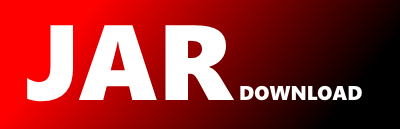
com.yahoo.config.LeafNodeMaps Maven / Gradle / Ivy
The newest version!
// Copyright Vespa.ai. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
package com.yahoo.config;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
/**
* @author gjoranv
*/
public class LeafNodeMaps {
/**
* Converts a map of String→NODE to String→REAL, where REAL is the underlying value type.
*/
public static , REAL>
Map asValueMap(Map input)
{
Map ret = new LinkedHashMap<>();
for(String key : input.keySet()) {
ret.put(key, input.get(key).value());
}
return Collections.unmodifiableMap(ret);
}
/**
* Converts a map of String→REAL to String→NODE, where REAL is the underlying value type.
*/
@SuppressWarnings("unchecked")
public static , REAL>
Map asNodeMap(Map input, NODE defaultNode)
{
Map ret = new LinkedHashMap<>();
for(String key : input.keySet()) {
NODE node = (NODE)defaultNode.clone();
node.value = input.get(key);
ret.put(key, node);
}
return Collections.unmodifiableMap(ret);
}
/**
* Special case for file type, since FileNode param type (FileReference) is not same as type (String) in config builder
*/
public static Map asFileNodeMap(Map stringMap) {
Map fileNodeMap = new LinkedHashMap<>();
for (Map.Entry e : stringMap.entrySet()) {
fileNodeMap.put(e.getKey(), new FileNode(e.getValue()));
}
return Collections.unmodifiableMap(fileNodeMap);
}
public static Map asPathNodeMap(Map fileReferenceMap) {
Map pathNodeMap = new LinkedHashMap<>();
for (Map.Entry e : fileReferenceMap.entrySet()) {
pathNodeMap.put(e.getKey(), new PathNode(e.getValue()));
}
return Collections.unmodifiableMap(pathNodeMap);
}
public static Map asOptionalPathNodeMap(Map> fileReferenceMap) {
Map pathNodeMap = new LinkedHashMap<>();
for (Map.Entry> e : fileReferenceMap.entrySet()) {
pathNodeMap.put(e.getKey(), new OptionalPathNode(e.getValue()));
}
return Collections.unmodifiableMap(pathNodeMap);
}
public static Map asUrlNodeMap(Map urlReferenceMap) {
return Collections.unmodifiableMap(
urlReferenceMap.entrySet().stream().collect(
Collectors.toMap(Map.Entry::getKey, e -> new UrlNode(e.getValue()))
));
}
public static Map asModelNodeMap(Map modelReferenceMap) {
return Collections.unmodifiableMap(
modelReferenceMap.entrySet().stream().collect(
Collectors.toMap(Map.Entry::getKey, e -> new ModelNode(e.getValue()))
));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy