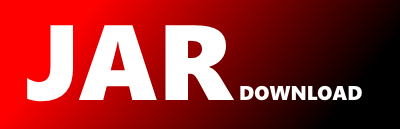
com.yahoo.container.handler.ThreadpoolConfig Maven / Gradle / Ivy
/**
* This file is generated from a config definition file.
* ------------ D O N O T E D I T ! ------------
*/
package com.yahoo.container.handler;
import java.util.*;
import java.io.File;
import java.nio.file.Path;
import com.yahoo.config.*;
/**
* This class represents the root node of threadpool
*
* Copyright Vespa.ai. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
*/
public final class ThreadpoolConfig extends ConfigInstance {
public final static String CONFIG_DEF_MD5 = "68f09a2773696a5224de584d1fb17673";
public final static String CONFIG_DEF_NAME = "threadpool";
public final static String CONFIG_DEF_NAMESPACE = "container.handler";
public final static String[] CONFIG_DEF_SCHEMA = {
"namespace=container.handler",
"maxthreads int default=500",
"corePoolSize int default=500",
"keepAliveTime double default=5.0",
"queueSize int default=0",
"maxThreadExecutionTimeSeconds int default=190",
"name string default=\"default-pool\""
};
public static String getDefMd5() { return CONFIG_DEF_MD5; }
public static String getDefName() { return CONFIG_DEF_NAME; }
public static String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
public interface Producer extends ConfigInstance.Producer {
void getConfig(Builder builder);
}
public static final class Builder implements ConfigInstance.Builder {
private Set __uninitialized = new HashSet();
private Integer maxthreads = null;
private Integer corePoolSize = null;
private Double keepAliveTime = null;
private Integer queueSize = null;
private Integer maxThreadExecutionTimeSeconds = null;
private String name = null;
public Builder() { }
public Builder(ThreadpoolConfig config) {
maxthreads(config.maxthreads());
corePoolSize(config.corePoolSize());
keepAliveTime(config.keepAliveTime());
queueSize(config.queueSize());
maxThreadExecutionTimeSeconds(config.maxThreadExecutionTimeSeconds());
name(config.name());
}
private Builder override(Builder __superior) {
if (__superior.maxthreads != null)
maxthreads(__superior.maxthreads);
if (__superior.corePoolSize != null)
corePoolSize(__superior.corePoolSize);
if (__superior.keepAliveTime != null)
keepAliveTime(__superior.keepAliveTime);
if (__superior.queueSize != null)
queueSize(__superior.queueSize);
if (__superior.maxThreadExecutionTimeSeconds != null)
maxThreadExecutionTimeSeconds(__superior.maxThreadExecutionTimeSeconds);
if (__superior.name != null)
name(__superior.name);
return this;
}
public Builder maxthreads(int __value) {
maxthreads = __value;
return this;
}
private Builder maxthreads(String __value) {
return maxthreads(Integer.valueOf(__value));
}
public Builder corePoolSize(int __value) {
corePoolSize = __value;
return this;
}
private Builder corePoolSize(String __value) {
return corePoolSize(Integer.valueOf(__value));
}
public Builder keepAliveTime(double __value) {
keepAliveTime = __value;
return this;
}
private Builder keepAliveTime(String __value) {
return keepAliveTime(Double.valueOf(__value));
}
public Builder queueSize(int __value) {
queueSize = __value;
return this;
}
private Builder queueSize(String __value) {
return queueSize(Integer.valueOf(__value));
}
public Builder maxThreadExecutionTimeSeconds(int __value) {
maxThreadExecutionTimeSeconds = __value;
return this;
}
private Builder maxThreadExecutionTimeSeconds(String __value) {
return maxThreadExecutionTimeSeconds(Integer.valueOf(__value));
}
public Builder name(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
name = __value;
return this;
}
private boolean _applyOnRestart = false;
@java.lang.Override
public final boolean dispatchGetConfig(ConfigInstance.Producer producer) {
if (producer instanceof Producer) {
((Producer)producer).getConfig(this);
return true;
}
return false;
}
@java.lang.Override
public final String getDefMd5() { return CONFIG_DEF_MD5; }
@java.lang.Override
public final String getDefName() { return CONFIG_DEF_NAME; }
@java.lang.Override
public final String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
@java.lang.Override
public final boolean getApplyOnRestart() { return _applyOnRestart; }
@java.lang.Override
public final void setApplyOnRestart(boolean applyOnRestart) { _applyOnRestart = applyOnRestart; }
public ThreadpoolConfig build() {
return new ThreadpoolConfig(this);
}
}
// Maximum number of thread in the thread pool
// 0 is translated to vcpu*4
// Negative value is interpreted as scale factor ( vcpu*abs(maxThreads) )
private final IntegerNode maxthreads;
// The number of threads to keep in the pool, even if they are idle
// 0 is translated to vcpu*4
// Negative value is interpreted as scale factor ( vcpu*abs(corePoolSize) )
private final IntegerNode corePoolSize;
// The number of seconds that excess idle threads will wait for new tasks before terminating
private final DoubleNode keepAliveTime;
// max queue size
// There can be queueSize + maxthreads requests inflight concurrently
// The container will start replying 503
// Negative value will cause it to set to maxthreads*4
private final IntegerNode queueSize;
// The max time the container tolerates having no threads available before it shuts down to
// get out of a bad state. This should be set a bit higher than the expected max execution
// time of each request when in a state of overload, i.e about "worst case execution time*2"
private final IntegerNode maxThreadExecutionTimeSeconds;
// Prefix for the name of the threads
private final StringNode name;
public ThreadpoolConfig(Builder builder) {
this(builder, true);
}
private ThreadpoolConfig(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"threadpool must be initialized: " + builder.__uninitialized);
maxthreads = (builder.maxthreads == null) ?
new IntegerNode(500) : new IntegerNode(builder.maxthreads);
corePoolSize = (builder.corePoolSize == null) ?
new IntegerNode(500) : new IntegerNode(builder.corePoolSize);
keepAliveTime = (builder.keepAliveTime == null) ?
new DoubleNode(5.0D) : new DoubleNode(builder.keepAliveTime);
queueSize = (builder.queueSize == null) ?
new IntegerNode(0) : new IntegerNode(builder.queueSize);
maxThreadExecutionTimeSeconds = (builder.maxThreadExecutionTimeSeconds == null) ?
new IntegerNode(190) : new IntegerNode(builder.maxThreadExecutionTimeSeconds);
name = (builder.name == null) ?
new StringNode("default-pool") : new StringNode(builder.name);
}
/**
* @return threadpool.maxthreads
*/
public int maxthreads() {
return maxthreads.value();
}
/**
* @return threadpool.corePoolSize
*/
public int corePoolSize() {
return corePoolSize.value();
}
/**
* @return threadpool.keepAliveTime
*/
public double keepAliveTime() {
return keepAliveTime.value();
}
/**
* @return threadpool.queueSize
*/
public int queueSize() {
return queueSize.value();
}
/**
* @return threadpool.maxThreadExecutionTimeSeconds
*/
public int maxThreadExecutionTimeSeconds() {
return maxThreadExecutionTimeSeconds.value();
}
/**
* @return threadpool.name
*/
public String name() {
return name.value();
}
private ChangesRequiringRestart getChangesRequiringRestart(ThreadpoolConfig newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("threadpool");
return changes;
}
private static boolean containsFieldsFlaggedWithRestart() {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy