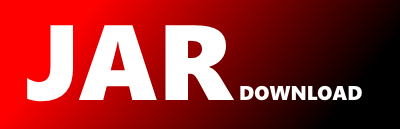
com.yahoo.container.core.ChainsConfig Maven / Gradle / Ivy
/**
* This file is generated from a config definition file.
* ------------ D O N O T E D I T ! ------------
*/
package com.yahoo.container.core;
import java.util.*;
import java.io.File;
import java.nio.file.Path;
import com.yahoo.config.*;
/**
* This class represents the root node of chains
*
* Copyright Vespa.ai. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
* Chains configuration
*/
public final class ChainsConfig extends ConfigInstance {
public final static String CONFIG_DEF_MD5 = "4bac3afe81c7cfcd57d7a90f64337f24";
public final static String CONFIG_DEF_NAME = "chains";
public final static String CONFIG_DEF_NAMESPACE = "container.core";
public final static String[] CONFIG_DEF_SCHEMA = {
"namespace=container.core",
"components[].id string",
"components[].dependencies.provides[] string",
"components[].dependencies.before[] string",
"components[].dependencies.after[] string",
"chains[].id string",
"chains[].type enum {DOCPROC, SEARCH} default=SEARCH",
"chains[].components[] string",
"chains[].inherits[] string",
"chains[].excludes[] string",
"chains[].phases[].id string",
"chains[].phases[].before[] string",
"chains[].phases[].after[] string"
};
public static String getDefMd5() { return CONFIG_DEF_MD5; }
public static String getDefName() { return CONFIG_DEF_NAME; }
public static String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
public interface Producer extends ConfigInstance.Producer {
void getConfig(Builder builder);
}
public static final class Builder implements ConfigInstance.Builder {
private Set __uninitialized = new HashSet();
public List components = new ArrayList<>();
public List chains = new ArrayList<>();
public Builder() { }
public Builder(ChainsConfig config) {
for (Components c : config.components()) {
components(new Components.Builder(c));
}
for (Chains c : config.chains()) {
chains(new Chains.Builder(c));
}
}
private Builder override(Builder __superior) {
if (!__superior.components.isEmpty())
components.addAll(__superior.components);
if (!__superior.chains.isEmpty())
chains.addAll(__superior.chains);
return this;
}
/**
* Add the given builder to this builder's list of Components builders
* @param __builder a builder
* @return this builder
*/
public Builder components(Components.Builder __builder) {
components.add(__builder);
return this;
}
/**
* Make a new builder and run the supplied function on it before adding it to the list
* @param __func lambda that modifies the given builder
* @return this builder
*/
public Builder components(java.util.function.Consumer __func) {
Components.Builder __inner = new Components.Builder();
__func.accept(__inner);
components.add(__inner);
return this;
}
/**
* Set the given list as this builder's list of Components builders
* @param __builders a list of builders
* @return this builder
*/
public Builder components(List __builders) {
components = __builders;
return this;
}
/**
* Add the given builder to this builder's list of Chains builders
* @param __builder a builder
* @return this builder
*/
public Builder chains(Chains.Builder __builder) {
chains.add(__builder);
return this;
}
/**
* Make a new builder and run the supplied function on it before adding it to the list
* @param __func lambda that modifies the given builder
* @return this builder
*/
public Builder chains(java.util.function.Consumer __func) {
Chains.Builder __inner = new Chains.Builder();
__func.accept(__inner);
chains.add(__inner);
return this;
}
/**
* Set the given list as this builder's list of Chains builders
* @param __builders a list of builders
* @return this builder
*/
public Builder chains(List __builders) {
chains = __builders;
return this;
}
private boolean _applyOnRestart = false;
@java.lang.Override
public final boolean dispatchGetConfig(ConfigInstance.Producer producer) {
if (producer instanceof Producer) {
((Producer)producer).getConfig(this);
return true;
}
return false;
}
@java.lang.Override
public final String getDefMd5() { return CONFIG_DEF_MD5; }
@java.lang.Override
public final String getDefName() { return CONFIG_DEF_NAME; }
@java.lang.Override
public final String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
@java.lang.Override
public final boolean getApplyOnRestart() { return _applyOnRestart; }
@java.lang.Override
public final void setApplyOnRestart(boolean applyOnRestart) { _applyOnRestart = applyOnRestart; }
public ChainsConfig build() {
return new ChainsConfig(this);
}
}
private final InnerNodeVector components;
private final InnerNodeVector chains;
public ChainsConfig(Builder builder) {
this(builder, true);
}
private ChainsConfig(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"chains must be initialized: " + builder.__uninitialized);
components = Components.createVector(builder.components);
chains = Chains.createVector(builder.chains);
}
/**
* @return chains.components[]
*/
public List components() {
return components;
}
/**
* @param i the index of the value to return
* @return chains.components[]
*/
public Components components(int i) {
return components.get(i);
}
/**
* @return chains.chains[]
*/
public List chains() {
return chains;
}
/**
* @param i the index of the value to return
* @return chains.chains[]
*/
public Chains chains(int i) {
return chains.get(i);
}
private ChangesRequiringRestart getChangesRequiringRestart(ChainsConfig newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("chains");
return changes;
}
private static boolean containsFieldsFlaggedWithRestart() {
return false;
}
/**
* This class represents chains.components[]
*/
public final static class Components extends InnerNode {
public static final class Builder implements ConfigBuilder {
private Set __uninitialized = new HashSet(List.of(
"id"
));
private String id = null;
public Dependencies.Builder dependencies = new Dependencies.Builder();
public Builder() { }
public Builder(Components config) {
id(config.id());
dependencies(new Dependencies.Builder(config.dependencies()));
}
private Builder override(Builder __superior) {
if (__superior.id != null)
id(__superior.id);
dependencies(dependencies.override(__superior.dependencies));
return this;
}
public Builder id(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
id = __value;
__uninitialized.remove("id");
return this;
}
public Builder dependencies(Dependencies.Builder __builder) {
dependencies = __builder;
return this;
}
/**
* Make a new builder and run the supplied function on it before adding it to the list
* @param __func lambda that modifies the given builder
* @return this builder
*/
public Builder dependencies(java.util.function.Consumer __func) {
Dependencies.Builder __inner = new Dependencies.Builder();
__func.accept(__inner);
dependencies = __inner;
return this;
}
public Components build() {
return new Components(this);
}
}
private final StringNode id;
private final Dependencies dependencies;
public Components(Builder builder) {
this(builder, true);
}
private Components(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"chains.components[] must be initialized: " + builder.__uninitialized);
id = (builder.id == null) ?
new StringNode() : new StringNode(builder.id);
dependencies = new Dependencies(builder.dependencies, throwIfUninitialized);
}
/**
* @return chains.components[].id
*/
public String id() {
return id.value();
}
/**
* @return chains.components[].dependencies
*/
public Dependencies dependencies() {
return dependencies;
}
private ChangesRequiringRestart getChangesRequiringRestart(Components newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("components");
return changes;
}
private static InnerNodeVector createVector(List builders) {
List elems = new ArrayList<>();
for (Builder b : builders) {
elems.add(new Components(b));
}
return new InnerNodeVector(elems);
}
/**
* This class represents chains.components[].dependencies
*/
public final static class Dependencies extends InnerNode {
public static final class Builder implements ConfigBuilder {
private Set __uninitialized = new HashSet();
public List provides = new ArrayList<>();
public List before = new ArrayList<>();
public List after = new ArrayList<>();
public Builder() { }
public Builder(Dependencies config) {
provides(config.provides());
before(config.before());
after(config.after());
}
private Builder override(Builder __superior) {
if (!__superior.provides.isEmpty())
provides.addAll(__superior.provides);
if (!__superior.before.isEmpty())
before.addAll(__superior.before);
if (!__superior.after.isEmpty())
after.addAll(__superior.after);
return this;
}
public Builder provides(String __value) {
provides.add(__value);
return this;
}
public Builder provides(Collection __values) {
provides.addAll(__values);
return this;
}
public Builder before(String __value) {
before.add(__value);
return this;
}
public Builder before(Collection __values) {
before.addAll(__values);
return this;
}
public Builder after(String __value) {
after.add(__value);
return this;
}
public Builder after(Collection __values) {
after.addAll(__values);
return this;
}
public Dependencies build() {
return new Dependencies(this);
}
}
// Configured functionality provided by this - comes in addition to those set in the code
private final LeafNodeVector provides;
// Configured "before" dependencies provided by this - comes in addition to those set in the code
private final LeafNodeVector before;
// Configured "after" dependencies provided by this - comes in addition to those set in the code
private final LeafNodeVector after;
public Dependencies(Builder builder) {
this(builder, true);
}
private Dependencies(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"chains.components[].dependencies must be initialized: " + builder.__uninitialized);
provides = new LeafNodeVector<>(builder.provides, new StringNode());
before = new LeafNodeVector<>(builder.before, new StringNode());
after = new LeafNodeVector<>(builder.after, new StringNode());
}
/**
* @return chains.components[].dependencies.provides[]
*/
public List provides() {
return provides.asList();
}
/**
* @param i the index of the value to return
* @return chains.components[].dependencies.provides[]
*/
public String provides(int i) {
return provides.get(i).value();
}
/**
* @return chains.components[].dependencies.before[]
*/
public List before() {
return before.asList();
}
/**
* @param i the index of the value to return
* @return chains.components[].dependencies.before[]
*/
public String before(int i) {
return before.get(i).value();
}
/**
* @return chains.components[].dependencies.after[]
*/
public List after() {
return after.asList();
}
/**
* @param i the index of the value to return
* @return chains.components[].dependencies.after[]
*/
public String after(int i) {
return after.get(i).value();
}
private ChangesRequiringRestart getChangesRequiringRestart(Dependencies newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("dependencies");
return changes;
}
}
}
/**
* This class represents chains.chains[]
*/
public final static class Chains extends InnerNode {
public static final class Builder implements ConfigBuilder {
private Set __uninitialized = new HashSet(List.of(
"id"
));
private String id = null;
private Type.Enum type = null;
public List components = new ArrayList<>();
public List inherits = new ArrayList<>();
public List excludes = new ArrayList<>();
public List phases = new ArrayList<>();
public Builder() { }
public Builder(Chains config) {
id(config.id());
type(config.type());
components(config.components());
inherits(config.inherits());
excludes(config.excludes());
for (Phases p : config.phases()) {
phases(new Phases.Builder(p));
}
}
private Builder override(Builder __superior) {
if (__superior.id != null)
id(__superior.id);
if (__superior.type != null)
type(__superior.type);
if (!__superior.components.isEmpty())
components.addAll(__superior.components);
if (!__superior.inherits.isEmpty())
inherits.addAll(__superior.inherits);
if (!__superior.excludes.isEmpty())
excludes.addAll(__superior.excludes);
if (!__superior.phases.isEmpty())
phases.addAll(__superior.phases);
return this;
}
public Builder id(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
id = __value;
__uninitialized.remove("id");
return this;
}
public Builder type(Type.Enum __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
type = __value;
return this;
}
private Builder type(String __value) {
return type(Type.Enum.valueOf(__value));
}
public Builder components(String __value) {
components.add(__value);
return this;
}
public Builder components(Collection __values) {
components.addAll(__values);
return this;
}
public Builder inherits(String __value) {
inherits.add(__value);
return this;
}
public Builder inherits(Collection __values) {
inherits.addAll(__values);
return this;
}
public Builder excludes(String __value) {
excludes.add(__value);
return this;
}
public Builder excludes(Collection __values) {
excludes.addAll(__values);
return this;
}
/**
* Add the given builder to this builder's list of Phases builders
* @param __builder a builder
* @return this builder
*/
public Builder phases(Phases.Builder __builder) {
phases.add(__builder);
return this;
}
/**
* Make a new builder and run the supplied function on it before adding it to the list
* @param __func lambda that modifies the given builder
* @return this builder
*/
public Builder phases(java.util.function.Consumer __func) {
Phases.Builder __inner = new Phases.Builder();
__func.accept(__inner);
phases.add(__inner);
return this;
}
/**
* Set the given list as this builder's list of Phases builders
* @param __builders a list of builders
* @return this builder
*/
public Builder phases(List __builders) {
phases = __builders;
return this;
}
public Chains build() {
return new Chains(this);
}
}
// The id of this chain. The id has the form name(:version)?
// where the version has the form 1(.2(.3(.identifier)?)?)?.
// The default chain must be called "default".
private final StringNode id;
// The type of this chain
private final Type type;
// The id of a component to include in this chain.
// The id has the form fullclassname(:version)?
// where the version has the form 1(.2(.3(.identifier)?)?)?.
private final LeafNodeVector components;
// The optional list of chain ids this inherits.
// The ids has the form name(:version)?
// where the version has the form 1(.2(.3(.identifier)?)?)?.
// If the version is not specified the newest version is used.
private final LeafNodeVector inherits;
// The optional list of component ids to exclude from this chain even if they exists in inherited chains
// If versions are specified in these ids, they are ignored.
private final LeafNodeVector excludes;
private final InnerNodeVector phases;
public Chains(Builder builder) {
this(builder, true);
}
private Chains(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"chains.chains[] must be initialized: " + builder.__uninitialized);
id = (builder.id == null) ?
new StringNode() : new StringNode(builder.id);
type = (builder.type == null) ?
new Type(Type.SEARCH) : new Type(builder.type);
components = new LeafNodeVector<>(builder.components, new StringNode());
inherits = new LeafNodeVector<>(builder.inherits, new StringNode());
excludes = new LeafNodeVector<>(builder.excludes, new StringNode());
phases = Phases.createVector(builder.phases);
}
/**
* @return chains.chains[].id
*/
public String id() {
return id.value();
}
/**
* @return chains.chains[].type
*/
public Type.Enum type() {
return type.value();
}
/**
* @return chains.chains[].components[]
*/
public List components() {
return components.asList();
}
/**
* @param i the index of the value to return
* @return chains.chains[].components[]
*/
public String components(int i) {
return components.get(i).value();
}
/**
* @return chains.chains[].inherits[]
*/
public List inherits() {
return inherits.asList();
}
/**
* @param i the index of the value to return
* @return chains.chains[].inherits[]
*/
public String inherits(int i) {
return inherits.get(i).value();
}
/**
* @return chains.chains[].excludes[]
*/
public List excludes() {
return excludes.asList();
}
/**
* @param i the index of the value to return
* @return chains.chains[].excludes[]
*/
public String excludes(int i) {
return excludes.get(i).value();
}
/**
* @return chains.chains[].phases[]
*/
public List phases() {
return phases;
}
/**
* @param i the index of the value to return
* @return chains.chains[].phases[]
*/
public Phases phases(int i) {
return phases.get(i);
}
private ChangesRequiringRestart getChangesRequiringRestart(Chains newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("chains");
return changes;
}
private static InnerNodeVector createVector(List builders) {
List elems = new ArrayList<>();
for (Builder b : builders) {
elems.add(new Chains(b));
}
return new InnerNodeVector(elems);
}
/**
* This class represents chains.chains[].type
*
* The type of this chain
*/
public final static class Type extends EnumNode {
public Type(){
this.value = null;
}
public Type(Enum enumValue) {
super(enumValue != null);
this.value = enumValue;
}
public enum Enum {DOCPROC, SEARCH}
public final static Enum DOCPROC = Enum.DOCPROC;
public final static Enum SEARCH = Enum.SEARCH;
@Override
protected boolean doSetValue(String name) {
try {
value = Enum.valueOf(name);
return true;
} catch (IllegalArgumentException e) {
}
return false;
}
}
/**
* This class represents chains.chains[].phases[]
*/
public final static class Phases extends InnerNode {
public static final class Builder implements ConfigBuilder {
private Set __uninitialized = new HashSet(List.of(
"id"
));
private String id = null;
public List before = new ArrayList<>();
public List after = new ArrayList<>();
public Builder() { }
public Builder(Phases config) {
id(config.id());
before(config.before());
after(config.after());
}
private Builder override(Builder __superior) {
if (__superior.id != null)
id(__superior.id);
if (!__superior.before.isEmpty())
before.addAll(__superior.before);
if (!__superior.after.isEmpty())
after.addAll(__superior.after);
return this;
}
public Builder id(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
id = __value;
__uninitialized.remove("id");
return this;
}
public Builder before(String __value) {
before.add(__value);
return this;
}
public Builder before(Collection __values) {
before.addAll(__values);
return this;
}
public Builder after(String __value) {
after.add(__value);
return this;
}
public Builder after(Collection __values) {
after.addAll(__values);
return this;
}
public Phases build() {
return new Phases(this);
}
}
// The phases for a chain
private final StringNode id;
private final LeafNodeVector before;
private final LeafNodeVector after;
public Phases(Builder builder) {
this(builder, true);
}
private Phases(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"chains.chains[].phases[] must be initialized: " + builder.__uninitialized);
id = (builder.id == null) ?
new StringNode() : new StringNode(builder.id);
before = new LeafNodeVector<>(builder.before, new StringNode());
after = new LeafNodeVector<>(builder.after, new StringNode());
}
/**
* @return chains.chains[].phases[].id
*/
public String id() {
return id.value();
}
/**
* @return chains.chains[].phases[].before[]
*/
public List before() {
return before.asList();
}
/**
* @param i the index of the value to return
* @return chains.chains[].phases[].before[]
*/
public String before(int i) {
return before.get(i).value();
}
/**
* @return chains.chains[].phases[].after[]
*/
public List after() {
return after.asList();
}
/**
* @param i the index of the value to return
* @return chains.chains[].phases[].after[]
*/
public String after(int i) {
return after.get(i).value();
}
private ChangesRequiringRestart getChangesRequiringRestart(Phases newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("phases");
return changes;
}
private static InnerNodeVector createVector(List builders) {
List elems = new ArrayList<>();
for (Builder b : builders) {
elems.add(new Phases(b));
}
return new InnerNodeVector(elems);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy