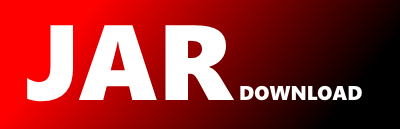
com.yahoo.vespa.model.container.component.ConfigProducerGroup Maven / Gradle / Ivy
// Copyright Vespa.ai. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
package com.yahoo.vespa.model.container.component;
import com.yahoo.component.ComponentId;
import com.yahoo.config.model.producer.AnyConfigProducer;
import com.yahoo.config.model.producer.TreeConfigProducer;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
/**
* A group of config producers that have a component id.
*
* @author Tony Vaagenes
*/
public class ConfigProducerGroup extends TreeConfigProducer {
private final Map producerById = new LinkedHashMap<>();
public ConfigProducerGroup(TreeConfigProducer super ConfigProducerGroup> parent, String subId) {
super(parent, subId);
}
public void addComponent(ComponentId id, CHILD producer) {
CHILD existing = producerById.put(id, producer);
if ( existing != null) {
throw new IllegalArgumentException("Both " + producer + " and " + existing + " are configured" +
" with the id '" + id + "'. All components must have a unique id.");
}
addChild(producer);
}
/**
* Removes a component by id
*
* @return the removed component, or null if it was not present
*/
public CHILD removeComponent(ComponentId componentId) {
CHILD component = producerById.remove(componentId);
if (component == null) return null;
removeChild(component);
return component;
}
public Collection getComponents() {
return Collections.unmodifiableCollection(getChildren().values());
}
public Collection getComponents(Class componentClass) {
return getChildren().values().stream()
.filter(componentClass::isInstance)
.map(componentClass::cast)
.sorted() // We need consistent ordering
.toList();
}
/** Returns a map of all components in this group, with (local) component ID as key. */
public Map getComponentMap() {
return Collections.unmodifiableMap(producerById);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy