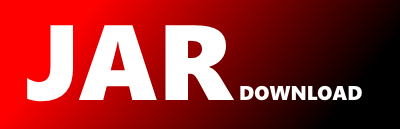
com.yahoo.memory.UnsafeBytesVsShifters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sketches-misc Show documentation
Show all versions of sketches-misc Show documentation
Data Sketches Miscellaneous
The newest version!
/*
* Copyright 2016, Yahoo! Inc. Licensed under the terms of the
* Apache License 2.0. See LICENSE file at the project root for terms.
*/
package com.yahoo.memory;
import static com.yahoo.memory.MemoryPerformance.milliSecToString;
import static com.yahoo.memory.UnsafeUtil.unsafe;
//import static com.yahoo.sketches.TestingUtil.milliSecToString;
import static java.lang.Math.pow;
//import static com.yahoo.sketches.memory.MemoryPerformance.*;
//CHECKSTYLE.OFF: JavadocMethod
//CHECKSTYLE.OFF: WhitespaceAround
public class UnsafeBytesVsShifters {
private int arrLongs_; //# entries in array
private int lgMinTrials_; //minimum # of trials
private int lgMaxTrials_; //maximum # of trials
private int lgMinLongs_; //minimum array longs
private int lgMaxLongs_; //maximum array longs
private double ppo_; //points per octave of arrLongs (x-axis)
private double minGI_; //min generating index
private double maxGI_; //max generating index
private int lgMaxOps_; //lgMaxLongs_ + lgMinTrials_
private long address_ = 0; //used for unsafe
public UnsafeBytesVsShifters() {
//Configure
lgMinTrials_ = 10; //was 6
lgMaxTrials_ = 20; //was 20
lgMinLongs_ = 5; //was 5
lgMaxLongs_ = 20; //was 26
ppo_ = 1.0; //was 4
//Compute
lgMaxOps_ = lgMaxLongs_ + lgMinTrials_;
maxGI_ = ppo_ * lgMaxLongs_;
minGI_ = ppo_ * lgMinLongs_;
}
private static class Point {
double ppo;
double gi;
int arrLongs;
int trials;
long sumReadTrials_nS = 0;
long sumWriteTrials_nS = 0;
Point(double ppo, double gi, int arrLongs, int trials) {
this.ppo = ppo;
this.gi = gi;
this.arrLongs = arrLongs;
this.trials = trials;
}
public static void printHeader() {
println("LgLongs\tLongs\tTrials\t#Ops\tAvgRTrial_nS\tAvgROp_nS\tAvgWTrial_nS\tAvgWOp_nS");
}
public void printRow() {
long numOps = (long)((double)trials * arrLongs);
double logArrLongs = gi/ppo;
double rTrial_nS = (double)sumReadTrials_nS/trials;
double wTrial_nS = (double)sumWriteTrials_nS/trials;
double rOp_nS = rTrial_nS/arrLongs;
double wOp_nS = wTrial_nS/arrLongs;
//Print
String out = String.format("%6.2f\t%d\t%d\t%d\t%.1f\t%8.3f\t%.1f\t%8.3f",
logArrLongs, arrLongs, trials, numOps, rTrial_nS, rOp_nS, wTrial_nS, wOp_nS);
println(out);
}
}
private Point getNextPoint(Point p) {
int lastArrLongs = (int)pow(2.0, p.gi/ppo_);
int nextArrLongs;
double logArrLongs;
do {
logArrLongs = (++p.gi)/ppo_;
if (p.gi > maxGI_) { return null; }
nextArrLongs = (int)pow(2.0, logArrLongs);
} while (nextArrLongs <= lastArrLongs);
p.arrLongs = nextArrLongs;
//compute trials
double logTrials = Math.min(lgMaxOps_- logArrLongs, lgMaxTrials_);
p.trials = (int)pow(2.0, logTrials);
return p;
}
/*************************************/
// UNSAFE BYTE
/*************************************/
private void testUnsafeByte() {
Point p = new Point(ppo_, minGI_-1, 1<>>= 8));
unsafe.putByte(address + j + 2, (byte) (k >>>= 8));
unsafe.putByte(address + j + 3, (byte) (k >>>= 8));
unsafe.putByte(address + j + 4, (byte) (k >>>= 8));
unsafe.putByte(address + j + 5, (byte) (k >>>= 8));
unsafe.putByte(address + j + 6, (byte) (k >>>= 8));
unsafe.putByte(address + j + 7, (byte) (k >>>= 8));
}
stopTime_nS = System.nanoTime();
}
return stopTime_nS - startTime_nS;
}
/*************************************/
// BYTES VIA SHIFTERS
/*************************************/
private void testBytesByShifters() {
Point p = new Point(ppo_, minGI_-1, 1<>>= 8, long0);
long0 = Shifters.putByte2(k >>>= 8, long0);
long0 = Shifters.putByte3(k >>>= 8, long0);
long0 = Shifters.putByte4(k >>>= 8, long0);
long0 = Shifters.putByte5(k >>>= 8, long0);
long0 = Shifters.putByte6(k >>>= 8, long0);
long0 = Shifters.putByte7(k >>>= 8, long0);
unsafe.putLong(address + (i << 3), long0);
}
stopTime_nS = System.nanoTime();
}
return stopTime_nS - startTime_nS;
}
/*************************************/
private final void freeMemory() {
if (address_ != 0) {
unsafe.freeMemory(address_);
address_ = 0L;
}
}
/**
* If the JVM calls this method and a "freeMemory() has not been called" a System.err
* message will be logged.
*/
@Override
protected void finalize() {
if (address_ > 0L) {
System.err.println("ERROR: freeMemory() has not been called: Address: " + address_
+ ", capacity: " + (arrLongs_ << 3));
java.lang.StackTraceElement[] arr = Thread.currentThread().getStackTrace();
for (int i = 0; i < arr.length; i++) {
System.err.println(arr[i].toString());
}
unsafe.freeMemory(address_);
address_ = 0L;
}
}
public void go() {
long startMillis = System.currentTimeMillis();
println("Test Unsafe Byte");
testUnsafeByte();
println("\nTest Bytes By Shifters");
testBytesByShifters();
long testMillis = System.currentTimeMillis() - startMillis;
println("Total Time: "+ milliSecToString(testMillis));
}
//MAIN
public static void main(String[] args) {
UnsafeBytesVsShifters test = new UnsafeBytesVsShifters();
test.go();
}
public static void println(String s) {System.out.println(s); }
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy