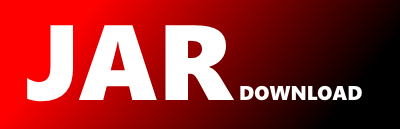
com.yahoo.maha.parrequest2.CollectionsUtil Maven / Gradle / Ivy
// Copyright 2017, Yahoo Holdings Inc.
// Licensed under the terms of the Apache License 2.0. Please see LICENSE file in project root for terms.
package com.yahoo.maha.parrequest2;
import org.apache.commons.lang3.ObjectUtils;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.PriorityQueue;
import scala.Tuple2;
/**
* Created by hiral on 6/26/14.
*/
public class CollectionsUtil {
public static List emptyList() {
return (List) Collections.emptyList();
}
public static Map emptyMap() {
return (Map) Collections.emptyMap();
}
public static > Map sortMapByValue(Map map) {
List> list = new LinkedList<>(map.entrySet());
Collections.sort(list, new Comparator>() {
public int compare(Map.Entry e1, Map.Entry e2) {
return ObjectUtils.compare(e1.getValue(), e2.getValue());
}
});
Map result = new LinkedHashMap<>();
for (Map.Entry entry : list) {
result.put(entry.getKey(), entry.getValue());
}
return result;
}
public static Map getTopNByValue(Map map, int topN, final Comparator comparator) {
Map result = new LinkedHashMap<>();
if (topN <= 0) {
return result;
}
// min heap
PriorityQueue> queue = new PriorityQueue<>(topN, new Comparator>() {
public int compare(Map.Entry e1, Map.Entry e2) {
return (-1) * comparator.compare(e1.getValue(), e2.getValue());
}
});
for (Map.Entry entry : map.entrySet()) {
if (queue.size() < topN) {
queue.offer(entry);
} else if (comparator.compare(queue.peek().getValue(), entry.getValue()) > 0) {
queue.poll();
queue.offer(entry);
}
}
List> list = new ArrayList<>();
while (!queue.isEmpty()) {
Map.Entry entry = queue.poll();
list.add(entry);
}
// reverse
for (int i = list.size() - 1; i >= 0; i--) {
result.put(list.get(i).getKey(), list.get(i).getValue());
}
return result;
}
public static Map sortMapByValue(Map map, final Comparator comparator) {
List> list = new LinkedList<>(map.entrySet());
Collections.sort(list, new Comparator>() {
public int compare(Map.Entry e1, Map.Entry e2) {
return comparator.compare(e1.getValue(), e2.getValue());
}
});
Map result = new LinkedHashMap<>();
for (Map.Entry entry : list) {
result.put(entry.getKey(), entry.getValue());
}
return result;
}
public static Map> combine2Map(Map map1, Map map2) {
HashSet combinedKeySet = new HashSet();
Map> combinedMap = new HashMap<>();
if (map1 != null && !map1.isEmpty()) {
combinedKeySet.addAll(map1.keySet());
}
if (map2 != null && !map2.isEmpty()) {
combinedKeySet.addAll(map2.keySet());
}
for (K key : combinedKeySet) {
V1 val1 = null;
if (map1 != null) {
val1 = map1.get(key);
}
V2 val2 = null;
if (map2 != null) {
val2 = map2.get(key);
}
combinedMap.put(key, new Tuple2(val1, val2));
}
return combinedMap;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy