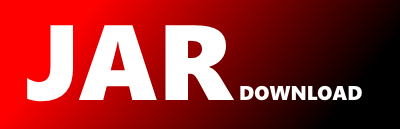
com.yahoo.container.core.ApplicationMetadataConfig Maven / Gradle / Ivy
/**
* This file is generated from a config definition file.
* ------------ D O N O T E D I T ! ------------
*/
package com.yahoo.container.core;
import java.util.*;
import java.nio.file.Path;
import edu.umd.cs.findbugs.annotations.NonNull;
import com.yahoo.config.*;
/**
* This class represents the root node of application-metadata
*
* Copyright 2017 Yahoo Holdings. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
* Contains meta info about one deployed application
*/
public final class ApplicationMetadataConfig extends ConfigInstance {
public final static String CONFIG_DEF_MD5 = "3cc681c3609eeb8d0f6748c90da39db0";
public final static String CONFIG_DEF_NAME = "application-metadata";
public final static String CONFIG_DEF_NAMESPACE = "container.core";
public final static String CONFIG_DEF_VERSION = "";
public final static String[] CONFIG_DEF_SCHEMA = {
"namespace=container.core",
"name string default=\"\"",
"user string default=\"\"",
"path string default=\"\"",
"timestamp long default=0",
"checksum string default=\"\"",
"generation long default=0"
};
public static String getDefMd5() { return CONFIG_DEF_MD5; }
public static String getDefName() { return CONFIG_DEF_NAME; }
public static String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
public static String getDefVersion() { return CONFIG_DEF_VERSION; }
public interface Producer extends ConfigInstance.Producer {
void getConfig(Builder builder);
}
public static class Builder implements ConfigInstance.Builder {
private Set __uninitialized = new HashSet();
private String name = null;
private String user = null;
private String path = null;
private Long timestamp = null;
private String checksum = null;
private Long generation = null;
public Builder() { }
public Builder(ApplicationMetadataConfig config) {
name(config.name());
user(config.user());
path(config.path());
timestamp(config.timestamp());
checksum(config.checksum());
generation(config.generation());
}
private Builder override(Builder __superior) {
if (__superior.name != null)
name(__superior.name);
if (__superior.user != null)
user(__superior.user);
if (__superior.path != null)
path(__superior.path);
if (__superior.timestamp != null)
timestamp(__superior.timestamp);
if (__superior.checksum != null)
checksum(__superior.checksum);
if (__superior.generation != null)
generation(__superior.generation);
return this;
}
public Builder name(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
name = __value;
return this;
}
public Builder user(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
user = __value;
return this;
}
public Builder path(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
path = __value;
return this;
}
public Builder timestamp(long __value) {
timestamp = __value;
return this;
}
private Builder timestamp(String __value) {
return timestamp(Long.valueOf(__value));
}
public Builder checksum(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
checksum = __value;
return this;
}
public Builder generation(long __value) {
generation = __value;
return this;
}
private Builder generation(String __value) {
return generation(Long.valueOf(__value));
}
@java.lang.Override
public final boolean dispatchGetConfig(ConfigInstance.Producer producer) {
if (producer instanceof Producer) {
((Producer)producer).getConfig(this);
return true;
}
return false;
}
@java.lang.Override
public final String getDefMd5() { return CONFIG_DEF_MD5; }
@java.lang.Override
public final String getDefName() { return CONFIG_DEF_NAME; }
@java.lang.Override
public final String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
}
// The name of the directory that contained the application package
private final StringNode name;
// The user name that deployed the application
private final StringNode user;
// The directory the application was deployed from
private final StringNode path;
// The application timestamp in ms
private final LongNode timestamp;
// The md5 hash of the application package contents
private final StringNode checksum;
// The application generation number
private final LongNode generation;
public ApplicationMetadataConfig(Builder builder) {
this(builder, true);
}
private ApplicationMetadataConfig(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"application-metadata must be initialized: " + builder.__uninitialized);
name = (builder.name == null) ?
new StringNode("") : new StringNode(builder.name);
user = (builder.user == null) ?
new StringNode("") : new StringNode(builder.user);
path = (builder.path == null) ?
new StringNode("") : new StringNode(builder.path);
timestamp = (builder.timestamp == null) ?
new LongNode(0L) : new LongNode(builder.timestamp);
checksum = (builder.checksum == null) ?
new StringNode("") : new StringNode(builder.checksum);
generation = (builder.generation == null) ?
new LongNode(0L) : new LongNode(builder.generation);
}
/**
* @return application-metadata.name
*/
public String name() {
return name.value();
}
/**
* @return application-metadata.user
*/
public String user() {
return user.value();
}
/**
* @return application-metadata.path
*/
public String path() {
return path.value();
}
/**
* @return application-metadata.timestamp
*/
public long timestamp() {
return timestamp.value();
}
/**
* @return application-metadata.checksum
*/
public String checksum() {
return checksum.value();
}
/**
* @return application-metadata.generation
*/
public long generation() {
return generation.value();
}
private ChangesRequiringRestart getChangesRequiringRestart(ApplicationMetadataConfig newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("application-metadata");
return changes;
}
private static boolean containsFieldsFlaggedWithRestart() {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy