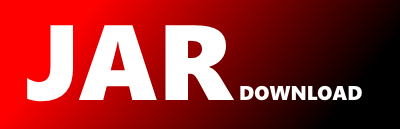
com.yahoo.parsec.clients.ParsecHttpUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parsec-clients Show documentation
Show all versions of parsec-clients Show documentation
The Parsec Java Libraries.
// Copyright 2016 Yahoo Inc.
// Licensed under the terms of the Apache license. Please see LICENSE.md file distributed with this work for terms.
package com.yahoo.parsec.clients;
import com.ning.http.client.Param;
import com.ning.http.client.cookie.Cookie;
import javax.ws.rs.core.NewCookie;
import javax.ws.rs.core.Response;
import java.io.IOException;
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* Utility class that helps convert Ning objects to {@link javax.ws.rs.core} objects.
*
* @author sho
*/
public final class ParsecHttpUtil {
/**
* Unused private constructor.
*/
private ParsecHttpUtil() {
}
/**
* Get {@link NewCookie} {@link List} from Ning {@link Cookie} {@link Collection}.
*
* @param ningCookies Ning {@link Cookie} {@link Collection}
* @return List<{@link NewCookie}>
*/
public static List getCookies(final Collection ningCookies) {
Stream s = ningCookies.stream().map(ParsecHttpUtil::getCookie);
return s.collect(Collectors.toList());
}
/**
* Get {@link NewCookie} from Ning {@link Cookie}.
*
* @param ningCookie Ning {@link Cookie}
* @return {@link NewCookie}
*/
public static NewCookie getCookie(final Cookie ningCookie) {
return new NewCookie(
ningCookie.getName(),
ningCookie.getValue(),
ningCookie.getPath(),
ningCookie.getDomain(),
"",
(int) ningCookie.getMaxAge(),
ningCookie.isSecure(),
ningCookie.isHttpOnly()
);
}
/**
* Get Map<String, List<String>> from Ning {@link Param} {@link List}.
*
* @param ningParams Ning {@link Param} {@link List}
* @return Map<String, List<String>>
*/
public static Map> getParamsMap(final List ningParams) {
Map> params = new HashMap<>();
for (Param ningParam : ningParams) {
String paramKey = ningParam.getName();
List paramValues = (params.containsKey(paramKey)) ? params.get(paramKey) : new ArrayList<>();
paramValues.add(ningParam.getValue());
params.put(paramKey, paramValues);
}
return params;
}
/**
* Get {@link javax.ws.rs.core} {@link Response} from Ning {@link com.ning.http.client.Response}.
*
* @param ningResponse Ning {@link com.ning.http.client.Response}
* @return {@link Response}
* @throws IOException IO exception
*/
public static Response getResponse(final com.ning.http.client.Response ningResponse) throws IOException {
Response.ResponseBuilder responseBuilder = Response
.status(ningResponse.getStatusCode())
.type(ningResponse.getContentType());
if (ningResponse.hasResponseHeaders()) {
ningResponse.getHeaders().entrySet()
.forEach(entry -> entry.getValue()
.forEach(value -> responseBuilder.header(entry.getKey(), value)));
}
if (ningResponse.hasResponseBody()) {
responseBuilder.entity(ningResponse.getResponseBody());
}
ningResponse.getCookies().forEach(ningCookie -> responseBuilder.cookie(getCookie(ningCookie)));
return responseBuilder.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy