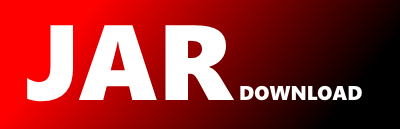
xtdb.api.YamlSerde.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xtdb-core Show documentation
Show all versions of xtdb-core Show documentation
An open source document database with bitemporal graph queries
The newest version!
@file:JvmName("YamlSerde")
package xtdb.api
import com.charleskorn.kaml.*
import kotlinx.serialization.KSerializer
import kotlinx.serialization.decodeFromString
import kotlinx.serialization.descriptors.PrimitiveKind
import kotlinx.serialization.descriptors.PrimitiveSerialDescriptor
import kotlinx.serialization.descriptors.SerialDescriptor
import kotlinx.serialization.descriptors.buildSerialDescriptor
import kotlinx.serialization.descriptors.StructureKind
import kotlinx.serialization.encoding.Decoder
import kotlinx.serialization.encoding.Encoder
import kotlinx.serialization.modules.SerializersModule
import kotlinx.serialization.modules.polymorphic
import kotlinx.serialization.modules.subclass
import xtdb.api.log.Log
import xtdb.api.log.Logs.InMemoryLogFactory
import xtdb.api.log.Logs.LocalLogFactory
import xtdb.api.module.XtdbModule
import xtdb.api.storage.ObjectStore
import xtdb.api.storage.ObjectStore.Factory
import java.nio.file.Path
import java.nio.file.Paths
import java.util.*
import java.util.ServiceLoader.Provider
import kotlin.reflect.KClass
internal object EnvironmentVariableProvider {
fun getEnvVariable(name: String): String? = System.getenv(name)
}
internal fun envFromTaggedNode(taggedNode: YamlTaggedNode ): String {
if (taggedNode.tag == "!Env") {
val value = taggedNode.innerNode.yamlScalar.content
return EnvironmentVariableProvider.getEnvVariable(value) ?: throw IllegalArgumentException("Environment variable '$value' not found")
}
return taggedNode.innerNode.yamlScalar.content
}
internal fun handleEnvTag(input: YamlInput): String {
val currentLocation = input.getCurrentLocation()
val scalar = input.node.yamlMap.entries.values.find { it.location == currentLocation }
return when (scalar) {
is YamlTaggedNode -> envFromTaggedNode(scalar.yamlTaggedNode)
is YamlScalar -> scalar.content
else -> throw IllegalStateException("Expected scalar or tagged node")
}
}
/**
* @suppress
*/
object PathWithEnvVarSerde : KSerializer {
override val descriptor = PrimitiveSerialDescriptor("PathWithEnvVars", PrimitiveKind.STRING)
override fun serialize(encoder: Encoder, value: Path) {
throw UnsupportedOperationException("YAML serialization of config is not supported.")
}
override fun deserialize(decoder: Decoder): Path {
val yamlInput: YamlInput = decoder as YamlInput
val str = handleEnvTag(yamlInput)
return Paths.get(str)
}
}
/**
* @suppress
*/
object StringWithEnvVarSerde : KSerializer {
override val descriptor: SerialDescriptor = PrimitiveSerialDescriptor("StringWithEnvVars", PrimitiveKind.STRING)
override fun serialize(encoder: Encoder, value: String) {
throw UnsupportedOperationException("YAML serialization of config is not supported.")
}
override fun deserialize(decoder: Decoder): String {
val yamlInput: YamlInput = decoder as YamlInput
return handleEnvTag(yamlInput)
}
}
object StringMapWithEnvVarsSerde : KSerializer
© 2015 - 2024 Weber Informatics LLC | Privacy Policy