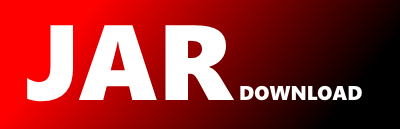
com.yahoo.sketches.performance.Stats Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sketches-misc Show documentation
Show all versions of sketches-misc Show documentation
Data Sketches Miscellaneous
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy