sql#directSqlStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDirectSqlStatement(Sql.DirectSqlStatementContext ctx);
/**
* Visit a parse tree produced by the {@code QueryExpr}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQueryExpr(Sql.QueryExprContext ctx);
/**
* Visit a parse tree produced by the {@code InsertStmt}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInsertStmt(Sql.InsertStmtContext ctx);
/**
* Visit a parse tree produced by the {@code UpdateStmt}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUpdateStmt(Sql.UpdateStmtContext ctx);
/**
* Visit a parse tree produced by the {@code DeleteStmt}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDeleteStmt(Sql.DeleteStmtContext ctx);
/**
* Visit a parse tree produced by the {@code EraseStmt}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEraseStmt(Sql.EraseStmtContext ctx);
/**
* Visit a parse tree produced by the {@code AssertStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAssertStatement(Sql.AssertStatementContext ctx);
/**
* Visit a parse tree produced by the {@code StartTransactionStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStartTransactionStatement(Sql.StartTransactionStatementContext ctx);
/**
* Visit a parse tree produced by the {@code SetTransactionStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetTransactionStatement(Sql.SetTransactionStatementContext ctx);
/**
* Visit a parse tree produced by the {@code CommitStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCommitStatement(Sql.CommitStatementContext ctx);
/**
* Visit a parse tree produced by the {@code RollbackStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRollbackStatement(Sql.RollbackStatementContext ctx);
/**
* Visit a parse tree produced by the {@code SetSessionCharacteristicsStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetSessionCharacteristicsStatement(Sql.SetSessionCharacteristicsStatementContext ctx);
/**
* Visit a parse tree produced by the {@code SetRoleStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetRoleStatement(Sql.SetRoleStatementContext ctx);
/**
* Visit a parse tree produced by the {@code SetTimeZoneStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetTimeZoneStatement(Sql.SetTimeZoneStatementContext ctx);
/**
* Visit a parse tree produced by the {@code SetSessionVariableStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetSessionVariableStatement(Sql.SetSessionVariableStatementContext ctx);
/**
* Visit a parse tree produced by the {@code ShowVariableStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitShowVariableStatement(Sql.ShowVariableStatementContext ctx);
/**
* Visit a parse tree produced by the {@code ShowSessionVariableStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitShowSessionVariableStatement(Sql.ShowSessionVariableStatementContext ctx);
/**
* Visit a parse tree produced by the {@code ShowLatestSubmittedTransactionStatement}
* labeled alternative in {@link Sql#directlyExecutableStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitShowLatestSubmittedTransactionStatement(Sql.ShowLatestSubmittedTransactionStatementContext ctx);
/**
* Visit a parse tree produced by the {@code ShowTransactionIsolationLevel}
* labeled alternative in {@link Sql#showVariable}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitShowTransactionIsolationLevel(Sql.ShowTransactionIsolationLevelContext ctx);
/**
* Visit a parse tree produced by the {@code ShowStandardConformingStrings}
* labeled alternative in {@link Sql#showVariable}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitShowStandardConformingStrings(Sql.ShowStandardConformingStringsContext ctx);
/**
* Visit a parse tree produced by the {@code ShowTimeZone}
* labeled alternative in {@link Sql#showVariable}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitShowTimeZone(Sql.ShowTimeZoneContext ctx);
/**
* Visit a parse tree produced by {@link Sql#settingQueryVariables}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSettingQueryVariables(Sql.SettingQueryVariablesContext ctx);
/**
* Visit a parse tree produced by the {@code SettingDefaultValidTime}
* labeled alternative in {@link Sql#settingQueryVariable}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSettingDefaultValidTime(Sql.SettingDefaultValidTimeContext ctx);
/**
* Visit a parse tree produced by the {@code SettingDefaultSystemTime}
* labeled alternative in {@link Sql#settingQueryVariable}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSettingDefaultSystemTime(Sql.SettingDefaultSystemTimeContext ctx);
/**
* Visit a parse tree produced by the {@code SettingBasis}
* labeled alternative in {@link Sql#settingQueryVariable}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSettingBasis(Sql.SettingBasisContext ctx);
/**
* Visit a parse tree produced by the {@code SettingCurrentTime}
* labeled alternative in {@link Sql#settingQueryVariable}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSettingCurrentTime(Sql.SettingCurrentTimeContext ctx);
/**
* Visit a parse tree produced by {@link Sql#intervalLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntervalLiteral(Sql.IntervalLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code DateLiteral}
* labeled alternative in {@link Sql#dateTimeLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateLiteral(Sql.DateLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code TimestampLiteral}
* labeled alternative in {@link Sql#dateTimeLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimestampLiteral(Sql.TimestampLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code FloatLiteral}
* labeled alternative in {@link Sql#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFloatLiteral(Sql.FloatLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code IntegerLiteral}
* labeled alternative in {@link Sql#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntegerLiteral(Sql.IntegerLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code CharacterStringLiteral}
* labeled alternative in {@link Sql#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCharacterStringLiteral(Sql.CharacterStringLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code BinaryStringLiteral}
* labeled alternative in {@link Sql#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBinaryStringLiteral(Sql.BinaryStringLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code DateTimeLiteral0}
* labeled alternative in {@link Sql#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateTimeLiteral0(Sql.DateTimeLiteral0Context ctx);
/**
* Visit a parse tree produced by the {@code TimeLiteral}
* labeled alternative in {@link Sql#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimeLiteral(Sql.TimeLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code IntervalLiteral0}
* labeled alternative in {@link Sql#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntervalLiteral0(Sql.IntervalLiteral0Context ctx);
/**
* Visit a parse tree produced by the {@code DurationLiteral}
* labeled alternative in {@link Sql#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDurationLiteral(Sql.DurationLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code UUIDLiteral}
* labeled alternative in {@link Sql#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUUIDLiteral(Sql.UUIDLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code BooleanLiteral}
* labeled alternative in {@link Sql#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBooleanLiteral(Sql.BooleanLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code NullLiteral}
* labeled alternative in {@link Sql#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNullLiteral(Sql.NullLiteralContext ctx);
/**
* Visit a parse tree produced by {@link Sql#dollarStringText}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDollarStringText(Sql.DollarStringTextContext ctx);
/**
* Visit a parse tree produced by the {@code SqlStandardString}
* labeled alternative in {@link Sql#characterString}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSqlStandardString(Sql.SqlStandardStringContext ctx);
/**
* Visit a parse tree produced by the {@code CEscapesString}
* labeled alternative in {@link Sql#characterString}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCEscapesString(Sql.CEscapesStringContext ctx);
/**
* Visit a parse tree produced by the {@code DollarString}
* labeled alternative in {@link Sql#characterString}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDollarString(Sql.DollarStringContext ctx);
/**
* Visit a parse tree produced by {@link Sql#intervalQualifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntervalQualifier(Sql.IntervalQualifierContext ctx);
/**
* Visit a parse tree produced by {@link Sql#startField}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStartField(Sql.StartFieldContext ctx);
/**
* Visit a parse tree produced by {@link Sql#endField}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEndField(Sql.EndFieldContext ctx);
/**
* Visit a parse tree produced by {@link Sql#intervalFractionalSecondsPrecision}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntervalFractionalSecondsPrecision(Sql.IntervalFractionalSecondsPrecisionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#nonSecondPrimaryDatetimeField}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNonSecondPrimaryDatetimeField(Sql.NonSecondPrimaryDatetimeFieldContext ctx);
/**
* Visit a parse tree produced by {@link Sql#singleDatetimeField}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSingleDatetimeField(Sql.SingleDatetimeFieldContext ctx);
/**
* Visit a parse tree produced by {@link Sql#identifierChain}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIdentifierChain(Sql.IdentifierChainContext ctx);
/**
* Visit a parse tree produced by the {@code RegularIdentifier}
* labeled alternative in {@link Sql#identifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRegularIdentifier(Sql.RegularIdentifierContext ctx);
/**
* Visit a parse tree produced by the {@code DelimitedIdentifier}
* labeled alternative in {@link Sql#identifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDelimitedIdentifier(Sql.DelimitedIdentifierContext ctx);
/**
* Visit a parse tree produced by {@link Sql#schemaName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSchemaName(Sql.SchemaNameContext ctx);
/**
* Visit a parse tree produced by {@link Sql#tableName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableName(Sql.TableNameContext ctx);
/**
* Visit a parse tree produced by {@link Sql#columnName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnName(Sql.ColumnNameContext ctx);
/**
* Visit a parse tree produced by {@link Sql#correlationName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCorrelationName(Sql.CorrelationNameContext ctx);
/**
* Visit a parse tree produced by {@link Sql#queryName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQueryName(Sql.QueryNameContext ctx);
/**
* Visit a parse tree produced by {@link Sql#fieldName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFieldName(Sql.FieldNameContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowName(Sql.WindowNameContext ctx);
/**
* Visit a parse tree produced by the {@code DurationType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDurationType(Sql.DurationTypeContext ctx);
/**
* Visit a parse tree produced by the {@code CharacterStringType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCharacterStringType(Sql.CharacterStringTypeContext ctx);
/**
* Visit a parse tree produced by the {@code IntervalType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntervalType(Sql.IntervalTypeContext ctx);
/**
* Visit a parse tree produced by the {@code BooleanType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBooleanType(Sql.BooleanTypeContext ctx);
/**
* Visit a parse tree produced by the {@code RegProcType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRegProcType(Sql.RegProcTypeContext ctx);
/**
* Visit a parse tree produced by the {@code RowType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRowType(Sql.RowTypeContext ctx);
/**
* Visit a parse tree produced by the {@code RealType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRealType(Sql.RealTypeContext ctx);
/**
* Visit a parse tree produced by the {@code TimestampTzType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimestampTzType(Sql.TimestampTzTypeContext ctx);
/**
* Visit a parse tree produced by the {@code FloatType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFloatType(Sql.FloatTypeContext ctx);
/**
* Visit a parse tree produced by the {@code TimeType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimeType(Sql.TimeTypeContext ctx);
/**
* Visit a parse tree produced by the {@code IntegerType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntegerType(Sql.IntegerTypeContext ctx);
/**
* Visit a parse tree produced by the {@code ArrayType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArrayType(Sql.ArrayTypeContext ctx);
/**
* Visit a parse tree produced by the {@code RegClassType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRegClassType(Sql.RegClassTypeContext ctx);
/**
* Visit a parse tree produced by the {@code DoubleType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDoubleType(Sql.DoubleTypeContext ctx);
/**
* Visit a parse tree produced by the {@code DecimalType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDecimalType(Sql.DecimalTypeContext ctx);
/**
* Visit a parse tree produced by the {@code TimestampType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimestampType(Sql.TimestampTypeContext ctx);
/**
* Visit a parse tree produced by the {@code DateType}
* labeled alternative in {@link Sql#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateType(Sql.DateTypeContext ctx);
/**
* Visit a parse tree produced by {@link Sql#precision}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPrecision(Sql.PrecisionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#scale}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitScale(Sql.ScaleContext ctx);
/**
* Visit a parse tree produced by {@link Sql#charLengthUnits}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCharLengthUnits(Sql.CharLengthUnitsContext ctx);
/**
* Visit a parse tree produced by the {@code WithTimeZone}
* labeled alternative in {@link Sql#withOrWithoutTimeZone}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWithTimeZone(Sql.WithTimeZoneContext ctx);
/**
* Visit a parse tree produced by the {@code WithoutTimeZone}
* labeled alternative in {@link Sql#withOrWithoutTimeZone}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWithoutTimeZone(Sql.WithoutTimeZoneContext ctx);
/**
* Visit a parse tree produced by {@link Sql#maximumCardinality}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMaximumCardinality(Sql.MaximumCardinalityContext ctx);
/**
* Visit a parse tree produced by {@link Sql#fieldDefinition}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFieldDefinition(Sql.FieldDefinitionContext ctx);
/**
* Visit a parse tree produced by the {@code AndExpr}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAndExpr(Sql.AndExprContext ctx);
/**
* Visit a parse tree produced by the {@code PeriodContainsPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPeriodContainsPredicate(Sql.PeriodContainsPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code PeriodImmediatelyPrecedesPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPeriodImmediatelyPrecedesPredicate(Sql.PeriodImmediatelyPrecedesPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code IsBooleanValueExpr}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsBooleanValueExpr(Sql.IsBooleanValueExprContext ctx);
/**
* Visit a parse tree produced by the {@code BetweenPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBetweenPredicate(Sql.BetweenPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code PostgresRegexPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPostgresRegexPredicate(Sql.PostgresRegexPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code UnaryNotExpr}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnaryNotExpr(Sql.UnaryNotExprContext ctx);
/**
* Visit a parse tree produced by the {@code PeriodSucceedsPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPeriodSucceedsPredicate(Sql.PeriodSucceedsPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code LikePredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLikePredicate(Sql.LikePredicateContext ctx);
/**
* Visit a parse tree produced by the {@code InPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInPredicate(Sql.InPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code PeriodPrecedesPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPeriodPrecedesPredicate(Sql.PeriodPrecedesPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code ComparisonPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitComparisonPredicate(Sql.ComparisonPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code OrExpr}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOrExpr(Sql.OrExprContext ctx);
/**
* Visit a parse tree produced by the {@code PeriodImmediatelySucceedsPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPeriodImmediatelySucceedsPredicate(Sql.PeriodImmediatelySucceedsPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code QuantifiedComparisonPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQuantifiedComparisonPredicate(Sql.QuantifiedComparisonPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code NullPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNullPredicate(Sql.NullPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code NumericExpr0}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumericExpr0(Sql.NumericExpr0Context ctx);
/**
* Visit a parse tree produced by the {@code PeriodEqualsPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPeriodEqualsPredicate(Sql.PeriodEqualsPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code LikeRegexPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLikeRegexPredicate(Sql.LikeRegexPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code PeriodOverlapsPredicate}
* labeled alternative in {@link Sql#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPeriodOverlapsPredicate(Sql.PeriodOverlapsPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code NumericBitwiseAndExpr}
* labeled alternative in {@link Sql#numericExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumericBitwiseAndExpr(Sql.NumericBitwiseAndExprContext ctx);
/**
* Visit a parse tree produced by the {@code NumericBitwiseNotExpr}
* labeled alternative in {@link Sql#numericExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumericBitwiseNotExpr(Sql.NumericBitwiseNotExprContext ctx);
/**
* Visit a parse tree produced by the {@code UnaryPlusExpr}
* labeled alternative in {@link Sql#numericExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnaryPlusExpr(Sql.UnaryPlusExprContext ctx);
/**
* Visit a parse tree produced by the {@code NumericTermExpr}
* labeled alternative in {@link Sql#numericExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumericTermExpr(Sql.NumericTermExprContext ctx);
/**
* Visit a parse tree produced by the {@code NumericBitwiseOrExpr}
* labeled alternative in {@link Sql#numericExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumericBitwiseOrExpr(Sql.NumericBitwiseOrExprContext ctx);
/**
* Visit a parse tree produced by the {@code NumericFactorExpr}
* labeled alternative in {@link Sql#numericExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumericFactorExpr(Sql.NumericFactorExprContext ctx);
/**
* Visit a parse tree produced by the {@code NumericBitwiseShiftExpr}
* labeled alternative in {@link Sql#numericExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumericBitwiseShiftExpr(Sql.NumericBitwiseShiftExprContext ctx);
/**
* Visit a parse tree produced by the {@code ExprPrimary1}
* labeled alternative in {@link Sql#numericExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExprPrimary1(Sql.ExprPrimary1Context ctx);
/**
* Visit a parse tree produced by the {@code UnaryMinusExpr}
* labeled alternative in {@link Sql#numericExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnaryMinusExpr(Sql.UnaryMinusExprContext ctx);
/**
* Visit a parse tree produced by the {@code LowerFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLowerFunction(Sql.LowerFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code UpperInfFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUpperInfFunction(Sql.UpperInfFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code AggregateFunctionExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAggregateFunctionExpr(Sql.AggregateFunctionExprContext ctx);
/**
* Visit a parse tree produced by the {@code TrimArrayFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrimArrayFunction(Sql.TrimArrayFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code CurrentSchemasFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCurrentSchemasFunction(Sql.CurrentSchemasFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code CurrentInstantFunction0}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCurrentInstantFunction0(Sql.CurrentInstantFunction0Context ctx);
/**
* Visit a parse tree produced by the {@code PgExpandArrayFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPgExpandArrayFunction(Sql.PgExpandArrayFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code OverlapsFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOverlapsFunction(Sql.OverlapsFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code TsTzRangeConstructor}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTsTzRangeConstructor(Sql.TsTzRangeConstructorContext ctx);
/**
* Visit a parse tree produced by the {@code PositionFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPositionFunction(Sql.PositionFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code LeastFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLeastFunction(Sql.LeastFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code FloorFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFloorFunction(Sql.FloorFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code AgeFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAgeFunction(Sql.AgeFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code CardinalityFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCardinalityFunction(Sql.CardinalityFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code ArrayAccess}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArrayAccess(Sql.ArrayAccessContext ctx);
/**
* Visit a parse tree produced by the {@code ObjectExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitObjectExpr(Sql.ObjectExprContext ctx);
/**
* Visit a parse tree produced by the {@code PgGetExprFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPgGetExprFunction(Sql.PgGetExprFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code ExtractFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExtractFunction(Sql.ExtractFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code CastExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCastExpr(Sql.CastExprContext ctx);
/**
* Visit a parse tree produced by the {@code CurrentSchemaFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCurrentSchemaFunction(Sql.CurrentSchemaFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code NestOneSubqueryExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNestOneSubqueryExpr(Sql.NestOneSubqueryExprContext ctx);
/**
* Visit a parse tree produced by the {@code UpperFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUpperFunction(Sql.UpperFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code CurrentTimeFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCurrentTimeFunction(Sql.CurrentTimeFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code PostgresCastExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPostgresCastExpr(Sql.PostgresCastExprContext ctx);
/**
* Visit a parse tree produced by the {@code PgGetIndexdefFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPgGetIndexdefFunction(Sql.PgGetIndexdefFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code HasTablePrivilegePredicate}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHasTablePrivilegePredicate(Sql.HasTablePrivilegePredicateContext ctx);
/**
* Visit a parse tree produced by the {@code OverlayFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOverlayFunction(Sql.OverlayFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code CharacterSubstringFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCharacterSubstringFunction(Sql.CharacterSubstringFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code NullIfExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNullIfExpr(Sql.NullIfExprContext ctx);
/**
* Visit a parse tree produced by the {@code HasAnyColumnPrivilegePredicate}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHasAnyColumnPrivilegePredicate(Sql.HasAnyColumnPrivilegePredicateContext ctx);
/**
* Visit a parse tree produced by the {@code ArrayExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArrayExpr(Sql.ArrayExprContext ctx);
/**
* Visit a parse tree produced by the {@code LowerInfFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLowerInfFunction(Sql.LowerInfFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code TrimFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrimFunction(Sql.TrimFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code PowerFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPowerFunction(Sql.PowerFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code DateBinFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateBinFunction(Sql.DateBinFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code CeilingFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCeilingFunction(Sql.CeilingFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code GreatestFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGreatestFunction(Sql.GreatestFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code LnFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLnFunction(Sql.LnFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code Log10Function}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLog10Function(Sql.Log10FunctionContext ctx);
/**
* Visit a parse tree produced by the {@code ColumnExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnExpr(Sql.ColumnExprContext ctx);
/**
* Visit a parse tree produced by the {@code ExistsPredicate}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExistsPredicate(Sql.ExistsPredicateContext ctx);
/**
* Visit a parse tree produced by the {@code ReplaceFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReplaceFunction(Sql.ReplaceFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code NestedWindowFunctionExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNestedWindowFunctionExpr(Sql.NestedWindowFunctionExprContext ctx);
/**
* Visit a parse tree produced by the {@code CurrentUserFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCurrentUserFunction(Sql.CurrentUserFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code SqrtFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSqrtFunction(Sql.SqrtFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code TrigonometricFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrigonometricFunction(Sql.TrigonometricFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code LiteralExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralExpr(Sql.LiteralExprContext ctx);
/**
* Visit a parse tree produced by the {@code SimpleCaseExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSimpleCaseExpr(Sql.SimpleCaseExprContext ctx);
/**
* Visit a parse tree produced by the {@code AbsFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAbsFunction(Sql.AbsFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code ModFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitModFunction(Sql.ModFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code PostgresVersionFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPostgresVersionFunction(Sql.PostgresVersionFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code LengthFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLengthFunction(Sql.LengthFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code ExpFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpFunction(Sql.ExpFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code ParamExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParamExpr(Sql.ParamExprContext ctx);
/**
* Visit a parse tree produced by the {@code ArrayUpperFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArrayUpperFunction(Sql.ArrayUpperFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code CoalesceExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCoalesceExpr(Sql.CoalesceExprContext ctx);
/**
* Visit a parse tree produced by the {@code HasSchemaPrivilegePredicate}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHasSchemaPrivilegePredicate(Sql.HasSchemaPrivilegePredicateContext ctx);
/**
* Visit a parse tree produced by the {@code DateTruncFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateTruncFunction(Sql.DateTruncFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code SearchedCaseExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSearchedCaseExpr(Sql.SearchedCaseExprContext ctx);
/**
* Visit a parse tree produced by the {@code CurrentDatabaseFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCurrentDatabaseFunction(Sql.CurrentDatabaseFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code LocalTimeFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLocalTimeFunction(Sql.LocalTimeFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code WrappedExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWrappedExpr(Sql.WrappedExprContext ctx);
/**
* Visit a parse tree produced by the {@code CharacterLengthFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCharacterLengthFunction(Sql.CharacterLengthFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code ConcatExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConcatExpr(Sql.ConcatExprContext ctx);
/**
* Visit a parse tree produced by the {@code RangeBinsFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRangeBinsFunction(Sql.RangeBinsFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code LogFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLogFunction(Sql.LogFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code GenerateSeriesFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGenerateSeriesFunction(Sql.GenerateSeriesFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code FieldAccess}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFieldAccess(Sql.FieldAccessContext ctx);
/**
* Visit a parse tree produced by the {@code NestManySubqueryExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNestManySubqueryExpr(Sql.NestManySubqueryExprContext ctx);
/**
* Visit a parse tree produced by the {@code OctetLengthFunction}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOctetLengthFunction(Sql.OctetLengthFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code WindowFunctionExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFunctionExpr(Sql.WindowFunctionExprContext ctx);
/**
* Visit a parse tree produced by the {@code ScalarSubqueryExpr}
* labeled alternative in {@link Sql#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitScalarSubqueryExpr(Sql.ScalarSubqueryExprContext ctx);
/**
* Visit a parse tree produced by {@link Sql#replaceTarget}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReplaceTarget(Sql.ReplaceTargetContext ctx);
/**
* Visit a parse tree produced by {@link Sql#replacement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReplacement(Sql.ReplacementContext ctx);
/**
* Visit a parse tree produced by the {@code CurrentDateFunction}
* labeled alternative in {@link Sql#currentInstantFunction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCurrentDateFunction(Sql.CurrentDateFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code CurrentTimestampFunction}
* labeled alternative in {@link Sql#currentInstantFunction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCurrentTimestampFunction(Sql.CurrentTimestampFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code LocalTimestampFunction}
* labeled alternative in {@link Sql#currentInstantFunction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLocalTimestampFunction(Sql.LocalTimestampFunctionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#booleanValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBooleanValue(Sql.BooleanValueContext ctx);
/**
* Visit a parse tree produced by {@link Sql#objectConstructor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitObjectConstructor(Sql.ObjectConstructorContext ctx);
/**
* Visit a parse tree produced by {@link Sql#objectNameAndValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitObjectNameAndValue(Sql.ObjectNameAndValueContext ctx);
/**
* Visit a parse tree produced by {@link Sql#objectName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitObjectName(Sql.ObjectNameContext ctx);
/**
* Visit a parse tree produced by the {@code DynamicParameter}
* labeled alternative in {@link Sql#parameterSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDynamicParameter(Sql.DynamicParameterContext ctx);
/**
* Visit a parse tree produced by the {@code PostgresParameter}
* labeled alternative in {@link Sql#parameterSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPostgresParameter(Sql.PostgresParameterContext ctx);
/**
* Visit a parse tree produced by {@link Sql#columnReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnReference(Sql.ColumnReferenceContext ctx);
/**
* Visit a parse tree produced by {@link Sql#generateSeries}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGenerateSeries(Sql.GenerateSeriesContext ctx);
/**
* Visit a parse tree produced by {@link Sql#seriesStart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSeriesStart(Sql.SeriesStartContext ctx);
/**
* Visit a parse tree produced by {@link Sql#seriesEnd}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSeriesEnd(Sql.SeriesEndContext ctx);
/**
* Visit a parse tree produced by {@link Sql#seriesStep}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSeriesStep(Sql.SeriesStepContext ctx);
/**
* Visit a parse tree produced by the {@code RankWindowFunction}
* labeled alternative in {@link Sql#windowFunctionType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRankWindowFunction(Sql.RankWindowFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code RowNumberWindowFunction}
* labeled alternative in {@link Sql#windowFunctionType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRowNumberWindowFunction(Sql.RowNumberWindowFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code AggregateWindowFunction}
* labeled alternative in {@link Sql#windowFunctionType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAggregateWindowFunction(Sql.AggregateWindowFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code NtileWindowFunction}
* labeled alternative in {@link Sql#windowFunctionType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNtileWindowFunction(Sql.NtileWindowFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code LeadOrLagWindowFunction}
* labeled alternative in {@link Sql#windowFunctionType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLeadOrLagWindowFunction(Sql.LeadOrLagWindowFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code FirstOrLastValueWindowFunction}
* labeled alternative in {@link Sql#windowFunctionType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFirstOrLastValueWindowFunction(Sql.FirstOrLastValueWindowFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code NthValueWindowFunction}
* labeled alternative in {@link Sql#windowFunctionType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNthValueWindowFunction(Sql.NthValueWindowFunctionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#rankFunctionType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRankFunctionType(Sql.RankFunctionTypeContext ctx);
/**
* Visit a parse tree produced by {@link Sql#numberOfTiles}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumberOfTiles(Sql.NumberOfTilesContext ctx);
/**
* Visit a parse tree produced by {@link Sql#leadOrLagExtent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLeadOrLagExtent(Sql.LeadOrLagExtentContext ctx);
/**
* Visit a parse tree produced by {@link Sql#offset}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOffset(Sql.OffsetContext ctx);
/**
* Visit a parse tree produced by {@link Sql#defaultExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDefaultExpression(Sql.DefaultExpressionContext ctx);
/**
* Visit a parse tree produced by the {@code RespectNulls}
* labeled alternative in {@link Sql#nullTreatment}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRespectNulls(Sql.RespectNullsContext ctx);
/**
* Visit a parse tree produced by the {@code IgnoreNulls}
* labeled alternative in {@link Sql#nullTreatment}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIgnoreNulls(Sql.IgnoreNullsContext ctx);
/**
* Visit a parse tree produced by {@link Sql#firstOrLastValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFirstOrLastValue(Sql.FirstOrLastValueContext ctx);
/**
* Visit a parse tree produced by {@link Sql#nthRow}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNthRow(Sql.NthRowContext ctx);
/**
* Visit a parse tree produced by the {@code FromFirst}
* labeled alternative in {@link Sql#fromFirstOrLast}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFromFirst(Sql.FromFirstContext ctx);
/**
* Visit a parse tree produced by the {@code FromLast}
* labeled alternative in {@link Sql#fromFirstOrLast}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFromLast(Sql.FromLastContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowNameOrSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowNameOrSpecification(Sql.WindowNameOrSpecificationContext ctx);
/**
* Visit a parse tree produced by the {@code NestedRowNumberFunction}
* labeled alternative in {@link Sql#nestedWindowFunction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNestedRowNumberFunction(Sql.NestedRowNumberFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code ValueOfExprAtRow}
* labeled alternative in {@link Sql#nestedWindowFunction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValueOfExprAtRow(Sql.ValueOfExprAtRowContext ctx);
/**
* Visit a parse tree produced by {@link Sql#rowMarker}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRowMarker(Sql.RowMarkerContext ctx);
/**
* Visit a parse tree produced by {@link Sql#rowMarkerExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRowMarkerExpression(Sql.RowMarkerExpressionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#rowMarkerDelta}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRowMarkerDelta(Sql.RowMarkerDeltaContext ctx);
/**
* Visit a parse tree produced by {@link Sql#rowMarkerOffset}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRowMarkerOffset(Sql.RowMarkerOffsetContext ctx);
/**
* Visit a parse tree produced by {@link Sql#valueOfDefaultValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValueOfDefaultValue(Sql.ValueOfDefaultValueContext ctx);
/**
* Visit a parse tree produced by {@link Sql#simpleWhenClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSimpleWhenClause(Sql.SimpleWhenClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#searchedWhenClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSearchedWhenClause(Sql.SearchedWhenClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#elseClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitElseClause(Sql.ElseClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#whenOperandList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhenOperandList(Sql.WhenOperandListContext ctx);
/**
* Visit a parse tree produced by {@link Sql#whenOperand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhenOperand(Sql.WhenOperandContext ctx);
/**
* Visit a parse tree produced by {@link Sql#extractField}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExtractField(Sql.ExtractFieldContext ctx);
/**
* Visit a parse tree produced by {@link Sql#primaryDatetimeField}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPrimaryDatetimeField(Sql.PrimaryDatetimeFieldContext ctx);
/**
* Visit a parse tree produced by {@link Sql#timeZoneField}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimeZoneField(Sql.TimeZoneFieldContext ctx);
/**
* Visit a parse tree produced by {@link Sql#extractSource}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExtractSource(Sql.ExtractSourceContext ctx);
/**
* Visit a parse tree produced by {@link Sql#trimSource}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrimSource(Sql.TrimSourceContext ctx);
/**
* Visit a parse tree produced by {@link Sql#trimSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrimSpecification(Sql.TrimSpecificationContext ctx);
/**
* Visit a parse tree produced by {@link Sql#trimCharacter}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrimCharacter(Sql.TrimCharacterContext ctx);
/**
* Visit a parse tree produced by {@link Sql#startPosition}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStartPosition(Sql.StartPositionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#stringLength}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringLength(Sql.StringLengthContext ctx);
/**
* Visit a parse tree produced by {@link Sql#dateTruncPrecision}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateTruncPrecision(Sql.DateTruncPrecisionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#dateTruncSource}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateTruncSource(Sql.DateTruncSourceContext ctx);
/**
* Visit a parse tree produced by {@link Sql#dateTruncTimeZone}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateTruncTimeZone(Sql.DateTruncTimeZoneContext ctx);
/**
* Visit a parse tree produced by {@link Sql#dateBinSource}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateBinSource(Sql.DateBinSourceContext ctx);
/**
* Visit a parse tree produced by {@link Sql#rangeBinsSource}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRangeBinsSource(Sql.RangeBinsSourceContext ctx);
/**
* Visit a parse tree produced by {@link Sql#dateBinOrigin}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateBinOrigin(Sql.DateBinOriginContext ctx);
/**
* Visit a parse tree produced by the {@code ArrayValueConstructorByEnumeration}
* labeled alternative in {@link Sql#arrayValueConstructor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArrayValueConstructorByEnumeration(Sql.ArrayValueConstructorByEnumerationContext ctx);
/**
* Visit a parse tree produced by the {@code ArrayValueConstructorByQuery}
* labeled alternative in {@link Sql#arrayValueConstructor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArrayValueConstructorByQuery(Sql.ArrayValueConstructorByQueryContext ctx);
/**
* Visit a parse tree produced by {@link Sql#trigonometricFunctionName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrigonometricFunctionName(Sql.TrigonometricFunctionNameContext ctx);
/**
* Visit a parse tree produced by {@link Sql#generalLogarithmBase}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGeneralLogarithmBase(Sql.GeneralLogarithmBaseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#generalLogarithmArgument}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGeneralLogarithmArgument(Sql.GeneralLogarithmArgumentContext ctx);
/**
* Visit a parse tree produced by the {@code SingleExprRowConstructor}
* labeled alternative in {@link Sql#rowValueConstructor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSingleExprRowConstructor(Sql.SingleExprRowConstructorContext ctx);
/**
* Visit a parse tree produced by the {@code MultiExprRowConstructor}
* labeled alternative in {@link Sql#rowValueConstructor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMultiExprRowConstructor(Sql.MultiExprRowConstructorContext ctx);
/**
* Visit a parse tree produced by {@link Sql#rowValueList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRowValueList(Sql.RowValueListContext ctx);
/**
* Visit a parse tree produced by {@link Sql#tableValueConstructor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableValueConstructor(Sql.TableValueConstructorContext ctx);
/**
* Visit a parse tree produced by the {@code ParameterRecord}
* labeled alternative in {@link Sql#recordValueConstructor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParameterRecord(Sql.ParameterRecordContext ctx);
/**
* Visit a parse tree produced by the {@code ObjectRecord}
* labeled alternative in {@link Sql#recordValueConstructor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitObjectRecord(Sql.ObjectRecordContext ctx);
/**
* Visit a parse tree produced by {@link Sql#recordsValueList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRecordsValueList(Sql.RecordsValueListContext ctx);
/**
* Visit a parse tree produced by {@link Sql#recordsValueConstructor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRecordsValueConstructor(Sql.RecordsValueConstructorContext ctx);
/**
* Visit a parse tree produced by {@link Sql#fromClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFromClause(Sql.FromClauseContext ctx);
/**
* Visit a parse tree produced by the {@code LateralDerivedTable}
* labeled alternative in {@link Sql#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLateralDerivedTable(Sql.LateralDerivedTableContext ctx);
/**
* Visit a parse tree produced by the {@code DerivedTable}
* labeled alternative in {@link Sql#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDerivedTable(Sql.DerivedTableContext ctx);
/**
* Visit a parse tree produced by the {@code GenerateSeriesTable}
* labeled alternative in {@link Sql#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGenerateSeriesTable(Sql.GenerateSeriesTableContext ctx);
/**
* Visit a parse tree produced by the {@code ArrowTable}
* labeled alternative in {@link Sql#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArrowTable(Sql.ArrowTableContext ctx);
/**
* Visit a parse tree produced by the {@code WrappedTableReference}
* labeled alternative in {@link Sql#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWrappedTableReference(Sql.WrappedTableReferenceContext ctx);
/**
* Visit a parse tree produced by the {@code BaseTable}
* labeled alternative in {@link Sql#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBaseTable(Sql.BaseTableContext ctx);
/**
* Visit a parse tree produced by the {@code CrossJoinTable}
* labeled alternative in {@link Sql#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCrossJoinTable(Sql.CrossJoinTableContext ctx);
/**
* Visit a parse tree produced by the {@code CollectionDerivedTable}
* labeled alternative in {@link Sql#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCollectionDerivedTable(Sql.CollectionDerivedTableContext ctx);
/**
* Visit a parse tree produced by the {@code NaturalJoinTable}
* labeled alternative in {@link Sql#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNaturalJoinTable(Sql.NaturalJoinTableContext ctx);
/**
* Visit a parse tree produced by the {@code JoinTable}
* labeled alternative in {@link Sql#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitJoinTable(Sql.JoinTableContext ctx);
/**
* Visit a parse tree produced by {@link Sql#withOrdinality}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWithOrdinality(Sql.WithOrdinalityContext ctx);
/**
* Visit a parse tree produced by {@link Sql#tableAlias}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableAlias(Sql.TableAliasContext ctx);
/**
* Visit a parse tree produced by {@link Sql#tableProjection}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableProjection(Sql.TableProjectionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#querySystemTimePeriodSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQuerySystemTimePeriodSpecification(Sql.QuerySystemTimePeriodSpecificationContext ctx);
/**
* Visit a parse tree produced by {@link Sql#queryValidTimePeriodSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQueryValidTimePeriodSpecification(Sql.QueryValidTimePeriodSpecificationContext ctx);
/**
* Visit a parse tree produced by the {@code TableAsOf}
* labeled alternative in {@link Sql#tableTimePeriodSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableAsOf(Sql.TableAsOfContext ctx);
/**
* Visit a parse tree produced by the {@code TableAllTime}
* labeled alternative in {@link Sql#tableTimePeriodSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableAllTime(Sql.TableAllTimeContext ctx);
/**
* Visit a parse tree produced by the {@code TableBetween}
* labeled alternative in {@link Sql#tableTimePeriodSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableBetween(Sql.TableBetweenContext ctx);
/**
* Visit a parse tree produced by the {@code TableFromTo}
* labeled alternative in {@link Sql#tableTimePeriodSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableFromTo(Sql.TableFromToContext ctx);
/**
* Visit a parse tree produced by the {@code PeriodSpecLiteral}
* labeled alternative in {@link Sql#periodSpecificationExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPeriodSpecLiteral(Sql.PeriodSpecLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code PeriodSpecParam}
* labeled alternative in {@link Sql#periodSpecificationExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPeriodSpecParam(Sql.PeriodSpecParamContext ctx);
/**
* Visit a parse tree produced by the {@code PeriodSpecNow}
* labeled alternative in {@link Sql#periodSpecificationExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPeriodSpecNow(Sql.PeriodSpecNowContext ctx);
/**
* Visit a parse tree produced by {@link Sql#tableOrQueryName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableOrQueryName(Sql.TableOrQueryNameContext ctx);
/**
* Visit a parse tree produced by {@link Sql#columnNameList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnNameList(Sql.ColumnNameListContext ctx);
/**
* Visit a parse tree produced by the {@code JoinCondition}
* labeled alternative in {@link Sql#joinSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitJoinCondition(Sql.JoinConditionContext ctx);
/**
* Visit a parse tree produced by the {@code NamedColumnsJoin}
* labeled alternative in {@link Sql#joinSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNamedColumnsJoin(Sql.NamedColumnsJoinContext ctx);
/**
* Visit a parse tree produced by {@link Sql#joinType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitJoinType(Sql.JoinTypeContext ctx);
/**
* Visit a parse tree produced by {@link Sql#outerJoinType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOuterJoinType(Sql.OuterJoinTypeContext ctx);
/**
* Visit a parse tree produced by {@link Sql#whereClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhereClause(Sql.WhereClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#groupByClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGroupByClause(Sql.GroupByClauseContext ctx);
/**
* Visit a parse tree produced by the {@code OrdinaryGroupingSet}
* labeled alternative in {@link Sql#groupingElement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOrdinaryGroupingSet(Sql.OrdinaryGroupingSetContext ctx);
/**
* Visit a parse tree produced by the {@code EmptyGroupingSet}
* labeled alternative in {@link Sql#groupingElement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEmptyGroupingSet(Sql.EmptyGroupingSetContext ctx);
/**
* Visit a parse tree produced by {@link Sql#havingClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHavingClause(Sql.HavingClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowClause(Sql.WindowClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowDefinitionList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowDefinitionList(Sql.WindowDefinitionListContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowDefinition}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowDefinition(Sql.WindowDefinitionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#newWindowName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNewWindowName(Sql.NewWindowNameContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowSpecification(Sql.WindowSpecificationContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowSpecificationDetails}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowSpecificationDetails(Sql.WindowSpecificationDetailsContext ctx);
/**
* Visit a parse tree produced by {@link Sql#existingWindowName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExistingWindowName(Sql.ExistingWindowNameContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowPartitionClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowPartitionClause(Sql.WindowPartitionClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowPartitionColumnReferenceList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowPartitionColumnReferenceList(Sql.WindowPartitionColumnReferenceListContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowPartitionColumnReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowPartitionColumnReference(Sql.WindowPartitionColumnReferenceContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowOrderClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowOrderClause(Sql.WindowOrderClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowFrameClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFrameClause(Sql.WindowFrameClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowFrameUnits}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFrameUnits(Sql.WindowFrameUnitsContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowFrameExtent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFrameExtent(Sql.WindowFrameExtentContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowFrameStart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFrameStart(Sql.WindowFrameStartContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowFramePreceding}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFramePreceding(Sql.WindowFramePrecedingContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowFrameBetween}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFrameBetween(Sql.WindowFrameBetweenContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowFrameBound1}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFrameBound1(Sql.WindowFrameBound1Context ctx);
/**
* Visit a parse tree produced by {@link Sql#windowFrameBound2}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFrameBound2(Sql.WindowFrameBound2Context ctx);
/**
* Visit a parse tree produced by {@link Sql#windowFrameBound}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFrameBound(Sql.WindowFrameBoundContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowFrameFollowing}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFrameFollowing(Sql.WindowFrameFollowingContext ctx);
/**
* Visit a parse tree produced by {@link Sql#windowFrameExclusion}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowFrameExclusion(Sql.WindowFrameExclusionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#selectClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectClause(Sql.SelectClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#selectList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectList(Sql.SelectListContext ctx);
/**
* Visit a parse tree produced by {@link Sql#selectListAsterisk}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectListAsterisk(Sql.SelectListAsteriskContext ctx);
/**
* Visit a parse tree produced by {@link Sql#selectSublist}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectSublist(Sql.SelectSublistContext ctx);
/**
* Visit a parse tree produced by {@link Sql#qualifiedAsterisk}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQualifiedAsterisk(Sql.QualifiedAsteriskContext ctx);
/**
* Visit a parse tree produced by {@link Sql#renameClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRenameClause(Sql.RenameClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#renameColumn}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRenameColumn(Sql.RenameColumnContext ctx);
/**
* Visit a parse tree produced by {@link Sql#qualifiedRenameClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQualifiedRenameClause(Sql.QualifiedRenameClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#qualifiedRenameColumn}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQualifiedRenameColumn(Sql.QualifiedRenameColumnContext ctx);
/**
* Visit a parse tree produced by {@link Sql#excludeClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExcludeClause(Sql.ExcludeClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#derivedColumn}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDerivedColumn(Sql.DerivedColumnContext ctx);
/**
* Visit a parse tree produced by {@link Sql#asClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAsClause(Sql.AsClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#queryExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQueryExpression(Sql.QueryExpressionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#queryExpressionNoWith}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQueryExpressionNoWith(Sql.QueryExpressionNoWithContext ctx);
/**
* Visit a parse tree produced by {@link Sql#withClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWithClause(Sql.WithClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#withListElement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWithListElement(Sql.WithListElementContext ctx);
/**
* Visit a parse tree produced by the {@code UnionQuery}
* labeled alternative in {@link Sql#queryExpressionBody}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnionQuery(Sql.UnionQueryContext ctx);
/**
* Visit a parse tree produced by the {@code QueryBodyTerm}
* labeled alternative in {@link Sql#queryExpressionBody}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQueryBodyTerm(Sql.QueryBodyTermContext ctx);
/**
* Visit a parse tree produced by the {@code ExceptQuery}
* labeled alternative in {@link Sql#queryExpressionBody}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExceptQuery(Sql.ExceptQueryContext ctx);
/**
* Visit a parse tree produced by the {@code QuerySpecification}
* labeled alternative in {@link Sql#queryTerm}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQuerySpecification(Sql.QuerySpecificationContext ctx);
/**
* Visit a parse tree produced by the {@code RecordsQuery}
* labeled alternative in {@link Sql#queryTerm}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRecordsQuery(Sql.RecordsQueryContext ctx);
/**
* Visit a parse tree produced by the {@code WrappedQuery}
* labeled alternative in {@link Sql#queryTerm}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWrappedQuery(Sql.WrappedQueryContext ctx);
/**
* Visit a parse tree produced by the {@code ValuesQuery}
* labeled alternative in {@link Sql#queryTerm}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValuesQuery(Sql.ValuesQueryContext ctx);
/**
* Visit a parse tree produced by the {@code IntersectQuery}
* labeled alternative in {@link Sql#queryTerm}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntersectQuery(Sql.IntersectQueryContext ctx);
/**
* Visit a parse tree produced by {@link Sql#orderByClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOrderByClause(Sql.OrderByClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#offsetAndLimit}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOffsetAndLimit(Sql.OffsetAndLimitContext ctx);
/**
* Visit a parse tree produced by {@link Sql#resultOffsetClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitResultOffsetClause(Sql.ResultOffsetClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#fetchFirstClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFetchFirstClause(Sql.FetchFirstClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#offsetRowCount}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOffsetRowCount(Sql.OffsetRowCountContext ctx);
/**
* Visit a parse tree produced by {@link Sql#fetchFirstRowCount}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFetchFirstRowCount(Sql.FetchFirstRowCountContext ctx);
/**
* Visit a parse tree produced by {@link Sql#subquery}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSubquery(Sql.SubqueryContext ctx);
/**
* Visit a parse tree produced by the {@code ComparisonPredicatePart2}
* labeled alternative in {@link Sql#predicatePart2}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitComparisonPredicatePart2(Sql.ComparisonPredicatePart2Context ctx);
/**
* Visit a parse tree produced by the {@code BetweenPredicatePart2}
* labeled alternative in {@link Sql#predicatePart2}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBetweenPredicatePart2(Sql.BetweenPredicatePart2Context ctx);
/**
* Visit a parse tree produced by the {@code InPredicatePart2}
* labeled alternative in {@link Sql#predicatePart2}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInPredicatePart2(Sql.InPredicatePart2Context ctx);
/**
* Visit a parse tree produced by the {@code LikePredicatePart2}
* labeled alternative in {@link Sql#predicatePart2}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLikePredicatePart2(Sql.LikePredicatePart2Context ctx);
/**
* Visit a parse tree produced by the {@code LikeRegexPredicatePart2}
* labeled alternative in {@link Sql#predicatePart2}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLikeRegexPredicatePart2(Sql.LikeRegexPredicatePart2Context ctx);
/**
* Visit a parse tree produced by the {@code PostgresRegexPredicatePart2}
* labeled alternative in {@link Sql#predicatePart2}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPostgresRegexPredicatePart2(Sql.PostgresRegexPredicatePart2Context ctx);
/**
* Visit a parse tree produced by the {@code NullPredicatePart2}
* labeled alternative in {@link Sql#predicatePart2}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNullPredicatePart2(Sql.NullPredicatePart2Context ctx);
/**
* Visit a parse tree produced by the {@code QuantifiedComparisonPredicatePart2}
* labeled alternative in {@link Sql#predicatePart2}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQuantifiedComparisonPredicatePart2(Sql.QuantifiedComparisonPredicatePart2Context ctx);
/**
* Visit a parse tree produced by the {@code QuantifiedComparisonSubquery}
* labeled alternative in {@link Sql#quantifiedComparisonPredicatePart3}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQuantifiedComparisonSubquery(Sql.QuantifiedComparisonSubqueryContext ctx);
/**
* Visit a parse tree produced by the {@code QuantifiedComparisonExpr}
* labeled alternative in {@link Sql#quantifiedComparisonPredicatePart3}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQuantifiedComparisonExpr(Sql.QuantifiedComparisonExprContext ctx);
/**
* Visit a parse tree produced by {@link Sql#compOp}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCompOp(Sql.CompOpContext ctx);
/**
* Visit a parse tree produced by the {@code InSubquery}
* labeled alternative in {@link Sql#inPredicateValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInSubquery(Sql.InSubqueryContext ctx);
/**
* Visit a parse tree produced by the {@code InRowValueList}
* labeled alternative in {@link Sql#inPredicateValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInRowValueList(Sql.InRowValueListContext ctx);
/**
* Visit a parse tree produced by {@link Sql#likePattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLikePattern(Sql.LikePatternContext ctx);
/**
* Visit a parse tree produced by {@link Sql#likeEscape}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLikeEscape(Sql.LikeEscapeContext ctx);
/**
* Visit a parse tree produced by {@link Sql#xqueryPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitXqueryPattern(Sql.XqueryPatternContext ctx);
/**
* Visit a parse tree produced by {@link Sql#xqueryOptionFlag}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitXqueryOptionFlag(Sql.XqueryOptionFlagContext ctx);
/**
* Visit a parse tree produced by {@link Sql#postgresRegexOperator}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPostgresRegexOperator(Sql.PostgresRegexOperatorContext ctx);
/**
* Visit a parse tree produced by {@link Sql#quantifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQuantifier(Sql.QuantifierContext ctx);
/**
* Visit a parse tree produced by {@link Sql#searchCondition}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSearchCondition(Sql.SearchConditionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#userString}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUserString(Sql.UserStringContext ctx);
/**
* Visit a parse tree produced by {@link Sql#tableString}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableString(Sql.TableStringContext ctx);
/**
* Visit a parse tree produced by {@link Sql#schemaString}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSchemaString(Sql.SchemaStringContext ctx);
/**
* Visit a parse tree produced by {@link Sql#privilegeString}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPrivilegeString(Sql.PrivilegeStringContext ctx);
/**
* Visit a parse tree produced by the {@code CountStarFunction}
* labeled alternative in {@link Sql#aggregateFunction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCountStarFunction(Sql.CountStarFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code ArrayAggFunction}
* labeled alternative in {@link Sql#aggregateFunction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArrayAggFunction(Sql.ArrayAggFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code SetFunction}
* labeled alternative in {@link Sql#aggregateFunction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetFunction(Sql.SetFunctionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#setFunctionType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetFunctionType(Sql.SetFunctionTypeContext ctx);
/**
* Visit a parse tree produced by {@link Sql#setQuantifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetQuantifier(Sql.SetQuantifierContext ctx);
/**
* Visit a parse tree produced by {@link Sql#sortSpecificationList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSortSpecificationList(Sql.SortSpecificationListContext ctx);
/**
* Visit a parse tree produced by {@link Sql#sortSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSortSpecification(Sql.SortSpecificationContext ctx);
/**
* Visit a parse tree produced by {@link Sql#orderingSpecification}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOrderingSpecification(Sql.OrderingSpecificationContext ctx);
/**
* Visit a parse tree produced by {@link Sql#nullOrdering}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNullOrdering(Sql.NullOrderingContext ctx);
/**
* Visit a parse tree produced by the {@code DmlReturningStatement}
* labeled alternative in {@link Sql#returningStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDmlReturningStatement(Sql.DmlReturningStatementContext ctx);
/**
* Visit a parse tree produced by {@link Sql#deleteStatementSearched}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDeleteStatementSearched(Sql.DeleteStatementSearchedContext ctx);
/**
* Visit a parse tree produced by the {@code DmlStatementValidTimePortion}
* labeled alternative in {@link Sql#dmlStatementValidTimeExtents}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDmlStatementValidTimePortion(Sql.DmlStatementValidTimePortionContext ctx);
/**
* Visit a parse tree produced by the {@code DmlStatementValidTimeAll}
* labeled alternative in {@link Sql#dmlStatementValidTimeExtents}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDmlStatementValidTimeAll(Sql.DmlStatementValidTimeAllContext ctx);
/**
* Visit a parse tree produced by {@link Sql#eraseStatementSearched}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEraseStatementSearched(Sql.EraseStatementSearchedContext ctx);
/**
* Visit a parse tree produced by {@link Sql#insertStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInsertStatement(Sql.InsertStatementContext ctx);
/**
* Visit a parse tree produced by the {@code InsertValues}
* labeled alternative in {@link Sql#insertColumnsAndSource}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInsertValues(Sql.InsertValuesContext ctx);
/**
* Visit a parse tree produced by the {@code InsertRecords}
* labeled alternative in {@link Sql#insertColumnsAndSource}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInsertRecords(Sql.InsertRecordsContext ctx);
/**
* Visit a parse tree produced by the {@code InsertFromSubquery}
* labeled alternative in {@link Sql#insertColumnsAndSource}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInsertFromSubquery(Sql.InsertFromSubqueryContext ctx);
/**
* Visit a parse tree produced by {@link Sql#updateStatementSearched}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUpdateStatementSearched(Sql.UpdateStatementSearchedContext ctx);
/**
* Visit a parse tree produced by {@link Sql#setClauseList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetClauseList(Sql.SetClauseListContext ctx);
/**
* Visit a parse tree produced by {@link Sql#setClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetClause(Sql.SetClauseContext ctx);
/**
* Visit a parse tree produced by {@link Sql#setTarget}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetTarget(Sql.SetTargetContext ctx);
/**
* Visit a parse tree produced by {@link Sql#updateSource}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUpdateSource(Sql.UpdateSourceContext ctx);
/**
* Visit a parse tree produced by the {@code SessionTxCharacteristics}
* labeled alternative in {@link Sql#sessionCharacteristic}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSessionTxCharacteristics(Sql.SessionTxCharacteristicsContext ctx);
/**
* Visit a parse tree produced by the {@code SessionIsolationLevel}
* labeled alternative in {@link Sql#sessionTxMode}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSessionIsolationLevel(Sql.SessionIsolationLevelContext ctx);
/**
* Visit a parse tree produced by the {@code ReadOnlySession}
* labeled alternative in {@link Sql#sessionTxMode}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReadOnlySession(Sql.ReadOnlySessionContext ctx);
/**
* Visit a parse tree produced by the {@code ReadWriteSession}
* labeled alternative in {@link Sql#sessionTxMode}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReadWriteSession(Sql.ReadWriteSessionContext ctx);
/**
* Visit a parse tree produced by {@link Sql#transactionCharacteristics}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTransactionCharacteristics(Sql.TransactionCharacteristicsContext ctx);
/**
* Visit a parse tree produced by the {@code IsolationLevel}
* labeled alternative in {@link Sql#transactionMode}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsolationLevel(Sql.IsolationLevelContext ctx);
/**
* Visit a parse tree produced by the {@code ReadOnlyTransaction}
* labeled alternative in {@link Sql#transactionMode}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReadOnlyTransaction(Sql.ReadOnlyTransactionContext ctx);
/**
* Visit a parse tree produced by the {@code ReadWriteTransaction}
* labeled alternative in {@link Sql#transactionMode}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReadWriteTransaction(Sql.ReadWriteTransactionContext ctx);
/**
* Visit a parse tree produced by the {@code TransactionSystemTime}
* labeled alternative in {@link Sql#transactionMode}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTransactionSystemTime(Sql.TransactionSystemTimeContext ctx);
/**
* Visit a parse tree produced by the {@code ReadUncommittedIsolation}
* labeled alternative in {@link Sql#levelOfIsolation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReadUncommittedIsolation(Sql.ReadUncommittedIsolationContext ctx);
/**
* Visit a parse tree produced by the {@code ReadCommittedIsolation}
* labeled alternative in {@link Sql#levelOfIsolation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReadCommittedIsolation(Sql.ReadCommittedIsolationContext ctx);
/**
* Visit a parse tree produced by the {@code RepeatableReadIsolation}
* labeled alternative in {@link Sql#levelOfIsolation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRepeatableReadIsolation(Sql.RepeatableReadIsolationContext ctx);
/**
* Visit a parse tree produced by the {@code SerializableIsolation}
* labeled alternative in {@link Sql#levelOfIsolation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSerializableIsolation(Sql.SerializableIsolationContext ctx);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy