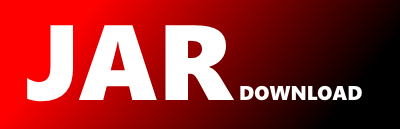
com.yahoo.config.LeafNodeVector Maven / Gradle / Ivy
The newest version!
// Copyright Vespa.ai. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
package com.yahoo.config;
import java.io.File;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
/**
* A vector of leaf nodes.
*
* @author gjoranv
*/
public class LeafNodeVector> extends NodeVector {
private final List realValues;
// TODO: take class instead of default node
public LeafNodeVector(List values, NODE defaultNode) {
assert (defaultNode != null) : "The default node cannot be null";
if (createNew(defaultNode) == null) {
throw new NullPointerException("Unable to duplicate the default node.");
}
for (REAL value : values) {
NODE node = createNew(defaultNode);
node.value = value;
vector.add(node);
}
realValues = realList(vector);
}
public List asList() {
return realValues;
}
/**
* Creates a new Node by cloning the default node.
*/
@SuppressWarnings("unchecked")
private static > NODE createNew(NODE defaultNode) {
return (NODE) (defaultNode).clone();
}
private static> List realList(List nodes) {
List reals = new ArrayList<>();
for(NODE node : nodes) {
reals.add(node.value());
}
return Collections.unmodifiableList(reals);
}
// TODO: Try to eliminate the need for this method when we have moved FileAcquirer to the config library
// It is needed now because the builder has a list of String, while REAL=FileReference.
public static LeafNodeVector createFileNodeVector(Collection values) {
List fileReferences = new ArrayList<>();
for (String s : values)
fileReferences.add(new FileReference(ReferenceNode.stripQuotes(s)));
return new LeafNodeVector<>(fileReferences, new FileNode());
}
public static LeafNodeVector createPathNodeVector(Collection values) {
List paths = new ArrayList<>();
for (FileReference fileReference : values)
paths.add(Paths.get(fileReference.value()));
return new LeafNodeVector<>(paths, new PathNode());
}
public static LeafNodeVector, OptionalPathNode> createOptionalPathNodeVector(Collection> values) {
List> paths = new ArrayList<>();
for (Optional fileReference : values)
paths.add(fileReference.map(reference -> Paths.get(reference.value())));
return new LeafNodeVector<>(paths, new OptionalPathNode());
}
public static LeafNodeVector createUrlNodeVector(Collection values) {
List files = new ArrayList<>();
for (UrlReference urlReference : values)
files.add(new File(urlReference.value()));
return new LeafNodeVector<>(files, new UrlNode());
}
public static LeafNodeVector createModelNodeVector(Collection values) {
List modelPaths = new ArrayList<>();
for (ModelReference modelReference : values)
modelPaths.add(modelReference.value());
return new LeafNodeVector<>(modelPaths, new ModelNode());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy