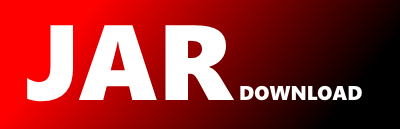
com.yahoo.elide.datastores.multiplex.MultiplexWriteTransaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elide-datastore-multiplex Show documentation
Show all versions of elide-datastore-multiplex Show documentation
Elide entity manager for multiple data store support
/*
* Copyright 2015, Yahoo Inc.
* Licensed under the Apache License, Version 2.0
* See LICENSE file in project root for terms.
*/
package com.yahoo.elide.datastores.multiplex;
import com.yahoo.elide.core.DataStore;
import com.yahoo.elide.core.DataStoreTransaction;
import com.yahoo.elide.core.exceptions.TransactionException;
import com.google.common.collect.Lists;
import java.io.IOException;
import java.io.Serializable;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Collections;
import java.util.IdentityHashMap;
import java.util.List;
import java.util.Map.Entry;
import javax.ws.rs.core.MultivaluedHashMap;
/**
* Multiplex transaction handler.
*/
public class MultiplexWriteTransaction extends MultiplexTransaction {
private static final Object NEWLY_CREATED_OBJECT = new Object();
private final IdentityHashMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy