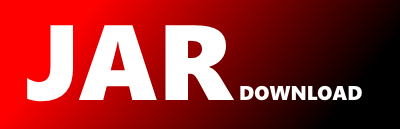
com.yahoo.config.InnerNode Maven / Gradle / Ivy
// Copyright Vespa.ai. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
package com.yahoo.config;
import java.lang.reflect.Field;
import java.util.Collection;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Objects;
/**
* Superclass for all inner nodes in a {@link ConfigInstance}.
*
* This class cannot have non-private members because such members
* will interfere with the members in the generated subclass.
*
* @author gjoranv
*/
public abstract class InnerNode extends Node {
/**
* Creates a new InnerNode.
*/
public InnerNode() {
}
@Override
public String toString() {
return mkString(ConfigInstance.serialize(this), "\n");
}
private static String mkString(Collection collection, String sep) {
StringBuilder sb = new StringBuilder();
boolean first = true;
for (T elem : collection) {
if (! first)
sb.append(sep);
first = false;
sb.append(elem);
}
return sb.toString();
}
/**
* Overrides {@link Node#postInitialize(String)}.
* Perform post initialization on this node's children.
*
* @param configId The config id of this instance.
*/
@Override
public void postInitialize(String configId) {
Map children = getChildrenWithVectorsFlattened();
for (Node node : children.values()) {
node.postInitialize(configId);
}
}
@Override
public boolean equals(Object other) {
if (other == this)
return true;
if ( !(other instanceof InnerNode) || (other.getClass() != this.getClass()))
return false;
/* This implementation requires getChildren() to return elements in order.
Hence, we should make it final. Or make equals independent of order. */
Collection