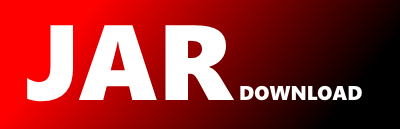
com.yahoo.elide.datastores.multiplex.MultiplexTransaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elide-datastore-multiplex Show documentation
Show all versions of elide-datastore-multiplex Show documentation
Elide entity manager for multiple data store support
/*
* Copyright 2016, Yahoo Inc.
* Licensed under the Apache License, Version 2.0
* See LICENSE file in project root for terms.
*/
package com.yahoo.elide.datastores.multiplex;
import com.yahoo.elide.core.DataStore;
import com.yahoo.elide.core.DataStoreTransaction;
import com.yahoo.elide.core.FilterScope;
import com.yahoo.elide.core.exceptions.InvalidCollectionException;
import com.yahoo.elide.core.filter.Predicate;
import com.yahoo.elide.security.User;
import java.io.IOException;
import java.io.Serializable;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.Set;
/**
* Multiplex transaction handler. Process each sub-database transactions within a single transaction.
* If any commit fails in process, reverse any commits already completed.
*/
public abstract class MultiplexTransaction implements DataStoreTransaction {
protected final LinkedHashMap transactions;
protected final MultiplexManager multiplexManager;
protected final DataStoreTransaction lastDataStoreTransaction;
/**
* Multiplex transaction handler.
* @param multiplexManager associated manager
*/
public MultiplexTransaction(MultiplexManager multiplexManager) {
this.multiplexManager = multiplexManager;
this.transactions = new LinkedHashMap<>(multiplexManager.dataStores.size());
// create each subordinate transaction
DataStoreTransaction transaction = null;
for (DataStore dataStore : multiplexManager.dataStores) {
transaction = beginTransaction(dataStore);
transactions.put(dataStore, transaction);
}
lastDataStoreTransaction = transaction;
}
protected abstract DataStoreTransaction beginTransaction(DataStore dataStore);
@Override
public User accessUser(Object opaqueUser) {
User user = new User(opaqueUser);
for (DataStore dataStore : multiplexManager.dataStores) {
DataStoreTransaction transaction = transactions.get(dataStore);
user = transaction.accessUser(user.getOpaqueUser());
}
return user;
}
@Override
public T createObject(Class createObject) {
return getTransaction(createObject).createObject(createObject);
}
@Override
public T loadObject(Class loadClass, Serializable id) {
return getTransaction(loadClass).loadObject(loadClass, id);
}
@Override
public Iterable loadObjects(Class loadClass) {
return getTransaction(loadClass).loadObjects(loadClass);
}
@Override
public Iterable loadObjects(Class entityClass, FilterScope filterScope) {
return getTransaction(entityClass).loadObjects(entityClass, filterScope);
}
@Override
public Collection filterCollection(Collection collection, Class entityClass, Set predicates) {
return getTransaction(entityClass).filterCollection(collection, entityClass, predicates);
}
@Override
public void flush() {
transactions.values().forEach(DataStoreTransaction::flush);
}
@Override
public void commit() {
// flush all before commit
flush();
transactions.values().forEach(DataStoreTransaction::commit);
transactions.clear();
}
@Override
public void close() throws IOException {
IOException cause = null;
for (DataStoreTransaction transaction : transactions.values()) {
try {
transaction.close();
} catch (IOException | Error | RuntimeException e) {
if (cause != null) {
cause.addSuppressed(e);
} else if (e instanceof IOException) {
cause = (IOException) e;
} else {
cause = new IOException(e);
}
}
}
if (cause != null) {
throw cause;
}
}
protected DataStoreTransaction getTransaction(Object object) {
return getTransaction(object.getClass());
}
protected DataStoreTransaction getTransaction(Class> cls) {
DataStoreTransaction transaction = transactions.get(this.multiplexManager.getSubManager(cls));
if (transaction == null) {
Class entityClass = multiplexManager.getDictionary().lookupEntityClass(cls);
throw new InvalidCollectionException(entityClass == null ? cls.getName() : entityClass.getName());
}
return transaction;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy