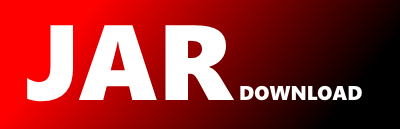
com.yahoo.sketches.theta.SetOperationBuilder Maven / Gradle / Ivy
/*
* Copyright 2015-16, Yahoo! Inc.
* Licensed under the terms of the Apache License 2.0. See LICENSE file at the project root for terms.
*/
package com.yahoo.sketches.theta;
import static com.yahoo.sketches.Util.DEFAULT_NOMINAL_ENTRIES;
import static com.yahoo.sketches.Util.DEFAULT_UPDATE_SEED;
import static com.yahoo.sketches.Util.LS;
import static com.yahoo.sketches.Util.TAB;
import static com.yahoo.sketches.Util.ceilingPowerOf2;
import com.yahoo.memory.Memory;
import com.yahoo.sketches.Family;
import com.yahoo.sketches.ResizeFactor;
import com.yahoo.sketches.SketchesArgumentException;
/**
* For building a new SetOperation.
*
* @author Lee Rhodes
*/
public class SetOperationBuilder {
private int bLgNomLongs;
private long bSeed;
private ResizeFactor bRF;
private float bP;
private Memory bDstMem;
/**
* Constructor for building a new SetOperation. The default configuration is
*
* - Nominal Entries: {@value com.yahoo.sketches.Util#DEFAULT_NOMINAL_ENTRIES}
* - Seed: {@value com.yahoo.sketches.Util#DEFAULT_UPDATE_SEED}
* - {@link ResizeFactor#X8}
* - Input Sampling Probability: 1.0
* - Memory: null
*
*/
public SetOperationBuilder() {
bLgNomLongs = Integer.numberOfTrailingZeros(DEFAULT_NOMINAL_ENTRIES);
bSeed = DEFAULT_UPDATE_SEED;
bP = (float) 1.0;
bRF = ResizeFactor.X8;
bDstMem = null;
}
/**
* Sets the Nominal Entries for this set operation.
* @param nomEntries Nominal Entres
* This will become the ceiling power of 2 if it is not.
* @return this SetOperationBuilder
*/
public SetOperationBuilder setNominalEntries(int nomEntries) {
bLgNomLongs = Integer.numberOfTrailingZeros(ceilingPowerOf2(nomEntries));
return this;
}
/**
* Returns Log-base 2 Nominal Entries
* @return Log-base 2 Nominal Entries
*/
public int getLgNominalEntries() {
return bLgNomLongs;
}
/**
* Sets the long seed value that is require by the hashing function.
* @param seed See seed
* @return this SetOperationBuilder
*/
public SetOperationBuilder setSeed(long seed) {
bSeed = seed;
return this;
}
/**
* Returns the seed
* @return the seed
*/
public long getSeed() {
return bSeed;
}
/**
* Sets the upfront uniform sampling probability, p. Although this functionality is
* implemented for Unions only, it rarely makes sense to use it. The proper use of upfront
* sampling is when building the sketches.
* @param p See Sampling Probability, p
* @return this SetOperationBuilder
*/
public SetOperationBuilder setP(float p) {
if ((p <= 0.0) || (p > 1.0)) {
throw new SketchesArgumentException("p must be > 0 and <= 1.0: " + p);
}
bP = p;
return this;
}
/**
* Returns the pre-sampling probability p
* @return the pre-sampling probability p
*/
public float getP() {
return bP;
}
/**
* Sets the cache Resize Factor
* @param rf See Resize Factor
* @return this SetOperationBuilder
*/
public SetOperationBuilder setResizeFactor(ResizeFactor rf) {
bRF = rf;
return this;
}
/**
* Returns the Resize Factor
* @return the Resize Factor
*/
public ResizeFactor getResizeFactor() {
return bRF;
}
/**
* Initializes the backing Memory store.
* @param dstMem The destination Memory.
* See Destination Memory
* @return this SetOperationBuilder
*/
public SetOperationBuilder initMemory(Memory dstMem) {
bDstMem = dstMem;
return this;
}
/**
* Returns the Destination Memory
* See Destination Memory.
* @return the Destination Memory
*/
public Memory getMemory() {
return bDstMem;
}
/**
* Returns a SetOperation with the current configuration of this Builder and the given Family.
* @param family the chosen SetOperation family
* @return a SetOperation
*/
public SetOperation build(Family family) {
SetOperation setOp = null;
switch (family) {
case UNION: {
if (bDstMem == null) {
setOp = UnionImpl.initNewHeapInstance(bLgNomLongs, bSeed, bP, bRF);
}
else {
setOp = UnionImpl.initNewDirectInstance(bLgNomLongs, bSeed, bP, bRF, bDstMem);
}
break;
}
case INTERSECTION: {
if (bDstMem == null) {
setOp = IntersectionImpl.initNewHeapInstance(bSeed);
}
else {
setOp = IntersectionImpl.initNewDirectInstance(bSeed, bDstMem);
}
break;
}
case A_NOT_B: {
if (bDstMem == null) {
setOp = new HeapAnotB(bSeed);
}
else {
throw new SketchesArgumentException(
"AnotB is a stateless operation and cannot be persisted.");
}
break;
}
default:
throw new SketchesArgumentException(
"Given Family cannot be built as a SetOperation: " + family.toString());
}
return setOp;
}
/**
* Returns a SetOperation with the current configuration of this Builder and the given
* Nominal Entries and Family.
* @param nomEntries Nominal Entres
* This will become the ceiling power of 2 if it is not.
* @param family build this SetOperation family
* @return a SetOperation
*/
public SetOperation build(int nomEntries, Family family) {
bLgNomLongs = Integer.numberOfTrailingZeros(ceilingPowerOf2(nomEntries));
return build(family);
}
/**
* Convenience method, returns a configured SetOperation Union with
* Default Nominal Entries
* @return a Union object
*/
public Union buildUnion() {
return (Union) build(Family.UNION);
}
/**
* Convenience method, returns a configured SetOperation Union with the given
* Nominal Entries.
* @param nomEntries Nominal Entres
* @return a Union object
*/
public Union buildUnion(int nomEntries) {
return (Union) build(nomEntries, Family.UNION);
}
/**
* Convenience method, returns a configured SetOperation Intersection with
* Default Nominal Entries
* @return an Intersection object
*/
public Intersection buildIntersection() {
return (Intersection) build(Family.INTERSECTION);
}
/**
* Convenience method, returns a configured SetOperation ANotB with
* Default Nominal Entries
* @return an ANotB object
*/
public AnotB buildANotB() {
return (AnotB) build(Family.A_NOT_B);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("SetOperationBuilder configuration:").append(LS)
.append("LgK:").append(TAB).append(bLgNomLongs).append(LS)
.append("K:").append(TAB).append(1 << bLgNomLongs).append(LS)
.append("Seed:").append(TAB).append(bSeed).append(LS)
.append("p:").append(TAB).append(bP).append(LS)
.append("ResizeFactor:").append(TAB).append(bRF).append(LS)
.append("DstMemory:").append(TAB).append(bDstMem != null).append(LS);
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy