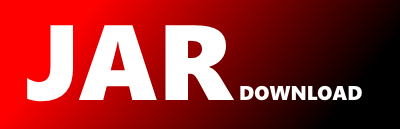
com.yahoo.bard.webservice.util.CacheLastObserver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fili-core Show documentation
Show all versions of fili-core Show documentation
Fili web service library provides core capabilities for RESTful aggregation navigation, query planning and
metadata
// Copyright 2016 Yahoo Inc.
// Licensed under the terms of the Apache license. Please see LICENSE.md file distributed with this work for terms.
package com.yahoo.bard.webservice.util;
import rx.Observer;
import java.util.Optional;
import java.util.concurrent.atomic.AtomicReference;
/**
* A thread-safe observable that caches the last message it received, and caches the last error (if any) it receives.
*
* @param The type of message this Observer caches
*/
public class CacheLastObserver implements Observer {
private AtomicReference lastMessageReceived = new AtomicReference<>();
private AtomicReference error = new AtomicReference<>();
@Override
public void onCompleted() {
//Intentionally left blank.
}
@Override
public void onError(Throwable e) {
error.set(e);
}
@Override
public void onNext(T message) {
lastMessageReceived.set(message);
}
/**
* The last message received by this Observable, if any.
*
* @return the last message received by this Observable, if any
*/
public Optional getLastMessageReceived() {
return Optional.ofNullable(lastMessageReceived.get());
}
/**
* Returns the last error received by this Observable, if any.
*
* @return The last error received by this Observable, if any
*/
public Optional getError() {
return Optional.ofNullable(error.get());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy