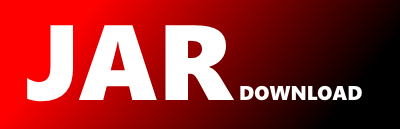
com.yahoo.jdisc.http.client.HttpClientConfig Maven / Gradle / Ivy
/**
* This file is generated from a config definition file.
* ------------ D O N O T E D I T ! ------------
*/
package com.yahoo.jdisc.http.client;
import java.util.*;
import java.io.File;
import java.nio.file.Path;
import com.yahoo.config.*;
/**
* This class represents the root node of http-client
*
* Copyright Vespa.ai. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
*/
public final class HttpClientConfig extends ConfigInstance {
public final static String CONFIG_DEF_MD5 = "0fc0ad6c1c0aef320f61b73edd7c4740";
public final static String CONFIG_DEF_NAME = "http-client";
public final static String CONFIG_DEF_NAMESPACE = "jdisc.http.client";
public final static String[] CONFIG_DEF_SCHEMA = {
"namespace=jdisc.http.client",
"userAgent string default = \"JDisc/1.0\"",
"chunkedEncodingEnabled bool default = false",
"compressionEnabled bool default = false",
"connectionPoolEnabled bool default = true",
"followRedirects bool default = false",
"removeQueryParamsOnRedirect bool default = true",
"sslConnectionPoolEnabled bool default = true",
"proxyServer string default = \"\"",
"useProxyProperties bool default = false",
"useRawUri bool default = false",
"compressionLevel int default = -1",
"maxNumConnections int default = -1",
"maxNumConnectionsPerHost int default = -1",
"maxNumRedirects int default = 5",
"maxNumRetries int default = 0",
"connectionTimeout double default = 60",
"idleConnectionInPoolTimeout double default = 60",
"idleConnectionTimeout double default = 60",
"idleWebSocketTimeout double default = 15",
"requestTimeout double default = 60",
"ssl.enabled bool default = false",
"ssl.keyStoreType string default = \"JKS\"",
"ssl.keyStorePath string default = \"jdisc_container/keyStore.jks\"",
"ssl.trustStorePath string default = \"conf/jdisc_container/trustStore.jks\"",
"ssl.keyDBKey string default = \"jdisc_container\"",
"ssl.algorithm string default = \"SunX509\"",
"ssl.protocol string default = \"TLS\""
};
public static String getDefMd5() { return CONFIG_DEF_MD5; }
public static String getDefName() { return CONFIG_DEF_NAME; }
public static String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
public interface Producer extends ConfigInstance.Producer {
void getConfig(Builder builder);
}
public static final class Builder implements ConfigInstance.Builder {
private Set __uninitialized = new HashSet();
private String userAgent = null;
private Boolean chunkedEncodingEnabled = null;
private Boolean compressionEnabled = null;
private Boolean connectionPoolEnabled = null;
private Boolean followRedirects = null;
private Boolean removeQueryParamsOnRedirect = null;
private Boolean sslConnectionPoolEnabled = null;
private String proxyServer = null;
private Boolean useProxyProperties = null;
private Boolean useRawUri = null;
private Integer compressionLevel = null;
private Integer maxNumConnections = null;
private Integer maxNumConnectionsPerHost = null;
private Integer maxNumRedirects = null;
private Integer maxNumRetries = null;
private Double connectionTimeout = null;
private Double idleConnectionInPoolTimeout = null;
private Double idleConnectionTimeout = null;
private Double idleWebSocketTimeout = null;
private Double requestTimeout = null;
public Ssl.Builder ssl = new Ssl.Builder();
public Builder() { }
public Builder(HttpClientConfig config) {
userAgent(config.userAgent());
chunkedEncodingEnabled(config.chunkedEncodingEnabled());
compressionEnabled(config.compressionEnabled());
connectionPoolEnabled(config.connectionPoolEnabled());
followRedirects(config.followRedirects());
removeQueryParamsOnRedirect(config.removeQueryParamsOnRedirect());
sslConnectionPoolEnabled(config.sslConnectionPoolEnabled());
proxyServer(config.proxyServer());
useProxyProperties(config.useProxyProperties());
useRawUri(config.useRawUri());
compressionLevel(config.compressionLevel());
maxNumConnections(config.maxNumConnections());
maxNumConnectionsPerHost(config.maxNumConnectionsPerHost());
maxNumRedirects(config.maxNumRedirects());
maxNumRetries(config.maxNumRetries());
connectionTimeout(config.connectionTimeout());
idleConnectionInPoolTimeout(config.idleConnectionInPoolTimeout());
idleConnectionTimeout(config.idleConnectionTimeout());
idleWebSocketTimeout(config.idleWebSocketTimeout());
requestTimeout(config.requestTimeout());
ssl(new Ssl.Builder(config.ssl()));
}
private Builder override(Builder __superior) {
if (__superior.userAgent != null)
userAgent(__superior.userAgent);
if (__superior.chunkedEncodingEnabled != null)
chunkedEncodingEnabled(__superior.chunkedEncodingEnabled);
if (__superior.compressionEnabled != null)
compressionEnabled(__superior.compressionEnabled);
if (__superior.connectionPoolEnabled != null)
connectionPoolEnabled(__superior.connectionPoolEnabled);
if (__superior.followRedirects != null)
followRedirects(__superior.followRedirects);
if (__superior.removeQueryParamsOnRedirect != null)
removeQueryParamsOnRedirect(__superior.removeQueryParamsOnRedirect);
if (__superior.sslConnectionPoolEnabled != null)
sslConnectionPoolEnabled(__superior.sslConnectionPoolEnabled);
if (__superior.proxyServer != null)
proxyServer(__superior.proxyServer);
if (__superior.useProxyProperties != null)
useProxyProperties(__superior.useProxyProperties);
if (__superior.useRawUri != null)
useRawUri(__superior.useRawUri);
if (__superior.compressionLevel != null)
compressionLevel(__superior.compressionLevel);
if (__superior.maxNumConnections != null)
maxNumConnections(__superior.maxNumConnections);
if (__superior.maxNumConnectionsPerHost != null)
maxNumConnectionsPerHost(__superior.maxNumConnectionsPerHost);
if (__superior.maxNumRedirects != null)
maxNumRedirects(__superior.maxNumRedirects);
if (__superior.maxNumRetries != null)
maxNumRetries(__superior.maxNumRetries);
if (__superior.connectionTimeout != null)
connectionTimeout(__superior.connectionTimeout);
if (__superior.idleConnectionInPoolTimeout != null)
idleConnectionInPoolTimeout(__superior.idleConnectionInPoolTimeout);
if (__superior.idleConnectionTimeout != null)
idleConnectionTimeout(__superior.idleConnectionTimeout);
if (__superior.idleWebSocketTimeout != null)
idleWebSocketTimeout(__superior.idleWebSocketTimeout);
if (__superior.requestTimeout != null)
requestTimeout(__superior.requestTimeout);
ssl(ssl.override(__superior.ssl));
return this;
}
public Builder userAgent(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
userAgent = __value;
return this;
}
public Builder chunkedEncodingEnabled(boolean __value) {
chunkedEncodingEnabled = __value;
return this;
}
private Builder chunkedEncodingEnabled(String __value) {
return chunkedEncodingEnabled(Boolean.valueOf(__value));
}
public Builder compressionEnabled(boolean __value) {
compressionEnabled = __value;
return this;
}
private Builder compressionEnabled(String __value) {
return compressionEnabled(Boolean.valueOf(__value));
}
public Builder connectionPoolEnabled(boolean __value) {
connectionPoolEnabled = __value;
return this;
}
private Builder connectionPoolEnabled(String __value) {
return connectionPoolEnabled(Boolean.valueOf(__value));
}
public Builder followRedirects(boolean __value) {
followRedirects = __value;
return this;
}
private Builder followRedirects(String __value) {
return followRedirects(Boolean.valueOf(__value));
}
public Builder removeQueryParamsOnRedirect(boolean __value) {
removeQueryParamsOnRedirect = __value;
return this;
}
private Builder removeQueryParamsOnRedirect(String __value) {
return removeQueryParamsOnRedirect(Boolean.valueOf(__value));
}
public Builder sslConnectionPoolEnabled(boolean __value) {
sslConnectionPoolEnabled = __value;
return this;
}
private Builder sslConnectionPoolEnabled(String __value) {
return sslConnectionPoolEnabled(Boolean.valueOf(__value));
}
public Builder proxyServer(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
proxyServer = __value;
return this;
}
public Builder useProxyProperties(boolean __value) {
useProxyProperties = __value;
return this;
}
private Builder useProxyProperties(String __value) {
return useProxyProperties(Boolean.valueOf(__value));
}
public Builder useRawUri(boolean __value) {
useRawUri = __value;
return this;
}
private Builder useRawUri(String __value) {
return useRawUri(Boolean.valueOf(__value));
}
public Builder compressionLevel(int __value) {
compressionLevel = __value;
return this;
}
private Builder compressionLevel(String __value) {
return compressionLevel(Integer.valueOf(__value));
}
public Builder maxNumConnections(int __value) {
maxNumConnections = __value;
return this;
}
private Builder maxNumConnections(String __value) {
return maxNumConnections(Integer.valueOf(__value));
}
public Builder maxNumConnectionsPerHost(int __value) {
maxNumConnectionsPerHost = __value;
return this;
}
private Builder maxNumConnectionsPerHost(String __value) {
return maxNumConnectionsPerHost(Integer.valueOf(__value));
}
public Builder maxNumRedirects(int __value) {
maxNumRedirects = __value;
return this;
}
private Builder maxNumRedirects(String __value) {
return maxNumRedirects(Integer.valueOf(__value));
}
public Builder maxNumRetries(int __value) {
maxNumRetries = __value;
return this;
}
private Builder maxNumRetries(String __value) {
return maxNumRetries(Integer.valueOf(__value));
}
public Builder connectionTimeout(double __value) {
connectionTimeout = __value;
return this;
}
private Builder connectionTimeout(String __value) {
return connectionTimeout(Double.valueOf(__value));
}
public Builder idleConnectionInPoolTimeout(double __value) {
idleConnectionInPoolTimeout = __value;
return this;
}
private Builder idleConnectionInPoolTimeout(String __value) {
return idleConnectionInPoolTimeout(Double.valueOf(__value));
}
public Builder idleConnectionTimeout(double __value) {
idleConnectionTimeout = __value;
return this;
}
private Builder idleConnectionTimeout(String __value) {
return idleConnectionTimeout(Double.valueOf(__value));
}
public Builder idleWebSocketTimeout(double __value) {
idleWebSocketTimeout = __value;
return this;
}
private Builder idleWebSocketTimeout(String __value) {
return idleWebSocketTimeout(Double.valueOf(__value));
}
public Builder requestTimeout(double __value) {
requestTimeout = __value;
return this;
}
private Builder requestTimeout(String __value) {
return requestTimeout(Double.valueOf(__value));
}
public Builder ssl(Ssl.Builder __builder) {
ssl = __builder;
return this;
}
/**
* Make a new builder and run the supplied function on it before adding it to the list
* @param __func lambda that modifies the given builder
* @return this builder
*/
public Builder ssl(java.util.function.Consumer __func) {
Ssl.Builder __inner = new Ssl.Builder();
__func.accept(__inner);
ssl = __inner;
return this;
}
private boolean _applyOnRestart = false;
@java.lang.Override
public final boolean dispatchGetConfig(ConfigInstance.Producer producer) {
if (producer instanceof Producer) {
((Producer)producer).getConfig(this);
return true;
}
return false;
}
@java.lang.Override
public final String getDefMd5() { return CONFIG_DEF_MD5; }
@java.lang.Override
public final String getDefName() { return CONFIG_DEF_NAME; }
@java.lang.Override
public final String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
@java.lang.Override
public final boolean getApplyOnRestart() { return _applyOnRestart; }
@java.lang.Override
public final void setApplyOnRestart(boolean applyOnRestart) { _applyOnRestart = applyOnRestart; }
public HttpClientConfig build() {
return new HttpClientConfig(this);
}
}
private final StringNode userAgent;
private final BooleanNode chunkedEncodingEnabled;
private final BooleanNode compressionEnabled;
private final BooleanNode connectionPoolEnabled;
private final BooleanNode followRedirects;
private final BooleanNode removeQueryParamsOnRedirect;
private final BooleanNode sslConnectionPoolEnabled;
private final StringNode proxyServer;
private final BooleanNode useProxyProperties;
private final BooleanNode useRawUri;
private final IntegerNode compressionLevel;
private final IntegerNode maxNumConnections;
private final IntegerNode maxNumConnectionsPerHost;
private final IntegerNode maxNumRedirects;
private final IntegerNode maxNumRetries;
private final DoubleNode connectionTimeout;
private final DoubleNode idleConnectionInPoolTimeout;
private final DoubleNode idleConnectionTimeout;
private final DoubleNode idleWebSocketTimeout;
private final DoubleNode requestTimeout;
private final Ssl ssl;
public HttpClientConfig(Builder builder) {
this(builder, true);
}
private HttpClientConfig(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"http-client must be initialized: " + builder.__uninitialized);
userAgent = (builder.userAgent == null) ?
new StringNode("JDisc/1.0") : new StringNode(builder.userAgent);
chunkedEncodingEnabled = (builder.chunkedEncodingEnabled == null) ?
new BooleanNode(false) : new BooleanNode(builder.chunkedEncodingEnabled);
compressionEnabled = (builder.compressionEnabled == null) ?
new BooleanNode(false) : new BooleanNode(builder.compressionEnabled);
connectionPoolEnabled = (builder.connectionPoolEnabled == null) ?
new BooleanNode(true) : new BooleanNode(builder.connectionPoolEnabled);
followRedirects = (builder.followRedirects == null) ?
new BooleanNode(false) : new BooleanNode(builder.followRedirects);
removeQueryParamsOnRedirect = (builder.removeQueryParamsOnRedirect == null) ?
new BooleanNode(true) : new BooleanNode(builder.removeQueryParamsOnRedirect);
sslConnectionPoolEnabled = (builder.sslConnectionPoolEnabled == null) ?
new BooleanNode(true) : new BooleanNode(builder.sslConnectionPoolEnabled);
proxyServer = (builder.proxyServer == null) ?
new StringNode("") : new StringNode(builder.proxyServer);
useProxyProperties = (builder.useProxyProperties == null) ?
new BooleanNode(false) : new BooleanNode(builder.useProxyProperties);
useRawUri = (builder.useRawUri == null) ?
new BooleanNode(false) : new BooleanNode(builder.useRawUri);
compressionLevel = (builder.compressionLevel == null) ?
new IntegerNode(-1) : new IntegerNode(builder.compressionLevel);
maxNumConnections = (builder.maxNumConnections == null) ?
new IntegerNode(-1) : new IntegerNode(builder.maxNumConnections);
maxNumConnectionsPerHost = (builder.maxNumConnectionsPerHost == null) ?
new IntegerNode(-1) : new IntegerNode(builder.maxNumConnectionsPerHost);
maxNumRedirects = (builder.maxNumRedirects == null) ?
new IntegerNode(5) : new IntegerNode(builder.maxNumRedirects);
maxNumRetries = (builder.maxNumRetries == null) ?
new IntegerNode(0) : new IntegerNode(builder.maxNumRetries);
connectionTimeout = (builder.connectionTimeout == null) ?
new DoubleNode(60D) : new DoubleNode(builder.connectionTimeout);
idleConnectionInPoolTimeout = (builder.idleConnectionInPoolTimeout == null) ?
new DoubleNode(60D) : new DoubleNode(builder.idleConnectionInPoolTimeout);
idleConnectionTimeout = (builder.idleConnectionTimeout == null) ?
new DoubleNode(60D) : new DoubleNode(builder.idleConnectionTimeout);
idleWebSocketTimeout = (builder.idleWebSocketTimeout == null) ?
new DoubleNode(15D) : new DoubleNode(builder.idleWebSocketTimeout);
requestTimeout = (builder.requestTimeout == null) ?
new DoubleNode(60D) : new DoubleNode(builder.requestTimeout);
ssl = new Ssl(builder.ssl, throwIfUninitialized);
}
/**
* @return http-client.userAgent
*/
public String userAgent() {
return userAgent.value();
}
/**
* @return http-client.chunkedEncodingEnabled
*/
public boolean chunkedEncodingEnabled() {
return chunkedEncodingEnabled.value();
}
/**
* @return http-client.compressionEnabled
*/
public boolean compressionEnabled() {
return compressionEnabled.value();
}
/**
* @return http-client.connectionPoolEnabled
*/
public boolean connectionPoolEnabled() {
return connectionPoolEnabled.value();
}
/**
* @return http-client.followRedirects
*/
public boolean followRedirects() {
return followRedirects.value();
}
/**
* @return http-client.removeQueryParamsOnRedirect
*/
public boolean removeQueryParamsOnRedirect() {
return removeQueryParamsOnRedirect.value();
}
/**
* @return http-client.sslConnectionPoolEnabled
*/
public boolean sslConnectionPoolEnabled() {
return sslConnectionPoolEnabled.value();
}
/**
* @return http-client.proxyServer
*/
public String proxyServer() {
return proxyServer.value();
}
/**
* @return http-client.useProxyProperties
*/
public boolean useProxyProperties() {
return useProxyProperties.value();
}
/**
* @return http-client.useRawUri
*/
public boolean useRawUri() {
return useRawUri.value();
}
/**
* @return http-client.compressionLevel
*/
public int compressionLevel() {
return compressionLevel.value();
}
/**
* @return http-client.maxNumConnections
*/
public int maxNumConnections() {
return maxNumConnections.value();
}
/**
* @return http-client.maxNumConnectionsPerHost
*/
public int maxNumConnectionsPerHost() {
return maxNumConnectionsPerHost.value();
}
/**
* @return http-client.maxNumRedirects
*/
public int maxNumRedirects() {
return maxNumRedirects.value();
}
/**
* @return http-client.maxNumRetries
*/
public int maxNumRetries() {
return maxNumRetries.value();
}
/**
* @return http-client.connectionTimeout
*/
public double connectionTimeout() {
return connectionTimeout.value();
}
/**
* @return http-client.idleConnectionInPoolTimeout
*/
public double idleConnectionInPoolTimeout() {
return idleConnectionInPoolTimeout.value();
}
/**
* @return http-client.idleConnectionTimeout
*/
public double idleConnectionTimeout() {
return idleConnectionTimeout.value();
}
/**
* @return http-client.idleWebSocketTimeout
*/
public double idleWebSocketTimeout() {
return idleWebSocketTimeout.value();
}
/**
* @return http-client.requestTimeout
*/
public double requestTimeout() {
return requestTimeout.value();
}
/**
* @return http-client.ssl
*/
public Ssl ssl() {
return ssl;
}
private ChangesRequiringRestart getChangesRequiringRestart(HttpClientConfig newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("http-client");
return changes;
}
private static boolean containsFieldsFlaggedWithRestart() {
return false;
}
/**
* This class represents http-client.ssl
*/
public final static class Ssl extends InnerNode {
public static final class Builder implements ConfigBuilder {
private Set __uninitialized = new HashSet();
private Boolean enabled = null;
private String keyStoreType = null;
private String keyStorePath = null;
private String trustStorePath = null;
private String keyDBKey = null;
private String algorithm = null;
private String protocol = null;
public Builder() { }
public Builder(Ssl config) {
enabled(config.enabled());
keyStoreType(config.keyStoreType());
keyStorePath(config.keyStorePath());
trustStorePath(config.trustStorePath());
keyDBKey(config.keyDBKey());
algorithm(config.algorithm());
protocol(config.protocol());
}
private Builder override(Builder __superior) {
if (__superior.enabled != null)
enabled(__superior.enabled);
if (__superior.keyStoreType != null)
keyStoreType(__superior.keyStoreType);
if (__superior.keyStorePath != null)
keyStorePath(__superior.keyStorePath);
if (__superior.trustStorePath != null)
trustStorePath(__superior.trustStorePath);
if (__superior.keyDBKey != null)
keyDBKey(__superior.keyDBKey);
if (__superior.algorithm != null)
algorithm(__superior.algorithm);
if (__superior.protocol != null)
protocol(__superior.protocol);
return this;
}
public Builder enabled(boolean __value) {
enabled = __value;
return this;
}
private Builder enabled(String __value) {
return enabled(Boolean.valueOf(__value));
}
public Builder keyStoreType(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
keyStoreType = __value;
return this;
}
public Builder keyStorePath(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
keyStorePath = __value;
return this;
}
public Builder trustStorePath(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
trustStorePath = __value;
return this;
}
public Builder keyDBKey(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
keyDBKey = __value;
return this;
}
public Builder algorithm(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
algorithm = __value;
return this;
}
public Builder protocol(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
protocol = __value;
return this;
}
public Ssl build() {
return new Ssl(this);
}
}
private final BooleanNode enabled;
private final StringNode keyStoreType;
// Vespa home is prepended is path is relative
private final StringNode keyStorePath;
// Vespa home is prepended is path is relative
private final StringNode trustStorePath;
private final StringNode keyDBKey;
private final StringNode algorithm;
private final StringNode protocol;
public Ssl(Builder builder) {
this(builder, true);
}
private Ssl(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"http-client.ssl must be initialized: " + builder.__uninitialized);
enabled = (builder.enabled == null) ?
new BooleanNode(false) : new BooleanNode(builder.enabled);
keyStoreType = (builder.keyStoreType == null) ?
new StringNode("JKS") : new StringNode(builder.keyStoreType);
keyStorePath = (builder.keyStorePath == null) ?
new StringNode("jdisc_container/keyStore.jks") : new StringNode(builder.keyStorePath);
trustStorePath = (builder.trustStorePath == null) ?
new StringNode("conf/jdisc_container/trustStore.jks") : new StringNode(builder.trustStorePath);
keyDBKey = (builder.keyDBKey == null) ?
new StringNode("jdisc_container") : new StringNode(builder.keyDBKey);
algorithm = (builder.algorithm == null) ?
new StringNode("SunX509") : new StringNode(builder.algorithm);
protocol = (builder.protocol == null) ?
new StringNode("TLS") : new StringNode(builder.protocol);
}
/**
* @return http-client.ssl.enabled
*/
public boolean enabled() {
return enabled.value();
}
/**
* @return http-client.ssl.keyStoreType
*/
public String keyStoreType() {
return keyStoreType.value();
}
/**
* @return http-client.ssl.keyStorePath
*/
public String keyStorePath() {
return keyStorePath.value();
}
/**
* @return http-client.ssl.trustStorePath
*/
public String trustStorePath() {
return trustStorePath.value();
}
/**
* @return http-client.ssl.keyDBKey
*/
public String keyDBKey() {
return keyDBKey.value();
}
/**
* @return http-client.ssl.algorithm
*/
public String algorithm() {
return algorithm.value();
}
/**
* @return http-client.ssl.protocol
*/
public String protocol() {
return protocol.value();
}
private ChangesRequiringRestart getChangesRequiringRestart(Ssl newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("ssl");
return changes;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy