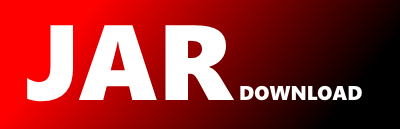
com.yahoo.elide.core.PersistentResourceSet Maven / Gradle / Ivy
/*
* Copyright 2015, Yahoo Inc.
* Licensed under the Apache License, Version 2.0
* See LICENSE file in project root for terms.
*/
package com.yahoo.elide.core;
import com.google.common.collect.UnmodifiableIterator;
import java.util.AbstractSet;
import java.util.Iterator;
import java.util.Spliterator;
import java.util.Spliterators;
/**
* Stream iterable list as a set of PersistentResource.
* @param type of resource
*/
public class PersistentResourceSet extends AbstractSet> {
final private PersistentResource> parent;
final private String parentRelationship;
final private Iterable list;
final private RequestScope requestScope;
public PersistentResourceSet(PersistentResource> parent, String parentRelationship,
Iterable list, RequestScope requestScope) {
this.parent = parent;
this.parentRelationship = parentRelationship;
this.list = list;
this.requestScope = requestScope;
}
public PersistentResourceSet(Iterable list, RequestScope requestScope) {
this(null, null, list, requestScope);
}
@Override
public Iterator> iterator() {
final Iterator iterator = list.iterator();
return new UnmodifiableIterator>() {
@Override
public boolean hasNext() {
return iterator.hasNext();
}
@Override
public PersistentResource next() {
T obj = iterator.next();
return new PersistentResource<>(obj, parent, parentRelationship,
requestScope.getUUIDFor(obj), requestScope);
}
};
}
@Override
public int size() {
throw new UnsupportedOperationException();
}
@Override
public Spliterator> spliterator() {
return Spliterators.spliteratorUnknownSize(iterator(), 0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy