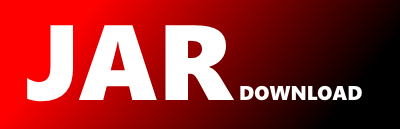
com.yahoo.bard.webservice.table.BaseCompositePhysicalTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fili-core Show documentation
Show all versions of fili-core Show documentation
Fili web service library provides core capabilities for RESTful aggregation navigation, query planning and
metadata
// Copyright 2017 Yahoo Inc.
// Licensed under the terms of the Apache license. Please see LICENSE.md file distributed with this work for terms.
package com.yahoo.bard.webservice.table;
import com.yahoo.bard.webservice.data.config.names.TableName;
import com.yahoo.bard.webservice.data.time.ZonedTimeGrain;
import com.yahoo.bard.webservice.table.availability.Availability;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Map;
import java.util.Set;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import javax.validation.constraints.NotNull;
/**
* An implementation of BasePhysicalTable that contains multiple tables.
*/
public class BaseCompositePhysicalTable extends BasePhysicalTable {
private static final Logger LOG = LoggerFactory.getLogger(BaseCompositePhysicalTable.class);
/**
* Constructor.
*
* @param name Name that represents set of fact table names that are put together under this table
* @param timeGrain The time grain of the table. The time grain has to satisfy all grains of the tables
* @param columns The columns for this table
* @param physicalTables A set of PhysicalTables that are put together under this table. The
* tables shall have zoned time grains that all satisfy the provided timeGrain
* @param logicalToPhysicalColumnNames Mappings from logical to physical names
* @param availability The Availability of this table
*/
public BaseCompositePhysicalTable(
@NotNull TableName name,
@NotNull ZonedTimeGrain timeGrain,
@NotNull Set columns,
@NotNull Set extends PhysicalTable> physicalTables,
@NotNull Map logicalToPhysicalColumnNames,
@NotNull Availability availability
) {
super(
name,
timeGrain,
columns,
logicalToPhysicalColumnNames,
availability
);
verifyGrainSatisfiesAllSourceTables(getSchema().getTimeGrain(), physicalTables);
}
/**
* Verifies that the ZonedTimeGrain satisfies all tables.
*
* @param timeGrain The ZonedTimeGrain being validated
* @param physicalTables A set of PhysicalTables whose ZonedTimeGrains are checked to make sure they all satisfy
* the given ZonedTimeGrain
*
* @throws IllegalArgumentException when the grain is not satisfied by the tables' time grains
*/
private void verifyGrainSatisfiesAllSourceTables(
ZonedTimeGrain timeGrain,
Set extends PhysicalTable> physicalTables
) throws IllegalArgumentException {
Predicate tableDoesNotSatisfy = physicalTable -> !physicalTable.getSchema()
.getTimeGrain()
.satisfies(timeGrain);
Set unsatisfied = physicalTables.stream()
.filter(tableDoesNotSatisfy)
.map(PhysicalTable::getName)
.collect(Collectors.toSet());
if (!unsatisfied.isEmpty()) {
String message = String.format(
"Time grain: '%s' cannot be satisfied by source table(s) %s",
timeGrain,
unsatisfied
);
LOG.error(message);
throw new IllegalArgumentException(message);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy