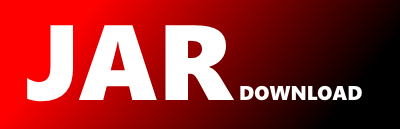
com.yahoo.container.jdisc.HttpMethodAclMapping Maven / Gradle / Ivy
// Copyright Vespa.ai. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
package com.yahoo.container.jdisc;
import com.yahoo.jdisc.http.HttpRequest;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import static com.yahoo.jdisc.http.HttpRequest.Method.CONNECT;
import static com.yahoo.jdisc.http.HttpRequest.Method.DELETE;
import static com.yahoo.jdisc.http.HttpRequest.Method.GET;
import static com.yahoo.jdisc.http.HttpRequest.Method.HEAD;
import static com.yahoo.jdisc.http.HttpRequest.Method.OPTIONS;
import static com.yahoo.jdisc.http.HttpRequest.Method.PATCH;
import static com.yahoo.jdisc.http.HttpRequest.Method.POST;
import static com.yahoo.jdisc.http.HttpRequest.Method.PUT;
import static com.yahoo.jdisc.http.HttpRequest.Method.TRACE;
/**
* Acl Mapping based on http method.
* Defaults to:
* {GET, HEAD, OPTIONS} -> READ
* {POST, DELETE, PUT, PATCH, CONNECT, TRACE} -> WRITE
* @author mortent
*/
public class HttpMethodAclMapping implements AclMapping {
private final Map mappings;
private HttpMethodAclMapping(Map overrides) {
HashMap tmp = new HashMap<>(defaultMappings());
tmp.putAll(overrides);
mappings = Map.copyOf(tmp);
}
private static Map defaultMappings() {
return Map.of(GET, Action.READ,
HEAD, Action.READ,
OPTIONS, Action.READ,
POST, Action.WRITE,
DELETE, Action.WRITE,
PUT, Action.WRITE,
PATCH, Action.WRITE,
CONNECT, Action.WRITE,
TRACE, Action.WRITE);
}
@Override
public Action get(RequestView requestView) {
return Optional.ofNullable(mappings.get(requestView.method()))
.orElseThrow(() -> new IllegalArgumentException("Illegal request method: " + requestView.method()));
}
public static HttpMethodAclMapping.Builder standard() {
return new HttpMethodAclMapping.Builder();
}
public static class Builder {
private final Map overrides = new HashMap<>();
public HttpMethodAclMapping.Builder override(HttpRequest.Method method, Action action) {
overrides.put(method, action);
return this;
}
public HttpMethodAclMapping build() {
return new HttpMethodAclMapping(overrides);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy