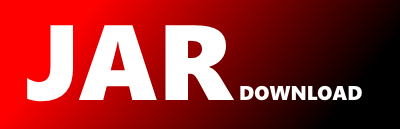
com.yahoo.elide.async.service.dao.DefaultAsyncApiDao Maven / Gradle / Ivy
/*
* Copyright 2020, Yahoo Inc.
* Licensed under the Apache License, Version 2.0
* See LICENSE file in project root for terms.
*/
package com.yahoo.elide.async.service.dao;
import static com.yahoo.elide.core.dictionary.EntityDictionary.NO_VERSION;
import com.yahoo.elide.ElideSettings;
import com.yahoo.elide.async.models.AsyncApi;
import com.yahoo.elide.async.models.AsyncApiResult;
import com.yahoo.elide.async.models.QueryStatus;
import com.yahoo.elide.core.RequestScope;
import com.yahoo.elide.core.datastore.DataStore;
import com.yahoo.elide.core.datastore.DataStoreTransaction;
import com.yahoo.elide.core.filter.expression.FilterExpression;
import com.yahoo.elide.core.request.EntityProjection;
import com.yahoo.elide.core.request.route.Route;
import com.yahoo.elide.jsonapi.JsonApiRequestScope;
import com.yahoo.elide.jsonapi.models.JsonApiDocument;
import jakarta.inject.Singleton;
import lombok.Getter;
import lombok.Setter;
import lombok.extern.slf4j.Slf4j;
import java.io.IOException;
import java.util.Iterator;
import java.util.UUID;
/**
* Utility class which implements AsyncApiDao.
*/
@Singleton
@Slf4j
@Getter
public class DefaultAsyncApiDao implements AsyncApiDao {
@Setter private ElideSettings elideSettings;
@Setter private DataStore dataStore;
// Default constructor is needed for standalone implementation for override in getAsyncApiDao
public DefaultAsyncApiDao() {
}
public DefaultAsyncApiDao(ElideSettings elideSettings, DataStore dataStore) {
this.elideSettings = elideSettings;
this.dataStore = dataStore;
}
@SuppressWarnings("unchecked")
@Override
public T updateStatus(String asyncApiId, QueryStatus status, Class type) {
T queryObj = (T) executeInTransaction(dataStore, (tx, scope) -> {
EntityProjection asyncApiIterable = EntityProjection.builder()
.type(type)
.build();
T query = (T) tx.loadObject(asyncApiIterable, asyncApiId, scope);
query.setStatus(status);
tx.save(query, scope);
return query;
});
return queryObj;
}
@Override
public Iterable updateStatusAsyncApiByFilter(FilterExpression filterExpression,
QueryStatus status, Class type) {
return updateAsyncApiIterable(filterExpression, asyncApi -> asyncApi.setStatus(status), type);
}
/**
* This method updates a Iterable of AsyncApi objects from database and
* returns the objects updated.
* @param filterExpression Filter expression to update AsyncApi Objects based on
* @param updateFunction Functional interface for updating AsyncApi Object
* @param type AsyncApi Type Implementation.
* @return query object list updated
*/
@SuppressWarnings("unchecked")
private Iterable updateAsyncApiIterable(FilterExpression filterExpression,
UpdateQuery updateFunction, Class type) {
log.debug("updateAsyncApiIterable");
Iterable asyncApiList = null;
asyncApiList = (Iterable) executeInTransaction(dataStore,
(tx, scope) -> {
EntityProjection asyncApiIterable = EntityProjection.builder()
.type(type)
.filterExpression(filterExpression)
.build();
Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy