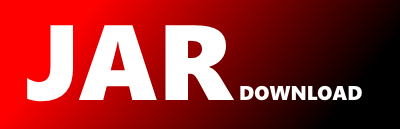
com.yahoo.sketches.benchmark.HllSketchBenchmark Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sketches-misc Show documentation
Show all versions of sketches-misc Show documentation
Data Sketches Miscellaneous
The newest version!
package com.yahoo.sketches.benchmark;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Random;
import com.yahoo.sketches.hll.HllSketch;
import com.yahoo.sketches.hll.HllSketchBuilder;
//CHECKSTYLE.OFF: JavadocMethod
//CHECKSTYLE.OFF: WhitespaceAround
/**
*/
public class HllSketchBenchmark implements SketchBenchmark {
private final String name;
private final Random rand;
private final HllSketchBuilder inputBob;
private final HllSketchBuilder unionBob;
private List sketches;
public HllSketchBenchmark(String name, Random rand, HllSketchBuilder inputBob, HllSketchBuilder unionBob)
{
this.name = name;
this.rand = rand;
this.inputBob = inputBob;
this.unionBob = unionBob;
}
@Override
public void setup(int numSketches, List specs)
{
sketches = new ArrayList<>(numSketches);
for (Spec spec : specs) {
for (int i = 0; i < spec.getNumSketches(); ++i) {
HllSketch sketch = inputBob.build();
for (int j = 0; j < spec.getNumEntries(); ++j) {
sketch.update(new long[]{rand.nextLong()});
}
sketches.add(sketch.asCompact());
}
}
Collections.shuffle(sketches);
}
@Override
public void runNTimes(int n)
{
for (int i = 0; i < n; ++i) {
HllSketch combined = unionBob.build();
for (HllSketch toUnion : sketches) {
combined.union(toUnion);
}
}
}
@Override
public void reset()
{
sketches = null;
}
@Override
public String toString()
{
return name;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy