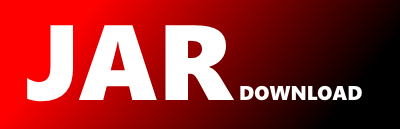
com.yahoo.container.core.QrTemplatesConfig Maven / Gradle / Ivy
/**
* This file is generated from a config definition file.
* ------------ D O N O T E D I T ! ------------
*/
package com.yahoo.container.core;
import java.util.*;
import java.nio.file.Path;
import edu.umd.cs.findbugs.annotations.NonNull;
import com.yahoo.config.*;
/**
* This class represents the root node of qr-templates
*
* Copyright 2017 Yahoo Holdings. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
* Not used
* TODO: Remove on Vespa 7
*/
public final class QrTemplatesConfig extends ConfigInstance {
public final static String CONFIG_DEF_MD5 = "9bc133313acf8166384e0f39484f8710";
public final static String CONFIG_DEF_NAME = "qr-templates";
public final static String CONFIG_DEF_NAMESPACE = "container.core";
public final static String CONFIG_DEF_VERSION = "";
public final static String[] CONFIG_DEF_SCHEMA = {
"namespace=container.core",
"templateset[].urlprefix string",
"templateset[].classid string default=\"\"",
"templateset[].bundle string default=\"\"",
"templateset[].mimetype string default=\"text/html\"",
"templateset[].encoding string default=\"iso-8859-1\"",
"templateset[].rankprofile int default=0",
"templateset[].keepalive bool default=false",
"templateset[].headertemplate string default=\"\"",
"templateset[].footertemplate string default=\"\"",
"templateset[].nohitstemplate string default=\"\"",
"templateset[].hittemplate string default=\"\"",
"templateset[].errortemplate string default=\"\"",
"templateset[].groupsheadertemplate string default=\"[DEFAULT]\"",
"templateset[].rangegrouptemplate string default=\"[DEFAULT]\"",
"templateset[].exactgrouptemplate string default=\"[DEFAULT]\"",
"templateset[].groupsfootertemplate string default=\"[DEFAULT]\"",
"templateset[].highlightstarttag string default=\"\"",
"templateset[].highlightendtag string default=\"\"",
"templateset[].highlightseptag string default=\"\"",
"templateset[].defaultsummaryclass string default=\"\""
};
public static String getDefMd5() { return CONFIG_DEF_MD5; }
public static String getDefName() { return CONFIG_DEF_NAME; }
public static String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
public static String getDefVersion() { return CONFIG_DEF_VERSION; }
public interface Producer extends ConfigInstance.Producer {
void getConfig(Builder builder);
}
public static class Builder implements ConfigInstance.Builder {
private Set __uninitialized = new HashSet();
public List templateset = new ArrayList<>();
public Builder() { }
public Builder(QrTemplatesConfig config) {
for (Templateset t : config.templateset()) {
templateset(new Templateset.Builder(t));
}
}
private Builder override(Builder __superior) {
if (!__superior.templateset.isEmpty())
templateset.addAll(__superior.templateset);
return this;
}
/**
* Add the given builder to this builder's list of Templateset builders
* @param __builder a builder
* @return this builder
*/
public Builder templateset(Templateset.Builder __builder) {
templateset.add(__builder);
return this;
}
/**
* Set the given list as this builder's list of Templateset builders
* @param __builders a list of builders
* @return this builder
*/
public Builder templateset(List __builders) {
templateset = __builders;
return this;
}
@java.lang.Override
public final boolean dispatchGetConfig(ConfigInstance.Producer producer) {
if (producer instanceof Producer) {
((Producer)producer).getConfig(this);
return true;
}
return false;
}
@java.lang.Override
public final String getDefMd5() { return CONFIG_DEF_MD5; }
@java.lang.Override
public final String getDefName() { return CONFIG_DEF_NAME; }
@java.lang.Override
public final String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
}
private final InnerNodeVector templateset;
public QrTemplatesConfig(Builder builder) {
this(builder, true);
}
private QrTemplatesConfig(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"qr-templates must be initialized: " + builder.__uninitialized);
templateset = Templateset.createVector(builder.templateset);
}
/**
* @return qr-templates.templateset[]
*/
public List templateset() {
return templateset;
}
/**
* @param i the index of the value to return
* @return qr-templates.templateset[]
*/
public Templateset templateset(int i) {
return templateset.get(i);
}
private ChangesRequiringRestart getChangesRequiringRestart(QrTemplatesConfig newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("qr-templates");
return changes;
}
private static boolean containsFieldsFlaggedWithRestart() {
return false;
}
/**
* This class represents qr-templates.templateset[]
*/
public final static class Templateset extends InnerNode {
public static class Builder implements ConfigBuilder {
private Set __uninitialized = new HashSet(Arrays.asList(
"urlprefix"
));
private String urlprefix = null;
private String classid = null;
private String bundle = null;
private String mimetype = null;
private String encoding = null;
private Integer rankprofile = null;
private Boolean keepalive = null;
private String headertemplate = null;
private String footertemplate = null;
private String nohitstemplate = null;
private String hittemplate = null;
private String errortemplate = null;
private String groupsheadertemplate = null;
private String rangegrouptemplate = null;
private String exactgrouptemplate = null;
private String groupsfootertemplate = null;
private String highlightstarttag = null;
private String highlightendtag = null;
private String highlightseptag = null;
private String defaultsummaryclass = null;
public Builder() { }
public Builder(Templateset config) {
urlprefix(config.urlprefix());
classid(config.classid());
bundle(config.bundle());
mimetype(config.mimetype());
encoding(config.encoding());
rankprofile(config.rankprofile());
keepalive(config.keepalive());
headertemplate(config.headertemplate());
footertemplate(config.footertemplate());
nohitstemplate(config.nohitstemplate());
hittemplate(config.hittemplate());
errortemplate(config.errortemplate());
groupsheadertemplate(config.groupsheadertemplate());
rangegrouptemplate(config.rangegrouptemplate());
exactgrouptemplate(config.exactgrouptemplate());
groupsfootertemplate(config.groupsfootertemplate());
highlightstarttag(config.highlightstarttag());
highlightendtag(config.highlightendtag());
highlightseptag(config.highlightseptag());
defaultsummaryclass(config.defaultsummaryclass());
}
private Builder override(Builder __superior) {
if (__superior.urlprefix != null)
urlprefix(__superior.urlprefix);
if (__superior.classid != null)
classid(__superior.classid);
if (__superior.bundle != null)
bundle(__superior.bundle);
if (__superior.mimetype != null)
mimetype(__superior.mimetype);
if (__superior.encoding != null)
encoding(__superior.encoding);
if (__superior.rankprofile != null)
rankprofile(__superior.rankprofile);
if (__superior.keepalive != null)
keepalive(__superior.keepalive);
if (__superior.headertemplate != null)
headertemplate(__superior.headertemplate);
if (__superior.footertemplate != null)
footertemplate(__superior.footertemplate);
if (__superior.nohitstemplate != null)
nohitstemplate(__superior.nohitstemplate);
if (__superior.hittemplate != null)
hittemplate(__superior.hittemplate);
if (__superior.errortemplate != null)
errortemplate(__superior.errortemplate);
if (__superior.groupsheadertemplate != null)
groupsheadertemplate(__superior.groupsheadertemplate);
if (__superior.rangegrouptemplate != null)
rangegrouptemplate(__superior.rangegrouptemplate);
if (__superior.exactgrouptemplate != null)
exactgrouptemplate(__superior.exactgrouptemplate);
if (__superior.groupsfootertemplate != null)
groupsfootertemplate(__superior.groupsfootertemplate);
if (__superior.highlightstarttag != null)
highlightstarttag(__superior.highlightstarttag);
if (__superior.highlightendtag != null)
highlightendtag(__superior.highlightendtag);
if (__superior.highlightseptag != null)
highlightseptag(__superior.highlightseptag);
if (__superior.defaultsummaryclass != null)
defaultsummaryclass(__superior.defaultsummaryclass);
return this;
}
public Builder urlprefix(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
urlprefix = __value;
__uninitialized.remove("urlprefix");
return this;
}
public Builder classid(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
classid = __value;
return this;
}
public Builder bundle(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
bundle = __value;
return this;
}
public Builder mimetype(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
mimetype = __value;
return this;
}
public Builder encoding(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
encoding = __value;
return this;
}
public Builder rankprofile(int __value) {
rankprofile = __value;
return this;
}
private Builder rankprofile(String __value) {
return rankprofile(Integer.valueOf(__value));
}
public Builder keepalive(boolean __value) {
keepalive = __value;
return this;
}
private Builder keepalive(String __value) {
return keepalive(Boolean.valueOf(__value));
}
public Builder headertemplate(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
headertemplate = __value;
return this;
}
public Builder footertemplate(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
footertemplate = __value;
return this;
}
public Builder nohitstemplate(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
nohitstemplate = __value;
return this;
}
public Builder hittemplate(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
hittemplate = __value;
return this;
}
public Builder errortemplate(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
errortemplate = __value;
return this;
}
public Builder groupsheadertemplate(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
groupsheadertemplate = __value;
return this;
}
public Builder rangegrouptemplate(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
rangegrouptemplate = __value;
return this;
}
public Builder exactgrouptemplate(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
exactgrouptemplate = __value;
return this;
}
public Builder groupsfootertemplate(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
groupsfootertemplate = __value;
return this;
}
public Builder highlightstarttag(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
highlightstarttag = __value;
return this;
}
public Builder highlightendtag(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
highlightendtag = __value;
return this;
}
public Builder highlightseptag(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
highlightseptag = __value;
return this;
}
public Builder defaultsummaryclass(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
defaultsummaryclass = __value;
return this;
}
}
// Prefix to use in queries to choose a given template
private final StringNode urlprefix;
// The id of the Java class which the given templateset
// should be an instance of. This is only used for implementing
// templates in the Java API instead of Velocity.
private final StringNode classid;
// The symbolic name of the Osgi bundle this template is located in.
// Assumed to be the same as the classid if not set, and is only used
// when classid is used.
private final StringNode bundle;
// The MIME type of a given template
private final StringNode mimetype;
// The character set of a given template
private final StringNode encoding;
// Not used
private final IntegerNode rankprofile;
// Not used in 1.0
private final BooleanNode keepalive;
// Header template. Always rendered.
private final StringNode headertemplate;
// Footer template. Always rendered.
private final StringNode footertemplate;
// Nohits template. Rendered if there are no hits in the result.
private final StringNode nohitstemplate;
// Hit template. Rendered if there are hits in the result.
private final StringNode hittemplate;
// Error template. Rendered if there is an error condition. This is
// not mutually exclusive with the (no)hit templates as such.
private final StringNode errortemplate;
// Aggregated groups header template.
// Default rendering is used if missing
private final StringNode groupsheadertemplate;
// Aggregated range group template.
// Default rendering is used if missing
private final StringNode rangegrouptemplate;
// Aggregated exact group template
// Default rendering is used if missing
private final StringNode exactgrouptemplate;
// Aggregated groups footer template.
// Default rendering is used if missing
private final StringNode groupsfootertemplate;
// Tags used to highlight results, starting a bolded section.
// An empty string means the template should no override what
// was inserted by the search chain.
private final StringNode highlightstarttag;
// Tags used to highlight results, ending a bolded section
// An empty string means the template should no override what
// was inserted by the search chain.
private final StringNode highlightendtag;
// Tags used to highlight results, separating dynamic snippets
// An empty string means the template should no override what
// was inserted by the search chain.
private final StringNode highlightseptag;
// The summary class to use for this template if there is none
// defined in the query.
private final StringNode defaultsummaryclass;
/**
* @deprecated Not for public use.
* Does not check for uninitialized fields.
* Replaced by Templateset(Builder)
*/
@Deprecated
private Templateset() {
this(new Builder(), false);
}
public Templateset(Builder builder) {
this(builder, true);
}
private Templateset(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"qr-templates.templateset[] must be initialized: " + builder.__uninitialized);
urlprefix = (builder.urlprefix == null) ?
new StringNode() : new StringNode(builder.urlprefix);
classid = (builder.classid == null) ?
new StringNode("") : new StringNode(builder.classid);
bundle = (builder.bundle == null) ?
new StringNode("") : new StringNode(builder.bundle);
mimetype = (builder.mimetype == null) ?
new StringNode("text/html") : new StringNode(builder.mimetype);
encoding = (builder.encoding == null) ?
new StringNode("iso-8859-1") : new StringNode(builder.encoding);
rankprofile = (builder.rankprofile == null) ?
new IntegerNode(0) : new IntegerNode(builder.rankprofile);
keepalive = (builder.keepalive == null) ?
new BooleanNode(false) : new BooleanNode(builder.keepalive);
headertemplate = (builder.headertemplate == null) ?
new StringNode("") : new StringNode(builder.headertemplate);
footertemplate = (builder.footertemplate == null) ?
new StringNode("") : new StringNode(builder.footertemplate);
nohitstemplate = (builder.nohitstemplate == null) ?
new StringNode("") : new StringNode(builder.nohitstemplate);
hittemplate = (builder.hittemplate == null) ?
new StringNode("") : new StringNode(builder.hittemplate);
errortemplate = (builder.errortemplate == null) ?
new StringNode("") : new StringNode(builder.errortemplate);
groupsheadertemplate = (builder.groupsheadertemplate == null) ?
new StringNode("[DEFAULT]") : new StringNode(builder.groupsheadertemplate);
rangegrouptemplate = (builder.rangegrouptemplate == null) ?
new StringNode("[DEFAULT]") : new StringNode(builder.rangegrouptemplate);
exactgrouptemplate = (builder.exactgrouptemplate == null) ?
new StringNode("[DEFAULT]") : new StringNode(builder.exactgrouptemplate);
groupsfootertemplate = (builder.groupsfootertemplate == null) ?
new StringNode("[DEFAULT]") : new StringNode(builder.groupsfootertemplate);
highlightstarttag = (builder.highlightstarttag == null) ?
new StringNode("") : new StringNode(builder.highlightstarttag);
highlightendtag = (builder.highlightendtag == null) ?
new StringNode("") : new StringNode(builder.highlightendtag);
highlightseptag = (builder.highlightseptag == null) ?
new StringNode("") : new StringNode(builder.highlightseptag);
defaultsummaryclass = (builder.defaultsummaryclass == null) ?
new StringNode("") : new StringNode(builder.defaultsummaryclass);
}
/**
* @return qr-templates.templateset[].urlprefix
*/
public String urlprefix() {
return urlprefix.value();
}
/**
* @return qr-templates.templateset[].classid
*/
public String classid() {
return classid.value();
}
/**
* @return qr-templates.templateset[].bundle
*/
public String bundle() {
return bundle.value();
}
/**
* @return qr-templates.templateset[].mimetype
*/
public String mimetype() {
return mimetype.value();
}
/**
* @return qr-templates.templateset[].encoding
*/
public String encoding() {
return encoding.value();
}
/**
* @return qr-templates.templateset[].rankprofile
*/
public int rankprofile() {
return rankprofile.value();
}
/**
* @return qr-templates.templateset[].keepalive
*/
public boolean keepalive() {
return keepalive.value();
}
/**
* @return qr-templates.templateset[].headertemplate
*/
public String headertemplate() {
return headertemplate.value();
}
/**
* @return qr-templates.templateset[].footertemplate
*/
public String footertemplate() {
return footertemplate.value();
}
/**
* @return qr-templates.templateset[].nohitstemplate
*/
public String nohitstemplate() {
return nohitstemplate.value();
}
/**
* @return qr-templates.templateset[].hittemplate
*/
public String hittemplate() {
return hittemplate.value();
}
/**
* @return qr-templates.templateset[].errortemplate
*/
public String errortemplate() {
return errortemplate.value();
}
/**
* @return qr-templates.templateset[].groupsheadertemplate
*/
public String groupsheadertemplate() {
return groupsheadertemplate.value();
}
/**
* @return qr-templates.templateset[].rangegrouptemplate
*/
public String rangegrouptemplate() {
return rangegrouptemplate.value();
}
/**
* @return qr-templates.templateset[].exactgrouptemplate
*/
public String exactgrouptemplate() {
return exactgrouptemplate.value();
}
/**
* @return qr-templates.templateset[].groupsfootertemplate
*/
public String groupsfootertemplate() {
return groupsfootertemplate.value();
}
/**
* @return qr-templates.templateset[].highlightstarttag
*/
public String highlightstarttag() {
return highlightstarttag.value();
}
/**
* @return qr-templates.templateset[].highlightendtag
*/
public String highlightendtag() {
return highlightendtag.value();
}
/**
* @return qr-templates.templateset[].highlightseptag
*/
public String highlightseptag() {
return highlightseptag.value();
}
/**
* @return qr-templates.templateset[].defaultsummaryclass
*/
public String defaultsummaryclass() {
return defaultsummaryclass.value();
}
private ChangesRequiringRestart getChangesRequiringRestart(Templateset newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("templateset");
return changes;
}
private static InnerNodeVector createVector(List builders) {
List elems = new ArrayList<>();
for (Builder b : builders) {
elems.add(new Templateset(b));
}
return new InnerNodeVector(elems, new Templateset());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy