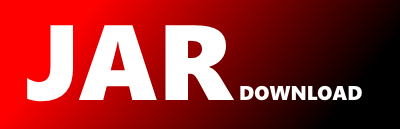
com.yahoo.maha.parrequest2.EitherUtils Maven / Gradle / Ivy
package com.yahoo.maha.parrequest2;
import com.google.common.base.Preconditions;
import scala.Function1;
import scala.Option;
import scala.util.Either;
import scala.util.Right;
import java.util.function.Function;
public class EitherUtils {
/**
* Casts the leftEither from to , meaning it is of type E already and not A or B
*/
public static Either castLeft(Either leftEither) {
Preconditions.checkArgument(leftEither.isLeft());
return (Either) leftEither;
}
/**
* Maps either by applying function doWithRight if the Either isRight(), otherwise returns the input either with
* cast to
*/
public static Either flatMap(Function> doWithRight, Either either) {
if (either.isRight()) {
return doWithRight.apply(either.right().get());
} else {
return ((Either) either);
}
}
/**
* Converts Either to T by applying the right function or left function depending on if it isRight or isLeft
*/
public static T fold(Function doWithLeft, Function doWithRight, Either either) {
if (either.isLeft()) {
return doWithLeft.apply(either.left().get());
} else {
return doWithRight.apply(either.right().get());
}
}
/**
* Converts Either to T by applying the right function or left function depending on if it isRight or isLeft
*/
public static T fold(Function1 doWithLeft, Function doWithRight, Either either) {
if (either.isLeft()) {
return doWithLeft.apply(either.left().get());
} else {
return doWithRight.apply(either.right().get());
}
}
/**
* Maps either by applying function doWithRight if the Either isRight(), otherwise, it passes the Either through
* with cast to
*/
public static Either map(Function doWithRight, Either either) {
if (either.isRight()) {
return new Right<>(doWithRight.apply(either.right().get()));
} else {
return ((Either) either);
}
}
/**
* If option is defined, applies doWithOption function, else returns Option.none()
*/
final public static Option map(Function doWithOption, Option option) {
if (option.isDefined()) {
T t = option.get();
return Option.apply(doWithOption.apply(t));
} else {
return Option.empty();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy